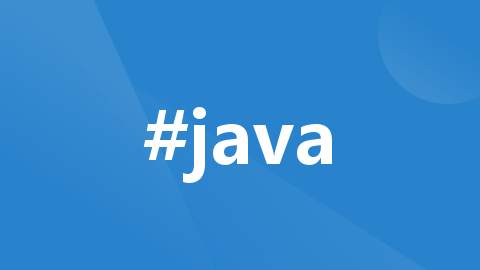
【springboot+vue项目(十)】springboot的增删改查
基于Spring Boot框架的上传日志表控制器,一些常见的CRUD(创建、读取、更新、删除)操作接口,UploadFileService继承自IService接口的一个服务接口。该控制器依赖于服务对象,通过Autowired注解进行注入。
·
一、 CRUD
基于Spring Boot框架的上传日志表控制器,一些常见的CRUD(创建、读取、更新、删除)操作接口,
UploadFileService
继承自IService
接口的一个服务接口。其中包括以下接口:
- GET请求:
/uploadlogs/listAll
,用于查询所有信息。- GET请求:
/uploadlogs/page/{pageNum}/{pageSize}
,用于分页查询。- GET请求:
/uploadlogs/{id}
,通过主键查询单条数据。- POST请求:
/uploadlogs
,用于新增数据。- PUT请求:
/uploadlogs
,用于修改数据。- DELETE请求:
/uploadlogs/{id}
,用于删除数据。- DELETE请求:
/uploadlogs/batch
,批量删除数据。该控制器依赖于
UploadFileService
服务对象,通过Autowired注解进行注入。
package com.example.demo.SystemCode.controller;
import com.example.demo.BaseCode.common.ResponseResult;
import com.example.demo.BaseCode.vo.PageVo;
import org.springframework.beans.factory.annotation.Autowired;
import com.example.demo.SystemCode.entity.UploadFile;
import com.example.demo.SystemCode.service.UploadFileService;
import org.springframework.web.bind.annotation.*;
import lombok.extern.slf4j.Slf4j;
import java.util.List;
/**
* 上传日志表(UploadFile)表控制层
*
* @author makejava
* @since 2023-07-18 10:42:48
*/
@RestController
@Slf4j
@CrossOrigin
@RequestMapping("/uploadlogs")
public class UploadFileController {
/**
* 服务对象
*/
@Autowired
private UploadFileService uploadFileService;
/**
* 查询所有信息
* @return
*/
@GetMapping("/listAll")
public ResponseResult listAllInfo(){
List<UploadFile> list = uploadFileService.list();
return ResponseResult.okResult(list);
}
/**
* 分页查询
* @param pageNum 查询页数
* @param pageSize 每页大小
* @return
*/
@GetMapping("/page/{pageNum}/{pageSize}")
public ResponseResult getPageInfo(@PathVariable Integer pageNum, @PathVariable Integer pageSize) {
PageVo pageVo = uploadFileService.selectUploadFilePage(pageNum, pageSize);
return ResponseResult.okResult(pageVo);
}
/**
* 通过主键查询单条数据
*
* @param id 主键
* @return 单条数据
*/
@GetMapping("{id}")
public ResponseResult selectOne(@PathVariable Long id) {
UploadFile uploadFile = uploadFileService.getById(id);
return ResponseResult.okResult(uploadFile);
}
/**
* 新增数据
*
* @param uploadFile 实体对象
* @return 新增结果
*/
@PostMapping
public ResponseResult insert(@RequestBody UploadFile uploadFile) {
uploadFileService.save(uploadFile);
return ResponseResult.okResult();
}
/**
* 修改数据
*
* @param uploadFile 实体对象
* @return 修改结果
*/
@PutMapping
public ResponseResult update(@RequestBody UploadFile uploadFile) {
uploadFileService.updateById(uploadFile);
return ResponseResult.okResult();
}
/**
* 删除数据
*
* @param id 主键结合
* @return 删除结果
*/
@DeleteMapping("/{id}")
public ResponseResult delete(@PathVariable("id") Long id) {
uploadFileService.removeById(id);
return ResponseResult.okResult();
}
/***
* 批量删除数据
* @param ids
* @return
*/
@DeleteMapping("/batch")
public ResponseResult deleteBatch(@RequestBody List<Long> ids) {
uploadFileService.removeBatchByIds(ids);
return ResponseResult.okResult();
}
}
二、 IService
