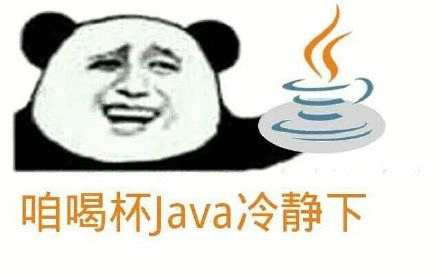
50个常用的Java代码示例
java常用的代码例程

50个常用的Java代码示例
1.输出语句:
System.out.println(Hello World!);
2.定义变量:
int x = 5;
String name = John;
3.循环语句:
for(int i = 0; i < 10; i++) {
System.out.println(i);
}
4.条件语句:
if(x > 0) {
System.out.println(x is positive);
} else if(x < 0) {
System.out.println(x is negative);
} else {
System.out.println(x is zero);
}
5.创建数组:
int[] arr = new int[5];
6.循环遍历数组:
for(int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
7.使用List集合:
List list = new ArrayList<>();
list.add(apple);
list.add(banana);
list.add(orange);
8.遍历List集合:
for(String fruit : list) {
System.out.println(fruit);
}
9.使用Map集合:
Map map = new HashMap<>();
map.put(apple, 1);
map.put(banana, 2);
map.put(orange, 3);
10.遍历Map集合:
for(String key : map.keySet()) {
System.out.println(key + : + map.get(key));
}
11.使用Scanner类读取用户输入:
Scanner scanner = new Scanner(System.in);
System.out.println(Enter your name:);
String name = scanner.nextLine();
System.out.println(Hello + name);
12.使用try-catch语句处理异常:
try {
// some code that might throw an exception
} catch(Exception e) {
System.out.println(An error occurred: + e.getMessage());
}
13.定义方法:
public static void sayHello() {
System.out.println(Hello);
}
14.调用方法:
sayHello();
15.方法传递参数:
public static void sayHelloTo(String name) {
System.out.println(Hello + name);
}
sayHelloTo(John);
16.定义类:
public class Person {
String name;
int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void sayHello() {
System.out.println(Hello, my name is + name + and I'm + age + years old.);
}
}
17.创建类的实例:
Person person = new Person(John, 30);
18.调用对象的方法:
person.sayHello();
19.继承类:
public class Student extends Person {
String major;
public Student(String name, int age, String major) {
super(name, age);
this.major = major;
}
public void sayHello() {
System.out.println(Hello, my name is + name + , I'm + age + years old, and I'm studying + major);
}
}
Student student = new Student(Jane, 20, Computer Science);
student.sayHello();
20.实现接口:
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
public void makeSound() {
System.out.println(Woof!);
}
}
Dog dog = new Dog();
dog.makeSound();
21.抛出异常:
public void doSomething() throws IOException {
// some code that might throw an IOException
}
try {
doSomething();
} catch(IOException e) {
System.out.println(An IO error occurred: + e.getMessage());
}
22.格式化字符串:
String name = John;
int age = 30;
String message = String.format(My name is %s and I'm %d years old, name, age);
System.out.println(message);
23.使用正则表达式:
String pattern = \\d+;
String text = 123abc456def789;
Pattern p = Pattern.compile(pattern);
Matcher m = p.matcher(text);
while(m.find()) {
System.out.println(m.group());
}
24.写入文件:
String content = Hello World!;
File file = new File(output.txt);
try {
FileWriter writer = new FileWriter(file);
writer.write(content);
writer.close();
} catch(IOException e) {
System.out.println(An IO error occurred: + e.getMessage());
}
25.读取文件:
File file = new File(input.txt);
try {
Scanner scanner = new Scanner(file);
while(scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
} catch(FileNotFoundException e) {
System.out.println(File not found: + e.getMessage());
}
26.使用日期和时间:
Date date = new Date();
DateFormat dateFormat = new SimpleDateFormat(yyyy/MM/dd HH:mm:ss);
String formattedDate = dateFormat.format(date);
System.out.println(formattedDate);
27.使用正则表达式替换字符串:
String text = Hello World!;
String pattern = l;
String replacement = -;
String replacedText = text.replaceAll(pattern, replacement);
System.out.println(replacedText);
28.使用Lambda表达式:
List list = Arrays.asList(1, 2, 3, 4, 5);
list.forEach(n -> System.out.println(n));
29.使用Stream API过滤数据:
List list = Arrays.asList(1, 2, 3, 4, 5);
list.stream()
.filter(n -> n % 2 == 0)
.forEach(n -> System.out.println(n));
30.使用Optional类避免空指针异常:
Optional optional = Optional.ofNullable(null);
String text = optional.orElse(default);
System.out.println(text);
31.使用Java集合框架之List:
List list = new ArrayList<>();
list.add(apple);
list.add(banana);
list.add(orange);
for(String item : list) {
System.out.println(item);
}
32.使用Java集合框架之Set:
Set set = new HashSet<>();
set.add(apple);
set.add(banana);
set.add(orange);
for(String item : set) {
System.out.println(item);
}
33.使用Java集合框架之Map:
Map map = new HashMap<>();
map.put(apple, 1);
map.put(banana, 2);
map.put(orange, 3);
for(Map.Entry entry : map.entrySet()) {
System.out.println(entry.getKey() + -> + entry.getValue());
}
34.使用Java集合框架之Queue:
Queue queue = new LinkedList<>();
queue.offer(apple);
queue.offer(banana);
queue.offer(orange);
while(!queue.isEmpty()) {
System.out.println(queue.poll());
}
35.使用Java集合框架之Stack:
Stack stack = new Stack<>();
stack.push(apple);
stack.push(banana);
stack.push(orange);
while(!stack.isEmpty()) {
System.out.println(stack.pop());
}
36.多线程编程:
class MyThread extends Thread {
public void run() {
System.out.println(Hello from thread + Thread.currentThread().getId());
}
}
