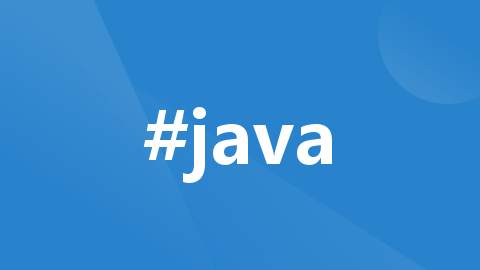
tinyxml类写入操作
title->LinkEndChild(new TiXmlText("背光"));
#include <iostream>
#include <string>
#include "tinyxml.h"
int main() {
TiXmlDocument doc;
TiXmlDeclaration* decl = new TiXmlDeclaration("1.0", "UTF-8", "");
doc.LinkEndChild(decl);
TiXmlElement* root = new TiXmlElement("LedList");
doc.LinkEndChild(root);
// CommSet
TiXmlElement* commSet = new TiXmlElement("CommSet");
root->LinkEndChild(commSet);
TiXmlElement* commNum = new TiXmlElement("CommNum");
commNum->LinkEndChild(new TiXmlText("3"));
commSet->LinkEndChild(commNum);
TiXmlElement* rate = new TiXmlElement("rate");
rate->LinkEndChild(new TiXmlText("57600"));
commSet->LinkEndChild(rate);
TiXmlElement* data = new TiXmlElement("data");
data->LinkEndChild(new TiXmlText("8"));
commSet->LinkEndChild(data);
TiXmlElement* stopbit = new TiXmlElement("stopbit");
stopbit->LinkEndChild(new TiXmlText("1"));
commSet->LinkEndChild(stopbit);
TiXmlElement* parity = new TiXmlElement("parity");
parity->LinkEndChild(new TiXmlText("No"));
commSet->LinkEndChild(parity);
TiXmlElement* flow = new TiXmlElement("flow");
flow->LinkEndChild(new TiXmlText("No"));
commSet->LinkEndChild(flow);
// LedA to LedZ
for (char c = 'A'; c <= 'Z'; c++) {
std::string name = "Led" + std::string(1, c);
TiXmlElement* led = new TiXmlElement(name.c_str());
root->LinkEndChild(led);
led->SetAttribute("CCD", std::to_string(c - 'A' + 1).c_str());
TiXmlElement* enable = new TiXmlElement("enable");
enable->LinkEndChild(new TiXmlText("true"));
led->LinkEndChild(enable);
TiXmlElement* title = new TiXmlElement("title");
title->LinkEndChild(new TiXmlText("背光"));
led->LinkEndChild(title);
TiXmlElement* chn = new TiXmlElement("chn");
chn->LinkEndChild(new TiXmlText(std::to_string(c - 'A').c_str()));
led->LinkEndChild(chn);
}
// Save XML to file
if (doc.SaveFile("led_config.xml")) {
std::cout << "LED configuration saved to file: led_config.xml" << std::endl;
} else {
std::cerr << "Failed to save LED configuration to file: led_config.xml" << std::endl;
}
return 0;
}
更多推荐
所有评论(0)