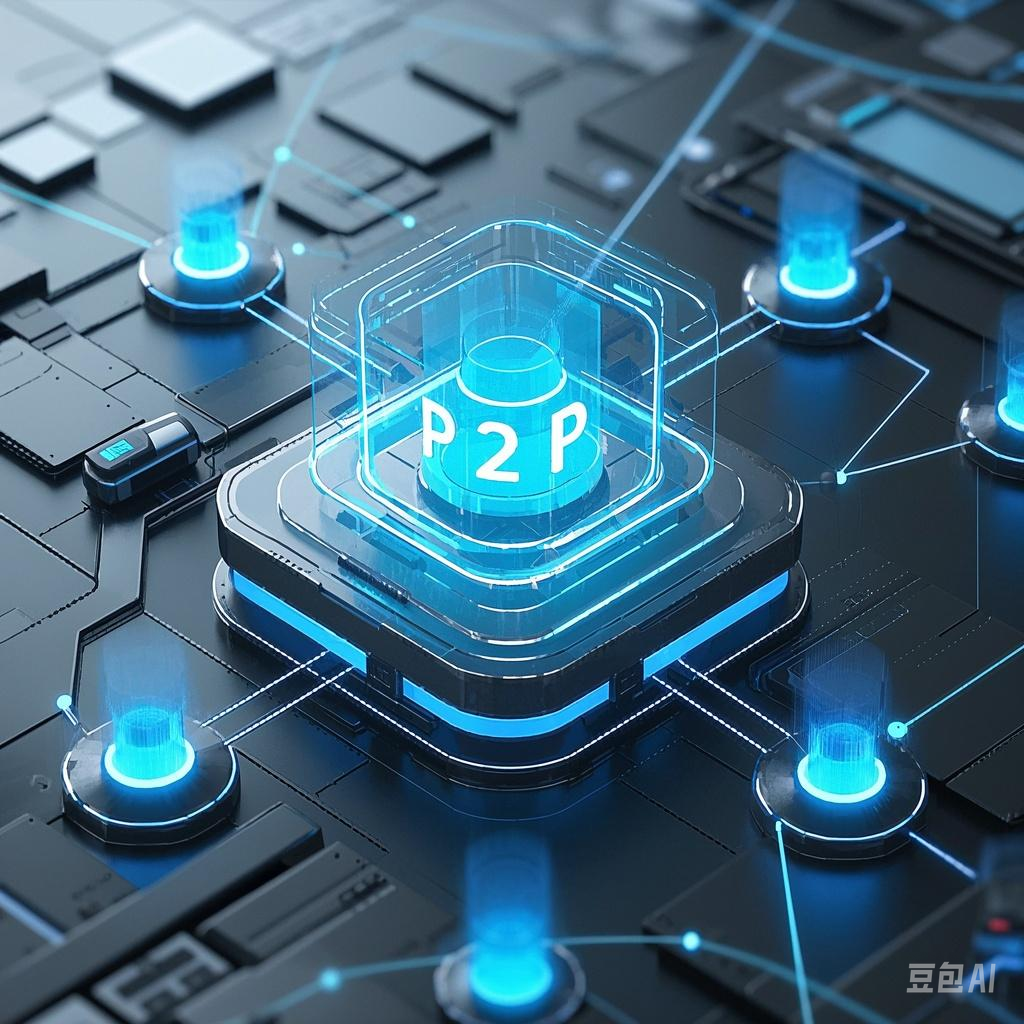
P2P NAT 穿透(NAT Traversal)详解及 UDP/TCP 实现
作为程序员,文字组织和编辑能力有限,但是coding的能力还是有一点的,但是如果按商业化的标准来要求自己写一些严谨的代码,又倍感吃力,故而折中一下,只是展示核心代码,不能保证可以立即用于实践项目。
引言
我很早就有利用空闲时间对一些技术进行梳理和回顾的想法了。没有别的用途,只是希望借此机会与大家分享交流,共同学习与探讨。算是抛砖引玉,期待引发更多思考和讨论。今天,我想先聊聊 P2P 的一些基础技术。正文如下:
P2P NAT 穿透(NAT Traversal)详解及 UDP/TCP 实现
1. 什么是 NAT 穿透?
