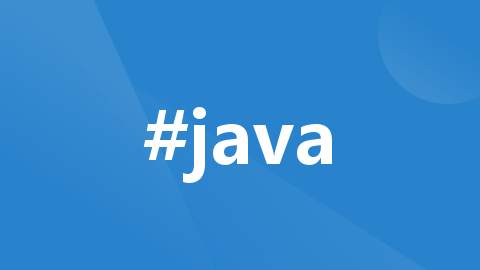
ObjectMapper使用
---这个是将所有非默认值输出。--这个是将所有非Null的输出。--这个是将所有的非’'的输出。
·
ObjectMapper使用
将Java对象序列化和反序列化操作
- fastJson
- Jackson的ObjectMapper操作
跟fastJson区别
- fastJson是阿里开发的,利用Java的反射和泛型机制
- ObjectMapper是jackson开发的,基于流的处理模式,对json字符串进行逐个字符的解析和构建
- 总体来说,fastjson的性能要比objectMapper更加优秀一点
ObjectMapper操作
特别的是使用JsonInclude注解
JsonInclude.Include.ALWAYS
:默认值,始终包含该字段。JsonInclude.Include.NON_NULL
:如果该字段不为 null,则输出该字段。JsonInclude.Include.NON_EMPTY
:如果该字段不为 null 且不为 “”,则输出该字段。JsonInclude.Include.NON_DEFAULT
:如果该字段的值与默认值不同,则输出该字段。
实体类带注解
- 当实体类中的某个字段为null时,不进行序列化
public class User {
private Integer id;
private String name;
@JsonInclude(JsonInclude.Include.NON_NULL)
private Integer age;
// getter and setter methods
}
User user = new User();
user.setId(1);
user.setName("Tom");
// age字段为null
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(user);
System.out.println(json); // 输出:{"id":1,"name":"Tom"}
- 当实体类中的某个字段为空字符串时,不进行序列化
public class User {
private Integer id;
private String name;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
private String email;
// getter and setter methods
}
User user = new User();
user.setId(1);
user.setName("Tom");
user.setEmail(""); // email字段为空字符串
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(user);
System.out.println(json); // 输出:{"id":1,"name":"Tom"}
- 当实体类中的某个字段为默认值时,不进行序列化
public class User {
private Integer id;
private String name;
@JsonInclude(JsonInclude.Include.NON_DEFAULT)
private boolean isAdmin;
// getter and setter methods
}
User user = new User();
user.setId(1);
user.setName("Tom");
// isAdmin字段为false,为boolean类型的默认值
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(user);
System.out.println(json); // 输出:{"id":1,"name":"Tom"}
实体类不带注解,直接用工具类操作
工具类为:
mapper.setSerializationInclusion(JsonInclude.Include.NON_DEFAULT); ----这个是将所有非默认值输出
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL); --这个是将所有非Null的输出
mapper.setSerializationInclusion(JsonInclude.Include.NON_EMPTY); --这个是将所有的非’'的输出
拿Non_Default举例
- 假设现在有个Person类
public class Person {
private String name;
private int age;
private boolean isMale;
private double salary;
// getter and setter methods
}
- 用下面代码进行序列化 —结果为{}
ObjectMapper mapper = new ObjectMapper();
mapper.setSerializationInclusion(JsonInclude.Include.NON_DEFAULT);
Person person = new Person();
String json = mapper.writeValueAsString(person);
System.out.println(json);
-
原因是non_default只会将所有非默认值的属性输出
- 上面的person类
- String默认值null
- int 默认值0
- boolean默认值false
- double 默认值0.0
- 上面代码只有Person person=new Person(); 里面的属性都还是默认值,所有进行non_default的时候,都不会被序列化,只有空串。
-
现在将类的属性赋值 {“age”:18,“isMale”:true}
Person person = new Person();
person.setName("");
person.setAge(18);
person.setMale(true);
person.setSalary(0.0);
String json = mapper.writeValueAsString(person);
System.out.println(json);
由于name属性的值为null或空字符串,因此被忽略。salary属性的值为0.0,因此也被忽略。只有age和isMale属性的值不为默认值,因此被序列化成了JSON字符串。
ObjectMapper工具类提供
public class JsonMapper {
private ObjectMapper mapper;
public JsonMapper() {
this(null);
}
public JsonMapper(JsonInclude.Include include) {
mapper = new ObjectMapper();
//设置输出时包含属性的风格
if (include != null) {
mapper.setSerializationInclusion(include);
}
//设置输入时忽略在JSON字符串中存在但Java对象实际没有的属性
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
/**
* 创建只输出非Null且非Empty(如List.isEmpty)的属性到Json字符串的Mapper,建议在外部接口中使用.
