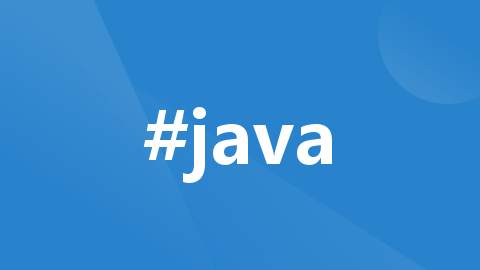
Java连接MySQL数据库具体操作
mysql.8及以上的版本获取驱动是Class.forName("com.mysql.cj.jdbc.Driver");mysql.5及以下的版本获取驱动是Class.forName("com.mysql.jdbc.Driver");db0613是自己创建的数据库,root表示数据库用户名,123表示数据库密码。将下载好的jar包复制到jdk的安装目录下的jre下的lib下的ext目录下。
一,主要分为5个步骤
1,获取驱动
首先下载自己mysql版本的jar包
下载地址:MySQL :: Download Connector/J
将下载好的jar包复制到jdk的安装目录下的jre下的lib下的ext目录下
mysql.5及以下的版本获取驱动是Class.forName("com.mysql.jdbc.Driver");
mysql.8及以上的版本获取驱动是Class.forName("com.mysql.cj.jdbc.Driver");
2,连接数据库
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/db0613?useUnicode=true&characterEncoding=utf8","root","123");
db0613是自己创建的数据库,root表示数据库用户名,123表示数据库密码
?useUnicode=true&characterEncoding=utf8 编码集
3,创建命令执行对象
Statement statement = connection.createStatement();
String sqlUpdate = "delete from student where stu_id = 1001";
String sqlQuery = "select * from student";
4,通过执行对象执行SQL语句
statement.executeUpdate(sqlUpdate); //增删改
ResultSet resultSet = statement.executeQuery(sqlQuery); //查询
5,释放资源
if(resultSet != null){
resultSet.close();
}
if(statement != null){
statement.close();
}
if(connection != null){
connection.close();
}
二,具体的代码实现
1,增删改
package com.jdbc.test;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
/**
* 增删改操作
*/
public class UpdateJdbc {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
//加载驱动
Class.forName("com.mysql.jdbc.Driver");
System.out.println("加载驱动成功");
//连接数据库
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/db0613?useUnicode=true&characterEncoding=utf8","root","123");
System.out.println("连接数据库成功");
//创建命令执行对象
Statement stmt = conn.createStatement();
String sql = "insert into student values(1007,'小春','1','1900-2-2','0','0','0')";
System.out.println("创建命令执行对象成功");
//执行对象执行SQL语句
stmt.executeUpdate(sql);
System.out.println("执行对象执行SQL语句成功");
//关闭资源
if (stmt != null) {
stmt.close();
stmt=null;
}
if (conn != null) {
conn.close();
conn=null;
}
System.out.println("关闭资源成功");
}
}
2,查询
package com.jdbc.test;
import com.jdbc.bean.Student;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
/**
* 查询操作
*/
public class QueryJdbc {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
//加载驱动
Class.forName("com.mysql.jdbc.Driver");
System.out.println("加载驱动成功");
//连接数据库
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/db0613?useUnicode=true&characterEncoding=utf8","root","123");
System.out.println("连接数据库成功");
//创建命令执行对象
Statement stmt = conn.createStatement();
String sql = "select * from student";
System.out.println("创建命令执行对象成功");
//执行对象执行SQL语句
//ResultSet只保存了一个地址
ResultSet rs = stmt.executeQuery(sql);
List<Student> list = new ArrayList<>();
while (rs.next()) {
int stu_id = rs.getInt("stu_id");
String stu_name = rs.getString("stu_name");
String stu_sex = rs.getString("stu_sex");
Date stu_both = rs.getDate("stu_both");
String stu_addr = rs.getString("stu_addr");
String stu_rec = rs.getString("stu_rec");
String stu_pwd = rs.getString("stu_pwd");
Student student = new Student(stu_id,stu_name,stu_sex,stu_both,stu_addr,stu_rec,stu_pwd);
list.add(student);
}
System.out.println(list.size());
System.out.println("执行对象执行SQL语句成功");
//关闭资源
if (rs != null) {
rs.close();
rs = null;
}
if (stmt != null) {
stmt.close();
stmt = null;
}
if (conn != null) {