MyThread thread = new MyThread();
thread.start();
37.使用Executor框架创建线程池:
ExecutorService executor = Executors.newFixedThreadPool(10);
for(int i = 0; i < 100; i++) {
executor.submit(() -> {
System.out.println(Hello from thread + Thread.currentThread().getId());
});
}
executor.shutdown();
38.使用ReentrantLock实现线程同步:
class Counter {
private int value = 0;
private ReentrantLock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
value++;
} finally {
lock.unlock();
}
}
public int getValue() {
return value;
}
}
Counter counter = new Counter();
executor.submit(() -> {
for(int i = 0; i < 100000; i++) {
counter.increment();
}
});
executor.submit(() -> {
for(int i = 0; i < 100000; i++) {
counter.increment();
}
});
executor.shutdown();
executor.awaitTermination(1, TimeUnit.MINUTES);
System.out.println(counter.getValue());
39.使用Semaphore实现资源共享:
Semaphore semaphore = new Semaphore(3);
for(int i = 0; i < 10; i++) {
executor.submit(() -> {
try {
semaphore.acquire();
System.out.println(Hello from thread + Thread.currentThread().getId());
Thread.sleep(1000);
semaphore.release();
} catch(InterruptedException e) {
e.printStackTrace();
}
});
}
executor.shutdown();
executor.awaitTermination(1, TimeUnit.MINUTES);
40.使用CountDownLatch实现线程同步:
CountDownLatch latch = new CountDownLatch(2);
executor.submit(() -> {
System.out.println(Task 1 is running);
Thread.sleep(1000);
latch.countDown();
});
executor.submit(() -> {
System.out.printlnTask 2 is running);
Thread.sleep(2000);
latch.countDown();
});
latch.await();
System.out.println(All tasks are completed);
41. 使用CyclicBarrier实现线程同步:
CyclicBarrier barrier = new CyclicBarrier(2, () -> {
System.out.println(Barrier action is running);
});
executor.submit(() -> {
System.out.println(Task 1 is running);
Thread.sleep(1000);
barrier.await();
});
executor.submit(() -> {
System.out.println(Task 2 is running);
Thread.sleep(2000);
barrier.await();
});
executor.shutdown();
executor.awaitTermination(1, TimeUnit.MINUTES);
42.使用Future和Callable实现异步编程:
class MyCallable implements Callable {
public String call() throws Exception {
Thread.sleep(1000);
return Hello from thread + Thread.currentThread().getId();
}
}
Future future = executor.submit(new MyCallable());
System.out.println(future.get());
43.使用Java8的Stream API进行集合操作:
List list = Arrays.asList(1, 2, 3, 4, 5);
int sum = list.stream().filter(x -> x % 2 == 0).mapToInt(x -> x).sum();
System.out.println(sum);
44.使用Java8的Optional类处理可能为空的对象:
Optional optional = Optional.ofNullable(null);
System.out.println(optional.orElse(default value));
45.使用Java8的Lambda表达式进行函数式编程:
interface MyFunction {
int apply(int x, int y);
}
MyFunction add = (x, y) -> x + y;
System.out.println(add.apply(1, 2));
46.使用Java8的方法引用简化Lambda表达式:
List list = Arrays.asList(apple, banana, orange);
list.forEach(System.out::println);
47.使用Java8的默认方法为接口提供默认实现:
interface MyInterface {
default void sayHello() {
System.out.println(Hello from MyInterface);
}
}
class MyClass implements MyInterface {
}
MyClass obj = new MyClass();
obj.sayHello();
48.使用Java8的接口中的静态方法:
interface MyInterface {
static void sayHello() {
System.out.println(Hello from MyInterface);
}
}
MyInterface.sayHello();
49.使用Java8的日期和时间API:
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(yyyy-MM-dd HH:mm:ss);
String formatted = now.format(formatter);
System.out.println(formatted);
50.使用Java8的Optional和Stream API组合进行复杂的操作:
class Person {
private String name;
private Optional
address;
public Person(String name, Optional
address) {
this.name = name;
this.address = address;
}
public String getName() {
return name;
}
public Optional
getAddress() {
return address;
}
}
class Address {
private String city;
public Address(String city) {
this.city = city;
}
public String getCity() {
return city;
}
}
List list = Arrays.asList(
new Person(Alice, Optional.of(new Address(New York))),
new Person(Bob, Optional.empty()),
new Person(Charlie,Optional.of(new Address(Los Angeles)))
);
String city = list.stream()
.filter(person -> person.getName().startsWith(B))
.flatMap(person -> person.getAddress().stream())
.map(Address::getCity)
.findFirst()
.orElse(Unknown);
System.out.println(city);
Optional和Stream API组合进行复杂的操作:
class Person {
private String name;
private Optional
address;
public Person(String name, Optional
address) {
this.name = name;
this.address = address;
}
public String getName() {
return name;
}
public Optional
getAddress() {
return address;
}
}
class Address {
private String city;
public Address(String city) {
this.city = city;
}
public String getCity() {
return city;
}
}
List list = Arrays.asList(
new Person(Alice, Optional.of(new Address(New York))),
new Person(Bob, Optional.empty()),
new Person(Charlie,Optional.of(new Address(Los Angeles)))
);
String city = list.stream()
.filter(person -> person.getName().startsWith(B))
.flatMap(person -> person.getAddress().stream())
.map(Address::getCity)
.findFirst()
.orElse(Unknown);
System.out.println(city);
以上就是50个常用的Java代码示例,希望对你有所帮助。
更多推荐
所有评论(0)