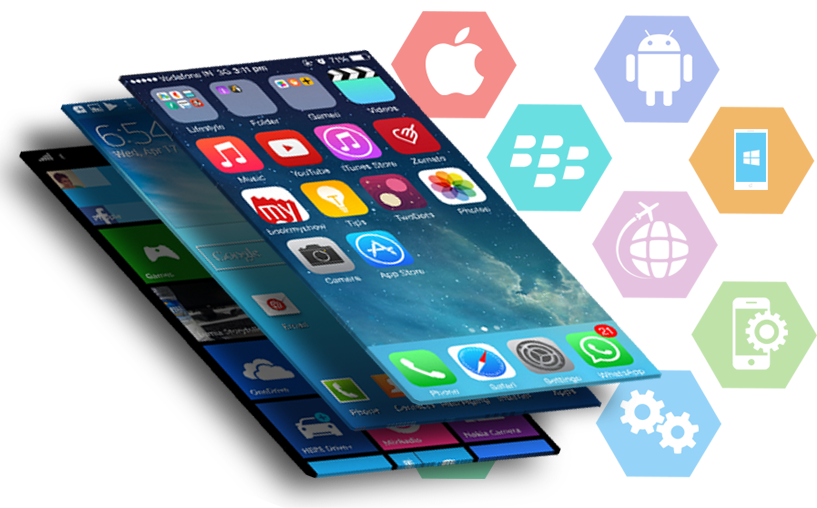
Vue实现excel导入
pc端有需要文件大批导入与导出可以使用,简单方便
·
excel导入功能需要使用npm包xlsx
,所以需要安装xlsx
插件
npm install xlsx@0.16.9 // 注意版本过高可能无法使用
UploadExcel文件,有基本样式,可修改
<template>
<div class="upload-excel">
<div class="btn-upload">
<el-button :loading="loading" size="mini" type="primary" @click="handleUpload">
点击上传
</el-button>
</div>
<input ref="excel-upload-input" class="excel-upload-input" type="file" accept=".xlsx, .xls" @change="handleClick">
<div class="drop" @drop="handleDrop" @dragover="handleDragover" @dragenter="handleDragover">
<i class="el-icon-upload" />
<span>将文件拖到此处</span>
</div>
</div>
</template>
<script>
// 导入
import XLSX from 'xlsx'
export default {
props: {
beforeUpload: Function, // eslint-disable-line
onSuccess: Function // eslint-disable-line
},
data() {
return {
loading: false,
excelData: {
header: null,
results: null
}
}
},
methods: {
generateData({ header, results }) {
this.excelData.header = header
this.excelData.results = results
this.onSuccess && this.onSuccess(this.excelData)
},
handleDrop(e) {
e.stopPropagation()
e.preventDefault()
if (this.loading) return
const files = e.dataTransfer.files
if (files.length !== 1) {
this.$message.error('Only support uploading one file!')
return
}
const rawFile = files[0] // only use files[0]
if (!this.isExcel(rawFile)) {
this.$message.error('Only supports upload .xlsx, .xls, .csv suffix files')
return false
}
this.upload(rawFile)
e.stopPropagation()
e.preventDefault()
},
handleDragover(e) {
e.stopPropagation()
e.preventDefault()
e.dataTransfer.dropEffect = 'copy'
},
handleUpload() {
this.$refs['excel-upload-input'].click()
},
handleClick(e) {
const files = e.target.files
const rawFile = files[0] // only use files[0]
if (!rawFile) return
this.upload(rawFile)
},
upload(rawFile) {
this.$refs['excel-upload-input'].value = null // fix can't select the same excel
if (!this.beforeUpload) {
this.readerData(rawFile)
return
}
const before = this.beforeUpload(rawFile)
if (before) {
this.readerData(rawFile)
}
},
readerData(rawFile) {
this.loading = true
return new Promise((resolve, reject) => {
const reader = new FileReader()
reader.onload = e => {
const data = e.target.result
const workbook = XLSX.read(data, { type: 'array' })
const firstSheetName = workbook.SheetNames[0]
const worksheet = workbook.Sheets[firstSheetName]
const header = this.getHeaderRow(worksheet)
const results = XLSX.utils.sheet_to_json(worksheet)
this.generateData({ header, results })
this.loading = false
resolve()
}
reader.readAsArrayBuffer(rawFile)
})
},
getHeaderRow(sheet) {
const headers = []
const range = XLSX.utils.decode_range(sheet['!ref'])
let C
const R = range.s.r
/* start in the first row */
for (C = range.s.c; C <= range.e.c; ++C) {
/* walk every column in the range */
