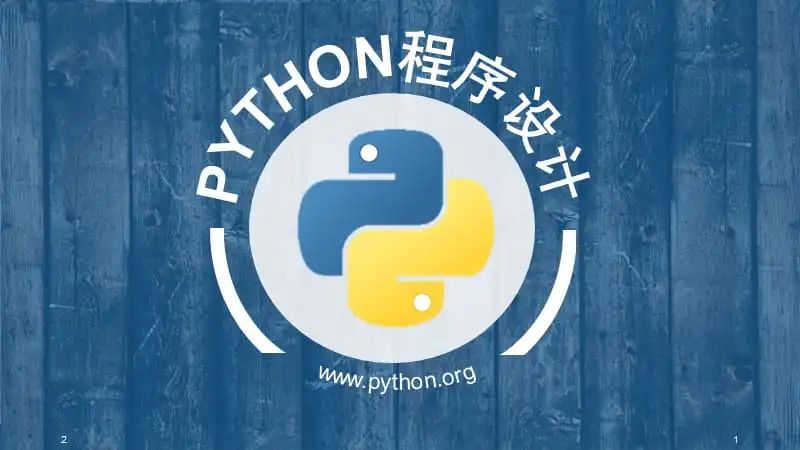
Python爬虫教程:从入门到实战
这篇文章全面涵盖了Python爬虫的核心概念和实际操作,提供了从基础知识到高级技巧的全面指南。深入剖析了HTTP请求、HTML解析,以及静态和动态网页爬取的基本原理。通过requests和Selenium等库的灵活运用,大家能够轻松获取和处理网页数据。数据存储方面,介绍了将数据保存到文件和数据库的方法,帮助大家有效管理爬取到的信息。高级主题涵盖了多线程、异步操作、使用代理、防反爬虫策略等内容,能够

更多Python学习内容:ipengtao.com
大家好,我是涛哥,今天为大家分享 Python爬虫教程:从入门到实战,文章3800字,阅读大约15分钟,大家enjoy~~
网络上的信息浩如烟海,而爬虫(Web Scraping)是获取和提取互联网信息的强大工具。Python作为一门强大而灵活的编程语言,拥有丰富的库和工具,使得编写爬虫变得更加容易。本文将从基础的爬虫原理和库介绍开始,逐步深入,通过实际示例代码,带领读者学习Python爬虫的使用和技巧,掌握从简单到复杂的爬虫实现。
1. 基础知识
1.1 HTTP请求
在开始爬虫之前,了解HTTP请求是至关重要的。Python中有许多库可以发送HTTP请求,其中requests
库是一个简单而强大的选择。
import requests
response = requests.get("https://www.example.com")
print(response.text)
1.2 HTML解析
使用BeautifulSoup
库可以方便地解析HTML文档,提取所需信息。
from bs4 import BeautifulSoup
html = """
<html>
<body>
<p>Example Page</p>
<a href="https://www.example.com">Link</a>
</body>
</html>
"""
soup = BeautifulSoup(html, 'html.parser')
print(soup.get_text())
2. 静态网页爬取
2.1 简单示例
爬取静态网页的基本步骤包括发送HTTP请求、解析HTML并提取信息。
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取标题
title = soup.title.text
print(f"Title: {title}")
# 提取所有链接
links = soup.find_all('a')
for link in links:
print(link['href'])
2.2 处理动态内容
对于使用JavaScript渲染的网页,可以使用Selenium
库模拟浏览器行为。
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
url = "https://www.example.com"
driver = webdriver.Chrome()
driver.get(url)
# 模拟滚动
driver.find_element_by_tag_name('body').send_keys(Keys.END)
# 提取渲染后的内容
rendered_html = driver.page_source
soup = BeautifulSoup(rendered_html, 'html.parser')
# 进一步处理渲染后的内容
3. 数据存储
3.1 存储到文件
将爬取的数据存储到本地文件是一种简单有效的方法。
import requests
url = "https://www.example.com"
response = requests.get(url)
with open('example.html', 'w', encoding='utf-8') as file:
file.write(response.text)
3.2 存储到数据库
使用数据库存储爬取的数据,例如使用SQLite
。
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# 创建表
cursor.execute('''CREATE TABLE IF NOT EXISTS pages (id INTEGER PRIMARY KEY, url TEXT, content TEXT)''')
# 插入数据
url = "https://www.example.com"
content = response.text
cursor.execute('''INSERT INTO pages (url, content) VALUES (?, ?)''', (url, content))
