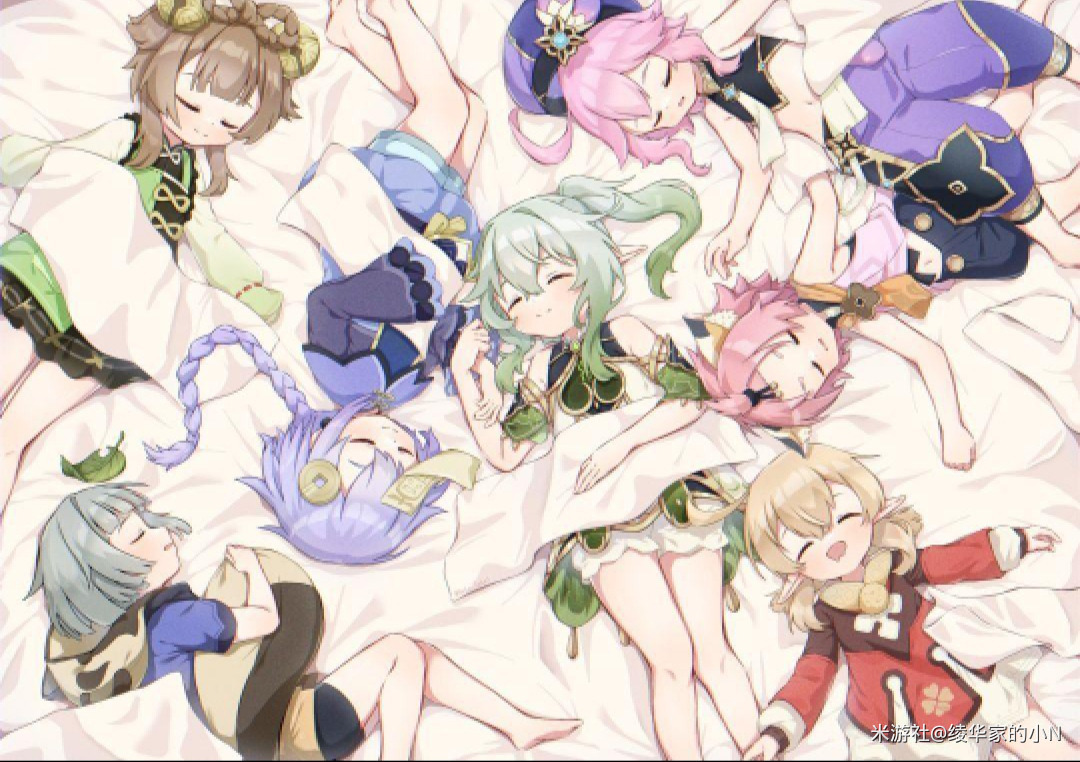
Spring中Bean的十步生命周期详解-----Spring框架
Spring中Bean的十步生命周期详解-----Spring框架
·
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- bean的生命周期五步版本:实例化,赋值,初始化,使用,销毁--> <!-- bean的生命周期七步版本:实例化,赋值,bean后处理器before方法,初始化,bean后处理器after方法,使用,销毁--> <!-- bean的生命周期十步版本:实例化,赋值,检查是否有aware接口,bean后处理器before方法,检查是否实现了InitializingBean接口--> <!-- 初始化,执行Bean后处理器after方法,使用Bean,检查Bean是否实现了DisposableBean接口,销毁Bean--> <!-- aware接口调用后就可以通过传递的classLoader和beanFactory和beanName实现一些内容--> <bean id="user" class="com.powernode.spring6.bean.User" init-method="initUser" destroy-method="destroyUser"> <property name="name" value="Jack"/> </bean> <bean class="com.powernode.spring6.bean.LogBeanPostProcessor"/> </beans>
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- bean的生命周期五步版本:实例化,赋值,初始化,使用,销毁--> <!-- bean的生命周期七步版本:实例化,赋值,bean后处理器before方法,初始化,bean后处理器after方法,使用,销毁--> <!-- bean的生命周期十步版本:实例化,赋值,检查是否有aware接口,bean后处理器before方法,检查是否实现了InitializingBean接口--> <!-- 初始化,执行Bean后处理器after方法,使用Bean,检查Bean是否实现了DisposableBean接口,销毁Bean--> <!-- aware接口调用后就可以通过传递的classLoader和beanFactory和beanName实现一些内容--> <bean id="user" class="com.powernode.spring6.bean.User" init-method="initUser" destroy-method="destroyUser"> <property name="name" value="Jack"/> </bean> <bean class="com.powernode.spring6.bean.LogBeanPostProcessor"/> </beans>
package com.powernode.spring6.bean; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.BeansException; import org.springframework.beans.factory.*; public class User implements BeanNameAware, BeanClassLoaderAware, BeanFactoryAware, InitializingBean,DisposableBean { private static final Logger logger = LoggerFactory.getLogger(User.class); private String name; public User() { logger.info("空参构造器实例化"); } public void setName(String name) { logger.info("赋值"); this.name = name; } public void initUser() { logger.info("初始化"); } public void destroyUser() { logger.info("销毁"); } @Override public void setBeanClassLoader(ClassLoader classLoader) { //ClassLoader的方法 logger.info("bean的类加载器是" + classLoader); } @Override public void setBeanFactory(BeanFactory beanFactory) throws BeansException { logger.info("bean的工厂是" + beanFactory); } @Override public void setBeanName(String name) { //在这里使用Bean的名字 logger.info("bean的名字是" + name); } @Override public void afterPropertiesSet() throws Exception { logger.info("initializingBean的afterPropertiesSet方法执行"); } @Override public void destroy() throws Exception { logger.info("DisposableBean的destroy方法执行"); } }
