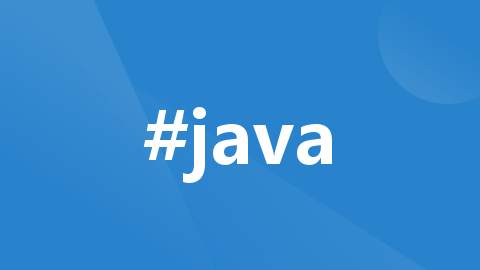
Java腾讯云文字识别相关问题以及使用方法,结合springboot+vue
Java实现文字识别(腾讯云)
·
文章目录
前言
Java调用腾讯云接口实现文字识别,使用base64传值,该文章主要使用通用印刷体识别和表格识别v2进行说明
试一试
提示:以下是本篇文章正文内容,下面案例可供参考
一、文字识别是什么?
文字识别(Optical Character Recognition,OCR)基于腾讯优图实验室的深度学习技术,将图片上的文字内容,智能识别成为可编辑的文本。OCR 支持身份证、名片等卡证类和票据类的印刷体识别,也支持运单等手写体识别,支持提供定制化服务,可以有效地代替人工录入信息。
二、使用步骤
1.安装依赖,通过 Maven 安装
<dependency>
<groupId>com.tencentcloudapi</groupId>
<artifactId>tencentcloud-sdk-java</artifactId>
<!-- go to https://search.maven.org/search?q=tencentcloud-sdk-java and get the latest version. -->
<!-- 请到https://search.maven.org/search?q=tencentcloud-sdk-java查询所有版本,最新版本如下 -->
<version>3.1.322</version>
</dependency>
2.前端上传图片,将图片转为base64传给后端
注:前端我使用的是vue,用的是element ui框架,我只是实现了能使用成功而已,逻辑上并不美观,不足之处,多包涵
前端学习参考网址:图片转base64
2.1页面
<template>
<div class="app-container">
<div>
<div class="badege_box">
<el-form ref="form" :model="form">
<el-form-item label="封面图" prop="educationPhoto">
<el-upload
v-model="form.imageBase"
ref="form"
class="avatar-uploader"
action=""
accept=".jpg,.jpeg,.webp,.png,.JPG,.JPEG"
:show-file-list="false"
:auto-upload="false"
:on-change="getFile">
<div class="uploadImg">
<img class="upload_icon_img" src="" alt="">
<el-button>点击上传图片</el-button>
</div>
</el-upload>
<div class="badge_size">
<div class="maximum">
<img class="school_badge_img" v-if="imageUrl" :src="imageUrl">
</div>
</div>
</el-form-item>
</el-form>
</div>
<el-button
type="primary"
plain
icon="el-icon-plus"
size="mini"
v-hasPermi="['test:ocr:upload']"
@click="submitForm"
></el-button>
</div>
<div>
</div>
</div>
</template>
<script>
import { upload} from "@/api/test/ocr";
export default {
data() {
return {
form:{},
imageUrl: '',
imageBase:''
};
},
methods: {
/** 新增按钮操作 */
/** 提交按钮 */
submitForm() {
this.form.imageUrl = "随意"
this.form.imageBase = this.imageBase
console.log(this.form)
this.$refs["form"].validate(valid => {
console.log("valid"+valid)
if (valid) {
upload(this.form).then(response => {
console.log(response)
this.$modal.msgSuccess("新增成功");
});
}
});
},
submitImg(){
//把this.imageBase传给后台就行了
},
resetImg(){
this.imageUrl='';
this.imageBase='';
},
getFile(file, fileList) {
this.getBase64(file.raw).then(res => {
this.imageUrl = res;
let badegeImg = this.imageUrl.split(',');
this.imageBase = badegeImg[1];
console.log(this.imageBase);
});
},
getBase64(file) {
return new Promise(function(resolve, reject) {
let reader = new FileReader();
let imgResult = "";
reader.readAsDataURL(file);
reader.onload = function() {
imgResult = reader.result;
};
reader.onerror = function(error) {
reject(error);
};
reader.onloadend = function() {
resolve(imgResult);
};
});
}
}
}
</script>
3.后端获取前端传来的base64,调用文字识别接口,返回解析出的文字
3.1通用印刷体识别
//实体类
public class SysOcr
{
/** 图片绝对地址 */
@Excel(name = "图片绝对地址")
private String imageUrl;
/** 图片Base的字符串 */
@Excel(name = "图片Base的字符串")
private String imageBase;
、、、、、get set toString
}
//主要逻辑操作
public String getBase(SysOcr sysOcr) {
try{
// 实例化一个认证对象,入参需要传入腾讯云账户 SecretId 和 SecretKey,此处还需注意密钥对的保密
// 代码泄露可能会导致 SecretId 和 SecretKey 泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考,建议采用更安全的方式来使用密钥,请参见:https://cloud.tencent.com/document/product/1278/85305
// 密钥可前往官网控制台 https://console.cloud.tencent.com/cam/capi 进行获取
Credential cred = new Credential("SecretId", "SecretKey");
