在PHP上使用Github Webhooks
In the first part of our series, we talked about the Github API and built a demo in the process. In this part we are going to explore the Webhooks API, and we will build a demo to showcase the API usa
In the first part of our series, we talked about the Github API and built a demo in the process. In this part we are going to explore the Webhooks API, and we will build a demo to showcase the API usage. Let’s get started.
在本系列的第一部分中,我们讨论了Github API,并在此过程中构建了一个演示。 在这一部分中,我们将探索Webhooks API,我们将构建一个演示以展示API的用法。 让我们开始吧。
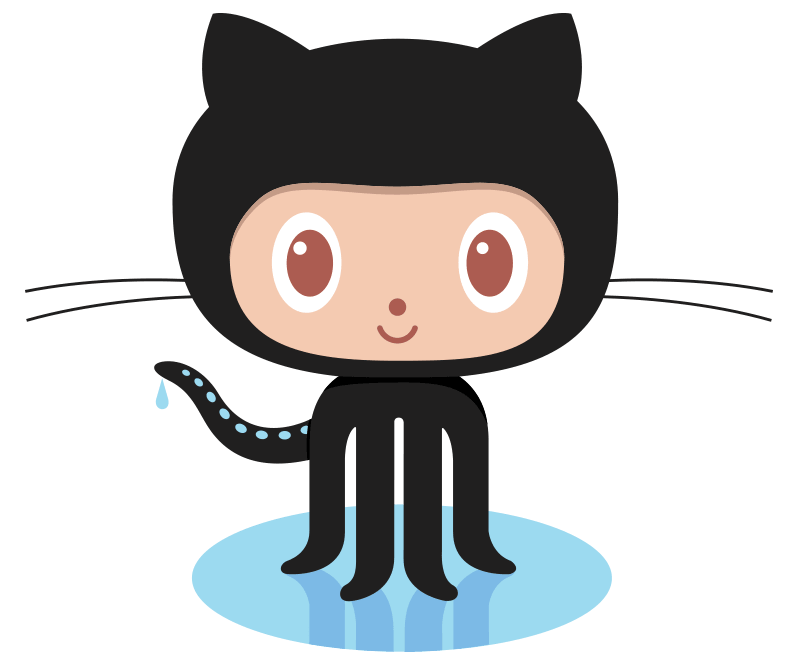
我们正在建设 (What We’re Building)
In this article we are going to set up a demo that receives every push from Github and saves it to a database. We are also going to create a page to display the list of our repository committers ordered by the number of commits. You can check the final result on Github.
在本文中,我们将建立一个演示,该演示会接收来自Github的所有推送并将其保存到数据库中。 我们还将创建一个页面,以显示按提交数量排序的存储库提交者列表。 您可以在Github上查看最终结果。
搭建环境 (Setting Up the Environment)
We will be using Laravel 5 as our framework, and because our application must be accessible from the internet to work with Github Webhooks, we can use Heroku to host our code. You can check this post to get started with Heroku.
我们将使用Laravel 5作为我们的框架,并且由于必须可以从Internet访问我们的应用程序才能与Github Webhooks一起使用,因此我们可以使用Heroku托管代码。 您可以查看此帖子以开始使用Heroku。
组态 (Configuration)
添加MySQL (Adding Mysql)
After creating our app on Heroku, we need to add MySQL to store the received data.
在Heroku上创建我们的应用程序后,我们需要添加MySQL来存储接收到的数据。
heroku addons:add cleardb
You can go to your dashboard to get your database connection credentials. Set the necessary variables inside your config as you can see in the example below.
您可以转到仪表板以获取数据库连接凭据。 如下面的示例所示,在配置中设置必要的变量。
MYSQL_HOST=‘HOST’
MYSQL_DATABASE=‘DB’
MYSQL_USERNAME=‘USERNAME’
MYSQL_PASSWORD=‘PASSWORD’
注册Github挂钩 (Register Github Hooks)
To register a new hook, navigate to a repository inside your account, then go to the settings tab and select Webhooks & Services
.