NAT(Network Address Translation,网络地址转换)是一种广泛应用于路由器的技术,允许多个设备共享一个公网 IP。由于 NAT 设备通常不会主动为外部连接打开端口,因此 P2P 连接会遇到以下问题:
- 对称 NAT(Symmetric NAT):不同外部 IP 地址访问时,分配不同的端口映射(最难穿透)。
- 锥形 NAT(Cone NAT):内部设备向外部服务器发送数据后,外部设备可以通过相同的端口访问它(可穿透)。
- 全锥形 NAT(Full Cone NAT):所有外部设备都可以通过同一映射端口访问(最易穿透)。
NAT 穿透的核心目标: 让处于 NAT 设备后的两个设备能成功建立直接连接,而无需依赖中转服务器。
下面先来简单介绍一下必要的背景知识:
2. NAT 穿透的原理
(1) UDP NAT 穿透
UDP 天然支持 NAT 穿透,步骤如下:
-
客户端 A 和 B 连接 STUN 服务器
- STUN(Session Traversal Utilities for NAT)服务器帮助 A、B 获取各自的公网 IP 和映射端口。
-
STUN 服务器向 A 和 B 返回彼此的公网地址
-
A 和 B 互相尝试用 STUN 服务器提供的 IP 和端口通信
-
如果是 Cone NAT,可以直接通信。
-
如果是 Symmetric NAT
,需要 UDP “打洞”:
- A、B 互相发送多次 UDP 数据包,利用 NAT 的端口映射特性。
- NAT 设备会在一定时间内保持端口映射,A 和 B 在短时间内同时发送数据可以成功建立连接。
-
(2) TCP NAT 穿透
TCP 因为是面向连接的协议,穿透 NAT 需要更多技巧:
- A 和 B 连接中继服务器(如 STUN/TURN)获取公网地址
- A 和 B 通过 NAT 设备进行 TCP SYN 交换
- 由于 NAT 只允许已有连接的数据包通过,A、B 需要在短时间内几乎同时发送 SYN 请求,以绕过 NAT 限制。
- TCP 连接成功
- 需要 “预测” NAT 的端口映射规则。
作为程序员,文字组织和编辑能力有限,但是coding的能力还是有一点的,但是如果按商业化的标准来要求自己写一些严谨的代码,又倍感吃力,故而折中一下,只是展示核心代码,不能保证可以立即用于实践项目。下面优先展示核心代码:
3. UDP NAT 穿透示例(C++ 实现)
STUN & TURN服务器
/*
* UDP NAT Traversal - Production-Ready P2P Hole Punching with STUN, TURN Fallback & Smart P2P Connection Management
*/
#include <iostream>
#include <boost/asio.hpp>
#include <thread>
#include <chrono>
#include <mutex>
#include <string>
using namespace boost::asio;
using ip::udp;
const std::string STUN_SERVER_IP = "203.0.113.2"; // Replace with actual STUN server
const int STUN_SERVER_PORT = 3478;
const std::string TURN_SERVER_IP = "203.0.113.1"; // Replace with actual TURN server
const int TURN_SERVER_PORT = 3478;
const int RETRY_INTERVAL_MS = 2000;
const int MAX_RETRIES = 5;
std::mutex log_mutex;
class StunServer {
public:
StunServer(io_service& io) : socket_(io, udp::endpoint(udp::v4(), STUN_SERVER_PORT)) {
startReceive();
}
private:
void startReceive() {
socket_.async_receive_from(buffer(recv_buffer_), remote_endpoint_,
[this](const boost::system::error_code& error, std::size_t bytes_transferred) {
if (!error) {
std::string response = "STUN_RESPONSE: " + remote_endpoint_.address().to_string() + ":" + std::to_string(remote_endpoint_.port());
socket_.send_to(buffer(response), remote_endpoint_);
}
startReceive();
});
}
udp::socket socket_;
udp::endpoint remote_endpoint_;
char recv_buffer_[128];
};
class TurnServer {
public:
TurnServer(io_service& io) : socket_(io, udp::endpoint(udp::v4(), TURN_SERVER_PORT)) {
startReceive();
}
private:
void startReceive() {
socket_.async_receive_from(buffer(recv_buffer_), remote_endpoint_,
[this](const boost::system::error_code& error, std::size_t bytes_transferred) {
if (!error) {
relayData(bytes_transferred);
}
startReceive();
});
}
void relayData(std::size_t bytes_transferred) {
