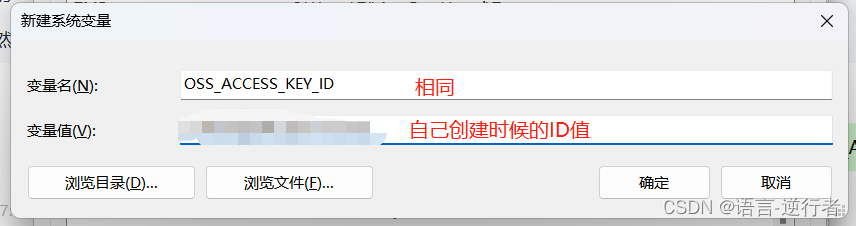
阿里云对象存储oss-文件上传过程详解(两种方式)
阿里云对象存储oss-文件上传过程详解{两种方式}方式一(最新代码,时间:2023/8/27)(1)如何配置系统变量(2)完整代码方式二(跟黑马最新教程同代码)(1)在复制下来的代码中(2)完整代码
·
阿里云对象存储oss-文件上传过程详解{两种方式}
方式一(最新代码,时间:2023/8/27)
问题:需要配置系统变量才能够使用
(1)如何配置系统变量
以win11为例
- (1)打开设置
- (2)选择----系统信息
- (3)选择----高级系统设置
- (4)选择----环境变量
- (5)选择----系统变量这栏-----新建
- (6)在“变量名”输入框中输入 OSS_ACCESS_KEY_ID,在“变量值”输入框中输入你的Access Key ID。再次点击“新建”按钮,输入变量名为 OSS_ACCESS_KEY_SECRET,变量值为您的Access Key Secret。
- (7)点击“确定”保存设置。
(2)完整代码
代码复制下来基本不变,只需要更改对应位位置的值,改成自己的对象存储oss的相关信息.包括:
-
endpoint 是用来指定阿里云 OSS 服务的访问域名或URL。
-
更改成自己的Bucket名称,例如web-3tlias。
-
填写Object完整路径,完整路径中不能包含Bucket名称,例如
String objectName = "1.txt";
表示存在Bucket该目录下 -
填写本地文件的完整路径,例如
D:C:\\Users\\ZJ\\Desktop\\1.txt。
如果未指定本地路径,则默认从示例程序所属项目对应本地路径中上传文件流。sql String filePath= "C:\\Users\\ZJ\\Desktop\\1.txt";
import com.aliyun.oss.ClientException;
import com.aliyun.oss.OSS;
import com.aliyun.oss.common.auth.*;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.OSSException;
import com.aliyun.oss.model.PutObjectRequest;
import com.aliyun.oss.model.PutObjectResult;
import java.io.FileInputStream;
import java.io.InputStream;
public class Demo {
public static void main(String[] args) throws Exception {
// Endpoint以华北2(北京)为例,其它Region请按实际情况填写。
String endpoint = "https://oss-cn-beijing.aliyuncs.com";
// 从环境变量中获取访问凭证。运行本代码示例之前,请确保已设置环境变量OSS_ACCESS_KEY_ID和OSS_ACCESS_KEY_SECRET。
EnvironmentVariableCredentialsProvider credentialsProvider = CredentialsProviderFactory.newEnvironmentVariableCredentialsProvider();
// 填写Bucket名称,例如web-3tlias。
String bucketName = "web-3tlias";
// 填写Object完整路径,完整路径中不能包含Bucket名称,例如1.txt。
String objectName = "1.txt";
// 填写本地文件的完整路径,例如D:C:\\Users\\ZJ\\Desktop\\1.txt。
// 如果未指定本地路径,则默认从示例程序所属项目对应本地路径中上传文件流。
String filePath= "C:\\Users\\ZJ\\Desktop\\1.txt";
// 创建OSSClient实例。
OSS ossClient = new OSSClientBuilder().build(endpoint, credentialsProvider);
try {
InputStream inputStream = new FileInputStream(filePath);
// 创建PutObjectRequest对象。
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, objectName, inputStream);
// 创建PutObject请求。
PutObjectResult result = ossClient.putObject(putObjectRequest);
} catch (OSSException oe) {
System.out.println("Caught an OSSException, which means your request made it to OSS, "
+ "but was rejected with an error response for some reason.");
System.out.println("Error Message:" + oe.getErrorMessage());
System.out.println("Error Code:" + oe.getErrorCode());
System.out.println("Request ID:" + oe.getRequestId());
System.out.println("Host ID:" + oe.getHostId());
} catch (ClientException ce) {
System.out.println("Caught an ClientException, which means the client encountered "
+ "a serious internal problem while trying to communicate with OSS, "
+ "such as not being able to access the network.");
System.out.println("Error Message:" + ce.getMessage());
