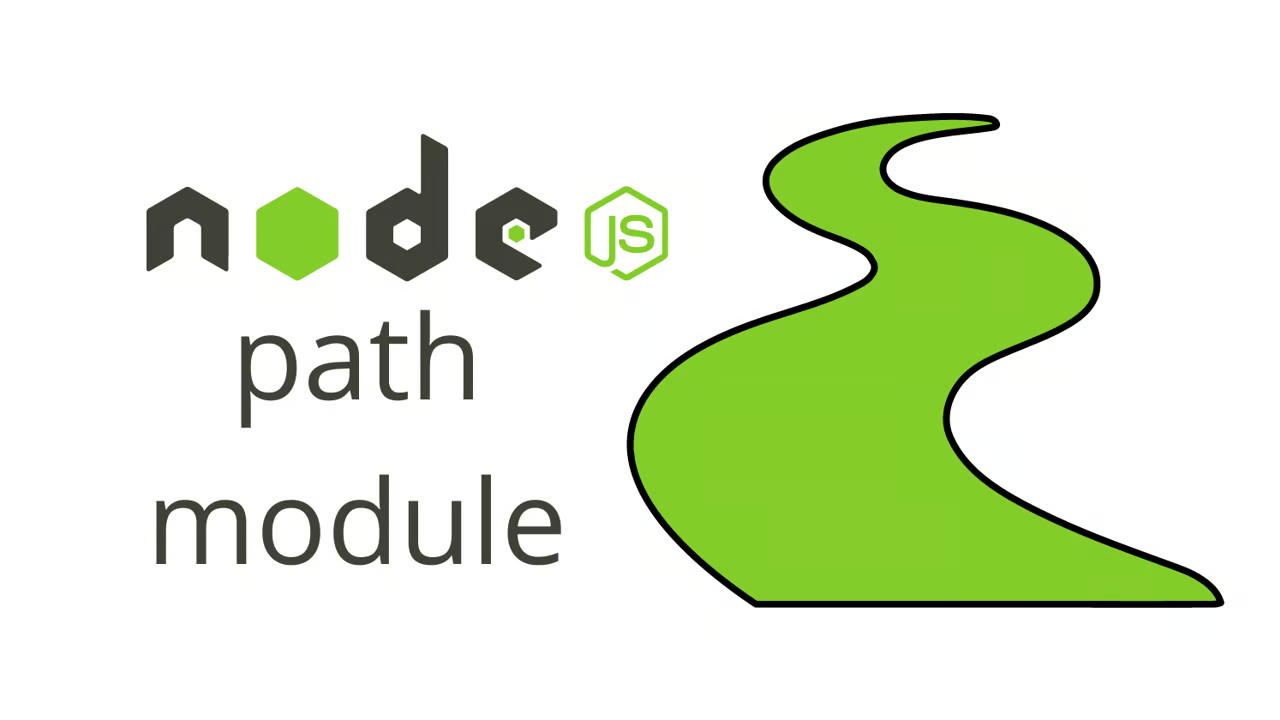
Node JS 基础知识:Node JS 中的路径模块
Node.js提供了异步和事件驱动的架构,但最重要的是Node.js的核心模块。Node.js有许多模块,使开发人员的生活更轻松,其中一个是 Node JS 中的路径模块,它非常高效和有用,为我们提供了访问和操作文件系统中。此方法解析从右侧到左侧的路径,并在构造生成的绝对路径之前为每个路径添加前缀。path 模块中有许多重要且有用的函数,用于在开发项目时处理文件或目录的路径。通俗地说,它返回目录的
概述
自 2009 年首次发布以来,Node.js 变得非常受欢迎。Node.js提供了异步和事件驱动的架构,但最重要的是Node.js的核心模块。Node.js有许多模块,使开发人员的生活更轻松,其中一个是 Node JS 中的路径模块,它非常高效和有用,为我们提供了访问和操作文件系统中路径的方法。通常,路径模块有助于使文件路径系统独立于任何系统环境。
先决条件
在 Node.js 中使用路径模块没有主要的先决条件。 您只需要在当前系统中安装 LTS(长期支持)或最新版本的 Node.js。作为 Node.js 核心模块的一部分,您不需要从任何外部源安装路径或使用 npm;您只需要 Node JS 中的 path 模块。
语法:
// Importing the path module
const path = require('path')
Node.js路径模块简介
许多人忘记了Node.js最简单和最重要的核心模块之一,路径模块。node js 中的 path 模块用于管理和更改文件路径。它是核心模块的一部分,其方法可帮助您处理本地计算机文件系统上的目录和文件路径名。 每个系统都有不同的环境和特定的操作系统来管理它。不同的操作系统以不同的方式管理与文件和目录相关的操作。
例如
在 Linux 中,文件的路径在目录之间使用“/”表示。 在 Windows 中,文件的路径通过在目录之间使用“\”来表示。 在宏中,文件的路径通过在目录之间使用“\”和“/”来表示。
LINUX:home/scaler/topics/file.txt
WINDOWS:C:\\user\scaler\topics\file.txt
宏:C:\user\scaler\topics\Img/nodejs.svg
为了使这些文件和目录路径独立于平台,Node.js有一个称为路径模块的模块。 path 模块中有许多重要且有用的函数,用于在开发项目时处理文件或目录的路径。那么我们就来一一了解 node js 中 path 模块中的那些重要函数。
语法
在任何Node.js项目中要求 path 模块的语法都非常简单明了。
const path = require('path')
例:
使用以下代码创建文件main.js
// Importing the path module in node js
const path = require('path');
// basename method gives the file name from path name
const filename = path.basename('/Users/scaler/demo_path.js');
console.log(filename);
使用以下命令运行上述文件
$ node main.js
输出:
demo_path.js
Node.js路径模块的方法
下面我们来一一讨论一下 node js 中 path 模块的方法:
Node.js path.basename() 方法:
path.basename 方法用于获取文件名的尾部。使用此方法时,将省略尾随目录分隔符。通俗地说,它返回目录的名称或文件路径引用的文件。
语法:
path.basename(path[, ext])
参数说明:此方法除以下两个参数外。
- path : <string> 这是用于提取文件名的文件路径或目录路径。
- ext : <string> 这是一个可选参数,用于删除指定文件的文件扩展名。
- 返回值:<string>此方法返回指定文件字符串的文件名。如果给定的文件路径不是字符串值或扩展名,并且不是字符串值,则会引发 TypeError。
例:
假设我们想知道图像的名称,并且我们以字符串的形式传递了整个文件路径。
// Importing the path module in node js
const path = require('path');
// file path of the image in your system
const filepath = 'C:/Users/.../path-module/images/scaler/william-smith.png';
// imageName will store the filename with extention from the given filepath
const imageName = path.basename(filepath);
console.log('Name of image: ', imageName);
输出:
Name of image: william-smith.png
现在假设我们想删除文件名的扩展名,那么
