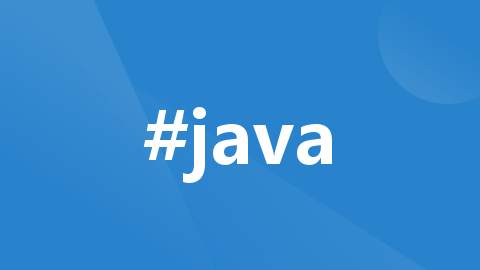
使用HttpClient进行HTTP请求的简易指南
引言: 在现代的网络应用程序中,进行HTTP请求是一项常见的任务。Apache HttpClient是一个功能强大且广泛使用的Java库,它提供了方便的方法来执行HTTP请求并处理响应。本文将介绍如何使用HttpClient库进行HTTP请求,包括GET请求、POST请求、添加参数和请求体、设置请求头等操作。HttpClient简介: HttpClient是一个开源的Java库,用于处理HTTP通

引言: 在现代的网络应用程序中,进行HTTP请求是一项常见的任务。Apache HttpClient是一个功能强大且广泛使用的Java库,它提供了方便的方法来执行HTTP请求并处理响应。本文将介绍如何使用HttpClient库进行HTTP请求,包括GET请求、POST请求、添加参数和请求体、设置请求头等操作。
HttpClient简介: HttpClient是一个开源的Java库,用于处理HTTP通信。它提供了各种类和方法,使得执行HTTP请求变得简单而直观。HttpClient不仅支持基本的HTTP协议功能,还提供了更高级的功能,如连接管理、cookie管理、代理设置等。
导入HttpClient库
在使用HttpClient之前,需要在项目中导入HttpClient库。可以通过在Maven项目的pom.xml文件中添加以下依赖项来实现:
xml
复制代码
<dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency>
发起GET请求
GET请求是获取服务器资源的一种常见方式。下面是使用HttpClient进行GET请求的示例代码:
ini
复制代码
HttpGet httpGet = new HttpGet("http://httpbin.org/get"); CloseableHttpResponse response = client.execute(httpGet); System.out.println(EntityUtils.toString(response.getEntity()));
在上述代码中,我们创建了一个HttpGet对象,并传入要请求的URL。然后使用HttpClient的execute
方法发送请求并获取响应。最后,通过EntityUtils
将响应内容转换为字符串并进行输出。
发起POST请求
POST请求用于向服务器提交数据。下面是使用HttpClient进行POST请求的示例代码:
ini
复制代码
HttpPost httpPost = new HttpPost("http://httpbin.org/post"); CloseableHttpResponse response = client.execute(httpPost); System.out.println(EntityUtils.toString(response.getEntity()));
与GET请求相比,POST请求的代码几乎相同。只需将HttpGet
替换为HttpPost
即可。
添加请求参数
有时候我们需要在请求中添加参数。使用HttpClient的URIBuilder
类可以方便地构建带有参数的URL。下面是一个示例:
ini
复制代码
URIBuilder builder = new URIBuilder("http://httpbin.org/post"); builder.addParameter("name", "Ru"); builder.addParameter("age", "18"); URI uri = builder.build(); HttpPost httpPost = new HttpPost(uri); CloseableHttpResponse response = client.execute(httpPost); System.out.println(EntityUtils.toString(response.getEntity()));
在上述代码中,我们创建了一个URIBuilder
对象,并使用addParameter
方法添加了两个参数。然后通过build
方法生成最终的URI,将其传递给HttpPost
对象进行请求。
设置请求体
有时候我们需要在POST请求中添加请求体,通常使用JSON格式进行数据传输。下面是一个示例:
ini
复制代码
HttpPost httpPost = new HttpPost("http://httpbin.org/post"); String jsonBody = "{"name": "Ru", "age": 18}"; // 设置请求头部信息 httpPost.setHeader("Content-Type", "application/json"); // 设置请求体 StringEntity requestEntity = new StringEntity(jsonBody, ContentType.APPLICATION_JSON); httpPost.setEntity(requestEntity); CloseableHttpResponse response = client.execute(httpPost); HttpEntity responseEntity = response.getEntity(); if (responseEntity != null) { String responseBody = EntityUtils.toString(responseEntity); System.out.println(responseBody); }
在上述代码中,我们首先设置了请求的Content-Type为application/json,然后创建了一个StringEntity对象来封装请求体数据。将其设置为HttpPost对象的实体,并执行请求。最后,通过EntityUtils将响应体转换为字符串并进行输出。
设置请求头
有时候我们需要在请求中添加自定义的请求头信息,如User-Agent、Authorization等。下面是一个示例:
vbscript
复制代码
HttpGet httpGet = new HttpGet("http://httpbin.org/get"); httpGet.setHeader("User-Agent", "MyHttpClient/1.0"); httpGet.setHeader("Authorization", "Bearer my_token"); CloseableHttpResponse response = client.execute(httpGet); System.out.println(EntityUtils.toString(response.getEntity()));