# 提交并关闭连接
conn.commit()
conn.close()
4. 处理动态网页
4.1 使用API
有些网站提供API接口,直接请求API可以获得数据,而无需解析HTML。
import requests
url = "https://api.example.com/data"
response = requests.get(url)
data = response.json()
print(data)
4.2 使用无头浏览器
使用Selenium
库模拟无头浏览器,适用于需要JavaScript渲染的网页。
from selenium import webdriver
url = "https://www.example.com"
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式
driver = webdriver.Chrome(options=options)
driver.get(url)
# 处理渲染后的内容
5. 高级主题
5.1 多线程和异步
使用多线程或异步操作可以提高爬虫的效率,特别是在爬取大量数据时。
import requests
from concurrent.futures import ThreadPoolExecutor
def fetch_data(url):
response = requests.get(url)
return response.text
urls = ["https://www.example.com/1", "https://www.example.com/2", "https://www.example.com/3"]
with ThreadPoolExecutor(max_workers=5) as executor:
results = list(executor.map(fetch_data, urls))
for result in results:
print(result)
5.2 使用代理
为了防止被网站封禁IP,可以使用代理服务器。
import requests
url = "https://www.example.com"
proxy = {
'http': 'http://your_proxy_here',
'https': 'https://your_proxy_here'
}
response = requests.get(url, proxies=proxy)
print(response.text)
6. 防反爬虫策略
6.1 限制请求频率
设置适当的请求间隔,模拟人类操作,避免过快爬取。
import time
url = "https://www.example.com"
for _ in range(5):
response = requests.get(url)
print(response.text)
time.sleep(2) # 2秒间隔
6.2 使用随机User-Agent
随机更换User-Agent头部,降低被识别为爬虫的概率。
import requests
from fake_useragent import UserAgent
ua = UserAgent()
headers = {'User-Agent': ua.random}
url = "https://www.example.com"
response = requests.get(url, headers=headers)
print(response.text)
总结
这篇文章全面涵盖了Python爬虫的核心概念和实际操作,提供了从基础知识到高级技巧的全面指南。深入剖析了HTTP请求、HTML解析,以及静态和动态网页爬取的基本原理。通过requests
、BeautifulSoup
和Selenium
等库的灵活运用,大家能够轻松获取和处理网页数据。数据存储方面,介绍了将数据保存到文件和数据库的方法,帮助大家有效管理爬取到的信息。高级主题涵盖了多线程、异步操作、使用代理、防反爬虫策略等内容,能够更高效地进行爬虫操作,并规避反爬虫机制。最后,提供了良好的实践建议,包括设置请求频率、使用随机User-Agent等,以确保爬虫操作的合法性和可持续性。
总体而言,本教程通过生动的示例代码和详实的解释,为学习和实践Python爬虫的读者提供了一份全面而实用的指南。希望大家通过学习本文,能够在实际应用中灵活驾驭爬虫技术,更深入地探索网络世界的无限可能。
如果你觉得文章还不错,请大家 点赞、分享、留言 下,因为这将是我持续输出更多优质文章的最强动力!
更多Python学习内容:ipengtao.com
干货笔记整理
最经典的编程教材《Think Python》开源中文版.PDF下载
点击“阅读原文”,获取更多学习内容
更多推荐
所有评论(0)