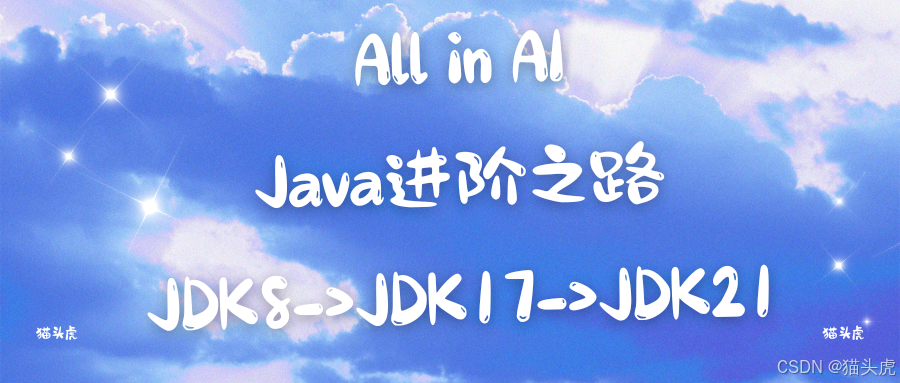
Instant与Duration:什么是时间戳和时间间隔?如何在开发中使用?
Instant与Duration:什么是时间戳和时间间隔?如何在开发中使用?粉丝提问:在Java开发中,Instant和Duration的具体作用是什么?如何用它们高效处理时间戳和时间间隔?本文将详细讲解Instant(时间戳)和Duration(时间间隔)的核心概念,配合代码示例演示如何在开发中灵活应用。
Instant与Duration:什么是时间戳和时间间隔?如何在开发中使用?
粉丝提问:
在Java开发中,
Instant
和Duration
的具体作用是什么?如何用它们高效处理时间戳和时间间隔?
本文将详细讲解Instant
(时间戳)和Duration
(时间间隔)的核心概念,配合代码示例演示如何在开发中灵活应用。
作者简介
猫头虎是谁?
大家好,我是 猫头虎,猫头虎技术团队创始人,也被大家称为猫哥。我目前是COC北京城市开发者社区主理人、COC西安城市开发者社区主理人,以及云原生开发者社区主理人,在多个技术领域如云原生、前端、后端、运维和AI都具备丰富经验。
我的博客内容涵盖广泛,主要分享技术教程、Bug解决方案、开发工具使用方法、前沿科技资讯、产品评测、产品使用体验,以及产品优缺点分析、横向对比、技术沙龙参会体验等。我的分享聚焦于云服务产品评测、AI产品对比、开发板性能测试和技术报告。
目前,我活跃在CSDN、51CTO、腾讯云、阿里云开发者社区、华为云开发者社区、知乎、微信公众号、视频号、抖音、B站、小红书等平台,全网粉丝已超过30万。我所有平台的IP名称统一为猫头虎或猫头虎技术团队。
我希望通过我的分享,帮助大家更好地掌握和使用各种技术产品,提升开发效率与体验。
作者名片 ✍️
- 博主:猫头虎
- 全网搜索关键词:猫头虎
- 作者微信号:Libin9iOak
- 作者公众号:猫头虎技术团队
- 更新日期:2024年12月16日
- 🌟 欢迎来到猫头虎的博客 — 探索技术的无限可能!
加入我们AI共创团队 🌐
- 猫头虎AI共创社群矩阵列表:
加入猫头虎的共创圈,一起探索编程世界的无限可能! 🚀
正文
一、什么是Instant
和Duration
?
