【cocos creator】加载地图,保存地图,创建地图块,关卡游戏
写入地图数据,保存预制体要放在resource目录下// Learn cc.Class://- [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/class.html//- [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/class...
·
DEMO下载地址:https://download.csdn.net/download/K86338236/85912392
写入地图数据,保存js
预制体要放在resource目录下
// Learn cc.Class:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/class.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/class.html
// Learn Attribute:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/reference/attributes.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/reference/attributes.html
// Learn life-cycle callbacks:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/life-cycle-callbacks.html
// - [English] https://www.cocos2d-x.org/docs/creator/manual/en/scripting/life-cycle-callbacks.html
cc.Class({
extends: cc.Component,
properties: {
// foo: {
// // ATTRIBUTES:
// default: null, // The default value will be used only when the component attaching
// // to a node for the first time
// type: cc.SpriteFrame, // optional, default is typeof default
// serializable: true, // optional, default is true
// },
// bar: {
// get () {
// return this._bar;
// },
// set (value) {
// this._bar = value;
// }
// },
prefab: [cc.Prefab],//需要的地图元素
mapNode: cc.Node,
choose: cc.Node,
count: cc.EditBox,//第几关
},
// LIFE-CYCLE CALLBACKS:
onLoad() {
},
start() {
},
//判断选中哪种对象创建对应预制体
creatMap() {
var i = 0;
var children = this.choose.children;
for (let i = 0; i < children.length; i++) {
if (children[i].getComponent(cc.Toggle).isChecked) {
var map = cc.instantiate(this.prefab[i]);
map.parent = this.mapNode;
map.name = this.prefab[i].name;
break;
}
}
},
//保存地图数据
onSaveBtn() {
let items = this.mapNode.children;
cc.log(items)
let itemList = [];
for (let i = 0; i < items.length; i++) {
let item = items[i];
if (item.name.indexOf('MapItem') != -1) {
let strs = item.name.split('_');
let data = {};
data.name = strs[0];
data.x = parseInt(item.x);
data.y = parseInt(item.y);
data.angle = item.angle;
data.color = strs[1];
// cc.log(i + ':', data);
itemList.push(data);
}
}
var text = "GameMap_0";//保存的文件名
var index = parseInt(this.count.string);
if (index < 10) {
text += "0" + index + ".txt";
}
else {
text += index + ".txt";
}
this.downloadTextFile(JSON.stringify(itemList), text);
cc.log("保存成功");
},
//移除所有预制体
removeAll() {
this.mapNode.removeAllChildren();
},
//保存
downloadTextFile: function (content, filename) {
//content 为写入文件的内容,可以通过获取文本框的value写入
var file = new File([content], filename, { type: "text/plain;" }); //type: "text/plain;charset=utf-8"
require('FileSaver').saveAs(file);
},
update(dt) {
},
});
工具类,文件名:FileSaver.js
var saveAs = saveAs || (function (view) {
"use strict";
// IE <10 is explicitly unsupported
if (typeof view === "undefined" || typeof navigator !== "undefined" && /MSIE [1-9]\./.test(navigator.userAgent)) {
return;
}
var
doc = view.document
// only get URL when necessary in case Blob.js hasn't overridden it yet
, get_URL = function () {
return view.URL || view.webkitURL || view;
}
, save_link = doc.createElementNS("http://www.w3.org/1999/xhtml", "a")
, can_use_save_link = "download" in save_link
, click = function (node) {
var event = new MouseEvent("click");
node.dispatchEvent(event);
}
, is_safari = /constructor/i.test(view.HTMLElement) || view.safari
, is_chrome_ios = /CriOS\/[\d]+/.test(navigator.userAgent)
, throw_outside = function (ex) {