} finally {
if (ossClient != null) {
ossClient.shutdown();
}
}
}
}
方式二(跟黑马最新教程同代码)
(1)在复制下来的代码中
- 将那个自动获取的实例删除
EnvironmentVariableCredentialsProvider credentialsProvider = CredentialsProviderFactory.newEnvironmentVariableCredentialsProvider();
这个实例用于获取环境变量中的凭证信息。
- 然后重新加入
String accessKeyId="/your accessKeyId";
String accessKeySecret="your accessKeySecret";
- 然后将
OSS ossClient = new OSSClientBuilder().build(endpoint, credentialsProvider);
这一句改成
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId,accessKeySecret);
(2)完整代码
import com.aliyun.oss.ClientException;
import com.aliyun.oss.OSS;
import com.aliyun.oss.common.auth.*;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.OSSException;
import com.aliyun.oss.model.PutObjectRequest;
import com.aliyun.oss.model.PutObjectResult;
import java.io.FileInputStream;
import java.io.InputStream;
public class Demo {
public static void main(String[] args) throws Exception {
// Endpoint以华北2(北京)为例,其它Region请按实际情况填写。
String endpoint = "https://oss-cn-beijing.aliyuncs.com";
// 从环境变量中获取访问凭证。运行本代码示例之前,请确保已设置环境变量OSS_ACCESS_KEY_ID和OSS_ACCESS_KEY_SECRET。
//EnvironmentVariableCredentialsProvider credentialsProvider = CredentialsProviderFactory.newEnvironmentVariableCredentialsProvider();
String accessKeyId="LTAI5t7jEXfK1jYtY1rCPtc9"; //your accessKeyId
String accessKeySecret="cQjd70rASM7mwmefIwm4DEbgRtph9s";//your accessKeySecret
// 填写Bucket名称,例如web-3tlias。
String bucketName = "web-3tlias";
// 填写Object完整路径,完整路径中不能包含Bucket名称,例如1.txt。
String objectName = "1.txt";
// 填写本地文件的完整路径,例如D:C:\Users\ZJ\Desktop\1.txt。
// 如果未指定本地路径,则默认从示例程序所属项目对应本地路径中上传文件流。
String filePath= "C:\\Users\\ZJ\\Desktop\\1.txt";
// 创建OSSClient实例。
//OSS ossClient = new OSSClientBuilder().build(endpoint, credentialsProvider);
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId,accessKeySecret);
try {
InputStream inputStream = new FileInputStream(filePath);
// 创建PutObjectRequest对象。
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, objectName, inputStream);
// 创建PutObject请求。
PutObjectResult result = ossClient.putObject(putObjectRequest);
} catch (OSSException oe) {
System.out.println("Caught an OSSException, which means your request made it to OSS, "
+ "but was rejected with an error response for some reason.");
System.out.println("Error Message:" + oe.getErrorMessage());
System.out.println("Error Code:" + oe.getErrorCode());
System.out.println("Request ID:" + oe.getRequestId());
System.out.println("Host ID:" + oe.getHostId());
} catch (ClientException ce) {
System.out.println("Caught an ClientException, which means the client encountered "
+ "a serious internal problem while trying to communicate with OSS, "
+ "such as not being able to access the network.");
System.out.println("Error Message:" + ce.getMessage());
} finally {
if (ossClient != null) {
ossClient.shutdown();
}
}
}
}
更多推荐
所有评论(0)