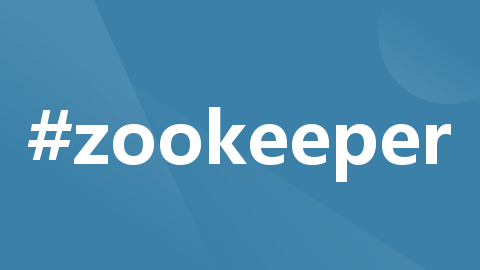
Zookeeper(96)如何在Zookeeper中实现服务发现?
服务发现是分布式系统中的一个关键组件,用于动态地发现和跟踪不同服务的实例。ZooKeeper 可以通过其节点存储和事件监听机制来实现服务发现,确保系统中的各个节点能够实时获取可用服务的列表。
·
服务发现是分布式系统中的一个关键组件,用于动态地发现和跟踪不同服务的实例。ZooKeeper 可以通过其节点存储和事件监听机制来实现服务发现,确保系统中的各个节点能够实时获取可用服务的列表。
实现原理
- 服务注册:每个服务实例在ZooKeeper中创建一个临时节点,表示该实例的存在。
- 服务发现:客户端监听特定路径下的子节点变化,从而获取当前可用的服务实例列表。
- 故障检测:由于服务实例创建的是临时节点,当实例故障或断开连接时,ZooKeeper会自动删除该节点,客户端会收到通知并更新服务列表。
代码示例
以下是一个实现服务发现的代码示例,展示了如何在ZooKeeper中实现一个简单而有效的服务发现系统。
依赖导入
首先,确保你已经导入了ZooKeeper的Java客户端库:
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.7.0</version>
</dependency>
服务发现实现
import org.apache.zookeeper.*;
import org.apache.zookeeper.data.Stat;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.CountDownLatch;
import java.util.stream.Collectors;
public class ServiceDiscovery implements Watcher {
private ZooKeeper zooKeeper;
private String registryPath;
private CountDownLatch connectedSignal = new CountDownLatch(1);
public ServiceDiscovery(String connectString, String registryPath) throws IOException, InterruptedException {
this.zooKeeper = new ZooKeeper(connectString, 3000, this);
this.registryPath = registryPath;
connectedSignal.await();
ensureRegistryPath();
}
private void ensureRegistryPath() {
try {
Stat stat = zooKeeper.exists(registryPath, false);
if (stat == null) {
zooKeeper.create(registryPath, new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
}
} catch (KeeperException | InterruptedException e) {
e.printStackTrace();
}
}
public void registerService(String serviceName, String serviceAddress) {
try {
String servicePath = registryPath + "/" + serviceName;
Stat stat = zooKeeper.exists(servicePath, false);
if (stat == null) {
zooKeeper.create(servicePath, new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
}
String instancePath = servicePath + "/instance_";
zooKeeper.create(instancePath, serviceAddress.getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL);
} catch (KeeperException | InterruptedException e) {
e.printStackTrace();
}
}
public List<String> discoverServices(String serviceName) {
try {
String servicePath = registryPath + "/" + serviceName;
List<String> children = zooKeeper.getChildren(servicePath, this);
return children.stream()
.map(child -> {
try {
byte[] data = zooKeeper.getData(servicePath + "/" + child, false, null);
return new String(data);
} catch (KeeperException | InterruptedException e) {
e.printStackTrace();
return null;
}
})
.collect(Collectors.toList());
} catch (KeeperException | InterruptedException e) {
e.printStackTrace();
}
return null;
}
@Override
public void process(WatchedEvent event) {
if (event.getState() == Event.KeeperState.SyncConnected) {
connectedSignal.countDown();
} else if (event.getType() == Event.EventType.NodeChildrenChanged) {
System.out.println("Service registry changed, discovering services again...");
// Re-discover services if needed
}
}
public static void main(String[] args) throws Exception {
ServiceDiscovery serviceDiscovery = new ServiceDiscovery("localhost:2181", "/services");
// Simulate service registration
serviceDiscovery.registerService("exampleService", "192.168.1.1:8080");
serviceDiscovery.registerService("exampleService", "192.168.1.2:8080");
// Simulate service discovery
List<String> services = serviceDiscovery.discoverServices("exampleService");
System.out.println("Discovered services: " + services);
// Keep the application running to listen for service changes
