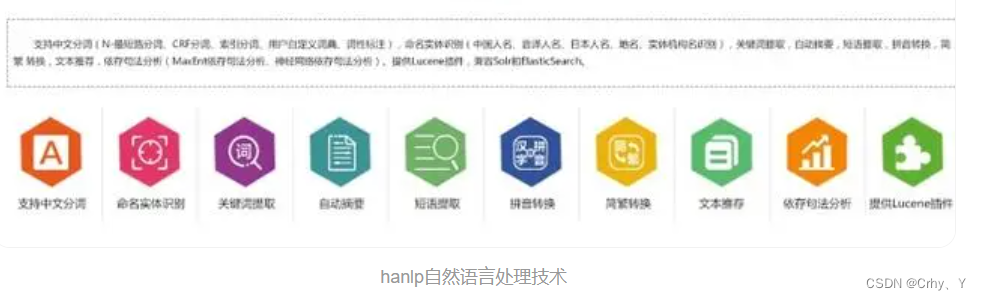
Hanlp自然语言处理如何再Spring Boot中使用
HanLP 可以在 Java、Python、Go 等多种编程语言中使用,也提供了各种语言的 API 接口,方便用户进行二次开发。启动SpringBoot应用,可以使用curl或其他工具,向http://localhost:8080/sentimentAnalysis发送POST请求,请求体为要进行情感分析的文本数据。可以通过调用不同的方法来实现这些功能,具体可参考HanLP官方文档(https:/

一、HanLP
HanLP (Hankcs' NLP) 是一个自然语言处理工具包,具有功能强大、性能高效、易于使用的特点。HanLP 主要支持中文文本处理,包括分词、词性标注、命名实体识别、依存句法分析、关键词提取、文本分类、情感分析等多种功能。 HanLP 可以在 Java、Python、Go 等多种编程语言中使用,也提供了各种语言的 API 接口,方便用户进行二次开发。HanLP 采用了深度学习和传统机器学习相结合的方法,具有较高的准确度和通用性。
二、java中用HanLP做情感分词场景
首先,下载HanLP jar包。可以从官方网站(https://github.com/hankcs/HanLP/releases)下载或者使用Maven配置。
<dependency>
<groupId>com.hankcs</groupId>
<artifactId>hanlp</artifactId>
<version>portable-1.7.8</version>
</dependency>
引入完成后,在代码中调用HanLP工具类的方法,例如:
import com.hankcs.hanlp.HanLP;
public class TestHanLP {
public static void main(String[] args) {
String text = "中华人民共和国成立了!";
System.out.println(HanLP.segment(text));
}
}
运行以上代码,可以得到分词结果:
[中华人民共和国, 成立, 了, !]
除了分词外,HanLP还提供了许多其他功能,例如实体识别、关键词提取、自动摘要等。可以通过调用不同的方法来实现这些功能,具体可参考HanLP官方文档(https://github.com/hankcs/HanLP)。
需要注意的是,HanLP默认使用的是繁体中文模型,如果需要使用简体中文模型,可以在代码中添加以下语句:
HanLP.Config.enableDebug();
HanLP.Config.Normalization = true;
这样就可以使用简体中文模型进行处理了。
三、SpringBoot中如何使用Hanlp进行文本情感分析
第一步:
在pom.xml文件中添加Hanlp的依赖
<dependency>
<groupId>com.hankcs</groupId>
<artifactId>hanlp</artifactId>
<version>portable-1.7.8</version>
</dependency>
第二步:
创建一个SpringBoot的Controller,用于接收文本数据,并进行情感分析
@RestController
public class SentimentAnalysisController {
@PostMapping("/sentimentAnalysis")
public String sentimentAnalysis(@RequestBody String text) {
String[] sentences = HanLP.extractSentence(text);
int positiveCount = 0;
int negativeCount = 0;
for (String sentence : sentences) {
List<String> keywords = HanLP.extractKeyword(sentence, 5);
for (String keyword : keywords) {
if (SentimentUtil.isPositive(keyword)) {
positiveCount++;
} else if (SentimentUtil.isNegative(keyword)) {
negativeCount++;
}
}
}
if (positiveCount > negativeCount) {
return "Positive";
} else if (positiveCount < negativeCount) {
return "Negative";
} else {
return "Neutral";
}
}
}
第三步:
上述代码中用到了SentimentUtil类,可以参考以下实现,用于判断一个词语的情感倾向
public class SentimentUtil {
private static final Set<String> POSITIVE_WORDS = new HashSet<>(Arrays.asList("好", "美", "乐", "棒", "赞", "爱", "优秀", "高兴", "满意", "友好", "感动"));
private static final Set<String> NEGATIVE_WORDS = new HashSet<>(Arrays.asList("坏", "丑", "难受", "差", "批评", "悲", "痛苦", "愤怒", "失望", "憎恶", "恐惧", "忧郁", "抱怨"));
public static boolean isPositive(String word) {
return POSITIVE_WORDS.contains(word);
}
public static boolean isNegative(String word) {
return NEGATIVE_WORDS.contains(word);
}
}
最后:
启动SpringBoot应用,可以使用curl或其他工具,向http://localhost:8080/sentimentAnalysis发送POST请求,请求体为要进行情感分析的文本数据。返回结果可以是Positive、Negative或Neutral。
注意:上述代码仅仅是示例代码,可以根据具体的需求进行修改和优化。在实际使用中,也需要根据具体情况对Hanlp的功能进行扩展和调整。
更多推荐
所有评论(0)