(view.setImmediate || view.setTimeout)(function () {
throw ex;
}, 0);
}
, force_saveable_type = "application/octet-stream"
// the Blob API is fundamentally broken as there is no "downloadfinished" event to subscribe to
, arbitrary_revoke_timeout = 1000 * 40 // in ms
, revoke = function (file) {
var revoker = function () {
if (typeof file === "string") { // file is an object URL
get_URL().revokeObjectURL(file);
} else { // file is a File
file.remove();
}
};
setTimeout(revoker, arbitrary_revoke_timeout);
}
, dispatch = function (filesaver, event_types, event) {
event_types = [].concat(event_types);
var i = event_types.length;
while (i--) {
var listener = filesaver["on" + event_types[i]];
if (typeof listener === "function") {
try {
listener.call(filesaver, event || filesaver);
} catch (ex) {
throw_outside(ex);
}
}
}
}
, auto_bom = function (blob) {
// prepend BOM for UTF-8 XML and text/* types (including HTML)
// note: your browser will automatically convert UTF-16 U+FEFF to EF BB BF
if (/^\s*(?:text\/\S*|application\/xml|\S*\/\S*\+xml)\s*;.*charset\s*=\s*utf-8/i.test(blob.type)) {
return new Blob([String.fromCharCode(0xFEFF), blob], { type: blob.type });
}
return blob;
}
, FileSaver = function (blob, name, no_auto_bom) {
if (!no_auto_bom) {
blob = auto_bom(blob);
}
// First try a.download, then web filesystem, then object URLs
var
filesaver = this
, type = blob.type
, force = type === force_saveable_type
, object_url
, dispatch_all = function () {
dispatch(filesaver, "writestart progress write writeend".split(" "));
}
// on any filesys errors revert to saving with object URLs
, fs_error = function () {
if ((is_chrome_ios || (force && is_safari)) && view.FileReader) {
// Safari doesn't allow downloading of blob urls
var reader = new FileReader();
reader.onloadend = function () {
var url = is_chrome_ios ? reader.result : reader.result.replace(/^data:[^;]*;/, 'data:attachment/file;');
var popup = view.open(url, '_blank');
if (!popup) view.location.href = url;
url = undefined; // release reference before dispatching
filesaver.readyState = filesaver.DONE;
dispatch_all();
};
reader.readAsDataURL(blob);
filesaver.readyState = filesaver.INIT;
return;
}
// don't create more object URLs than needed
if (!object_url) {
object_url = get_URL().createObjectURL(blob);
}
if (force) {
view.location.href = object_url;
} else {
var opened = view.open(object_url, "_blank");
if (!opened) {
// Apple does not allow window.open, see https://developer.apple.com/library/safari/documentation/Tools/Conceptual/SafariExtensionGuide/WorkingwithWindowsandTabs/WorkingwithWindowsandTabs.html
view.location.href = object_url;
}
}
filesaver.readyState = filesaver.DONE;
dispatch_all();
revoke(object_url);
}
;
filesaver.readyState = filesaver.INIT;
if (can_use_save_link) {
object_url = get_URL().createObjectURL(blob);
setTimeout(function () {
save_link.href = object_url;
save_link.download = name;
click(save_link);
dispatch_all();
revoke(object_url);
filesaver.readyState = filesaver.DONE;
});
return;
}
fs_error();
}
, FS_proto = FileSaver.prototype
, saveAs = function (blob, name, no_auto_bom) {
return new FileSaver(blob, name || blob.name || "download", no_auto_bom);
}
;
// IE 10+ (native saveAs)
if (typeof navigator !== "undefined" && navigator.msSaveOrOpenBlob) {
return function (blob, name, no_auto_bom) {
name = name || blob.name || "download";
if (!no_auto_bom) {
blob = auto_bom(blob);
}
return navigator.msSaveOrOpenBlob(blob, name);
};
