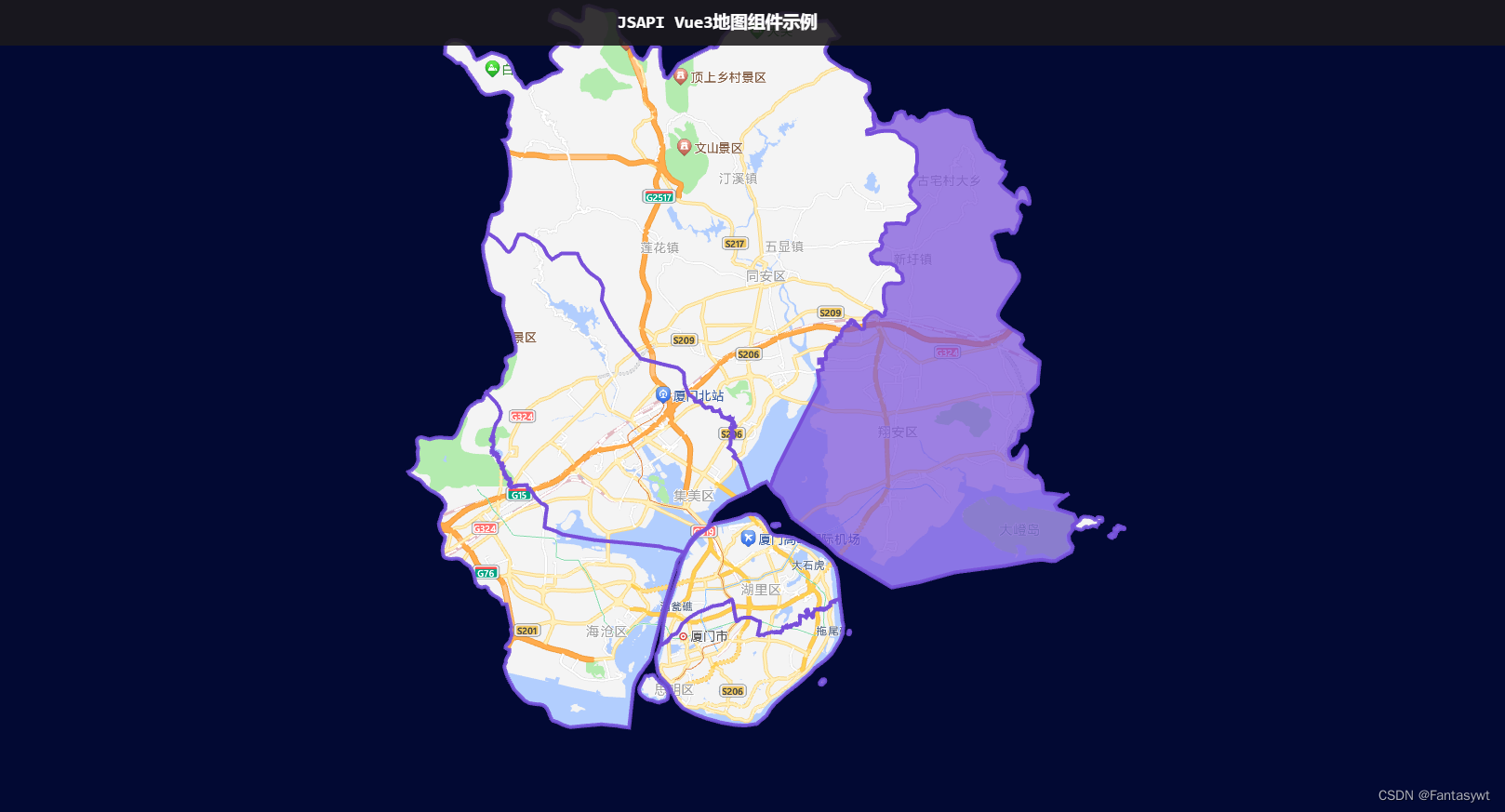
vue3+高德地图只展示指定市、区的行政区域的地图,遮罩反向镂空其他地区
例如像这样,只展示厦门的地图,隐藏其他地方,点击区域,在单独展示区,点击返回回到总览。
·
实现:例如像这样,只展示厦门的地图,隐藏其他地方,点击区域,在单独展示区,点击返回回到总览。 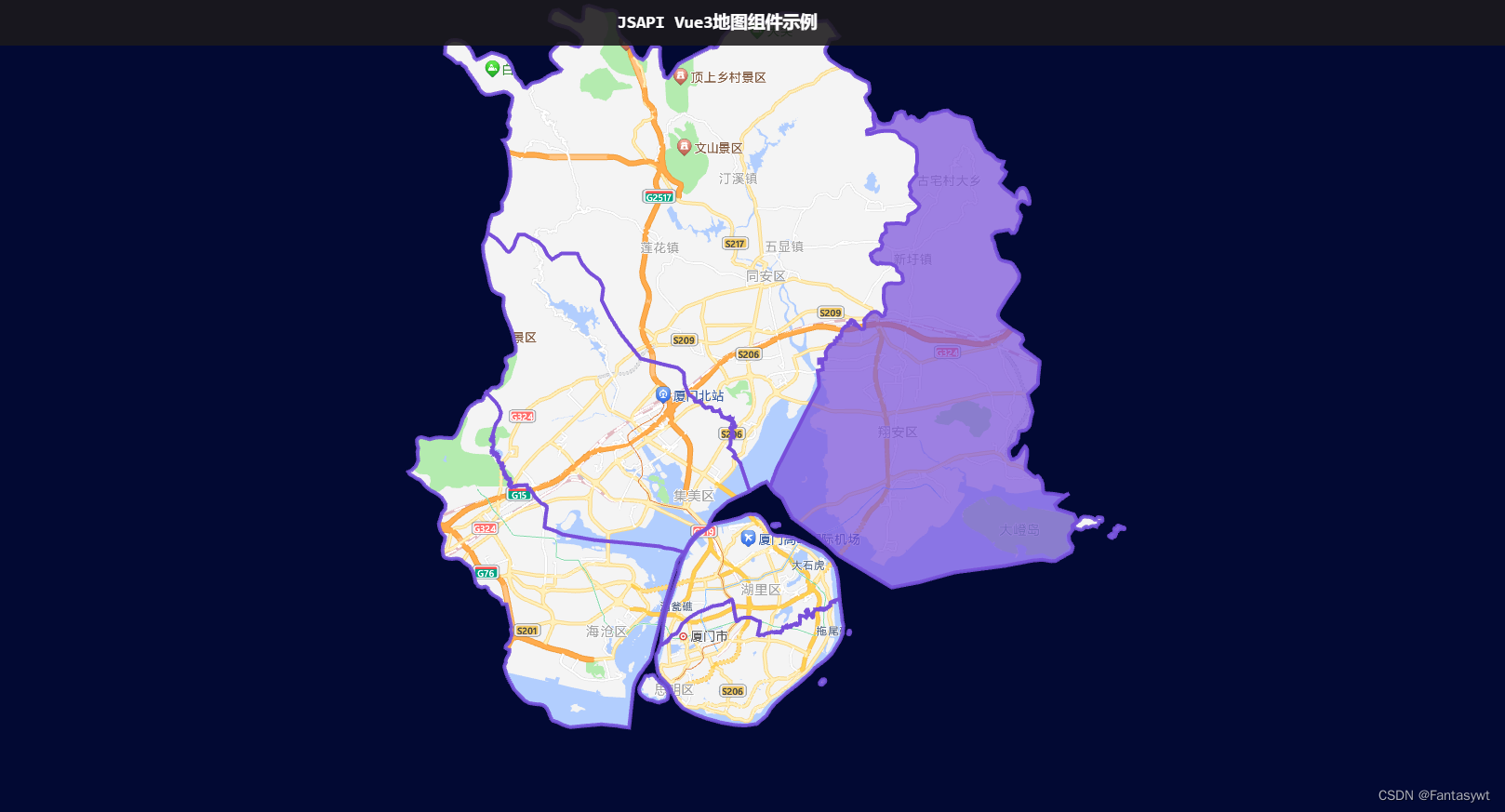
一、获取高德地图的key,和密钥
地址:高德控制台
具体的获取流程就不教授了,百度很多
二、安装
npm i @amap/amap-jsapi-loader --save
三、引入使用
要注意的是,因为使用了行政服务搜索的api,它的search()方法要有回调,就必须配置密钥
点击查看官方推荐的密钥使用方式
window._AMapSecurityConfig = {
securityJsCode: "你的密钥",
};
主要使用的api: 行政区查询服务(AMap.DistrictSearch)
完整代码
<template>
<div class="home_div">
<div class="map-title">
<h3>JSAPI Vue3地图组件示例</h3>
<div v-if="back" id="back" @click="showAllMap" style="cursor: pointer;">返回</div>
</div>
<div id="container"></div>
</div>
</template>
<script lang="ts">
import AMapLoader from "@amap/amap-jsapi-loader";
import { shallowRef } from "@vue/reactivity";
import { ref } from "vue";
export default {
name: "mapComtaint",
setup() {
const map = shallowRef(null) as any;
const AMap = shallowRef(null) as any;
const back = ref(false);
const polygons = shallowRef([]) as any; // 区描边、遮罩
const district = shallowRef(null) as any;
const polygon = shallowRef(null) as any; // 市描边、遮罩
return {
map,
AMap,
back,
polygons,
district,
polygon,
};
},
create() {},
methods: {
/*
返回区域对应颜色
adcode:厦门市 350200
厦门市市辖区 350201
思明区 350203
海沧区 350205
湖里区 350206
集美区 350211
同安区 350212
翔安区 350213
*/
getColorByAdcode(adcode: string) {
let colors = {
"350200": "#111111",
"350201": "#456aed",
"350203": "428cced",
"350205": "#a456e1",
"350206": "#123fee",
"350211": "#666eee",
"350212": "#963qde",
"350213": "#784eda",
} as any;
return colors[adcode];
},
// 初始化遮罩
initArea(city: string, isChildDraw: boolean = false) {
let that = this;
