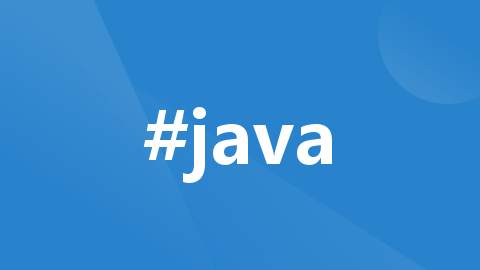
2023年第十四届蓝桥杯省赛Java C组题解
只做出来(ACDFGH),挑几个出来,答案不一定正确,但自己测试通过了。

只做出来(ACDFGH),挑几个出来,答案不一定正确,但自己测试通过了
A、求和
求1~20230408的和
public class Main{
public static void main(String[] args) {
System.out.println((long)20230409*10115204);
}
}
这里就直接套等差数列的求和公式,答案:204634714038436
D、平均
【问题描述】
有一个长度为n的数组(n是10的倍数),每个数 Ai 都是区间[0,9]中的整数,小明发现数组里每种数出现的次数不太平均,而更改第 i 个数的代价为bi,他想更改着若干个数的值使得这 10 种数出现的次数相等(都等于n/10,请问代价和最少为多少。
【输入格式】
输入的第一行包含一个正整数 n。
接下来n行,第i行包含两个整数ai,bi,用一个空格分隔。
【输出格式】
输出一行包含一个正整数表示答案。
【样例输入】
10
1 1
1 2
1 3
2 4
2 5
2 6
3 7
3 8
3 9
4 10
【样例输出】
27
【样例说明】
只更改第 1,2,4,5.7,8 个数,需要花费代价 1+2+4+5+7+8=27。
【评测用例规模与约定】
对于20%的评测用例,n<=1000;
对于所有评测用例n <= 100000,0<bi<=2×10^5。
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StreamTokenizer;
import java.util.*;
public class Main {
private static final StreamTokenizer st = new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
public static void main(String[] args) throws IOException {
int n = next();
List<Queue<Integer>> list = new ArrayList<>(10);
for (int i = 0; i < 10; i++) {
list.add(new PriorityQueue());
}
for (int i = 1; i <= n; i++) {
int a = next();
int b = next();
list.get(a).offer(b);
}
int count = 0;
n /= 10;
for (int i = 0; i < 10; i++) {
Queue<Integer> queue = list.get(i);
if (queue.size() <= n)
continue;
for (int j = 0; j <= queue.size() - n; j++) {
count += queue.poll();
}
}
System.out.println(count);
}
private static int next() throws IOException {
st.nextToken();
return (int) st.nval;
}
}
F、棋盘
【问题描述】
小蓝拥有 n × n 大小的棋盘,一开始棋盘上全都是白子。小蓝进行了m次操作,每次操作会将棋盘上某个范围内的所有棋子的颜色取反(也就是白色棋子变为黑色,黑色棋子变为白色)。请输出所有操作做完后棋盘上每个棋子的颜色。
【输入格式】
输入的第一行包含两个整数n,m,用一个空格分隔,表示棋盘大小与操作数。
接下来m行每行包含四个整数 X1,Y1,X2,Y2,相邻整数之间使用一个空格分隔,表示将在X1至X2行和Y1至Y2列中的棋子颜色取反。
【输出格式】
输出n行,每行n个0或1表示该位置棋子的颜色。如果是白色则输出0,否则输出1。
【样例输入】
3 3
1 1 2 2
2 2 3 3
1 1 3 3
【样例输出】
001
010
100
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StreamTokenizer;
public class Main {
private static final StreamTokenizer st = new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
public static void main(String[] args) throws IOException {
int n, m;
n = next();
m = next();
int[][] qp = new int[n + 1][n + 1];
for (int i = 1; i <= m; i++) {
int x1, y1, x2, y2;
x1 = next();
y1 = next();
x2 = next();
y2 = next();
for (int j = x1; j <= x2; j++) {
for (int k = y1; k <= y2; k++) {
int v = qp[j][k];
if (v == 0)
qp[j][k] = 1;
else
qp[j][k] = 0;
}
}
}
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
System.out.print(qp[i][j]);
}
System.out.println();
}
}
private static int next() throws IOException {
st.nextToken();
return (int) st.nval;
}
}
G、子矩阵
【问题描述】
给定一个n × m(n行m列)的矩阵。
设一个矩阵的价值为其所有数中的最大值和最小值的乘积。求给定矩阵的所有大小为a × b(a行b)的子矩阵的价值的和。
答案可能很大,你只需要输出答案对998244353 取模后的结果。
【输入格式】
输入的第一行包含四个整数分别表示 n,m,a,b,相邻整数之间使用一个空格分隔。
