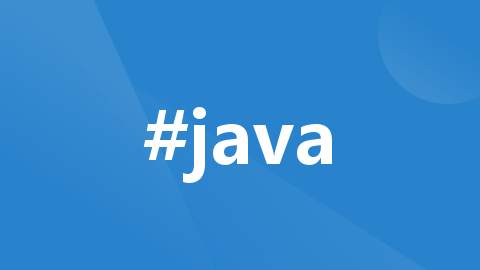
Spring Cloud Gateway Server MVC
之前你如果要用spring cloud gateway ,就必须是webflux 的,也就是必须是异步响应式编程。不能和spring mvc 一起使用。现在spring cloud 新出了一个可以不用webflux的gateway。
·
之前你如果要用spring cloud gateway ,就必须是webflux 的,也就是必须是异步响应式编程。不能和spring mvc 一起使用。现在spring cloud 新出了一个可以不用webflux的gateway。
具体使用mvc的gateway步骤如下
普通的Eureka Client的项目
如果你只是想测试一下gateway mvc,可以建一个普通的spring boot项目,然后写一个/test rest api就可以了。
application.yml
spring:
application:
name: eureka-client
cloud:
compatibility-verifier:
enabled: false
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
instance:
hostname: localhost
server:
servlet:
context-path: /eureka-client
port: 8080
TestController.java
@RestController
public class TestController {
@RequestMapping(value = "/test", method = RequestMethod.GET)
public String test() {
return "test";
}
}
EurekaClientApplication.java
@SpringBootApplication
@EnableDiscoveryClient
public class EurekaClientApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaClientApplication.class, args);
}
}
访问http://localhost:8080/eureka-client/test
Gateway MVC 的项目
在pom.xml加spring-cloud-starter-gateway-mvc
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<java.version>17</java.version>
<spring-cloud.version>2023.0.0</spring-cloud.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway-mvc</artifactId>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
application.yml
spring:
application:
name: gateway-mvc
server:
port: 8088
RouteConfiguration.java
package com.example.gateway;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.function.RouterFunction;
import org.springframework.web.servlet.function.ServerResponse;
import static org.springframework.cloud.gateway.server.mvc.handler.GatewayRouterFunctions.route;
import static org.springframework.cloud.gateway.server.mvc.handler.HandlerFunctions.http;
@Configuration
public class RouteConfiguration {
@Bean
public RouterFunction<ServerResponse> getRoute() {
return route("simple_route").GET("/eureka-client/**", http("http://localhost:8080")).build();
}
}
或者用配置文件的方式.application.yml
spring:
cloud:
gateway:
mvc:
routes:
- id: simple_route
uri: http://localhost:8080
predicates:
- Path=/eureka-client/**
GatewayApplication.java
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
访问http://localhost:8080/eureka-client/test
如果你不想hardcode 你的hostname和端口,也可以用Eureka 的方式来获取hostname和端口号
@Bean
public RouterFunction<ServerResponse> gatewayRouterFunctionsAddReqHeader() {
return route("api_route")
.GET("/eureka-client/**", http())
.filter(lb("EUREKA-CLIENT"))
.build();
}
在application.properties配置的实现方式
spring:
cloud:
gateway:
mvc:
routes:
- id: simple_route
uri: lb://EUREKA-CLIENT
predicates:
- Path=/eureka-client/**
filters:
- StripPrefix=1
FAQ
怎么去掉server.servlet.contex-path?
spring.cloud.gateway.mvc.routes[0].filters[0]=RewritePath=${server.servlet.context-path}(?<remaining>.*), \${remaining}
更多推荐
所有评论(0)