const cell = sheet[XLSX.utils.encode_cell({ c: C, r: R })]
/* find the cell in the first row */
let hdr = 'UNKNOWN ' + C // <-- replace with your desired default
if (cell && cell.t) hdr = XLSX.utils.format_cell(cell)
headers.push(hdr)
}
return headers
},
isExcel(file) {
return /\.(xlsx|xls|csv)$/.test(file.name)
}
}
}
</script>
<style scoped lang="scss">
.upload-excel {
display: flex;
justify-content: center;
margin-top: 100px;
.excel-upload-input{
display: none;
z-index: -9999;
}
.btn-upload , .drop{
border: 1px dashed #bbb;
width: 350px;
height: 160px;
text-align: center;
line-height: 160px;
}
.drop{
line-height: 80px;
color: #bbb;
i {
font-size: 60px;
display: block;
}
}
}
</style>
注册全局的导入excel组件
import UploadExcel from './UploadExcel'
export default {
install(Vue) {
Vue.component('UploadExcel', UploadExcel) // 注册导入excel组件
}
}
创建import路由组件
<template>
<!-- 公共导入组件 -->
<upload-excel :on-success="success" />
</template>
配置路由
{
path: '/import',
component: Layout,
children: [{
path: '', // 二级路由path什么都不写 表示二级默认路由
component: () => import('@/views/import')
}]
},
获取导入的excel数据, 导入excel接口
<template>
<div>
<upload-excel :on-success="handleSuccess" />
</div>
</template>
<script>
import { importEmployee } from '@/api/employees'
export default {
name: 'Import',
// created() {
// this.handleSuccess()
// },
methods: {
formatDate(numb, format) {
const time = new Date((numb - 1) * 24 * 3600000 + 1)
time.setYear(time.getFullYear() - 70)
const year = time.getFullYear() + ''
const month = time.getMonth() + 1 + ''
const date = time.getDate() - 1 + ''
if (format && format.length === 1) {
return year + format + month + format + date
}
return year + (month < 10 ? '0' + month : month) + (date < 10 ? '0' + date : date)
},
// 格式化函数
// 1.中文字段转英文字段
// 2.还原exxel中的日期(转为英文格式)
formatData(header, results) {
const mapInfo = {
入职日期: 'timeOfEntry',
手机号: 'mobile',
姓名: 'username',
转正日期: 'correctionTime',
工号: 'workNumber',
部门: 'departmentName',
聘用形式: 'formOfEmployment'
}
// 数组转数组map
const data = results.map(obj => {
// obj是姓名:xxx 手机号:xxxx
const newObj = {}
// 对于所有的obj的属性来说
// Object.keys(obj)===>['姓名'手机号']
Object.keys(obj).forEach(zhkey => {
// 从中文的key转成英文的key
const enKey = mapInfo[zhkey]
// 判断key如果是日期的话
if (enKey === 'timeOfEntry' || enKey === 'correctionTime') {
// 对日期做特殊的处理,使用函数处理事件对象
// new Date('2020-12-12')做这个转换,是因为后端需要的标准日期格式
newObj[enKey] = new Date(this.formatDate(obj[zhkey], '/'))
} else {
// value不变
newObj[enKey] = obj[zhkey]
}
})
return newObj
})
return data
},
async handleSuccess({ header, results }) {
// 1. 格式转换
const data = this.formatData(header, results)
console.log('做格式转换之后', data)
// 2.发请求
const res = await importEmployee(data)
console.log(res)
this.$router.back()
// console.log('做格式转换之前', data)
// console.log(header, results)
// 后退
}
}
}
</script>
更多推荐
所有评论(0)