// Importing the path module in node js
const path = require('path');
// file path of the image in your system
const filepath = 'C:/Users/.../path-module/images/scaler/william-smith.png';
// imageName will store the filename without extention from the given filepath
const imageName = path.basename(filepath, '.png');
console.log('Name of image: ', imageName);
输出:
Name of image: william-smith
尽管 Windows 通常以不区分大小写的方式处理文件名及其扩展名,但 path.basename 不会。 例如,在 Windows 中,C:\\filename.html 和 C:\\filename.HTML 引用同一个文件,但 path.basename 将扩展名视为区分大小写的字符串:
Node.js path.dirname() 方法
path.dirname 方法用于获取存在特定文件的目录名称。当我们想知道最低的目录名称但有一个指向目录内文件的完整路径名时,此方法很有用。path.dirname 方法忽略尾随目录分隔符。
语法:
path.dirname(path)
参数说明:此方法除以下两个参数外。
- path : <string> 此方法接受单个参数,该参数是要知道其目录的特定文件的文件路径名。
- 返回值:<string>此方法返回指定文件字符串的最低目录名称。如果文件路径不是字符串值,则会引发 TypeError。
例:
假设我们想知道存在图像的目录名称,并且我们以字符串的形式传递了整个文件路径。
// Importing the path module in node js
const path = require('path');
// file path of the image in your system
const filepath = 'C:/Users/path-module/images/scaler/william-smith.png';
// directoryName will store the lowest directory of the particular image
const directoryName = path.dirname(filepath);
console.log('Directory structure is: ', directoryName);
输出:
Directory structure is: C:/Users/path-module/images/scaler
Node.js path.extname() 方法
path.extname 方法用于获取文件路径字符串中指定文件的扩展名。它将从句点 (.) 字符的最后一次出现提取到文件路径字符串末尾的文件扩展名。如果文件路径中没有句点 (.) 字符,则返回一个空字符串。
语法:
path.extname(path)
参数说明:此方法不包括以下两个参数。
- path : <string> 此方法接受单个参数,该参数是要知道其扩展名的特定文件的文件路径。
- 返回值:<string> 此方法返回文件路径中指定文件的扩展名。如果文件路径不是字符串值,则会引发 TypeError。
例:
// Importing the path module in node js
const path = require('path');
// file path of the markdown from your system
const path1 = path.extname("readme.md");
console.log(path1)
// file path of the text from your system
const path2 = path.extname("hello.txt");
console.log(path2)
// File with blank extension hence return only the period (.)
const path3 = path.extname("example.")
console.log(path3)
// File with no extension hence returns an empty string
const path4 = path.extname("fileDump")
console.log(path4)
// File with two periods only return from the last occurence
const path5 = path.extname("readme.md.txt")
console.log(path5)
// Extension name of the current script
const path6 = path.extname(__filename)
console.log(path6)
输出:
.md
.txt
.