要注册新的挂钩,请导航至您帐户内的存储库,然后转到“设置”标签并选择“ Webhooks & Services
。
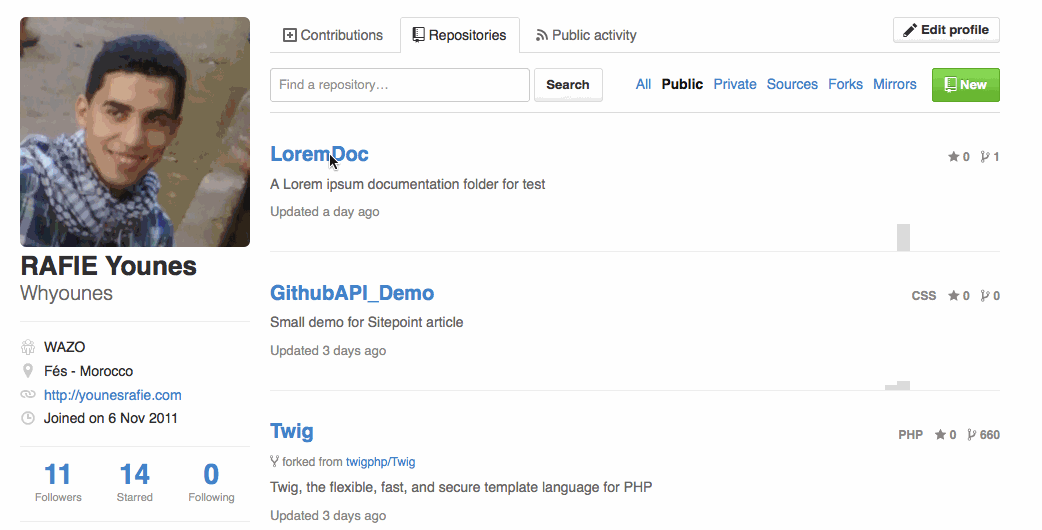
After setting your application URL endpoint to receive the payload, you can set a secret key to be sent along with the payload to verify the request origin. By default, Github will send you only push events, but you can select individual events from the list. For our demo, the push event is sufficient.
在将应用程序URL端点设置为接收有效负载之后,可以设置要与有效负载一起发送的密钥以验证请求的来源。 默认情况下,Github将仅向您发送推送事件,但是您可以从列表中选择单个事件。 对于我们的演示,push事件就足够了。
制作演示 (Building a Demo)
创建数据库表 (Creating The Database Tables)
php artisan make:migration create_hooks_table
// database/migrations/XXX_create_hooks_table.php
class CreateHooksTable extends Migration
{
public function up()
{
Schema::create('hooks', function (Blueprint $table) {
$table->increments('id');
$table->string('event_name', 100);
//you can separate the sender, repo...etc. but let's just keep it simple
$table->text('payload');
$table->timestamps();
});
}
public function down()
{
Schema::drop('hooks');
}
}
We only need a hooks
table to store the incoming requests. Registering the event_name
will help us in the future if we plan to track other events, and it will make it easier for us to look up the database. The payload field holds the request body. You can split this field into multiple parts and create a sender, a repository, a list of commits, etc.
我们只需要一个hooks
表来存储传入的请求。 如果我们计划跟踪其他事件,则注册event_name
将在将来对我们有帮助,这将使我们更轻松地查找数据库。 有效负载字段保存请求正文。 您可以将该字段分为多个部分,并创建一个发送方,一个存储库,一个提交列表等。
After running the migration using php artisan migrate
, we will create our Hook
model class.
使用php artisan migrate
运行php artisan migrate
,我们将创建Hook
模型类。
// app/Hook.php
class Hook extends Model
{
protected $table = ‘hooks’;
}
创建路线 (Creating Routes)
Our demo application will only have two routes.
我们的演示应用程序只有两条路线。
an
events
route, which will receive the Github event payload.events
路由,它将接收Github事件有效负载。a
/report/contributions
route, which will display a chart of project contributors./report/contributions
路线,它将显示项目贡献者的图表。
// app/Http/routes.php
Route::post(‘/events’, [‘uses’ => ‘GithubController@storeEvents’]);
// app/Http/Controllers/GithubController.php
public function storeEvents(Request $request) {
$event_name = $request->header(‘X-Github-Event’);
$body = json_encode(Input::all());
$hook = new Hook;
$hook->event_name = $event_name;
$hook->payload = $body;
$hook->save();
return ‘’;// 200 OK
}
The X-Github-Event
header value contains the event name that occurred. In this case, it will be equal to push
. If you want to test the request origin using the secret key you provided previously on Github, you can use the X-Hub-Signature
header value. You can read more in the documentation.
X-Github-Event
标头值包含发生的事件名称。 在这种情况下,它等于push
。 如果您想使用之前在Github上提供的密钥来测试请求的来源,则可以使用X-Hub-Signature
标头值。 您可以在文档中阅读更多内容 。
Github will send you an almost real time request. If you are using Heroku for deployment, you can use the logger to monitor requests while testing. You can read more about that in the documentation. You can also navigate to your Webhook configuration on Github and scroll to the Recent Deliveries
tab at the bottom.