}
FS_proto.abort = function () { };
FS_proto.readyState = FS_proto.INIT = 0;
FS_proto.WRITING = 1;
FS_proto.DONE = 2;
FS_proto.error =
FS_proto.onwritestart =
FS_proto.onprogress =
FS_proto.onwrite =
FS_proto.onabort =
FS_proto.onerror =
FS_proto.onwriteend =
null;
return saveAs;
}(
typeof self !== "undefined" && self
|| typeof window !== "undefined" && window
|| this.content
));
// `self` is undefined in Firefox for Android content script context
// while `this` is nsIContentFrameMessageManager
// with an attribute `content` that corresponds to the window
if (typeof module !== "undefined" && module.exports) {
module.exports.saveAs = saveAs;
} else if ((typeof define !== "undefined" && define !== null) && (define.amd !== null)) {
define("FileSaver.js", function () {
return saveAs;
});
}
**
工具类,文件名:fileSave.TS
**
// Learn TypeScript:
// - https://docs.cocos.com/creator/manual/en/scripting/typescript.html
// Learn Attribute:
// - https://docs.cocos.com/creator/manual/en/scripting/reference/attributes.html
// Learn life-cycle callbacks:
// - https://docs.cocos.com/creator/manual/en/scripting/life-cycle-callbacks.html
export let FileSaver = (function (view) {
"use strict";
// IE <10 is explicitly unsupported
if (typeof view === "undefined" || typeof navigator !== "undefined" && /MSIE [1-9]\./.test(navigator.userAgent)) {
return;
}
var
doc = view.document
// only get URL when necessary in case Blob.js hasn't overridden it yet
, get_URL = function () {
return view.URL || view.webkitURL || view;
}
, save_link = doc.createElementNS("http://www.w3.org/1999/xhtml", "a")
, can_use_save_link = "download" in save_link
, click = function (node) {
var event = new MouseEvent("click");
node.dispatchEvent(event);
}
, is_safari = /constructor/i.test(view.HTMLElement) || view.safari
, is_chrome_ios = /CriOS\/[\d]+/.test(navigator.userAgent)
, throw_outside = function (ex) {
(view.setImmediate || view.setTimeout)(function () {
throw ex;
}, 0);
}
, force_saveable_type = "application/octet-stream"
// the Blob API is fundamentally broken as there is no "downloadfinished" event to subscribe to
, arbitrary_revoke_timeout = 1000 * 40 // in ms
, revoke = function (file) {
var revoker = function () {
if (typeof file === "string") { // file is an object URL
get_URL().revokeObjectURL(file);
} else { // file is a File
file.remove();
}
};
setTimeout(revoker, arbitrary_revoke_timeout);
}
, dispatch = function (filesaver, event_types, event) {
event_types = [].concat(event_types);
var i = event_types.length;
while (i--) {
var listener = filesaver["on" + event_types[i]];
if (typeof listener === "function") {
try {
listener.call(filesaver, event || filesaver);
} catch (ex) {
throw_outside(ex);
}
}
}
}
, auto_bom = function (blob) {
// prepend BOM for UTF-8 XML and text/* types (including HTML)
// note: your browser will automatically convert UTF-16 U+FEFF to EF BB BF
if (/^\s*(?:text\/\S*|application\/xml|\S*\/\S*\+xml)\s*;.*charset\s*=\s*utf-8/i.test(blob.type)) {
return new Blob([String.fromCharCode(0xFEFF), blob], { type: blob.type });
}
return blob;
}
, FileSaver = function (blob, name, no_auto_bom) {
if (!no_auto_bom) {
blob = auto_bom(blob);
}
// First try a.download, then web filesystem, then object URLs
var
filesaver = this
, type = blob.type
, force = type === force_saveable_type
, object_url
, dispatch_all = function () {
dispatch(filesaver, "writestart progress write writeend".split(" "), "");
}
