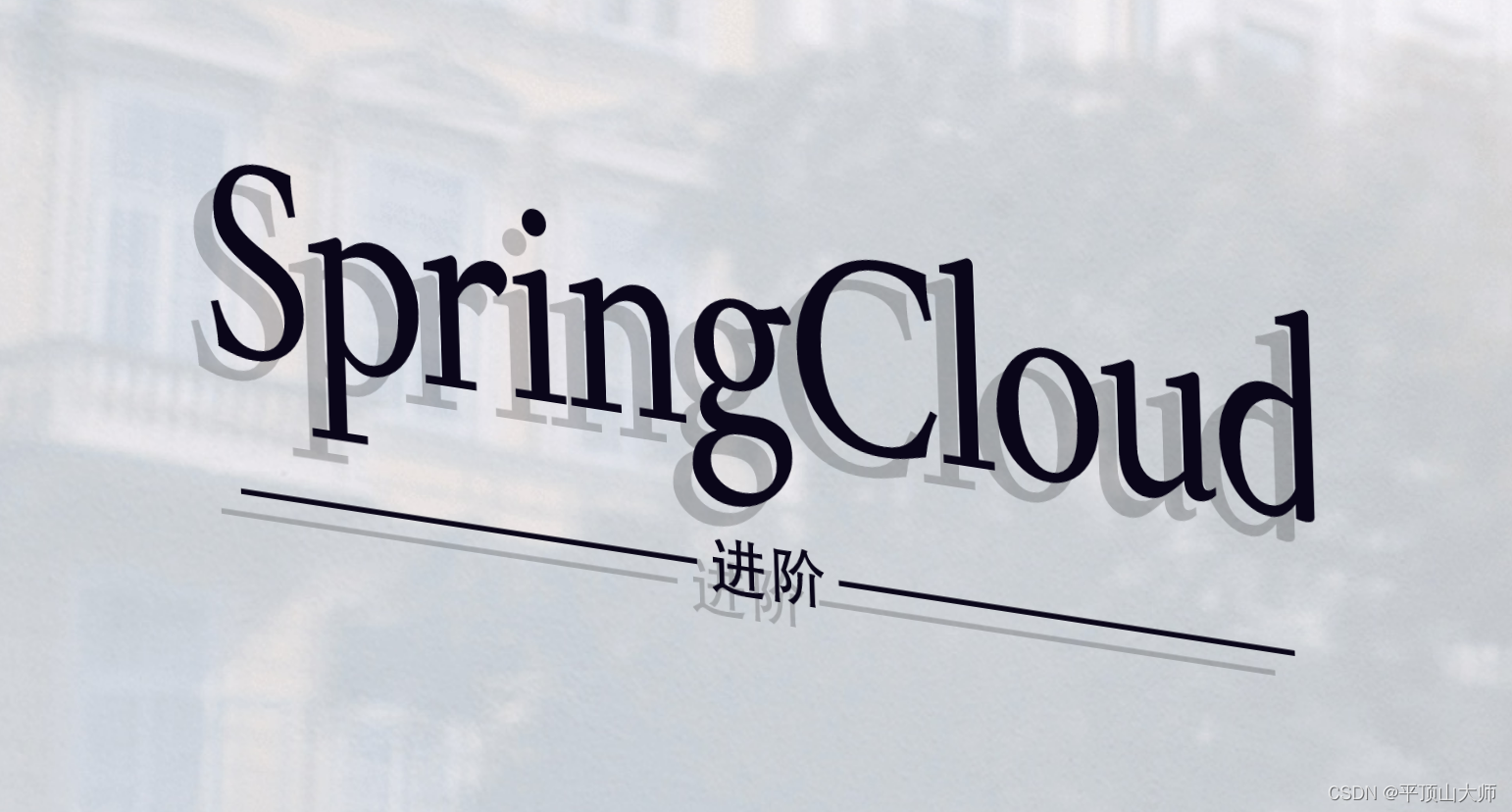
【SpringCloud】之远程消费(进阶使用)以及问题The bean ‘provider.FeignClientSpecification‘ could not be registered.