基于MyBatis-Plus框架开发的通用Service接口。该接口提供了各种数据操作的方法,包括保存、更新、删除、查询等操作。它定义了一些默认的实现方法,也可以根据需要进行扩展和自定义。
接口中的方法包括:
save
:保存实体对象。saveBatch
:批量保存实体对象。saveOrUpdateBatch
:批量保存或更新实体对象。removeById
:根据主键ID删除实体对象。removeByMap
:根据条件删除实体对象。remove
:根据条件删除实体对象。removeByIds
:根据主键ID列表批量删除实体对象。updateById
:根据主键ID更新实体对象。update
:根据条件更新实体对象。updateBatchById
:根据主键ID列表批量更新实体对象。saveOrUpdate
:保存或更新实体对象。getById
:根据主键ID查询实体对象。listByIds
:根据主键ID列表查询实体对象列表。listByMap
:根据条件查询实体对象列表。getOne
:根据条件查询单个实体对象。getMap
:根据条件查询实体对象的字段映射。getObj
:根据条件查询实体对象,并通过函数转换为另一种对象。count
:查询满足条件的实体对象数量。list
:根据条件查询实体对象列表。page
:根据条件分页查询实体对象列表。listMaps
:根据条件查询实体对象的字段映射列表。listObjs
:根据条件查询实体对象的字段列表。pageMaps
:根据条件分页查询实体对象的字段映射列表。除了上述方法,还有一些链式调用的方法,如
query()
、lambdaQuery()
等,用于构建更复杂的查询条件。在实际使用中,可以根据具体业务需求选择合适的方法进行数据操作。该接口提供了一个
getBaseMapper()
方法,用于获取底层的Mapper组件,通过Mapper可以直接操作数据库进行数据存取。另外,接口还包含一些默认常量和泛型定义,用于指定实体类型和批量操作的默认批次大小。
总之,该接口提供了一种规范和便捷的方式来操作实体对象的增删改查等常见操作,简化了代码开发和维护的工作量。
package com.baomidou.mybatisplus.extension.service;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.baomidou.mybatisplus.core.toolkit.CollectionUtils;
import com.baomidou.mybatisplus.core.toolkit.Wrappers;
import com.baomidou.mybatisplus.extension.conditions.query.LambdaQueryChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.query.QueryChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.update.LambdaUpdateChainWrapper;
import com.baomidou.mybatisplus.extension.conditions.update.UpdateChainWrapper;
import com.baomidou.mybatisplus.extension.kotlin.KtQueryChainWrapper;
import com.baomidou.mybatisplus.extension.kotlin.KtUpdateChainWrapper;
import com.baomidou.mybatisplus.extension.toolkit.ChainWrappers;
import com.baomidou.mybatisplus.extension.toolkit.SqlHelper;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
import org.springframework.transaction.annotation.Transactional;
public interface IService<T> {
int DEFAULT_BATCH_SIZE = 1000;
// 插入一条记录
default boolean save(T entity) {
return SqlHelper.retBool(this.getBaseMapper().insert(entity));
}
@Transactional(
rollbackFor = {Exception.class}
)
// 批量插入记录
default boolean saveBatch(Collection<T> entityList) {
return this.saveBatch(entityList, 1000);
}
// 批量插入记录,可指定批大小
boolean saveBatch(Collection<T> entityList, int batchSize);
@Transactional(
rollbackFor = {Exception.class}
)
// 批量插入或更新记录
default boolean saveOrUpdateBatch(Collection<T> entityList) {
return this.saveOrUpdateBatch(entityList, 1000);
}
// 批量插入或更新记录,可指定批大小
boolean saveOrUpdateBatch(Collection<T> entityList, int batchSize);
// 根据ID删除记录
default boolean removeById(Serializable id) {
return SqlHelper.retBool(this.getBaseMapper().deleteById(id));
}
// 不支持的方法,根据ID删除记录并使用填充策略
default boolean removeById(Serializable id, boolean useFill) {
throw new UnsupportedOperationException("不支持的方法!");
}
// 根据实体对象删除记录
default boolean removeById(T entity) {
return SqlHelper.retBool(this.getBaseMapper().deleteById(entity));
}
// 根据列条件删除记录