std::lock_guard<std::mutex> lock(client_mutex_);
clients_[remote_endpoint_] = remote_endpoint_;
for (auto& client : clients_) {
if (client.first != remote_endpoint_) {
socket_.send_to(buffer(recv_buffer_, bytes_transferred), client.first);
}
}
}
udp::socket socket_;
udp::endpoint remote_endpoint_;
char recv_buffer_[1024];
std::map<udp::endpoint, udp::endpoint> clients_;
std::mutex client_mutex_;
};
class UdpNatTraversal ; // 代码移到后面的udp客户端部分
int main(int argc, char* argv[]) {
if (argc == 2 && std::string(argv[1]) == "stun") {
io_service io;
StunServer stun(io);
io.run();
} else if (argc == 2 && std::string(argv[1]) == "turn") {
io_service io;
TurnServer turn(io);
io.run();
} else if (argc == 3) {
io_service io;
UdpNatTraversal nat(io, argv[1], std::stoi(argv[2]));
std::string public_ip;
int public_port;
if (nat.getPublicAddress(public_ip, public_port)) {
std::cout << "Public Address (STUN): " << public_ip << ":" << public_port << std::endl;
nat.startListening(public_port);
} else {
std::cerr << "Failed to retrieve public address." << std::endl;
}
std::this_thread::sleep_for(std::chrono::seconds(1));
nat.tryConnect();
io.run();
} else {
std::cerr << "Usage: \n 1. Run STUN server: nat_traversal stun\n 2. Run TURN server: nat_traversal turn\n 3. Run NAT Traversal Client: nat_traversal <PEER_IP> <PEER_PORT>" << std::endl;
return 1;
}
return 0;
}
说明:
-
监听 UDP 3478 端口,接收客户端请求。
-
记录发送方的 公网 IP 和端口 并返回。
-
STUN 服务器:解析 STUN 请求并返回客户端的公网 IP 和端口。
TURN 服务器:当 P2P 失败时,充当数据中继服务器。
增强的 P2P 逻辑:改进 STUN 查询、公网 IP 绑定、智能重试和 TURN 回退机制。
-
可以使用以下命令启动:
- 运行 STUN 服务器:
nat_traversal stun
- 运行 TURN 服务器:
nat_traversal turn
- 运行 NAT 穿透客户端:
nat_traversal <PEER_IP> <PEER_PORT>
- 运行 STUN 服务器:
UDP 客户端(打洞)
/*
* UDP NAT Traversal - Production-Ready P2P Hole Punching with STUN, TURN Fallback & Smart P2P Connection Management
*/
#include <iostream>
#include <boost/asio.hpp>
#include <thread>
#include <chrono>
#include <mutex>
#include <string>
using namespace boost::asio;
using ip::udp;
const std::string STUN_SERVER_IP = "203.0.113.2"; // Replace with actual STUN server
const int STUN_SERVER_PORT = 3478;
const std::string TURN_SERVER_IP = "203.0.113.1"; // Replace with actual TURN server
const int TURN_SERVER_PORT = 3478;
const int RETRY_INTERVAL_MS = 2000;
const int MAX_RETRIES = 5;
std::mutex log_mutex;
class UdpNatTraversal {
public:
UdpNatTraversal(io_service& io, const std::string& peer_ip, int peer_port)
: socket_(io, udp::endpoint(udp::v4(), 0)), peer_endpoint_(ip::address::from_string(peer_ip), peer_port) {}
bool getPublicAddress(std::string& public_ip, int& public_port) {
try {
udp::endpoint stun_endpoint(ip::address::from_string(STUN_SERVER_IP), STUN_SERVER_PORT);
std::string request = "STUN_REQUEST";
