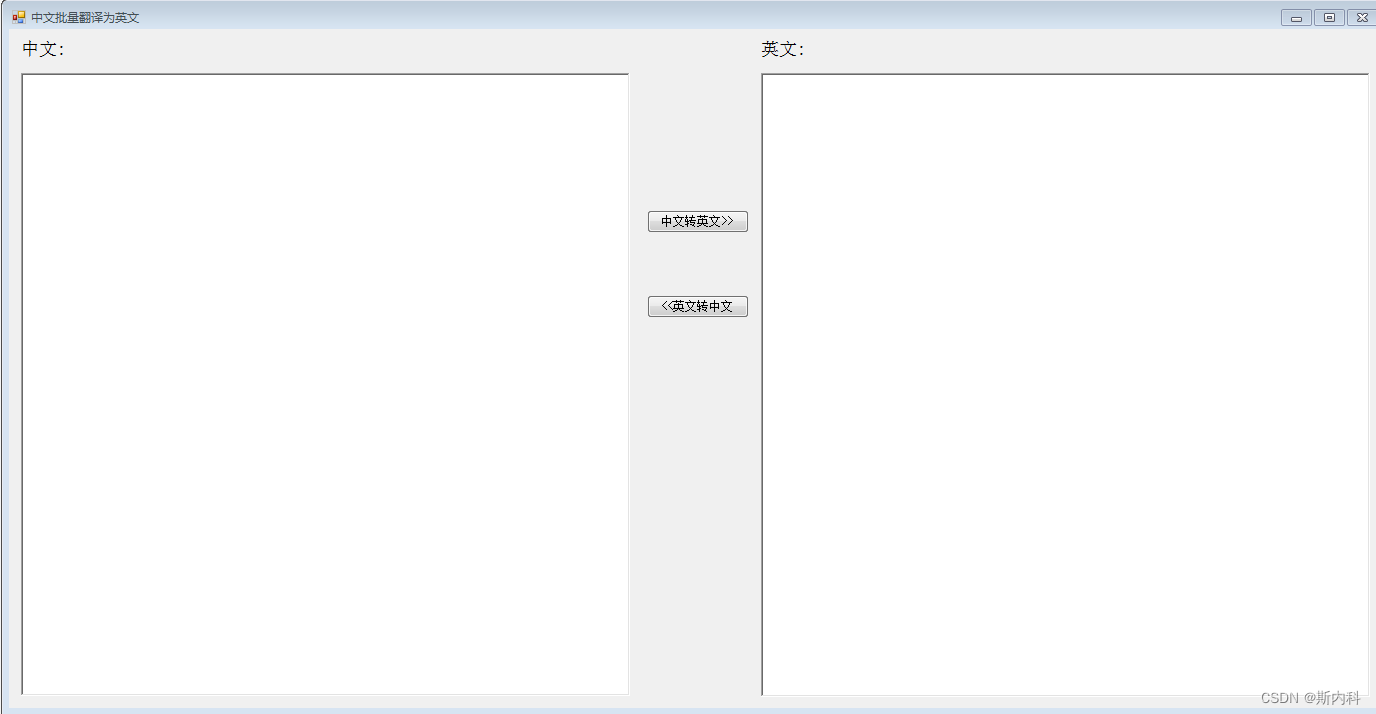
C#调用百度翻译API自动将中文转化为英文,按行转换
【代码】C#调用百度翻译API自动将中文转化为英文,按行转换。
·
我们可以使用百度翻译API获取到翻译结果
翻译API地址:
http://api.fanyi.baidu.com/api/trans/vip/translate
一、新建窗体应用程序TranslatorDemo,将默认的Form1重命名为FormTranslator。
窗体FormTranslator设计器如图:
窗体设计器源代码如下:
文件:FormTranslator.Designer.cs
namespace TranslatorDemo
{
partial class FormTranslator
{
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
/// <param name="disposing">如果应释放托管资源,为 true;否则为 false。</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要修改
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.bntToEnglish = new System.Windows.Forms.Button();
this.label1 = new System.Windows.Forms.Label();
this.rtxtChinese = new System.Windows.Forms.RichTextBox();
this.label2 = new System.Windows.Forms.Label();
this.rtxtEnglish = new System.Windows.Forms.RichTextBox();
this.btnToChinese = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// bntToEnglish
//
this.bntToEnglish.Location = new System.Drawing.Point(638, 181);
this.bntToEnglish.Name = "bntToEnglish";
this.bntToEnglish.Size = new System.Drawing.Size(102, 23);
this.bntToEnglish.TabIndex = 2;
this.bntToEnglish.Text = "中文转英文>>";
this.bntToEnglish.UseVisualStyleBackColor = true;
this.bntToEnglish.Click += new System.EventHandler(this.bntToEnglish_Click);
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("宋体", 13F);
this.label1.Location = new System.Drawing.Point(9, 11);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(53, 18);
this.label1.TabIndex = 1;
this.label1.Text = "中文:";
//
// rtxtChinese
//
this.rtxtChinese.Location = new System.Drawing.Point(12, 44);
this.rtxtChinese.Name = "rtxtChinese";
this.rtxtChinese.Size = new System.Drawing.Size(609, 623);
this.rtxtChinese.TabIndex = 0;
this.rtxtChinese.Text = "";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Font = new System.Drawing.Font("宋体", 13F);
this.label2.Location = new System.Drawing.Point(749, 11);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(53, 18);
this.label2.TabIndex = 3;
this.label2.Text = "英文:";
//
// rtxtEnglish
//
this.rtxtEnglish.Location = new System.Drawing.Point(752, 44);
this.rtxtEnglish.Name = "rtxtEnglish";
this.rtxtEnglish.Size = new System.Drawing.Size(609, 624);
this.rtxtEnglish.TabIndex = 1;
this.rtxtEnglish.Text = "";
//
// btnToChinese
//
this.btnToChinese.Location = new System.Drawing.Point(638, 266);
this.btnToChinese.Name = "btnToChinese";
this.btnToChinese.Size = new System.Drawing.Size(102, 23);
this.btnToChinese.TabIndex = 3;
this.btnToChinese.Text = "<<英文转中文";
this.btnToChinese.UseVisualStyleBackColor = true;
this.btnToChinese.Click += new System.EventHandler(this.btnToChinese_Click);
//
// FormTranslator
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(1371, 679);