package com.powernode.spring6.bean; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.BeansException; import org.springframework.beans.factory.*; public class User implements BeanNameAware, BeanClassLoaderAware, BeanFactoryAware, InitializingBean,DisposableBean { private static final Logger logger = LoggerFactory.getLogger(User.class); private String name; public User() { logger.info("空参构造器实例化"); } public void setName(String name) { logger.info("赋值"); this.name = name; } public void initUser() { logger.info("初始化"); } public void destroyUser() { logger.info("销毁"); } @Override public void setBeanClassLoader(ClassLoader classLoader) { //ClassLoader的方法 logger.info("bean的类加载器是" + classLoader); } @Override public void setBeanFactory(BeanFactory beanFactory) throws BeansException { logger.info("bean的工厂是" + beanFactory); } @Override public void setBeanName(String name) { //在这里使用Bean的名字 logger.info("bean的名字是" + name); } @Override public void afterPropertiesSet() throws Exception { logger.info("initializingBean的afterPropertiesSet方法执行"); } @Override public void destroy() throws Exception { logger.info("DisposableBean的destroy方法执行"); } }
package com.powernode.spring6.test; import com.powernode.spring6.bean.User; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Test { @org.junit.Test public void TestBeanInitiation() { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring.xml"); User user = applicationContext.getBean("user",User.class); System.out.println(user); ClassPathXmlApplicationContext classPathXmlApplicationContext = (ClassPathXmlApplicationContext) applicationContext; classPathXmlApplicationContext.close(); } }
package com.powernode.spring6.test; import com.powernode.spring6.bean.User; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Test { @org.junit.Test public void TestBeanInitiation() { ApplicationContext applicationContext = new ClassPathXmlApplicationContext("spring.xml"); User user = applicationContext.getBean("user",User.class); System.out.println(user); ClassPathXmlApplicationContext classPathXmlApplicationContext = (ClassPathXmlApplicationContext) applicationContext; classPathXmlApplicationContext.close(); } }
更多推荐
所有评论(0)