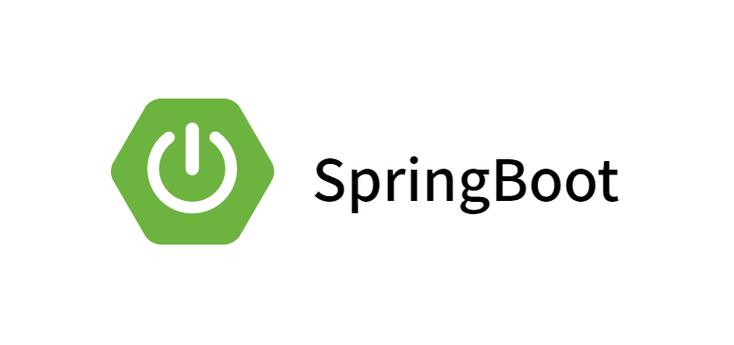
springboot基础学习 之 使用Spring Cloud进行服务注册与发现。
使用 Spring Cloud 进行服务注册与发现通常涉及使用 Eureka 作为注册中心。以下是一些基本步骤,通过这些步骤,你可以在微服务架构中实现服务的注册与发现。
使用 Spring Cloud 进行服务注册与发现通常涉及使用 Eureka 作为注册中心。以下是一些基本步骤,通过这些步骤,你可以在微服务架构中实现服务的注册与发现。
步骤:
通过以上步骤,你可以在 Spring Cloud 中实现服务注册与发现,使得微服务能够动态地发现和调用彼此,从而实现了分布式系统的协同工作。确保理解和遵循 Spring Cloud 的最佳实践以确保系统的稳定性和性能。
-
添加依赖: 在你的 Spring Boot 项目中,通过 Maven 或 Gradle 添加 Spring Cloud Eureka 依赖。
Maven 依赖:
xmlCopy code
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency>
或者使用 Gradle:
groovyCopy code
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-server'
-
配置文件: 在你的 Eureka 服务端应用程序的配置文件(
application.yml
或application.properties
)中,添加以下配置:yamlCopy code
spring: application: name: eureka-server server: port: 8761 eureka: client: register-with-eureka: false fetch-registry: false
在上述配置中,
register-with-eureka
和fetch-registry
设置为false
表示这是一个 Eureka 服务器,而不是普通的 Eureka 客户端。 -
启动类: 在你的 Eureka 服务端应用程序的启动类上添加
@EnableEurekaServer
注解。javaCopy code
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @SpringBootApplication @EnableEurekaServer public class EurekaServerApplication { public static void main(String[] args) { SpringApplication.run(EurekaServerApplication.class, args); } }
-
启动应用程序: 启动 Eureka 服务器应用程序。访问
http://localhost:8761
可以看到 Eureka 的管理界面,其中会显示注册的服务信息。 -
添加 Eureka 客户端: 对于每个微服务项目,添加 Eureka 客户端依赖。
Maven 依赖:
xmlCopy code
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency>
Gradle:
groovyCopy code
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-client'
-
客户端配置文件: 在每个微服务应用程序的配置文件中,配置 Eureka 客户端。
yamlCopy code
spring: application: name: your-service-name eureka: client: service-url: defaultZone: http://localhost:8761/eureka/
其中,
your-service-name
是你的微服务的名称。 -
启动客户端应用程序: 启动每个微服务应用程序,它们将自动注册到 Eureka 服务器,并在 Eureka 管理界面中显示。
-
查看服务注册情况: 访问 Eureka 管理界面(http://localhost:8761),你将看到注册的服务列表和它们的状态。
-
服务发现和调用: 在需要调用其他服务的微服务中,使用 Spring Cloud 提供的
@LoadBalanced
注解和RestTemplate
实现服务的发现和调用。javaCopy code
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.cloud.client.loadbalancer.LoadBalanced; import org.springframework.context.annotation.Bean; import org.springframework.stereotype.Service; import org.springframework.web.client.RestTemplate; @Service public class MyService { @Autowired private RestTemplate restTemplate; public String callOtherService() { // 使用服务名调用其他微服务 String result = restTemplate.getForObject("http://your-service-name/your-endpoint", String.class); return "Result from other service: " + result; } @Bean @LoadBalanced public RestTemplate restTemplate() { return new RestTemplate(); } }
上述代码中,
@LoadBalanced
注解启用了负载均衡功能,RestTemplate
可以通过服务名进行调用。确保在需要发起服务调用的微服务中注入了RestTemplate
实例。 -
服务间通信: 确保微服务之间的通信遵循服务名而不是硬编码的方式。这使得服务的位置可以通过服务注册表的动态更新而不需要手动更改配置。
javaCopy code
@RestController public class MyController { @Autowired private MyService myService; @GetMapping("/my-endpoint") public String myEndpoint() { // 调用其他微服务 return myService.callOtherService(); } }
在微服务的控制器中,通过调用
MyService
中的方法来发起对其他服务的调用。 -
多实例和负载均衡: 当多个实例注册到 Eureka 服务器时,Eureka 将自动实现负载均衡。
@LoadBalanced
注解使得RestTemplate
在调用其他服务时会自动选择一个可用的实例。 -
安全性: 如果你的服务需要安全性,考虑使用 Spring Cloud Security 或其他身份验证和授权的机制来保护服务之间的通信。
-
集群部署: 当你需要部署多个 Eureka 服务器时,确保它们互相感知,以实现高可用性。配置 Eureka 客户端的
defaultZone
属性时,使用所有 Eureka 服务器的地址。yamlCopy code
eureka: client: service-url: defaultZone: http://eureka-server1:8761/eureka/,http://eureka-server2:8762/eureka/
上述配置中,
eureka-server1
和eureka-server2
是两个 Eureka 服务器的地址。
更多推荐
所有评论(0)