1. 概述在 Spring Cloud 中,远程消费通常指的是一个微服务通过网络调用另一个微服务的接口,以实现跨服务的功能调用。这种跨服务的通信方式常见于分布式系统中,其中不同的微服务协同工作以完成更大的业务目标。在微服务架构中,各个微服务通常拥有独立的代码库和部署单元。今天遇到一个问题在这里记录一下:原因大概是Spring不允许同名的bean定义进行覆盖,相当于只有一个可以调用要做一个限制:接着
目录
🎉🎉欢迎来到我的CSDN主页!🎉🎉
🏅我是平顶山大师,一个在CSDN分享笔记的博主。📚📚
🌟推荐给大家我的博客专栏《【SpringCloud】之远程消费(进阶使用)》。🎯🎯
🎁如果感觉还不错的话请给我关注加三连吧!🎁🎁
前言
在上一期的SpringCloud的分享中我们对其SpringCloud有了一个初步的了解,在上一期我们一起学习了什么是SpringCloud、详细的讲述了SpringCloud核心组件在业务中的一些用法以及作用,最后我们还涉及到了nacos在SpringCloud中的集成使用。本期的博客分享基于上期的博客进行一个远程消费的一个分享
一、 什么是远程消费
1. 概述
在 Spring Cloud 中,远程消费通常指的是一个微服务通过网络调用另一个微服务的接口,以实现跨服务的功能调用。这种跨服务的通信方式常见于分布式系统中,其中不同的微服务协同工作以完成更大的业务目标。在微服务架构中,各个微服务通常拥有独立的代码库和部署单元。
二, 实现方式
2.1 使用RestTemplate
2.1 RestTemplate
RestTemplate 是 Spring 提供的一个用于访问 REST 服务的模板类。通过 RestTemplate,你可以发起 HTTP 请求,从而实现对其他微服务提供的 RESTful API 的调用。在 Spring Cloud 中,可以通过使用 @LoadBalanced 注解来启用负载均衡,使得 RestTemplate 可以根据服务名称自动选择合适的实例。
@Bean @LoadBalanced public RestTemplate restTemplate() { return new RestTemplate(); }
2.2,使用Feign(开启负载均衡)
步骤:
2.2.1在公共类中引入依赖:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency>
2.2.2标记使用远程消费
我们在需要远程消费的服务的启动类上打上注解进行标记说明该服务启用远程消费。注解:@EnableFeignClients
该注解是开启Feign功能,我们的消费者需要启用,因此在我们的消费者的启动类上打上其注解。
生产者编写接口
我们需要模拟远程消费,对应的生产者是不是应该编写一个Controller去提供一些接口让我们的消费者进行调用。在编写的过程中只要参数是复杂对象,即使指定了是GET方法,feign依然会以POST方法进行发送请求,同时生产者必 须支持POST请求并给参数添加@RequestBody注解
// 路径接收参数的请求 @RequestMapping("/{account}") public String getByPath(@PathVariable("account") String account){ log.info("account:{}",account);//打印输出查看 return "🍗"; }
2.2.2 接收多个参数
//RequestParam是从前台传来 @RequestMapping("/param") public String getbyParam(@RequestParam("account") String account , @RequestParam("password") String password) { log.info("account:{},password:{}",account,password); return "🍗"; }
2.2.3 使用对象接收
//RequestParam是从前台传来 @RequestMapping("/pojo") public String getbypojo(UserDto userDto) { User user = new User(); BeanUtils.copyProperties(userDto,user); // 1, user.setInetAddress(userDto.getEmail()); UserDto userVo = new UserDto(); // userVo.setEmail("test@qq.com"); // factory.classMap(UserVo.class, User.class) // .field("inetAddress", "email") // .byDefault().register(); // User u = factory.getMapperFacade().map(userVo, User.class) log.info("user:{}",user); return "🍗"; }
2.2.4 使用Map接收
//RequestParam是从前台传来 @RequestMapping("/map") public String mp(Map<String, Objects> map) { log.info("map:{}",map); return "🍗"; }
3. 编写 FeginService.java
如果我们的消费者还是使用上期博客中的那种方式去调用的话不方便,也不好。因此我们可以在消费者编写一个接口类用于调用。
FeginService.java
package com.lya.consumer.service; import com.lya.consumer.pojo.User; import dto.UserDto; import org.springframework.cloud.openfeign.FeignClient; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import java.util.Map; /** * @author 程序猿-小李哥 * @site www.xiaolige.com * @company 猪八戒有限集团 * @create 2024-01-06-15:38 */ //从这个类可以调到provider方法 @FeignClient("provider") @RequestMapping("/user") public interface FeignUserService { @RequestMapping("/account") String getbypath(@PathVariable("account") String account); @RequestMapping("/param") String getbyParam(@RequestParam("account") String account , @RequestParam("password") String password); //RequestParam是从前台传来 @RequestMapping("/pojo") String getbypojo(@RequestParam("user") UserDto user); //RequestParam是从前台传来 @RequestMapping("/map") String map(@RequestParam("map") Map map); }