.txt
.js
Node.js path.format() 方法
path.format 方法用于从给定的路径对象中获取路径字符串。path.format 方法接收 pathObject,它是许多属性的组合,其中一个属性可能优先于另一个属性。 一些优先顺序如下:
- 如果 Object 中提供了 pathObject.base 参数,则忽略 path 对象的 pathObject.ext 和 pathObject.name 参数。
- .如果在 Object 中提供了 pathObject.dir 参数,则忽略路径 Object 的 pathObject.root 参数。
语法:
path.format(pathObject)
参数说明:此方法不包括以下两个参数。
- pathObject : <Object> 具有以下属性的任何 JavaScript 对象:
- dir : <string> 指定路径 Object 的目录名称。
- root : <string> 这指定路径 Object 的根。
- base : <string> 这指定路径 Object 的基数。
- name : <string> 这指定路径 Object 的名称。
- ext : <string> 这指定路径 Object 的文件扩展名。
- 返回值:此方法从提供的路径 Object 返回路径字符串。它基本上与 path.parse 相反
例:
在POSIX上,
// Importing the path module in node js
const path = require('path');
// CASE 1:
// If "root", "dir" and "base" are all given, then "root" is ignored.
const path1 = path.format({
root: "/ignored/root/",
dir: "/home/user/scaler",
base: "topics.txt",
});
console.log("Path 1:", path1);
// CASE 2:
// If "dir" is not specified then "root" will be used
// If only "root" is provided
// platform separator will not be included.
// "ext" will be ignored.
const path2 = path.format({
root: "/",
base: "lostcause.dat",
ext: ".noextension",
});
console.log("Path 2:", path2);
// CASE 3:
// If "base" is not specified
// "name" and "ext" will be used
const path3 = path.format({
root: "/movies/",
name: "Rush",
ext: ".mp3",
});
console.log("Path 3:", path3);
输出:
Path 1: /home/user/scaler/topics.txt
Path 2: /lostcause.dat
Path 3: /movies/Rush.mp3
在 Windows 上,
// Importing the path module
const path = require('path');
const path1 = path.format({
dir: 'C:\\path\\dir',
base: 'details.txt'
});
console.log("Path 1:", path1);
输出:
Path 1: C:\\path\\dir\\details.txt
Node.js path.isAbsolute() 方法:
path.isAbsolute 方法用于检查指定的路径是否为绝对路径。绝对路径被描述为包含查找文件所需的全部详细信息的路径。
语法:
path.isAbsolute(path)
参数说明:此方法不包括以下两个参数。
- path : <string> 此方法接受单个参数,该参数是指定文件的文件路径,用于检查输入路径是否为绝对路径。
- 返回值:<bool>此方法返回一个 true 或 false 值,指示路径是否为绝对路径。如果文件路径不是字符串值,则会引发 TypeError。如果文件路径的长度为零,它还返回一个 false 值。
例:
在POSIX上,
// Importing the path module in node js
const path = require('path');
const path1 = path.isAbsolute("/user/scaler/");
console.log(path1);
const path2 = path.isAbsolute("user/scaler/detail.txt");
console.log(path2);
const path3 = path.isAbsolute("..");
console.log(path3);
输出:
true
false
false
在 Windows 上,
// Importing the path module
const path = require('path');
const path1 = path.isAbsolute("\\user\\scaler\\");
console.log(path1);
const path2 = path.isAbsolute("user\\scaler\\details.text");
console.log(path2);
const path3 = path.isAbsolute("bar/baz");
console.log(path3);
输出:
true
false
false
Node.js path.join() 方法
path.join 方法用于使用特定于平台的分隔符将两个、三个或多个路径段联接到单个路径中。 要连接的路径段使用逗号分隔值进行定义。归一化发生在所有路径联接后。生成的字符串可以根据需要在任何地方使用。 如果输入中提供的任何路径段的长度为零,则忽略这些路径段。 如果通过连接路径段字符串形成的结果路径长度为零,则 (.) 将作为结果返回,表示当前工作目录。
语法:
path.join([...paths])
参数说明:此方法不包括以下两个参数。
- path: <string> 此方法接受参数,这些参数是要联接的逗号分隔的文件路径。
- 返回值:<string> 此方法在连接所有逗号分隔路径并对其进行规范化后返回字符串(文件路径)。如果任何逗号分隔的文件路径不是字符串值,则会引发 TypeError。
例:
// Importing the path module in node js
const path = require('path');
// Joining 2 comma separated path-segments
const path1 = path.join("abc/admin/files", "deatils.txt");
console.log(path1)
// Joining 3 comma separate path-segments
const path2 = path.join("abc", "scaler/topics", "deatils.txt");
console.log(path2)
// Joining with non-zero and zero-length paths
const path3 = path.join("abc", "", "", "deatils.txt");
console.log(path3)
// Joining with zero-length paths
const path3 = path.join("", "", "");
console.log(path3)
输出:
abc\admin\files\deatils.txt
abc\scaler\topics\deatils.txt
abc\deatils.txt
.