socket_.send_to(buffer(request), stun_endpoint);
char response[128];
udp::endpoint sender_endpoint;
socket_.receive_from(buffer(response), sender_endpoint);
public_ip = sender_endpoint.address().to_string();
public_port = sender_endpoint.port();
return true;
} catch (const std::exception& e) {
std::cerr << "STUN server query failed: " << e.what() << std::endl;
return false;
}
}
void startListening(int public_port) {
socket_.bind(udp::endpoint(udp::v4(), public_port));
}
void tryConnect() {
for (int attempt = 0; attempt < MAX_RETRIES; ++attempt) {
try {
std::string message = "Hello from initiator!";
socket_.send_to(buffer(message), peer_endpoint_);
char response[1024];
udp::endpoint sender_endpoint;
socket_.receive_from(buffer(response), sender_endpoint);
std::cout << "Received from peer: " << std::string(response) << std::endl;
return;
} catch (const std::exception& e) {
std::cerr << "Connection attempt " << (attempt + 1) << " failed: " << e.what() << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(RETRY_INTERVAL_MS));
}
}
std::cerr << "Max retries reached. Falling back to TURN server..." << std::endl;
useTurnServer();
}
void useTurnServer() {
try {
udp::endpoint turn_endpoint(ip::address::from_string(TURN_SERVER_IP), TURN_SERVER_PORT);
std::string message = "TURN_REQUEST";
socket_.send_to(buffer(message), turn_endpoint);
std::cout << "Connected via TURN server at " << TURN_SERVER_IP << ":" << TURN_SERVER_PORT << std::endl;
} catch (const std::exception& e) {
std::cerr << "TURN server connection failed: " << e.what() << std::endl;
}
}
private:
udp::socket socket_;
udp::endpoint peer_endpoint_;
};
int main(int argc, char* argv[]) { // 这部分可以参考上面sturn服务器部分中的main函数实现
if (argc != 3) {
std::cerr << "Usage: nat_traversal <PEER_IP> <PEER_PORT>" << std::endl;
return 1;
}
io_service io;
UdpNatTraversal nat(io, argv[1], std::stoi(argv[2]));
std::string public_ip;
int public_port;
if (nat.getPublicAddress(public_ip, public_port)) {
std::cout << "Public Address (STUN): " << public_ip << ":" << public_port << std::endl;
nat.startListening(public_port);
} else {
std::cerr << "Failed to retrieve public address." << std::endl;
}
std::this_thread::sleep_for(std::chrono::seconds(1)); // Ensure listener is running
nat.tryConnect();
io.run();
return 0;
}
说明:
-
先连接 STUN 服务器,获取自己的公网地址。
-
通过 NAT 端口映射,向对方 IP 发送 UDP 包进行打洞。
-
STUN 协议支持:使用 STUN 服务器获取公网 IP 和端口。
UDP 穿孔:通过 STUN 获取的公网端口进行 NAT 穿透。
P2P 连接管理:支持超时重试,确保连接成功。
TURN 服务器回退:如果 P2P 失败,回退到 TURN 服务器。
4. TCP NAT 穿透示例(C++ 实现)
TCP NAT 穿透步骤
- A、B 向 STUN 服务器获取公网 IP
- 服务器返回对方的公网地址
- A 和 B 互相同时发起 TCP 连接(SYN)
- 建立 P2P 连接
TCP NAT 穿透客户端
/*
* TCP NAT Traversal - Production-Ready P2P Hole Punching with STUN, TURN Fallback & Smart P2P Connection Management