this.district.search(city, function (status: string, result: any) {
// 外多边形坐标数组和内多边形坐标数组
var outer = [
new that.AMap.LngLat(-360, 90, true),
new that.AMap.LngLat(-360, -90, true),
new that.AMap.LngLat(360, -90, true),
new that.AMap.LngLat(360, 90, true),
];
console.log(result);
// 绘制遮罩
var holes = result.districtList[0].boundaries;
var pathArray = [outer];
pathArray.push.apply(pathArray, holes);
that.polygon = new that.AMap.Polygon({
pathL: pathArray,
strokeColor: "#00eeff",
strokeWeight: 1,
fillColor: "#020933",
fillOpacity: 1,
});
that.polygon.setPath(pathArray);
that.map.add(that.polygon);
// 判断是否要绘制子区域
if (isChildDraw) {
// 将搜索层级设置为 区、县
that.district.setLevel("district");
for (let i = 0; i < result.districtList[0].districtList.length; i++) {
that.areaPolyline(result.districtList[0].districtList[i].adcode);
}
}
});
},
// 显示总览
showAllMap() {
this.back = false;
if (this.polygon) {
// 清除遮罩
this.map.remove(this.polygon);
}
this.initArea("厦门市", true);
this.map.setCenter([118.113994, 24.614998]);
this.map.setZoom(11);
},
// 绘制区域描边
areaPolyline(adcode: string) {
let that = this;
if (this.polygons.length) {
this.map.remove(this.polygons);
this.polygons = [];
}
this.district.search(adcode, function (status: string, result: any) {
console.log("区", result);
// 绘制区域描边
let bounds = result.districtList[0].boundaries;
for (let i = 0; i < bounds.length; i++) {
const color = that.getColorByAdcode(result.districtList[0].adcode);
const polygon = new that.AMap.Polygon({
path: bounds[i],
strokeColor: "#784eda",
strokeWeight: 4,
fillColor: "#784eda",
fillOpacity: 0,
});
// 添加监听事件
polygon.on("mouseover", () => {
if (!that.back) {
polygon.setOptions({
fillOpacity: 0.7,
});
}
});
// 添加点击事件