4. 编写消费者的Controller
重新编写请求方法进行调用生产者的方法
package com.lya.consumer; import com.lya.consumer.pojo.User; import com.lya.consumer.service.FeignUserService; import dto.UserDto; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.openfeign.EnableFeignClients; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import java.util.HashMap; import java.util.Map; /** * @author 微微 */ @RestController @EnableDiscoveryClient @EnableFeignClients public class ConsumerController { @Autowired private FeignUserService feginUserService; @RequestMapping("/test01") public String run() { return feginUserService.getbypath("root"); } @RequestMapping("/test02") public String test02() { return feginUserService.getbyParam("root","123"); } @RequestMapping("/test03") public String test03() { return feginUserService.getbypojo(new UserDto(1l,"孤流光姬","1","5466")); } @RequestMapping("/test04") public String test04() { Map map = new HashMap<String,Object>(); map.put("id","100"); map.put("身份","码农"); return feginUserService.map(map); } }
DTO封装:
VO(View Object):视图对象,用于展示层,它的作用是把某个指定页面(或组件)的所有数据 封装起来。 DTO(Data Transfer Object):数据传输对象,这个概念来源于J2EE的设计模式,原来的目的是 为了EJB的分布式应用提供粗粒度的数据实体,以减少分布式调用的次数,从而提高分布式调用的 性能和降低网络负载,但在这里,我泛指用于展示层与服务层之间的数据传输对象。 DO(Domain Object):领域对象,就是从现实世界中抽象出来的有形或无形的业务实体。 PO(Persistent Object):持久化对象,它跟持久层(通常是关系型数据库)的数据结构形成一 一对应的映射关系,如果持久层是关系型数据库,那么,数据表中的每个字段(或若干个)就对应 PO的一个(或若干个)属性。
package dto; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; /** * @author 微微 */ @Data @AllArgsConstructor @NoArgsConstructor public class UserDto { private long id; private String account; private String password; private String email; }
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <properties> <spring-boot.version>2.4.1</spring-boot.version> <spring-cloud.version>2020.0.0</spring-cloud.version> <spring-cloud-alibaba.version>2021.1</spring-cloud-alibaba.version> </properties> <artifactId>common</artifactId> <packaging>jar</packaging> <name>common Maven Webapp</name> <url>http://maven.apache.org</url> <!-- 继承--> <parent> <groupId>org.example</groupId> <artifactId>SpringCould1</artifactId> <version>1.0-SNAPSHOT</version> </parent> </project>
生产者,消费者引用:
可以调用了
三,The bean 'provider.FeignClientSpecification' could not be registered. A bean with that name has already been defined and overriding is disabled.的解决
今天遇到一个问题在这里记录一下:原因大概是Spring不允许同名的bean定义进行覆盖,相当于只有一个可以调用要做一个限制:接着就是重新启动项目,再不行就重启idea
解决问题:
The bean 'provider.FeignClientSpecification' could not be registered. A bean with that name has already been defined and overriding is disabled.
main: allow-bean-definition-overriding: true
更多推荐
所有评论(0)