// 实例化一个http选项,可选的,没有特殊需求可以跳过
HttpProfile httpProfile = new HttpProfile();
httpProfile.setEndpoint("ocr.tencentcloudapi.com");
// 实例化一个client选项,可选的,没有特殊需求可以跳过
ClientProfile clientProfile = new ClientProfile();
clientProfile.setHttpProfile(httpProfile);
// 实例化要请求产品的client对象,clientProfile是可选的
OcrClient client = new OcrClient(cred, "ap-beijing", clientProfile);
// 实例化一个请求对象,每个接口都会对应一个request对象
GeneralBasicOCRRequest req = new GeneralBasicOCRRequest();
req.setImageBase64(sysOcr.getImageBase());
// 返回的resp是一个GeneralBasicOCRResponse的实例,与请求对象对应
GeneralBasicOCRResponse resp = client.GeneralBasicOCR(req);
// 输出json格式的字符串回包
System.out.println(GeneralBasicOCRResponse.toJsonString(resp));
/*
* 将内容取出
* */
Object[] data = null;
TextDetection[] textDetections = resp.getTextDetections();
System.out.println(textDetections[0].getDetectedText());
for (int i = 0; i < textDetections.length; i++) {
data[i] = textDetections[i];
}
System.out.println(data);
return textDetections[0].getDetectedText();
} catch (TencentCloudSDKException e) {
System.out.println(e.toString());
return e.toString();
}
}
3.2表格识别v2
@Override
public String getTable(SysOcr sysOcr) {
// 实例化一个认证对象,入参需要传入腾讯云账户 SecretId 和 SecretKey,此处还需注意密钥对的保密
// 代码泄露可能会导致 SecretId 和 SecretKey 泄露,并威胁账号下所有资源的安全性。以下代码示例仅供参考,建议采用更安全的方式来使用密钥,请参见:https://cloud.tencent.com/document/product/1278/85305
// 密钥可前往官网控制台 https://console.cloud.tencent.com/cam/capi 进行获取
Credential cred = new Credential("SecretId", "SecretKey");
// 实例化一个http选项,可选的,没有特殊需求可以跳过
HttpProfile httpProfile = new HttpProfile();
httpProfile.setEndpoint("ocr.tencentcloudapi.com");
// 实例化一个client选项,可选的,没有特殊需求可以跳过
ClientProfile clientProfile = new ClientProfile();
clientProfile.setHttpProfile(httpProfile);
// 实例化要请求产品的client对象,clientProfile是可选的
OcrClient client = new OcrClient(cred, "ap-beijing", clientProfile);
// 实例化一个请求对象,每个接口都会对应一个request对象
RecognizeTableOCRRequest tableOCRRequest = new RecognizeTableOCRRequest();
tableOCRRequest.setImageBase64(sysOcr.getImageBase());
// 返回的resp是一个RecognizeTableOCRResponse的实例,与请求对象对应
RecognizeTableOCRResponse tableOCRResponse = null;
try {
tableOCRResponse = client.RecognizeTableOCR(tableOCRRequest);
} catch (TencentCloudSDKException e) {
e.printStackTrace();
}
// 输出json格式的字符串回包
// System.out.println(RecognizeTableOCRResponse.toJsonString(tableOCRResponse));
// 下面是将识别的表格图片转为excel文件,并存入本地
File file = null;
String base64 = tableOCRResponse.getData();
String filePath = "你想把文件放在哪(绝对地址)";
File dir=new File(filePath);
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss");//设置日期格式(年-月-日-时-分-秒)
String createTime = dateFormat.format(now);//格式化然后放入字符串中
if (!dir.exists() && !dir.isDirectory()) {
dir.mkdirs();
}
BufferedOutputStream bos = null;
java.io.FileOutputStream fos = null;
try {
byte[] bytes = Base64.getDecoder().decode(base64);
file=new File(filePath+"/"+ createTime+".xlsx");
fos = new java.io.FileOutputStream(file);
bos = new BufferedOutputStream(fos);
bos.write(bytes);
return "成功";
} catch (Exception e) {
e.printStackTrace();
return "失败";
}finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
4.文字识别相关网址
我阅读的时候也是找了半天,因为太多了也是第一次使用吧,整理了一下比较重要的,仅供参考
腾讯云开发者工具套件(SDK)3.0
相关接口文档说明
访问密钥
文字识别控制台
以上就是文字识别的部分调用,本文仅仅简单介绍了文字识别两个接口的使用,不足之处请多指教
遇见的错误
java整合腾讯云ocr出现java.lang.NoClassDefFoundError
看看是不是jar包冲突
解决办法:删除多余的maven依赖
更多推荐
所有评论(0)