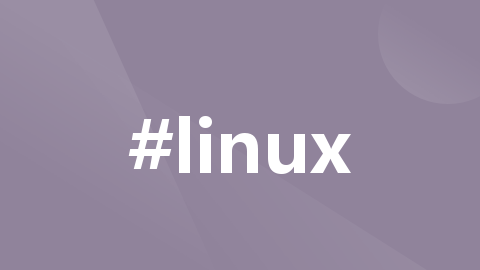
【C/C++】Linux C++ 获取当前时间
结构体中获取需要的时间信息,例如年、月、日、时、分、秒等。结构体中获取年、月、日、时、分、秒等信息,并输出当前时间。函数将 Unix 时间戳转换为本地时间。结构体,其中包含了年、月、日、时、分、秒等信息。函数获取当前时间的 Unix 时间戳,然后调用。函数获取当前时间的秒数,即 Unix 时间戳。将 Unix 时间戳转换为本地时间,可以使用。该函数将 Unix 时间戳转换为一个。在 Linux C
·
在 Linux C++ 中,可以使用 time
和 localtime
函数来获取当前时间。具体步骤如下:
-
调用
time
函数获取当前时间的秒数,即 Unix 时间戳。 -
将 Unix 时间戳转换为本地时间,可以使用
localtime
函数。该函数将 Unix 时间戳转换为一个tm
结构体,其中包含了年、月、日、时、分、秒等信息。 -
从
tm
结构体中获取需要的时间信息,例如年、月、日、时、分、秒等。
以下是一个获取当前时间的例子:
#include <iostream>
#include <ctime>
int main() {
// 获取当前时间的 Unix 时间戳
std::time_t now = std::time(nullptr);
// 将 Unix 时间戳转换为本地时间
std::tm* local_time = std::localtime(&now);
// 获取年、月、日、时、分、秒等信息
int year = local_time->tm_year + 1900;
int month = local_time->tm_mon + 1;
int day = local_time->tm_mday;
int hour = local_time->tm_hour;
int minute = local_time->tm_min;
int second = local_time->tm_sec;
// 输出当前时间
std::cout << "Current time: " << year << "-" << month << "-" << day << " "
<< hour << ":" << minute << ":" << second << std::endl;
return 0;
}
在上面的例子中,我们首先调用 time
函数获取当前时间的 Unix 时间戳,然后调用 localtime
函数将 Unix 时间戳转换为本地时间。最后,我们从 tm
结构体中获取年、月、日、时、分、秒等信息,并输出当前时间。
更多推荐
所有评论(0)