Thread.sleep(Long.MAX_VALUE);
}
}
详细说明
-
初始化ZooKeeper客户端:
public ServiceDiscovery(String connectString, String registryPath) throws IOException, InterruptedException { this.zooKeeper = new ZooKeeper(connectString, 3000, this); this.registryPath = registryPath; connectedSignal.await(); ensureRegistryPath(); }
在初始化时,连接到ZooKeeper服务器,并确保注册路径存在。如果节点不存在,则创建一个持久节点表示注册路径。
-
确保注册路径存在:
private void ensureRegistryPath() { try { Stat stat = zooKeeper.exists(registryPath, false); if (stat == null) { zooKeeper.create(registryPath, new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT); } } catch (KeeperException | InterruptedException e) { e.printStackTrace(); } }
检查注册路径是否存在,如果不存在,则创建一个持久节点。
-
注册服务:
public void registerService(String serviceName, String serviceAddress) { try { String servicePath = registryPath + "/" + serviceName; Stat stat = zooKeeper.exists(servicePath, false); if (stat == null) { zooKeeper.create(servicePath, new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT); } String instancePath = servicePath + "/instance_"; zooKeeper.create(instancePath, serviceAddress.getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL); } catch (KeeperException | InterruptedException e) { e.printStackTrace(); } }
每个服务实例在注册路径下创建一个临时顺序节点,表示该实例的存在,并存储服务地址。
-
发现服务:
public List<String> discoverServices(String serviceName) { try { String servicePath = registryPath + "/" + serviceName; List<String> children = zooKeeper.getChildren(servicePath, this); return children.stream() .map(child -> { try { byte[] data = zooKeeper.getData(servicePath + "/" + child, false, null); return new String(data); } catch (KeeperException | InterruptedException e) { e.printStackTrace(); return null; } }) .collect(Collectors.toList()); } catch (KeeperException | InterruptedException e) { e.printStackTrace(); } return null; }
获取注册路径下的所有子节点,并获取每个子节点的数据(服务地址),返回当前可用的服务实例列表。
-
事件处理:
@Override public void process(WatchedEvent event) { if (event.getState() == Event.KeeperState.SyncConnected) { connectedSignal.countDown(); } else if (event.getType() == Event.EventType.NodeChildrenChanged) { System.out.println("Service registry changed, discovering services again..."); // Re-discover services if needed } }
当ZooKeeper客户端连接建立时,释放连接信号。当注册路径下的子节点发生变化时,打印消息并重新发现服务。
-
主函数:
public static void main(String[] args) throws Exception { ServiceDiscovery serviceDiscovery = new ServiceDiscovery("localhost:2181", "/services"); // Simulate service registration serviceDiscovery.registerService("exampleService", "192.168.1.1:8080"); serviceDiscovery.registerService("exampleService", "192.168.1.2:8080"); // Simulate service discovery List<String> services = serviceDiscovery.discoverServices("exampleService"); System.out.println("Discovered services: " + services); // Keep the application running to listen for service changes Thread.sleep(Long.MAX_VALUE); }
主函数创建一个服务发现管理器,并模拟服务注册和发现操作,同时保持应用程序运行以监听服务变化。
性能优化建议
-
异步操作:
- 使用ZooKeeper的异步API,减少同步阻塞,提高并发性能。
-
批处理操作:
- 可以通过一次性读取多个节点的状态来减少网络请求的次数,提高性能。
-
本地缓存:
- 在客户端实现本地缓存,减少频繁的读请求,提升系统性能。
通过合理的设计和实现,ZooKeeper可以有效地解决分布式服务发现的需求,确保系统的高可用性和一致性。
推荐内容
点击阅读全文
更多推荐
社区排行榜
Go语言基础
活动日历
查看更多
直播时间 2022-05-31 13:51:24
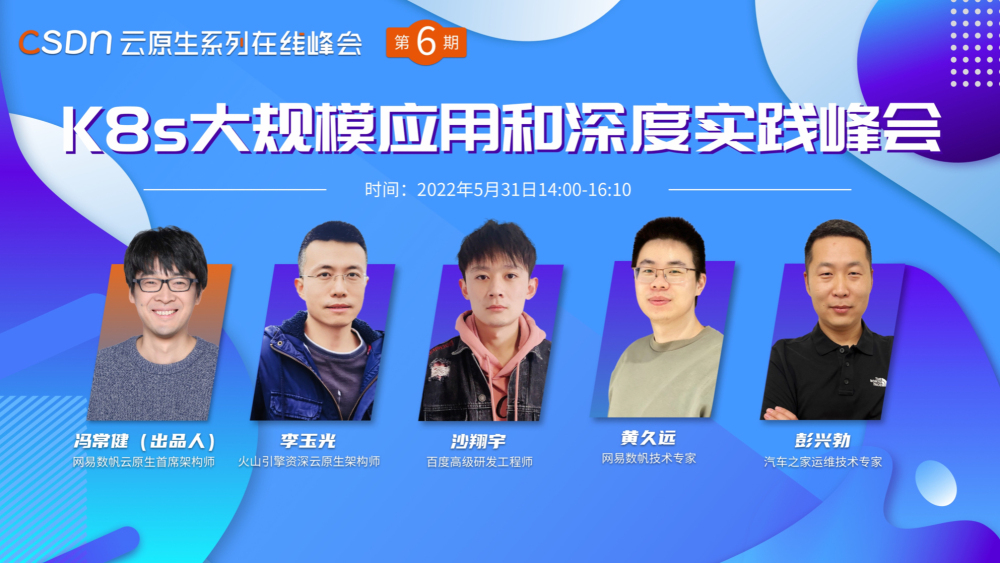
CSDN云原生系列在线峰会:K8s大规模应用和深度实践峰会
直播时间 2023-09-14 18:47:34

腾讯云优质活动用户线上技术漫谈
直播时间 2023-09-13 13:47:50

CloudOps云上自动化运维,助力云上业务高效、稳定运行
直播时间 2023-09-06 09:21:46

2023 Google 开发者大会 主旨演讲
直播时间 2023-08-31 18:49:52

TDSQL-C Serverless助力企业降本增效
所有评论(0)