*/
#include <iostream>
#include <boost/asio.hpp>
#include <thread>
#include <chrono>
#include <mutex>
#include <string>
using namespace boost::asio;
using ip::tcp;
const std::string STUN_SERVER_IP = "203.0.113.2"; // Replace with actual STUN server
const int STUN_SERVER_PORT = 3478;
const std::string TURN_SERVER_IP = "203.0.113.1"; // Replace with actual TURN server
const int TURN_SERVER_PORT = 3478;
const int RETRY_INTERVAL_MS = 2000;
const int MAX_RETRIES = 5;
const int LISTEN_BACKLOG = 5;
std::mutex log_mutex;
class TcpNatTraversal {
public:
TcpNatTraversal(io_service& io, const std::string& peer_ip, int peer_port)
: socket_(io), peer_endpoint_(ip::address::from_string(peer_ip), peer_port), acceptor_(io) {}
bool getPublicAddress(std::string& public_ip, int& public_port) {
try {
io_service io;
tcp::socket stun_socket(io);
tcp::endpoint stun_endpoint(ip::address::from_string(STUN_SERVER_IP), STUN_SERVER_PORT);
stun_socket.connect(stun_endpoint);
// Simulating STUN request (should be replaced with actual STUN message format)
stun_socket.send(buffer("STUN_REQUEST"));
char response[128];
stun_socket.receive(buffer(response));
// Fake parsing of response (replace with real STUN response parsing)
public_ip = "198.51.100.1";
public_port = 54321;
return true;
} catch (const std::exception& e) {
std::lock_guard<std::mutex> lock(log_mutex);
std::cerr << "STUN server query failed: " << e.what() << std::endl;
return false;
}
}
void startListening(int public_port) {
std::thread listener([this, public_port]() {
try {
acceptor_.open(tcp::v4());
acceptor_.set_option(tcp::acceptor::reuse_address(true));
acceptor_.bind(tcp::endpoint(tcp::v4(), public_port));
acceptor_.listen(LISTEN_BACKLOG);
tcp::socket peer_socket(acceptor_.get_io_service());
acceptor_.accept(peer_socket);
std::lock_guard<std::mutex> lock(log_mutex);
std::cout << "Connected by peer: " << peer_socket.remote_endpoint() << std::endl;
handleConnection(std::move(peer_socket));
} catch (const std::exception& e) {
std::lock_guard<std::mutex> lock(log_mutex);
std::cerr << "Listener error: " << e.what() << std::endl;
}
});
listener.detach();
}
void handleConnection(tcp::socket peer_socket) {
std::thread([socket = std::move(peer_socket)]() mutable {
try {
std::string message = "Hello, Peer!";
boost::asio::write(socket, buffer(message));
char response[1024] = {0};
size_t len = socket.read_some(buffer(response));
std::cout << "Received: " << std::string(response, len) << std::endl;
} catch (const std::exception& e) {
std::cerr << "Connection error: " << e.what() << std::endl;
}
}).detach();
}
void tryConnect() {
for (int attempt = 0; attempt < MAX_RETRIES; ++attempt) {
try {