1. Instant
:时间戳
- 定义:
Instant
表示从 1970-01-01T00:00:00Z(UTC时间)开始的时间点。 - 特点:
- 精确到纳秒。
- 适合表示绝对时间点,常用于计算时间间隔或记录操作时间。
2. Duration
:时间间隔
- 定义:
Duration
表示两个时间点之间的间隔,精确到秒和纳秒。 - 特点:
- 用于表示绝对时间间隔。
- 适合进行时间计算和性能分析。
二、Instant
的常见用法
1. 获取当前时间戳
import java.time.Instant;
public class InstantExample {
public static void main(String[] args) {
Instant now = Instant.now();
System.out.println("当前时间戳:" + now);
}
}
2. 自定义时间戳
import java.time.Instant;
public class CustomInstantExample {
public static void main(String[] args) {
Instant specificTime = Instant.ofEpochSecond(1672531200); // 秒级时间戳
System.out.println("自定义时间戳:" + specificTime);
}
}
3. 比较时间
import java.time.Instant;
public class CompareInstantExample {
public static void main(String[] args) {
Instant time1 = Instant.now();
Instant time2 = time1.plusSeconds(10);
System.out.println("time1 是否在 time2 之前? " + time1.isBefore(time2));
System.out.println("time1 是否在 time2 之后? " + time1.isAfter(time2));
}
}
三、Duration
的常见用法
1. 计算两个时间点之间的间隔
import java.time.Duration;
import java.time.Instant;
public class DurationBetweenExample {
public static void main(String[] args) {
Instant start = Instant.now();
// 模拟延时
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
Instant end = Instant.now();
Duration duration = Duration.between(start, end);
System.out.println("操作耗时:" + duration.toMillis() + " 毫秒");
}
}
2. 创建指定间隔的Duration
import java.time.Duration;
public class SpecificDurationExample {
public static void main(String[] args) {
Duration duration = Duration.ofMinutes(5);
System.out.println("间隔时间:" + duration.getSeconds() + " 秒");
}
}
3. 加减时间间隔
import java.time.Duration;
import java.time.Instant;
public class DurationArithmeticExample {
public static void main(String[] args) {
Instant now = Instant.now();
Duration duration = Duration.ofHours(2);
Instant twoHoursLater = now.plus(duration);
Instant twoHoursAgo = now.minus(duration);
System.out.println("当前时间:" + now);
System.out.println("两小时后:" + twoHoursLater);
System.out.println("两小时前:" + twoHoursAgo);
}
}
四、Instant
与Duration
的组合应用
1. 计算程序运行时间
import java.time.Duration;
import java.time.Instant;
public class ExecutionTimeExample {
public static void main(String[] args) {
Instant start = Instant.now();
// 模拟任务
try {
Thread.sleep(1500);
} catch (InterruptedException e) {
e.printStackTrace();
}
Instant end = Instant.now();
Duration executionTime = Duration.between(start, end);
System.out.println("程序运行时间:" + executionTime.toMillis() + " 毫秒");
}
}
2. 模拟任务延迟
import java.time.Instant;
import java.time.Duration;
public class SimulateDelayExample {
public static void main(String[] args) {
Instant start = Instant.now();
System.out.println("任务开始时间:" + start);
Duration delay = Duration.ofSeconds(3);
Instant delayedTime = start.plus(delay);
System.out.println("预计结束时间:" + delayedTime);
}
}
五、常见问题 Q&A
Q:Instant
和LocalDateTime
有什么区别?
A:
Instant
:表示从1970-01-01T00:00:00Z开始的绝对时间点,适合记录操作时间或计算时间间隔。LocalDateTime
:表示本地时间,不含时区信息,适合表示用户日常时间。
Q:如何将秒级时间戳转换为Instant
?
A:使用Instant.ofEpochSecond(long)
方法。
Q:Duration
可以用于日期间隔计算吗?
A:Duration
适合处理以秒和纳秒为单位的时间间隔。如果需要以天、月或年为单位,推荐使用Period
。
六、总结
Instant
与Duration
的核心优势:
Instant
是绝对时间点,适合时间戳操作和比较。Duration
是绝对时间间隔,适合性能分析和时间计算。- 它们共同组成了Java 8时间API的重要工具,简化了开发中的时间操作。
想了解更多Java时间工具类用法?快加入猫头虎的AI技术社群,与60万开发者共同成长!
🚀 提问你的技术问题,和猫头虎一起探索更高效的解决方案!
粉丝福利
👉 更多信息:有任何疑问或者需要进一步探讨的内容,欢迎点击文末名片获取更多信息。我是猫头虎,期待与您的交流! 🦉💬
🌐 第一板块:
- 链接:[直达链接]https://zhaimengpt1.kimi.asia/list
💳 第二板块:最稳定的AI全平台可支持平台
- 链接:[粉丝直达链接]https://bewildcard.com/?code=CHATVIP
联系我与版权声明 📩
- 联系方式:
- 微信: Libin9iOak
- 公众号: 猫头虎技术团队
- 版权声明:
本文为原创文章,版权归作者所有。未经许可,禁止转载。更多内容请访问猫头虎的博客首页。
点击✨⬇️下方名片
⬇️✨,加入猫头虎AI共创社群,交流AI新时代变现的无限可能。一起探索科技的未来,共同成长。🚀
更多推荐
所有评论(0)