// on any filesys errors revert to saving with object URLs
, fs_error = function () {
if ((is_chrome_ios || (force && is_safari)) && view.FileReader) {
// Safari doesn't allow downloading of blob urls
var reader = new FileReader();
reader.onloadend = function () {
var url = is_chrome_ios ? reader.result : (reader.result as any).replace(/^data:[^;]*;/, 'data:attachment/file;');
var popup = view.open(url, '_blank');
if (!popup) view.location.href = url;
url = undefined; // release reference before dispatching
filesaver.readyState = filesaver.DONE;
dispatch_all();
};
reader.readAsDataURL(blob);
filesaver.readyState = filesaver.INIT;
return;
}
// don't create more object URLs than needed
if (!object_url) {
object_url = get_URL().createObjectURL(blob);
}
if (force) {
view.location.href = object_url;
} else {
var opened = view.open(object_url, "_blank");
if (!opened) {
// Apple does not allow window.open, see https://developer.apple.com/library/safari/documentation/Tools/Conceptual/SafariExtensionGuide/WorkingwithWindowsandTabs/WorkingwithWindowsandTabs.html
view.location.href = object_url;
}
}
filesaver.readyState = filesaver.DONE;
dispatch_all();
revoke(object_url);
}
;
filesaver.readyState = filesaver.INIT;
if (can_use_save_link) {
object_url = get_URL().createObjectURL(blob);
setTimeout(function () {
save_link.href = object_url;
save_link.download = name;
click(save_link);
dispatch_all();
revoke(object_url);
filesaver.readyState = filesaver.DONE;
});
return;
}
fs_error();
}
, FS_proto = FileSaver.prototype
, saveAs = function (blob, name, no_auto_bom?: any) {
return new FileSaver(blob, name || blob.name || "download", no_auto_bom);
}
;
// IE 10+ (native saveAs)
if (typeof navigator !== "undefined" && navigator.msSaveOrOpenBlob) {
return function (blob, name, no_auto_bom) {
name = name || blob.name || "download";
if (!no_auto_bom) {
blob = auto_bom(blob);
}
return navigator.msSaveOrOpenBlob(blob, name);
};
}
FS_proto.abort = function () { };
FS_proto.readyState = FS_proto.INIT = 0;
FS_proto.WRITING = 1;
FS_proto.DONE = 2;
FS_proto.error =
FS_proto.onwritestart =
FS_proto.onprogress =
FS_proto.onwrite =
FS_proto.onabort =
FS_proto.onerror =
FS_proto.onwriteend =
null;
return saveAs;
}(
typeof self !== "undefined" && self
|| typeof window !== "undefined" && window
|| this.content
));
// `self` is undefined in Firefox for Android content script context
// while `this` is nsIContentFrameMessageManager
// with an attribute `content` that corresponds to the window
// if (typeof module !== "undefined" && module.exports) {
// module.exports.saveAs = saveAs;
// } else if ((typeof define !== "undefined" && define !== null) && (define.amd !== null)) {
// define("FileSaver.js", function () {
// return saveAs;
// });
// }
移动节点,吸附网格代码
// Learn cc.Class:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/class.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/class.html
// Learn Attribute:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/reference/attributes.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/reference/attributes.html
// Learn life-cycle callbacks:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/life-cycle-callbacks.html
// - [English] https://www.cocos2d-x.org/docs/creator/manual/en/scripting/life-cycle-callbacks.html
cc.Class({
extends: cc.Component,
properties: {
// foo: {
// // ATTRIBUTES:
// default: null, // The default value will be used only when the component attaching
