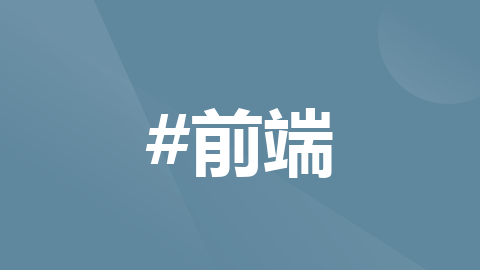
查天气(Vue.js,Element UI)
【代码】查天气(Vue.js,Element UI)
·
演示图
几点注意
- 有亿点简陋,凑合能用,button一定要 !important 覆盖原本的 element ui ,不然无效
- axios回调函数中 this 指向改变了,需要额外的保存一份
- 服务器返回的数据比较复杂时,获取的时候需要注意层级结构
- methods 中定义的方法内部,可以通过 this 关键字点出其他的方法
- 使用的是和风天气API,可以免费申请哦
完整代码
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>查天气</title>
<link rel="stylesheet" href="css/index.css" />
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
</head>
<body>
<div id="app">
<div class="logo"><img src="img/logo.png" alt="logo" /></div>
<div class="header">
<!-- 搜索框 -->
<div class="input_group">
<el-input class="input_border" v-model="city" placeholder="请输入查询的城市"
@keyup.enter.native="queryWeather"></el-input>
<el-button class="input_sub" @click="queryWeather">搜索</el-button>
</div>
<!-- 热门城市 -->
<el-row class="hotkey-container">
<el-tag class="hotkey" v-for="city in hotCitys" :key="city" @click="clickSearch(city)">{{ city
}}</el-tag>
</el-row>
</div>
<!-- 天气列表 -->
<ul class="weather_list">
<li v-if="weatherData" v-for="(day, index) in weatherData" :key="index">
<!-- 天气 -->
<div class="info_type">
<span class="iconfont">{{ day.textDay }}</span>
</div>
<!-- 温度 -->
<div class="info_temp">
<b>{{ day.tempMin }}</b> ~ <b>{{ day.tempMax }}</b> °C
</div>
<!-- 日期 -->
<div class="info_date">
<span>{{ day.fxDate }}</span>
</div>
<!-- 空气质量 -->
<div class="quality">
<p>空气质量指数: {{ airQualities[index].aqi }}</p>
<p>空气质量级别: {{ airQualities[index].category }}</p>
</div>
</li>
</ul>
</div>
<!-- 开发环境版本,包含了有帮助的命令行警告 -->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://unpkg.com/vue/dist/vue.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<script src="./查天气.js"></script>
</body>
</html>
css
/* 设置 Logo 图片的样式 */
.logo img {
display: block;
/* 将图片显示为块级元素 */
weight: 130px;
height: 130px;
margin: 30px auto;
/* border: #666 1px solid; */
}
/* 搜索框和热门城市 */
.header {
width: 600px;
margin: 0 auto;
}
/* 设置表单组的样式 */
.input_group {
display: flex;
}
/* 设置文本输入框的样式 */
.input_border {
width: 500px;
}
/* 设置提交按钮的样式 */
.input_sub {
width: 100px;
color: #fff !important;
background-color: #dca6e2 !important;
/* 使用 !important 来覆盖 Element UI 默认样式 */
}
/* 热门城市容器 */
.hotkey-container {
/* margin:0 auto; */
width: 310px;
}
/* 热门城市 */
.hotkey {
margin: 2px;
cursor: pointer;
color: #fff !important;
background-color: #e3b7df !important;
}
/* 热门城市,搜索按钮点击变暗 */
.hotkey:active,
.input_sub:active {
opacity: 0.8; /* 设置点击时的透明度 */
}
/* 设置天气列表的样式 */
.weather_list {
/* border: 1px solid #cc91d3; */
height: 200px;
text-align: center;
/* 文字居中 */
margin-top: 50px;
font-size: 0px;
/* 字体大小为0,避免列表项之间出现间隙 */
}
/* 设置天气列表中每个天气项的样式 */
.weather_list li {
display: inline-block;
/* 内联块元素 */
color: #E38EFF;
font-size: 33px;
line-height: 40px;
width: 180px;
height: 250px;
padding: 0 10px;
overflow: hidden;
position: relative;
/* 相对定位 */
background: url('../img/line.png') right center no-repeat;
background-size: 1px 200px;
/* border:1px solid #666; */
}
/* 最后一个天气列表项不显示背景 */
.weather_list li:last-child {
background: none;
}
/* 设置天气温度的样式 */
.info_temp {
font-size: 18px;
color: #f692ce;
/* border:1px solid #8e3a3a; */
}
/* 日期 */
.info_date {
width: 100%;
color: #999;
font-size: 16px;
/* border:1px solid #8e3a3a; */
}
/* 设置空气质量的样式 */
.quality {
position: absolute;
/* 绝对定位 */
left: 0;
/* 左边距为0 */
top: 122px;
line-height: 15px;
color: #666;
/* 文字颜色 */
font-size: 15px;
/* 文字大小 */
width: 100%;
/* 宽度100% */
text-align: center;
/* 文字居中对齐 */
/* border:1px solid #8e3a3a; */
}
js
new Vue({
el: "#app",
data: {
city: "北京",
weatherData: null,
airQualities: null, // 添加空气质量数据
hotCitys: ["北京", "上海", "广州", "深圳"],
apiKey: "xxxxxxx" // 直接将 API 密钥硬编码到 JavaScript 中
},
methods: {
// 查询天气
queryWeather() {
axios
.get(`https://geoapi.qweather.com/v2/city/lookup?key=${this.apiKey}&location=${encodeURIComponent(this.city)}`)
.then(res => {
const locationData = res.data.location[0];
const cityId = locationData.id;
// 获取天气数据
this.fetchWeatherData(cityId);
// 获取空气质量数据
this.fetchAirQuality(cityId);
})
.catch(err => {
console.log(err);
});
},
// 获取天气数据
fetchWeatherData(cityId) {
axios
.get(`https://devapi.qweather.com/v7/weather/3d?key=${this.apiKey}&location=${cityId}`)
.then(res => {
this.weatherData = res.data.daily;
})
.catch(err => {
console.log(err);
});
},
// 获取空气质量数据
fetchAirQuality(cityId) {
axios
.get(`https://devapi.qweather.com/v7/air/5d?key=${this.apiKey}&location=${cityId}`)
.then(res => {
this.airQualities = res.data.daily;
})
.catch(err => {
console.log(err);
});
},
// 点击搜索
clickSearch(city) {
this.city = city;
this.queryWeather();
}
}
});
更多推荐
所有评论(0)