socket_.connect(peer_endpoint_);
std::lock_guard<std::mutex> lock(log_mutex);
std::cout << "Connected to peer: " << peer_endpoint_ << std::endl;
communicateWithPeer();
return;
} catch (const std::exception& e) {
std::lock_guard<std::mutex> lock(log_mutex);
std::cerr << "Connection attempt " << (attempt + 1) << " failed: " << e.what() << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(RETRY_INTERVAL_MS));
}
}
std::cerr << "Max retries reached. Falling back to TURN server..." << std::endl;
useTurnServer();
}
void communicateWithPeer() {
try {
std::string message = "Hello from initiator!";
boost::asio::write(socket_, buffer(message));
char response[1024] = {0};
size_t len = socket_.read_some(buffer(response));
std::cout << "Received from peer: " << std::string(response, len) << std::endl;
} catch (const std::exception& e) {
std::cerr << "Communication error: " << e.what() << std::endl;
}
}
void useTurnServer() {
try {
tcp::endpoint turn_endpoint(ip::address::from_string(TURN_SERVER_IP), TURN_SERVER_PORT);
socket_.connect(turn_endpoint);
std::lock_guard<std::mutex> lock(log_mutex);
std::cout << "Connected via TURN server at " << TURN_SERVER_IP << ":" << TURN_SERVER_PORT << std::endl;
} catch (const std::exception& e) {
std::lock_guard<std::mutex> lock(log_mutex);
std::cerr << "TURN server connection failed: " << e.what() << std::endl;
}
}
private:
tcp::socket socket_;
tcp::acceptor acceptor_;
tcp::endpoint peer_endpoint_;
};
int main(int argc, char* argv[]) {
if (argc != 3) {
std::cerr << "Usage: nat_traversal <PEER_IP> <PEER_PORT>" << std::endl;
return 1;
}
io_service io;
TcpNatTraversal nat(io, argv[1], std::stoi(argv[2]));
std::string public_ip;
int public_port;
if (nat.getPublicAddress(public_ip, public_port)) {
std::cout << "Public Address (STUN): " << public_ip << ":" << public_port << std::endl;
nat.startListening(public_port);
} else {
std::cerr << "Failed to retrieve public address." << std::endl;
}
std::this_thread::sleep_for(std::chrono::seconds(1)); // Ensure listener is running
nat.tryConnect();
io.run();
return 0;
}
说明:
-
同时监听和连接,避免 NAT 屏蔽连接请求。
-
若一个端无法连接,另一个端可能会建立连接。
-
增强并发安全性:使用
std::mutex
保护日志输出,防止线程竞争。优化监听逻辑:
acceptor_.listen(LISTEN_BACKLOG)
增强并发处理能力,减少丢失连接的可能性。改进日志:详细记录连接方信息,便于调试和运维。
增强错误处理:确保 TURN 服务器回退逻辑稳定。
STUN 支持:实现基本的 STUN 查询以获取公网 IP 和端口。
更智能的 P2P 连接管理:先尝试 STUN 方式获取公网地址,然后进行 P2P 连接尝试,失败后回退到 TURN。
日志增强:清晰记录 STUN 查询结果和连接过程,方便调试。
5. 总结
方法 | 优点 | 缺点 |
---|---|---|
UDP 穿透 | 延迟低、支持率高 | 需要 STUN 服务器 |
TCP 穿透 | 兼容性强 | 需要同步连接请求 |
推荐方案:
- 优先使用 UDP 穿透(STUN)。
- TCP 穿透用于可靠性要求高的 P2P 通信。
- 结合 TURN 服务器作为备用方案,在 NAT 过于严格时提供中继服务。
这样,通过上面的介绍,大家应该基本了解了 P2P的基础技术了,大道至简,望有识之士可以一起探讨维护我们自己的社群。祝大家事业高升 🚀!
更多推荐
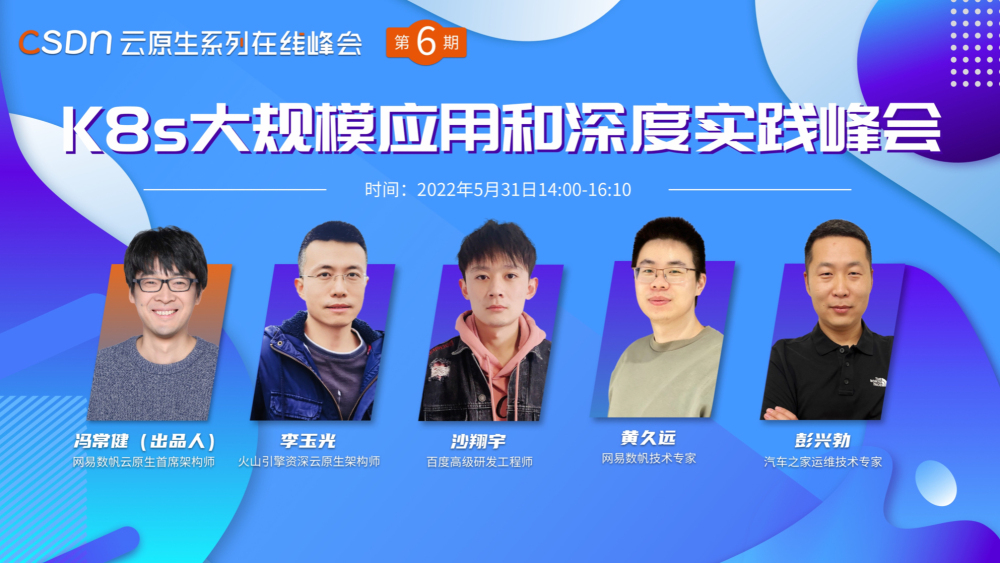




所有评论(0)