Node.js path.normalize() 方法
path.normalize() 方法用于从给定路径获取归一化结果。path.normalize() 方法规范化给定的路径,以正确且可读的形式解析 .. 和 . 段。 如果方法遇到多个路径段分隔符(/、\、//、\\),则所有字符都将替换为特定于平台的路径段分隔符的单个实例。path.normalize() 方法保留所有尾随分隔符,甚至能够处理最复杂的路径。
语法:
path.normalize(path)
参数说明:此方法不包括以下两个参数。
- path: <string> 此方法接受单个参数,该参数是要规范化的文件路径。
- 返回值:<string>此方法在规范化字符串(文件路径)后返回字符串。如果文件路径不是字符串值,则会引发 TypeError。
例:
// Importing the path module in node js
const path = require('path');
const path1 = path.normalize("/abcd/scaler/.");
console.log(path1)
const path2 = path.normalize("/abcd/scaler/..");
console.log(path2)
const path3 = path.normalize("/abcd/scaler/../topics")
console.log(path3);
const path4 = path.normalize("/abcd///scaler///topics")
console.log(path4);
// all the path segment separation characters are converted into one
const path5 = path.win32.normalize('C:temp\\\\/\\/\\/foo/bar');
console.log(path5)
输出:
\abcd\scaler
\abcd
\abcd\topics
\abcd\scaler\topics
C:\\temp\\foo\\ba
Node.js path.parse() 方法
对于每个平台,这些属性的值可能不同。它在解析过程中忽略平台的尾随目录分隔符。 path.parse 返回具有以下属性的对象:
- dir : <string>目录名
- root : <string>根名称
- base : <string> 带扩展名的文件名
- name : <string> only 文件名
- ext : <string>唯一的扩展名
语法:
path.parse(path)
参数说明:此方法不包括以下两个参数。
- path: <string> 此方法接受单个参数,该参数是要分析的文件路径。
- 返回值:<string>此方法在分析文件路径后返回一个对象。如果文件路径不是字符串值,则会引发 TypeError。
例:
在POSFIX上,
// Importing the path module in node js
const path = require('path');
const path1 = path.parse("/users/scaler/topics/details.txt");
console.log(path1);
const path2 = path.parse("topics/readme.md");
console.log(path2);
输出:
{
root: '/',
dir: '/users/scaler/topics',
base: 'details.txt',
ext: '.txt',
name: 'details'
}
{
root: '',
dir: 'topics',
base: 'readme.md',
ext: '.md',
name: 'readme'
}
Node.js path.relative() 方法
path.relative 方法用于根据当前工作目录获取从给定路径到另一个路径的相对路径。如果在参数中传递了零长度的字符串,则使用当前工作目录,而不是零长度字符串。如果 from 和 to 的参数相同,则返回长度为零的字符串。
语法:
path.relative(from, to)
参数说明:此方法不包括以下两个参数。
- from <string> 这是将用作基本路径的文件路径。
- to <string>这是将用于查找相对路径的文件路径。
- 返回值:<string>此方法以规范化形式返回字符串。如果参数 from 或 to 不是字符串值,则会引发 TypeError。
例:
// Importing the path module in node js
const path = require('path');
path1 = path.relative("scaler/website", "scaler/nodejs.html");
console.log(path1)
// When both the paths are the same it will return a zero length string
path2 = path.relative("users/scaler", "users/scaler");
console.log(path2)
path3 = path.relative("users/scaler", "scaler/files/website");
console.log(path3)
输出:
..\index.html
..\..\scaler\files\website
Node.js path.resolve() 方法
path.resolve 方法用于获取任何文件的绝对路径。 它用于解析任意系列的路径段。此方法解析从右侧到左侧的路径,并在构造生成的绝对路径之前为每个路径添加前缀。生成的绝对路径将规范化,并根据需要删除以下所有斜杠。 如果参数中提供了零路径段,则使用当前工作目录的绝对路径。此方法将忽略零长度路径。
语法:
path.resolve([...paths])
参数说明:此方法除以下两个参数外。
- path: <string> 此方法接受一系列路径,这些路径将被解析为构造绝对路径。
- 返回值:<string>此方法返回绝对构造路径。如果文件路径不是字符串值,则会引发 TypeError。
例:
// Importing the path module in node js
const path = require('path');
// printing the current directory path
console.log("Current directory:", __dirname);
// Resolving 2 path-segments with the current directory
const path1 = path.resolve("scaler/topics", "details.txt");
console.log(path1)
// Resolving 3 path-segments with the current directory
const path2 = path.resolve("scaler", "topics", "details.txt");
console.log(path2)
// Treating of the first segment as root, ignoring the current directory
const path3 = path.resolve("/scaler/topics", "details.txt");
console.log(path3)
输出:
Current directory: C:\abc\nodejs-resolve-example
C:\abc\nodejs-resolve-example\scaler\topics\details.txt
C:\abc\nodejs-resolve-example\scaler\topics\details.txt
C:\scaler\topics\details.txt
Node.js path.toNamespacedPath() 方法
path.toNamespacedPath 方法用于从给定的输入路径中查找等效的命名空间前缀路径。path.toNamespacedPath 方法仅适用于 Windows 系统,因为它在 POSIX 系统上不起作用。在 POSTIX 系统上,它只返回相同的路径,无需修改。如果路径不是字符串,则将返回该路径而不进行修改。
语法:
path.toNamespacedPath(path)
参数说明:此方法不包括以下两个参数。
- path: <string> 此方法接受要转换的路径。
- 返回值:<string> 此方法返回具有等效命名空间前缀路径的字符串。
例:
// Importing the path module in node js
const path = require('path');
let originalPath = "C:\\Windows\\abcd";
console.log("Original Path is:", originalPath);
let nameSpacedPath = path.toNamespacedPath(originalPath);
console.log("Namespaced Path is:", nameSpacedPath);
输出:
Original Path is: C:\Windows\abcd
Namespaced Path is: \\?\C:\Windows\abcd
node js 中路径模块的所有方法简述:
方法 | 描述 |
---|---|
basename() | 此方法返回文件名的尾随部分 |
delimiter | 此方法返回平台的指定分隔符 |
dirname() | 此方法返回路径名的最低目录 |
extname() | 此方法返回路径名的文件扩展名 |
format() | 此方法将路径名对象格式化为路径名字符串 |
isAbsolute() | 如果路径名是绝对路径名,则此方法返回 true,否则此方法返回 false |
join() | 此方法将指定的逗号分隔的路径名联接到一个路径中 |
normalize() | 此方法规范化指定的路径名 |
parse() | 此方法将路径名字符串格式化为路径名对象 |
posix | 此方法返回一个包含特定于 POSIX 的属性和方法的对象 |
relative() | 此方法将相对路径名从一个指定路径名返回到另一个指定路径名 |
resolve() | 此方法将指定的路径名解析为绝对路径名 |
sep | 此方法返回为平台指定的段分隔符 |
win32 | 此方法返回一个对象,其中包含特定于 Windows 的属性和方法 |
准备好创建超快的应用程序了吗?报名参加我们的免费 Node JS 认证课程,学习优化您的 Web 项目以提高速度。
结论
- Node.js 是一个开源的跨平台 Javascript 运行时环境,在内部运行在 chrome 的 V8 引擎上。
- Node.js核心模块包含一个路径模块,用于与系统中存在的文件路径进行交互。
- node js 中的 path 模块使文件路径系统独立于任何系统环境。
- 路径模块是Node.js核心模块的一部分,因此我们不需要从任何外部资源(如 npm(节点包管理器))下载它。
- 路径模块可以在项目中使用,只需它。常量路径 = require('path');
- 通常,Node.js中的 Path 模块提供的方法仅采用字符串参数,如果参数不是字符串,则引发 TypeError。
- 节点 js 中的 path 模块包含许多导入方法,其中一些是上面解释的 dirname()、join()、extname()、basename() 和 normalize()。
- Path 模块还包含 path.toNamespacedPath() 方法,该方法仅适用于 Windows 环境。
更多推荐
所有评论(0)