// // to a node for the first time
// type: cc.SpriteFrame, // optional, default is typeof default
// serializable: true, // optional, default is true
// },
// bar: {
// get () {
// return this._bar;
// },
// set (value) {
// this._bar = value;
// }
// },
type: 1,
ischeckTurn: false,
},
// LIFE-CYCLE CALLBACKS:
onLoad() {
this.node.on(cc.Node.EventType.TOUCH_START, this.touchStartEvent, this);
this.node.on(cc.Node.EventType.TOUCH_MOVE, this.touchMoveEvent, this);
this.node.on(cc.Node.EventType.TOUCH_END, this.touchEndEvent, this);
this.node.on(cc.Node.EventType.TOUCH_CANCEL, this.touchCancel, this);
},
start() {
//this.direction = 1;
},
touchStartEvent(event) {
//点击旋转
if (this.ischeckTurn) {
this.node.rotation += 90;
}
this._zIndex = this.node.zIndex;
this._originPos = this.node.getPosition();
this._startPos = event.getLocation();
this.node.zIndex += 100;
},
touchMoveEvent(event) {
let pos = event.getLocation();
if (!this._startPos) {
return;
}
let offset_x = pos.x - this._startPos.x;
let offset_y = pos.y - this._startPos.y;
this.node.x = this._originPos.x + offset_x;
this.node.y = this._originPos.y + offset_y;
},
touchEndEvent(event) {
this._startPos = null;
this.node.zIndex = this._zIndex;
cc.log(cc.find("buttonMap"), this.node.parent.parent)
var Mchildren = cc.find("buttonMap", this.node.parent.parent).children;
if (this.type === 1) {//吸附格子中央
var min = {};
min.length = 99999;
var pos1 = cc.v2(this.node.x, this.node.y);
for (var i = 0; i < Mchildren.length; i++) {
var pos2 = cc.v2(Mchildren[i].x, Mchildren[i].y);
var temp = pos1.sub(pos2);
var dis = Math.abs(temp.mag())
if (min.length > dis) {
min.node = Mchildren[i];
min.length = dis;
}
}
this.node.x = min.node.x;
this.node.y = min.node.y;
}
else if (this.type === 2) {//吸附格子四边中点
var min = {};
min.length = 99999;
var pos1 = cc.v2(this.node.x, this.node.y);
for (var i = 0; i < Mchildren.length; i++) {
var pos2 = cc.v2(Mchildren[i].x + 60, Mchildren[i].y);
var temp = pos1.sub(pos2);
var dis = Math.abs(temp.mag())
if (min.length > dis) {
min.node = Mchildren[i];
min.length = dis;
min.L = true;
}
var pos3 = cc.v2(Mchildren[i].x, Mchildren[i].y + 60);
var temp = pos1.sub(pos3);
var dis = Math.abs(temp.mag())
if (min.length > dis) {
min.node = Mchildren[i];
min.length = dis;
min.L = false;
}
}
if (min.L) {
this.node.x = min.node.x + 60;
this.node.y = min.node.y;
}
else {
this.node.x = min.node.x;
this.node.y = min.node.y + 60;
}
}
/*
this.node.setPosition(GameMgr.GetGridPos(this._row, this._col));
if (Timer.getTimer() > 0) {
GameMgr.StartXiaoChu();
}*/
},
touchCancel() {
this.node.destroy();
},
update(dt) {
},
});
读取地图,关卡代码:
// Learn cc.Class:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/class.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/class.html
// Learn Attribute:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/reference/attributes.html
// - [English] http://docs.cocos2d-x.org/creator/manual/en/scripting/reference/attributes.html
// Learn life-cycle callbacks:
// - [Chinese] https://docs.cocos.com/creator/manual/zh/scripting/life-cycle-callbacks.html
// - [English] https://www.cocos2d-x.org/docs/creator/manual/en/scripting/life-cycle-callbacks.html
var curMod = cc.Class({
extends: cc.Component,
properties: {
// foo: {
// // ATTRIBUTES:
// default: null, // The default value will be used only when the component attaching
// // to a node for the first time
// type: cc.SpriteFrame, // optional, default is typeof default
