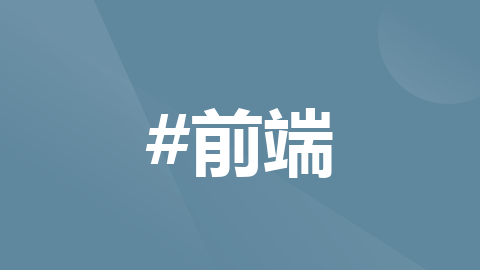
js、jq点击返回顶部(vue)、window.scrollTo
<div id="return" style="display: block;"><a href="" onclick="document.documentElement.scrollTop=document.body.scrollTop=0;return false;"&am
·
scrollTo平滑滚动
window.scrollTo() 滚动到文档中的某个坐标
第一种写法:
// 直接指定滚动到x轴和y轴位置
scrollTo(0, 0) //x,y
第二种写法:
/** scrollTo(options)
- left: 等同于 (x坐标)
options 有三个参数
- top: 等同于 (y坐标)
- behavior: 类型为string, 表示滚动行为, 支持参数 smooth(平滑滚动), instant(瞬间滚动), 默认值auto, 实测效果等同于instant
*/
// 示例:
window.scrollTo({
left: 0,
top: 100,
behavior: "smooth"
})
如何平滑滚动到页面顶部
const scrollToTop = () => {
const c = document.documentElement.scrollTop || document.body.scrollTop;
if (c > 0) {
window.requestAnimationFrame(scrollToTop);
window.scrollTo(0, c - c / 8);
}
}
// 事例
scrollToTop()
vue
vue实现平滑返回顶部,实时显示和隐藏返回顶部按钮,_vue 移动端网页去掉顶部标题 和返回左键-CSDN博客
方法二:到顶部使用elemenUI置顶组件(只能用于到顶部,没有到底部组件)
方法三:使用scrollTo属性来实现滚动效果(到达底部和到达顶部都能使用,且能设置滚动的效果,最简单的办法)
注意:scrollTo有两种语法:
(1):scrollTo(x,y) //使用最多的方法:指定滚动到x轴y轴的地方,直接到达,没有滚动效果
(2):scrollTo(options) //options是个对象,有三个属性,left、top、behavior
left:等同于x轴
top:等同于y轴
behavior:类型String,表示滚动行为,支持参数 smooth(平滑滚动),instant(瞬间滚动),默认值auto,实测效果等同于instant
<template>
<div class="container">
<div class="go-top" @click="backTop">
<img :src="imgGoTopSrc" />
<span>回顶部</span>
</div>
<div class="go-bottom" @click="goBottom">
<img :src="imgGoTopSrc" />
<span>到顶部</span>
</div>
</div>
</template>
<script>
export default {
data() {
return {
scrollTop: 0,
imgGoTopSrc: require('@/assets/img/goTop.png'),
}
},
mounted() {
let that = this
window.addEventListener('scroll', that.scrollToTop, true)
},
destroyed: function () {
window.removeEventListener('scroll', this.scrollToTop)
},
methods: {
//返回顶部
backTop() {
this.$nextTick(function() {
document.querySelector('.container').scrollTo({
top: 0,
behavior: "smooth"
});
})
},
//到底部
backTop() {
this.$nextTick(function() {
document.querySelector('.container').scrollTo({
top: document.querySelector('.container').scrollHeight,
behavior: "smooth"
});
})
},
// 为了计算距离顶部的高度,当高度大于60显示回顶部图标,小于60则隐藏
scrollToTop() {
const that = this
let scrollTop =
window.pageYOffset ||
document.documentElement.scrollTop ||
document.body.scrollTop
that.scrollTop = scrollTop
},
},
}
</script>
<style scoped lang="less">
.go-top,.go-bottom {
background: #fff;
position: fixed;
z-index: 99;
right: 13px;
bottom: 50px;
text-align: center;
padding: 10px 0;
margin-bottom: 50px;
cursor: pointer;
box-shadow: 0 0 6px rgb(0 0 0 / 12%);
img {
width: 20px;
height: 20px;
}
span {
font-size: 12px;
}
.go-top{
bottom:100px;
}
}
</style>
方法一:到顶部和到底部都使用定时器实现滚动的效果
<template>
<div class="container">
<div class="go-top" v-if="btnFlag" @click="backTop">
<img :src="imgGoTopSrc" />
<br />
<span>回顶部</span>
</div>
</div>
</template>
<script>
export default {
data() {
return {
btnFlag: false,
//记录屏幕滑动的值
scrollTop: 0,
imgGoTopSrc: require('@/assets/img/goTop.png'),
}
},
mounted() {
let that = this
window.addEventListener('scroll', that.scrollToTop, true)
},
destroyed: function () {
window.removeEventListener('scroll', this.scrollToTop)
},
methods: {
//返回顶部
backTop() {
const that = this
//使用定时器设置滑动过渡效果
let timer = setInterval(() => {
let ispeed = Math.floor(-that.scrollTop / 5)
document.documentElement.scrollTop = document.body.scrollTop =
that.scrollTop + ispeed
if (that.scrollTop === 0) {
clearInterval(timer)
}
}, 16)
},
// 为了计算距离顶部的高度,当高度大于60显示回顶部图标,小于60则隐藏
scrollToTop() {
const that = this
let scrollTop =
window.pageYOffset ||
document.documentElement.scrollTop ||
document.body.scrollTop
that.scrollTop = scrollTop
if (that.scrollTop > 60) {
that.btnFlag = true
} else {
that.btnFlag = false
}
},
},
}
</script>
<style scoped lang="less">
.go-top {
background: #fff;
position: fixed;
z-index: 99;
right: 13px;
bottom: 50px;
text-align: center;
padding: 10px 0;
margin-bottom: 50px;
cursor: pointer;
box-shadow: 0 0 6px rgb(0 0 0 / 12%);
img {
width: 20px;
height: 20px;
}
span {
font-size: 12px;
}
}
</style>
jq:
<div><a href="javascript:;" class="gotop" style="display:none;"></a></div>
<style>
.gotop {
position: fixed;
right: 25px;
bottom: 25px;
display: block;
width: 50px;
height: 50px;
background: url(../images/backtop.png) no-repeat 0 0;
opacity: 0.5;
}
</style>
//返回顶部按钮
$(function(){
$(window).scroll(function(){
if($(window).scrollTop()>100){
$(".gotop").fadeIn(400);
}
else{
$(".gotop").fadeOut(400);
}
});
$(".gotop").click(function(event){
event.preventDefault();
$('html,body').animate({'scrollTop':0},500);
return false;
});
});
<body>
<div id="backtop">
<img src="Top.jpg" />
</div>
<script type="text/javascript">
var oDiv = document.getElementById('backtop');
var oImg = oDiv.getElementsByTagName('img')[0];
window.onscroll = function(){
if(scroll().top>100){
oDiv.style.display = 'block';
}else{
oDiv.style.display = 'none';
}
}
oImg.onclick = function(){
clearInterval(oImg.timer);
oImg.timer = setInterval(function(){
var iSpeed = (0-scroll().top)/10;
iSpeed = iSpeed>0? Math.ceil(iSpeed):Math.floor(iSpeed);
window.scrollTo(0,scroll().top+iSpeed);
if(scroll().top ==0){
clearInterval(oImg.timer);
}
},30)
}
function scroll(){
return{
'top':document.documentElement.scrollTop+document.body.scrollTop,
'left':document.documentElement.scrollLeft+document.body.scrollLeft
}
}
</script>
</body>
更多推荐
所有评论(0)