default boolean removeByMap(Map<String, Object> columnMap) {
Assert.notEmpty(columnMap, "error: columnMap must not be empty", new Object[0]);
return SqlHelper.retBool(this.getBaseMapper().deleteByMap(columnMap));
}
// 根据条件包装器删除记录
default boolean remove(Wrapper<T> queryWrapper) {
return SqlHelper.retBool(this.getBaseMapper().delete(queryWrapper));
}
// 根据ID集合批量删除记录
default boolean removeByIds(Collection<?> list) {
return CollectionUtils.isEmpty(list) ? false : SqlHelper.retBool(this.getBaseMapper().deleteBatchIds(list));
}
@Transactional(
rollbackFor = {Exception.class}
)
// 根据ID集合批量删除记录并使用填充策略
default boolean removeByIds(Collection<?> list, boolean useFill) {
if (CollectionUtils.isEmpty(list)) {
return false;
} else {
return useFill ? this.removeBatchByIds(list, true) : SqlHelper.retBool(this.getBaseMapper().deleteBatchIds(list));
}
}
@Transactional(
rollbackFor = {Exception.class}
)
// 批量删除记录
default boolean removeBatchByIds(Collection<?> list) {
return this.removeBatchByIds(list, 1000);
}
@Transactional(
rollbackFor = {Exception.class}
)
// 批量删除记录,并使用填充策略
default boolean removeBatchByIds(Collection<?> list, boolean useFill) {
return this.removeBatchByIds(list, 1000, useFill);
}
// 不支持的方法,根据ID集合批量删除记录,并指定批大小
default boolean removeBatchByIds(Collection<?> list, int batchSize) {
throw new UnsupportedOperationException("不支持的方法!");
}
// 不支持的方法,根据ID集合批量删除记录,并指定批大小和填充策略
default boolean removeBatchByIds(Collection<?> list, int batchSize, boolean useFill) {
throw new UnsupportedOperationException("不支持的方法!");
}
// 根据ID更新记录
default boolean updateById(T entity) {
return SqlHelper.retBool(this.getBaseMapper().updateById(entity));
}
// 根据条件包装器更新记录
default boolean update(Wrapper<T> updateWrapper) {
return this.update((Object)null, updateWrapper);
}
// 根据实体对象和条件包装器更新记录
default boolean update(T entity, Wrapper<T> updateWrapper) {
return SqlHelper.retBool(this.getBaseMapper().update(entity, updateWrapper));
}
@Transactional(
rollbackFor = {Exception.class}
)
// 根据ID集合批量更新记录
default boolean updateBatchById(Collection<T> entityList) {
return this.updateBatchById(entityList, 1000);
}
// 根据ID集合批量更新记录,并指定批大小
boolean updateBatchById(Collection<T> entityList, int batchSize);
// 保存或更新记录
boolean saveOrUpdate(T entity);
// 根据ID查询记录
default T getById(Serializable id) {
return this.getBaseMapper().selectById(id);
}
// 根据ID集合查询记录
default List<T> listByIds(Collection<? extends Serializable> idList) {
return this.getBaseMapper().selectBatchIds(idList);
}
// 根据列条件查询记录
default List<T> listByMap(Map<String, Object> columnMap) {
return this.getBaseMapper().selectByMap(columnMap);
}
// 根据查询条件返回单个实体对象
default T getOne(Wrapper<T> queryWrapper) {
return this.getOne(queryWrapper, true);
}
T getOne(Wrapper<T> queryWrapper, boolean throwEx);
// 根据查询条件返回一个Map对象
Map<String, Object> getMap(Wrapper<T> queryWrapper);
// 根据查询条件返回自定义对象
<V> V getObj(Wrapper<T> queryWrapper, Function<? super Object, V> mapper);