在上述代码中,我们使用setHeader
方法设置了两个自定义的请求头信息,然后执行GET请求并输出响应结果。
完整demo:
java
复制代码
package org.example.TestMaven; import org.apache.http.HttpEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.client.utils.URIBuilder; import org.apache.http.entity.ContentType; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; import java.io.IOException; import java.net.URI; import java.net.URISyntaxException; public class HttpClientDemo { static CloseableHttpClient client = HttpClients.createDefault(); public static void main(String[] args) throws IOException, URISyntaxException { // httpGetDemo(); // httpPostDemo(); // httpParamsDemo(); // httpBodyDemo(); httpHeaderDemo(); } public static void httpGetDemo() throws IOException { HttpGet httpGet = new HttpGet("http://httpbin.org/get"); CloseableHttpResponse response = client.execute(httpGet); System.out.println(EntityUtils.toString(response.getEntity())); } public static void httpPostDemo() throws IOException { HttpPost httpPost = new HttpPost("http://httpbin.org/post"); CloseableHttpResponse response = client.execute(httpPost); System.out.println(EntityUtils.toString(response.getEntity())); } public static void httpParamsDemo() throws IOException, URISyntaxException { URIBuilder builder = new URIBuilder("http://httpbin.org/post"); builder.addParameter("name", "Ru"); builder.addParameter("age", "18"); URI uri = builder.build(); HttpPost httpPost = new HttpPost(uri); CloseableHttpResponse response = client.execute(httpPost); System.out.println(EntityUtils.toString(response.getEntity())); } public static void httpBodyDemo() throws IOException { HttpPost httpPost = new HttpPost("http://httpbin.org/post"); String jsonBody = "{"name": "Ru", "age": 18}"; // 设置请求头部信息 httpPost.setHeader("Content-Type", "application/json"); // 设置请求体 StringEntity requestEntity = new StringEntity(jsonBody, ContentType.APPLICATION_JSON); httpPost.setEntity(requestEntity); CloseableHttpResponse response = client.execute(httpPost); HttpEntity responseEntity = response.getEntity(); if (responseEntity != null) { String responseBody = EntityUtils.toString(responseEntity); System.out.println(responseBody); } } public static void httpHeaderDemo() throws IOException { HttpGet httpGet = new HttpGet("http://httpbin.org/get"); httpGet.setHeader("User-Agent", "MyHttpClient/1.0"); httpGet.setHeader("Authorization", "Bearer my_token"); CloseableHttpResponse response = client.execute(httpGet); System.out.println(EntityUtils.toString(response.getEntity())); } }
结论: 本文介绍了如何使用HttpClient库进行HTTP请求的常见操作,包括GET请求、POST请求、添加请求参数和请求体、设置请求头等。通过使用HttpClient,我们可以轻松地与服务器进行通信并处理响应数据。希望本文对你理解和使用HttpClient有所帮助!
作者:MerkleJqueryRu
链接:https://juejin.cn/post/7234342980156915769
来源:稀土掘金
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
更多推荐
所有评论(0)