接下来n 行每行包含m个整数,相邻整数之间使用一个空格分隔,表示矩阵中的每个数Aij。
【输出格式】
输出一行包含一个整数表示答案。
【样例输入】
2 3 1 2
1 2 3
4 5 6
【样例输出】
58
【样例说明】
1×2 + 2×3 + 4×5 + 5×6 = 58。
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StreamTokenizer;
import java.math.BigInteger;
public class Main {
private static final StreamTokenizer st = new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
public static void main(String[] args) throws IOException {
int m, n, a, b;
m = next();
n = next();
a = next();
b = next();
int[][] nums = new int[m + 1][n + 1];
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
nums[i][j] = next();
}
}
BigInteger count = new BigInteger("0");
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
BigInteger temp = null;
if (i + a - 1 <= m && j + b - 1 <= n) {
temp = new BigInteger("1");
for (int k = i; k <= i + a - 1; k++) {
for (int l = j; l <= j + b - 1; l++) {
temp = temp.multiply(BigInteger.valueOf(nums[k][l]));
}
}
}
if (temp == null)
continue;
count = count.add(temp);
}
}
System.out.println(count.mod(new BigInteger("998244353")));
}
private static int next() throws IOException {
st.nextToken();
return (int) st.nval;
}
}
H、公因数匹配
【问题描述】
给定n个正整数Ai,请找出两个数 i,j 使得 i < j 且 Ai 和 Aj 存在大于1的公因数。
如果存在多组 i,j,请输出 i 最小的那组。如果仍然存在多组 i,j, 请输出最小的所有方案中 j最小的那组。
【输入格式】
输入的第一行包含一个整数 n。
第二行包含n个整数分别表示A1 A2 ......An,相邻整数之间使用一个空格分隔。
【输出格式】
输出一行包含两个整数分别表示题目要求的 i,j,用一个空格分隔。
【样例输入】
5
5 3 2 6 9
【样例输出】
2 4
【评测用例规模与约定】
对于40%的评测用例,n≤5000;
对于所有评测用例,1<=n<=10^5,1<=Ai<=10^6;
由于Scanner的nextInt()底层用了正则表达式,会降低输入效率,这里我用StreamTokenizer
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StreamTokenizer;
import java.util.Comparator;
import java.util.PriorityQueue;
public class Main {
private static final StreamTokenizer st = new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
static class MyComparator implements Comparator<Node> {
@Override
public int compare(Node o1, Node o2) {
if (o1.i == o2.i)
return o1.j - o2.j;
return o1.i - o2.i;
}
}
public static void main(String[] args) throws IOException {
int n = next();
int[] nums = new int[n + 1];
for (int i = 1; i <= n; i++) {
nums[i] = next();
}
PriorityQueue<Node> queue = new PriorityQueue<>(new MyComparator());
for (int i = 1; i <= n - 1; i++) {
for (int j = i + 1; j <= n; j++) {
int gcd = getGCD(nums[i], nums[j]);
if (gcd == 1)
continue;
queue.offer(new Node(i, j));
}
}
Node node = queue.poll();
System.out.println(node.i + " " + node.j);
}
private static int getGCD(int m, int n) {
if (n > m) {
m = m ^ n;
n = m ^ n;
m = m ^ n;
}
if (n == 0)
return m;
else
return getGCD(n, m % n);
}
private static int next() throws IOException {
st.nextToken();
return (int) st.nval;
}
}
class Node {
//索引i和j
int i;
int j;
public Node(int i, int j) {
this.i = i;
this.j = j;
}
}
更多推荐
所有评论(0)