Github将向您发送几乎实时的请求。 如果使用Heroku进行部署,则可以在测试过程中使用记录器监视请求。 您可以在文档中阅读有关此内容的更多信息。 您还可以导航到Github上的Webhook配置,然后滚动到底部的“ Recent Deliveries
选项卡。
heroku logs —tail -n 0 —ps router
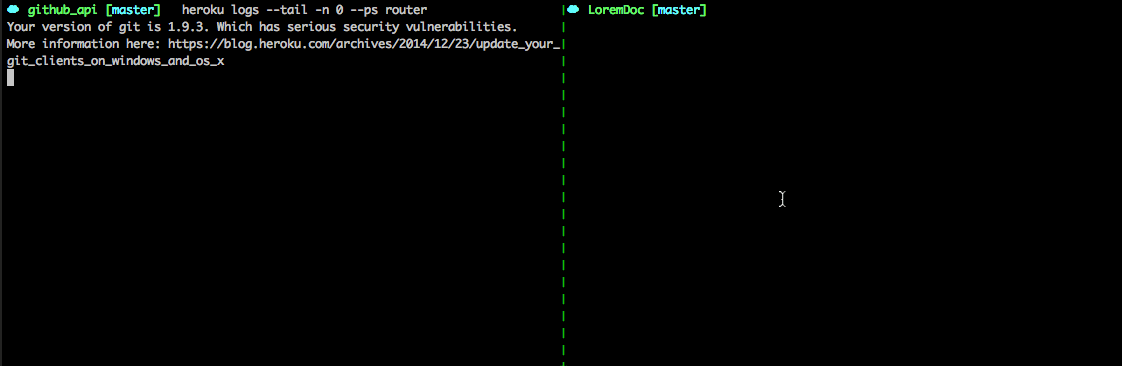
To display the list of contributors I’m going to use the ChartJs JavaScript library. You can check out this getting started guide for a good intro.
为了显示贡献者列表,我将使用ChartJs JavaScript库。 您可以查看此入门指南以获得良好的介绍。
// app/Http/routes.php
Route::get(‘/reports/contributions.json’, [‘uses’ => ‘GithubController@contributionsJson’]);
// app/Http/Controllers/GithubController.php
public function contributionsJson()
{
$hooks = Hook::where('event_name', '=', 'push')->get(['payload']);
$users = [];
$hooks->each(function ($item) use (&$users) {
$item = json_decode($item['payload']);
$pusherName = $item->pusher->name;
$commitsCount = count($item->commits);
$users[$pusherName] = array_pull($users, $pusherName, 0) + $commitsCount;
});
return [
'users' => array_keys($users),
'commits' => array_values($users)
];
}
The /reports/contributions.json
route will retrieve the list of push events from our database and loop through it to retrieve the list of users by commits. We then return the list of users and commits separately to suit the library needs. However, you can also choose to do it on the front-end using some Javascript.
/reports/contributions.json
路由将从我们的数据库中检索推送事件的列表,并循环遍历以通过提交检索用户列表。 然后,我们返回用户列表,并分别提交以满足库的需求。 但是,您也可以选择使用某些Javascript在前端进行操作。
// app/Http/routes.php
Route::get(‘/reports/contributions’, [‘uses’ => ‘GithubController@contributions’]);
// app//Http/Contrllers/GithubController.php
public function contributions()
{
return View::make('reports.contributions');
}
// resources/views/reports/contributions.blade.php
<canvas id=“contributions” width=“400” height=“400”></canvas>
<script>
var ctx = document.getElementById(“contributions”).getContext(“2d”);
$.ajax({
url: “/reports/contributions.json”,
dataType: “json”,
type: “GET”,
success: function(response){
var data = {
labels: response.users,
datasets: [
{
data: response.commits
}]
};
new Chart(ctx).Bar(data, {});
}
});
</script>
After getting the data from our JSON endpoint, we create a new Chart
object. You can read more about the available options in the documentation.
从JSON端点获取数据后,我们创建一个新的Chart
对象。 您可以在文档中阅读有关可用选项的更多信息。
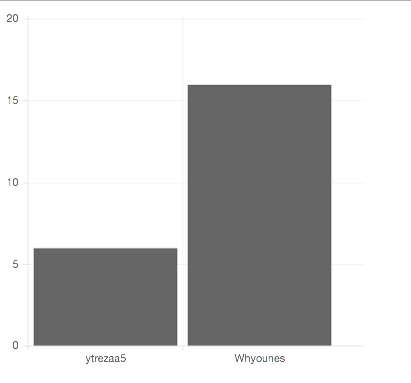
结论 (Conclusion)
Here’s a real world example – if you’ve ever contributed to a Symfony project on Github, you’ll have noticed that when you send a pull request, your code is being checked by an external service called fabbot.io. This service is watching the repository for every new pull request and does some checks on your code. You can visit the website for more info, and you can also register your own repository to be checked.
这是一个真实的例子–如果您曾经为Github上的Symfony项目做过贡献,您会注意到,当您发送拉取请求时,您的代码正在由名为fabbot.io的外部服务检查 。 该服务正在监视存储库中的每个新的请求请求,并对您的代码进行一些检查。 您可以访问该网站以获取更多信息,也可以注册自己的存储库以进行检查。
The Github API has a lot to offer, and the documentation makes it really easy to get started. I do recommend you check it out. Additionally, you can check the final result on Github. And like always, if you have any questions, don’t hesitate to post them below.
Github API提供了很多功能,而且文档非常容易上手。 我建议您检查一下。 此外,您可以在Github上查看最终结果。 而且像往常一样,如果您有任何疑问,请随时在下面发布。
更多推荐
所有评论(0)