polygon.on("click", () => {
// 判断是否为市级
if (!that.back) {
// 显示返回按钮
that.back = true;
that.map.setZoom(12);
// 修改中心位置为区级中心
that.map.setCenter([
result.districtList[0].center.lng,
result.districtList[0].center.lat,
]);
// 绘画
that.initArea(result.districtList[0].adcode, false);
}
});
polygon.on("mouseout", () => {
polygon.setOptions({
fillOpacity: 0,
});
});
that.polygons.push(polygon);
}
that.map.add(that.polygons);
});
},
ininMap() {
let that = this;
// 这个配置很重要,必须设置,否则你的 行政服务搜索api无法使用生成回调
window._AMapSecurityConfig = {
securityJsCode: "密钥",
};
AMapLoader.load({
key: "你的key", //设置您的key
version: "2.0",
plugins: [
"AMap.ToolBar",
"AMap.Driving",
"AMap.Polygon",
"AMap.DistrictSearch",
"AMap.Object3DLayer",
"AMap.Object3D",
"AMap.Polyline",
],
AMapUI: {
version: "1.1",
plugins: [],
},
Loca: {
version: "2.0.0",
},
})
.then((AMap) => {
that.AMap = AMap;
that.map = new AMap.Map("container", {
viewMode: "3D",
zoom: 11,
zooms: [10, 20],
center: [118.113994, 24.614998],
});
that.district = new AMap.DistrictSearch({
subdistrict: 3, //获取边界返回下级行政区
extensions: "all", //返回行政区边界坐标组等具体信息
level: "city", //查询行政级别为 市
});
that.initArea("厦门市", true);
})
.catch((e) => {
console.log(e);
});
},
},
mounted() {
//DOM初始化完成进行地图初始化
this.ininMap();
},
};
</script>
<style scope>
.home_div {
height: 100%;
width: 100%;
padding: 0px;
margin: 0px;
position: relative;
}
#container {
height: 100vh;
width: 100%;
padding: 0px;
margin: 0px;
}
.map-title {
position: absolute;
z-index: 1;
width: 100%;
height: 50px;
background-color: rgba(27, 25, 27, 0.884);
display: flex;
justify-content: space-around;
align-items: center;
}
h3 {
z-index: 2;
color: white;
}
</style>
效果图如文章开头图片
更多推荐
所有评论(0)