conn.close();
conn=null;
}
System.out.println("关闭资源成功");
}
}
3,封装后的增删改查
package com.jdbc.dao;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
/**
*
*/
public class BaseDao<E> {
private static final String DRIVER = "com.mysql.jdbc.Driver";
private static final String URL = "jdbc:mysql://localhost:3306/db0613?useUnicode=true&characterEncoding=utf8";
private static final String USERNAME = "root";
private static final String PASSWORD = "123";
private Connection conn = null;
private Statement stmt = null;
protected ResultSet rs = null;
//加载驱动,只加载一次
static {
try {
Class.forName(DRIVER);
} catch (ClassNotFoundException e) {
throw new RuntimeException(e);
}
}
//连接数据库
public void getConn() {
try {
conn = DriverManager.getConnection(URL,USERNAME,PASSWORD);
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
//创建命令执行对象
public void getStmt() {
try {
stmt = conn.createStatement();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
//关闭资源
public void closeRes() {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
//增删改
public void update(String sql) throws Exception {
getConn();
getStmt();
stmt.executeUpdate(sql);
closeRes();
}
//查询
public List<E> query(String sql) throws SQLException {
getConn();
getStmt();
rs = stmt.executeQuery(sql);
List<E> list = new ArrayList<>();
rsList(list);
closeRes();
return list;
}
public void rsList(List<E> list) throws SQLException {
}
}
4,具体的实现
package com.jdbc.dao;
import com.jdbc.bean.Student;
import java.sql.Date;
import java.sql.SQLException;
import java.util.List;
/**
*
*/
public class StudentDao extends BaseDao<Student>{
public List<Student> findAll() throws SQLException {
String sql = "select * from student";
return super.query(sql);
}
public List<Student> findStudentById(String stu_id) throws SQLException {
String sql = "select * from student where stu_id = "+stu_id+"";
return super.query(sql);
}
public List<Student> addStudent(Student stu) throws SQLException {
String sql = "insert into student values('"+stu.getStu_id()+"')";
return super.query(sql);
}
@Override
public void rsList(List<Student> list) throws SQLException {
while (rs.next()) {
int stu_id = rs.getInt("stu_id");
String stu_name = rs.getString("stu_name");
String stu_sex = rs.getString("stu_sex");
Date stu_both = rs.getDate("stu_both");
String stu_addr = rs.getString("stu_addr");
String stu_rec = rs.getString("stu_rec");
String stu_pwd = rs.getString("stu_pwd");
Student student = new Student(stu_id,stu_name,stu_sex,stu_both,stu_addr,stu_rec,stu_pwd);
list.add(student);
}
}
}
5,测试类
package com.jdbc.test;
import com.jdbc.bean.Student;
import com.jdbc.dao.StudentDao;
import java.util.List;
/**
* 测试
*/
public class Test1 {
public static void main(String[] args) throws Exception {
String sql = "select * from student";
List<Student> list = new StudentDao().findAll();
for(Student lists : list){
System.out.print(lists.getStu_id()+"\t\t");
System.out.print(lists.getStu_name()+"\t\t");
System.out.print(lists.getStu_sex()+"\t\t");
System.out.print(lists.getStu_both()+"\t\t");
System.out.print(lists.getStu_addr()+"\t\t");
System.out.print(lists.getStu_rec()+"\t\t");
System.out.println(lists.getStu_pwd()+"\t\t");
}
}
}
6,测试结果
更多推荐
所有评论(0)