// 返回记录总数
default long count() {
return this.count(Wrappers.emptyWrapper());
}
long count(Wrapper<T> queryWrapper);
// 根据查询条件返回实体对象列表
default List<T> list(Wrapper<T> queryWrapper) {
return this.getBaseMapper().selectList(queryWrapper);
}
default List<T> list() {
return this.list(Wrappers.emptyWrapper());
}
// 根据查询条件返回实体对象的分页结果
default <E extends IPage<T>> E page(E page, Wrapper<T> queryWrapper) {
return this.getBaseMapper().selectPage(page, queryWrapper);
}
default <E extends IPage<T>> E page(E page) {
return this.page(page, Wrappers.emptyWrapper());
}
// 根据查询条件返回Map对象的列表
default List<Map<String, Object>> listMaps(Wrapper<T> queryWrapper) {
return this.getBaseMapper().selectMaps(queryWrapper);
}
default List<Map<String, Object>> listMaps() {
return this.listMaps(Wrappers.emptyWrapper());
}
// 根据查询条件返回自定义对象的列表
default List<Object> listObjs() {
return this.listObjs(Function.identity());
}
default <V> List<V> listObjs(Function<? super Object, V> mapper) {
return this.listObjs(Wrappers.emptyWrapper(), mapper);
}
default List<Object> listObjs(Wrapper<T> queryWrapper) {
return this.listObjs(queryWrapper, Function.identity());
}
default <V> List<V> listObjs(Wrapper<T> queryWrapper, Function<? super Object, V> mapper) {
return (List) this.getBaseMapper().selectObjs(queryWrapper)
.stream()
.filter(Objects::nonNull)
.map(mapper)
.collect(Collectors.toList());
}
// 根据查询条件返回Map对象的分页结果
default <E extends IPage<Map<String, Object>>> E pageMaps(E page, Wrapper<T> queryWrapper) {
return this.getBaseMapper().selectMapsPage(page, queryWrapper);
}
default <E extends IPage<Map<String, Object>>> E pageMaps(E page) {
return this.pageMaps(page, Wrappers.emptyWrapper());
}
BaseMapper<T> getBaseMapper();
Class<T> getEntityClass();
// 创建QueryChainWrapper对象,用于构建查询条件链式调用
default QueryChainWrapper<T> query() {
return ChainWrappers.queryChain(this.getBaseMapper());
}
// 创建LambdaQueryChainWrapper对象,用于使用Lambda表达式构建查询条件链式调用
default LambdaQueryChainWrapper<T> lambdaQuery() {
return ChainWrappers.lambdaQueryChain(this.getBaseMapper());
}
// 创建KtQueryChainWrapper对象,用于在Kotlin项目中构建查询条件链式调用
default KtQueryChainWrapper<T> ktQuery() {
return ChainWrappers.ktQueryChain(this.getBaseMapper(), this.getEntityClass());
}
// 创建KtUpdateChainWrapper对象,用于在Kotlin项目中构建更新条件链式调用
default KtUpdateChainWrapper<T> ktUpdate() {
return ChainWrappers.ktUpdateChain(this.getBaseMapper(), this.getEntityClass());
}
// 创建UpdateChainWrapper对象,用于构建更新条件链式调用
default UpdateChainWrapper<T> update() {
return ChainWrappers.updateChain(this.getBaseMapper());
}
// 创建LambdaUpdateChainWrapper对象,用于使用Lambda表达式构建更新条件链式调用
default LambdaUpdateChainWrapper<T> lambdaUpdate() {
return ChainWrappers.lambdaUpdateChain(this.getBaseMapper());
}
// 保存或更新实体对象
default boolean saveOrUpdate(T entity, Wrapper<T> updateWrapper) {
return this.update(entity, updateWrapper) || this.saveOrUpdate(entity);
}
}
更多推荐
所有评论(0)