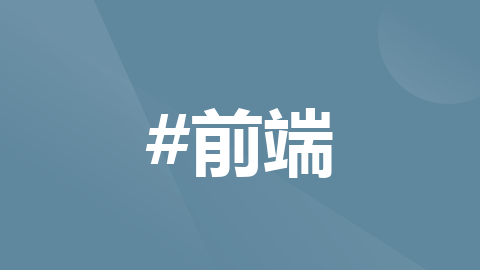
在 Vuex 中,获取值(state)和设置值(通过 mutations 或 actions)是通过一些特定的概念和方法来实现的。
Mutation 是 Vuex 中用来直接变更状态的方法。记住,直接修改 state 是不被允许的,所有的状态变更都必须通过提交 mutation 或 dispatch action 来完成。这样可以确保状态变更的可追踪性和易调试性。: 如果你在非组件的文件中(例如,Vue 插件、Node.js 服务器等)需要访问 Vuex store 的状态,可以通过注入 store 实例来实现。在 Vuex
获取值(State)
在 Vuex 中,state 是存储在 Vuex store 中的数据对象,可以通过以下几种方式获取 state 中的值:
-
在组件中获取 state: 在 Vue 组件内部,你可以使用
this.$store.state
来访问 store 中的状态。例如,如果你想获取名为count
的状态值,可以这样做:
computed: {
countValue() {
return this.$store.state.count;
}
}
2.使用 mapState
辅助函数: mapState
辅助函数帮助我们创建计算属性,从而直接获取 state 中的值。这样可以避免在每个计算属性中重复 this.$store.state
代码。
import { mapState } from 'vuex';
export default {
computed: {
...mapState({
countValue: state => state.count
})
}
};
3.在非组件的文件中获取 state: 如果你在非组件的文件中(例如,Vue 插件、Node.js 服务器等)需要访问 Vuex store 的状态,可以通过注入 store 实例来实现。
import store from './store';
// 直接访问 state
const count = store.state.count;
设置值(Mutations 和 Actions)
在 Vuex 中,状态的变更是通过提交 mutation(变更)或 dispatch actions(行动)来实现的。
-
Mutations: Mutation 是 Vuex 中用来直接变更状态的方法。每个 mutation 都有一个字符串的 type 和一个 handler 函数。你不能直接调用 mutation handler 函数,而是通过 store.commit 方法来触发。
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++;
}
}
});
// 通过 commit 方法触发 mutation
store.commit('increment');
2.Actions: Action 类似于 mutation,但是它是异步的。Action 提交的是 mutation,而不是直接变更状态。
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++;
}
},
actions: {
incrementIfOdd({ state, commit }) {
if (state.count % 2 !== 0) {
commit('increment');
}
}
}
});
// 通过 dispatch 方法触发 action
store.dispatch('incrementIfOdd');
3.使用 mapMutations
和 mapActions
辅助函数: 类似于 mapState
,Vuex 还提供了 mapMutations
和 mapActions
辅助函数,帮助你在组件中更容易地调用 mutations 和 actions。
import { mapMutations, mapActions } from 'vuex';
export default {
methods: {
...mapMutations([
'increment' // 将 `this.$store.commit('increment')` 映射为 `this.increment()`
]),
...mapActions([
'incrementIfOdd' // 将 `this.$store.dispatch('incrementIfOdd')` 映射为 `this.incrementIfOdd()`
])
}
};
通过上述方法,你可以在 Vuex 中方便地获取和设置状态值。记住,直接修改 state 是不被允许的,所有的状态变更都必须通过提交 mutation 或 dispatch action 来完成。这样可以确保状态变更的可追踪性和易调试性。
更多推荐
所有评论(0)