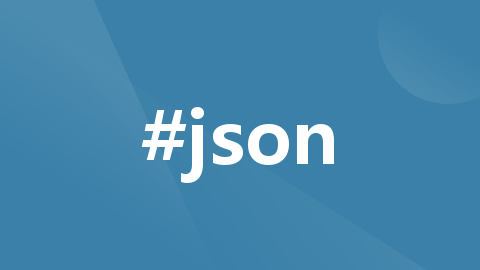
对象转json字符串时跳过某个属性
在本教程中,我将通过一个示例向您展示如何在使用 Jackson @JsonIgnore、和注释将对象序列化为 JSON 时忽略某些字段。这些注解用于忽略 JSON 序列化和反序列化中的逻辑属性。用于忽略序列化和反序列化中使用的逻辑属性。可用于 setter、getter 或字段。忽略 JSON 序列化和反序列化中的指定逻辑属性。它在类级别进行了注释。在类级别进行了注释,它忽略了整个类。当 Jack
·
在本教程中,我将通过一个示例向您展示如何在使用 Jackson @JsonIgnore、@JsonIgnoreProperties 和 @JsonIgnoreType注释将对象序列化为 JSON 时忽略某些字段。这些注解用于忽略 JSON 序列化和反序列化中的逻辑属性。
@JsonIgnore用于忽略序列化和反序列化中使用的逻辑属性。@JsonIgnore 可用于 setter、getter 或字段。
@JsonIgnoreProperties忽略 JSON 序列化和反序列化中的指定逻辑属性。它在类级别进行了注释。
@JsonIgnoreType在类级别进行了注释,它忽略了整个类。
当 Jackson 的默认值不够并且我们需要准确控制序列化为 JSON 的内容时,这非常有用。让我们通过示例来演示这些注解的用法。
相关依赖:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.8</version>
</dependency>
1.使用@JsonIgnoreProperties 在类级别忽略字段
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
@JsonIgnoreProperties(value = {
"id",
"firstName"
})
public class CustomerDTO {
private final String id;
private final String firstName;
private final String lastName;
public CustomerDTO(String id, String firstName, String lastName) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
}
public String getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
测试:
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class IgnoreFieldTest {
public static void main(String[] args) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
CustomerDTO dtoObject = new CustomerDTO("CUST100", "Tony", "Stark");
String dtoAsString = mapper.writeValueAsString(dtoObject);
System.out.println(dtoAsString);
}
}
//输出:{"lastName":"Stark"}
//请注意,我们忽略了CustomerDTO的两个字段“id”和“firstName” ,只打印了“lastName”。
2.使用@JsonIgnore 在字段级别忽略字段
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.annotation.JsonIgnore;
public class CustomerDTO {
@JsonIgnore
private final String id;
@JsonIgnore
private final String firstName;
private final String lastName;
public CustomerDTO(String id, String firstName, String lastName) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
}
public String getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
测试:
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class IgnoreFieldTest {
public static void main(String[] args) throws JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
CustomerDTO dtoObject = new CustomerDTO("CUST100", "Tony", "Stark");
String dtoAsString = mapper.writeValueAsString(dtoObject);
System.out.println(dtoAsString);
}
}
//输出:{"lastName":"Stark"}
//请注意,我们使用@JsonIgnore注释忽略了CustomerDTO的两个字段“id”和“firstName” ,
// 并且只打印了“lastName”。
3.使用@JsonIgnoreType 按类型忽略所有字段
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.annotation.JsonIgnoreType;
public class UserDTO {
public int id;
public Name name;
public UserDTO(int id, Name name) {
super();
this.id = id;
this.name = name;
}
@JsonIgnoreType
public static class Name {
public String firstName;
public String lastName;
public Name(String firstName, String lastName) {
super();
this.firstName = firstName;
this.lastName = lastName;
}
}
}
测试:
package net.javaguides.jackson.ignore;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonIgnoreTypeTest {
public static void main(String[] args) throws JsonProcessingException {
UserDTO.Name name = new UserDTO.Name("John", "Doe");
UserDTO user = new UserDTO(1, name);
String result = new ObjectMapper()
.writeValueAsString(user);
System.out.println(result);
}
}
//输出:{"id":1}
//请注意,使用@JsonIgnoreType注释会忽略 Name 类字段。
5.使用JSONObject怎么移除不需要转化为json的属性
import org.json.JSONObject;
public class RemovePropertyExample {
public static void main(String[] args) {
// 创建JSONObject对象
JSONObject jsonObject = new JSONObject();
// 添加需要转化为JSON的属性
jsonObject.put("name", "Alice");
jsonObject.put("age", 25);
jsonObject.put("gender", "female");
// 移除不需要转化为JSON的属性
jsonObject.remove("gender");
// 输出结果
System.out.println(jsonObject.toString());
}
}
//在上面的示例中,我们首先创建了一个JSONObject对象并添加了三个属性:name、
// age和gender。然后使用remove方法移除了gender属性。
// 最后,使用toString方法将JSONObject对象转换为字符串并打印输出。
//输出结果:{"name":"Alice","age":25}
更多推荐
所有评论(0)