*/
public static JsonMapper nonEmptyMapper() {
return new JsonMapper(JsonInclude.Include.NON_EMPTY);
}
/**
* 创建只输出初始值被改变的属性到Json字符串的Mapper, 最节约的存储方式,建议在内部接口中使用。
*/
public static JsonMapper nonDefaultMapper() {
return new JsonMapper(JsonInclude.Include.NON_DEFAULT);
}
public static JsonMapper defaultMapper() {
return new JsonMapper(JsonInclude.Include.ALWAYS);
}
/**
* Object可以是POJO,也可以是Collection或数组。
* 如果对象为Null, 返回"null".
* 如果集合为空集合, 返回"[]".
*/
public String toJson(Object object) {
try {
return mapper.writeValueAsString(object);
} catch (IOException e) {
System.err.println("write to json string error:" + e);
return null;
}
}
/**
* 反序列化POJO或简单Collection如List<String>.
* <p>
* 如果JSON字符串为Null或"null"字符串, 返回Null.
* 如果JSON字符串为"[]", 返回空集合.
* <p>
* 如需反序列化复杂Collection如List<MyBean>, 请使用fromJson(String,JavaType)
*
* @see # fromJson(String, com.fasterxml.jackson.databind.JavaType)
*/
public <T> T fromJson(String jsonString, Class<T> clazz) {
if (StringUtils.isEmpty(jsonString)) {
return null;
}
try {
return mapper.readValue(jsonString, clazz);
} catch (IOException e) {
System.err.println("parse json string error:" + e);
return null;
}
}
public <T> T fromJson(String jsonString, TypeReference<T> clazz) {
if (StringUtils.isEmpty(jsonString)) {
return null;
}
try {
return mapper.readValue(jsonString, clazz);
} catch (IOException e) {
System.err.println("parse json string error:" + e);
return null;
}
}
/**
* 反序列化复杂Collection如List<Bean>, 先使用函數createCollectionType构造类型,然后调用本函数.
*
* @see #createCollectionType(Class, Class...)
*/
public <T> T fromJson(String jsonString, JavaType javaType) {
if (StringUtils.isEmpty(jsonString)) {
return null;
}
try {
return (T) mapper.readValue(jsonString, javaType);
} catch (IOException e) {
System.err.println("from json string error:" + e);
return null;
}
}
/**
* 構造泛型的Collection Type如:
* ArrayList<MyBean>, 则调用constructCollectionType(ArrayList.class,MyBean.class)
* HashMap<String,MyBean>, 则调用(HashMap.class,String.class, MyBean.class)
*/
public JavaType createCollectionType(Class<?> collectionClass, Class<?>... elementClasses) {
return mapper.getTypeFactory().constructParametricType(collectionClass, elementClasses);
}
/**
* 輸出JSONP格式數據.
*/
public String toJsonP(String functionName, Object object) {
return toJson(new JSONPObject(functionName, object));
}
/**
* 設定是否使用Enum的toString函數來讀寫Enum,
* 為False時時使用Enum的name()函數來讀寫Enum, 默認為False.
* 注意本函數一定要在Mapper創建後, 所有的讀寫動作之前調用.
*/
public void enableEnumUseToString() {
mapper.enable(SerializationFeature.WRITE_ENUMS_USING_TO_STRING);
mapper.enable(DeserializationFeature.READ_ENUMS_USING_TO_STRING);
}
/**
* 取出Mapper做进一步的设置或使用其他序列化API.
*/
public ObjectMapper getMapper() {
return mapper;
}
更多推荐
所有评论(0)