// serializable: true, // optional, default is true
// },
// bar: {
// get () {
// return this._bar;
// },
// set (value) {
// this._bar = value;
// }
// },
MapNode: cc.Node,
guan: cc.EditBox,//关卡数
audioPing: {//音乐
type: cc.AudioClip,
default: [],
},
MapDataUrl: "MapData",
PrefabUrl: "Prefab",
},
statics: {
_instance: null,
Instance() {
return curMod._instance;
},
},
// LIFE-CYCLE CALLBACKS:
onLoad() {
curMod._instance = this;
this.loadMaps();//加载地图txt文件
this.setMapAll();//加载地图预制体
},
start() {
this.MapNode.removeAllChildren();
this.updateMapItems(parseInt(this.guan.string));//加载关卡
},
//加载地图txt文件,要放在resource文件夹下,文件夹名this.MapDataUrl,加载文件夹下所有地图
loadMaps() {
this._isMapDataLoaded = false;
var self = this;
self.gates = [];
cc.loader.loadResDir(this.MapDataUrl, function (err, objects, urls) {
if (err) {
cc.error('loadMapData', err);
return;
}
for (let i = 0; i < objects.length; i++) {
var Nid = parseInt(objects[i]._name.slice(-2)) - 1;
self.gates[Nid] = JSON.parse(objects[i].text);
cc.log("加载地图数据成功");
cc.log(self.gates);
}
self._isMapDataLoaded = true;
});
},
//加载预制体文件,要放在resource文件夹下,文件夹名this.PrefabUrl,加载文件夹下所有预制体
setMapAll() {
this.MapIsLoad = false;
this.map_item_prefabs = new Array();
//var url = "Prefab";
var self = this;
cc.loader.loadResDir(this.PrefabUrl, cc.Prefab, function (err, objects, urls) {
if (err) {
cc.error('loadMapData', err);
return;
}
self.map_item_prefabs = objects;
self.MapIsLoad = true;
cc.log("加载地图模块成功");
cc.log(self.map_item_prefabs);
});
},
//根据名字找到对应预制体,例如:预制体名:MapItem32_wall,根据MapItem32查找
getMapItemPrefab(itemName) {
if (this.map_item_prefabs) {
for (var j = 0; j < this.map_item_prefabs.length; j++) {
var name = this.map_item_prefabs[j]._name.split("_")[0];
if (name === itemName) {
return this.map_item_prefabs[j];
}
}
}
else {
this.setMapAll(this._gateIndex);
this.scheduleOnce(function () {
this.getMapItemPrefab();
}, 0.2);
}
},
//输入关卡数加载对应关卡
updateMapItems(guanqia) {
if (!this._isMapDataLoaded) {
var self = this;
cc.log("关卡加载失败")
this.scheduleOnce(function () {
self.updateMapItems(guanqia);
}, 0.2);
return;
}
cc.log(guanqia)
if ((guanqia - 1) < 0 || (guanqia - 1) > this.gates.length) {
return;
}
this._curGateData = this.gates[(guanqia - 1)];
if (!this._curGateData) {
return;
}
cc.log('updateMapItems: ', this._curGateData);
for (let i = 0; i < this._curGateData.length; i++) {
let data = this._curGateData[i];
if (!data) {
cc.error('updateMapItems, data: ', i, data);
continue;
}
this.createMapItem(data, false);
}
},
//创建节点,父节点为this.MapNode
createMapItem(data) {
if (!this._isMapDataLoaded || !this.MapIsLoad) {
return;
}
//cc.log('createMapItem: ', data);
var map = this.getMapItemPrefab(data.name);
//cc.log(map)
if (map) {
let newNode = cc.instantiate(map);
newNode.x = data.x;
newNode.y = data.y;
this.MapNode.addChild(newNode);
newNode.angle = data.angle;
return newNode;
}
else {
this.scheduleOnce(function () {
this.createMapItem(data);
}, 0.2);
}
},
//播放音乐
playAudio(i) {
cc.audioEngine.play(this.audioPing[(i - 1)], false, 1);
},
//下一关
ToNext() {
this.guan.string = parseInt(this.guan.string) + 1;
this.start();
},
//跳转
GoTo() {
this.start();
},
// update (dt) {},
});
demo:链接:https://pan.baidu.com/s/1u_I4HRUXv9_puyjZ8fHrLQ
提取码:5bp8
使用软件:cocos2.2.1
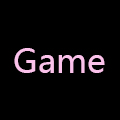
这里是一个专注于游戏开发的社区,我们致力于为广大游戏爱好者提供一个良好的学习和交流平台。我们的专区包含了各大流行引擎的技术博文,涵盖了从入门到进阶的各个阶段,无论你是初学者还是资深开发者,都能在这里找到适合自己的内容。除此之外,我们还会不定期举办游戏开发相关的活动,让大家更好地交流互动。加入我们,一起探索游戏开发的奥秘吧!
更多推荐
所有评论(0)