this.Controls.Add(this.btnToChinese);
this.Controls.Add(this.rtxtEnglish);
this.Controls.Add(this.label2);
this.Controls.Add(this.rtxtChinese);
this.Controls.Add(this.label1);
this.Controls.Add(this.bntToEnglish);
this.Name = "FormTranslator";
this.Text = "中文批量翻译为英文";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Button bntToEnglish;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.RichTextBox rtxtChinese;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.RichTextBox rtxtEnglish;
private System.Windows.Forms.Button btnToChinese;
}
}
二、新建关键翻译类TranslateUtil
TranslateUtil.cs源程序如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using System.Web.Script.Serialization;
using System.Web.Security;
namespace TranslatorDemo
{
/// <summary>
/// 翻译过程
/// </summary>
public class TranslateUtil
{
/// <summary>
/// 使用Get的方式调用百度API进行翻译
/// </summary>
/// <param name="content">要转化的文本</param>
/// <param name="languageFrom">要转化的语言</param>
/// <param name="languageTo">目标语言</param>
/// <returns></returns>
public static FeedbackResult TranslateGet(string content, string languageFrom, string languageTo)
{
string appId = "20230722001753350";
string passWord = "your password";
string randomNum = new Random().Next().ToString();
string md5Sign = FormsAuthentication.HashPasswordForStoringInConfigFile(appId + content + randomNum + passWord, "MD5").ToLower();
string FullRequest = "http://api.fanyi.baidu.com/api/trans/vip/translate?q=" + content + "&from=" + languageFrom + "&to=" + languageTo + "&appid=" + appId + "&salt=" + randomNum + "&sign=" + md5Sign;
try
{
string resultContent = new WebClient().DownloadString(FullRequest);
FeedbackResult feedbackResult = new JavaScriptSerializer().Deserialize<FeedbackResult>(resultContent);
feedbackResult.ResponseJson = resultContent;
return feedbackResult;
}
catch (Exception ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message);
return new FeedbackResult()
{
Error_code = "-1",
Error_msg = ex.Message
};
}
}
/// <summary>
/// 使用Post的方式调用百度API进行翻译
/// </summary>
/// <param name="content">要转化的文本</param>
/// <param name="languageFrom">要转化的语言</param>
/// <param name="languageTo">目标语言</param>
/// <returns></returns>
public static FeedbackResult TranslatePost(string content, string languageFrom, string languageTo)
{
string appId = "20230722001753350";
string passWord = "your password";
string randomNum = new Random().Next().ToString();
#region 获取MD5加密编码后的小写格式文本
System.Security.Cryptography.MD5 md5 = System.Security.Cryptography.MD5.Create();
byte[] buffer = md5.ComputeHash(Encoding.UTF8.GetBytes(appId + content + randomNum + passWord));
string md5Sign = string.Join("", buffer.Select(x => x.ToString("x2")));//转化为小写
#endregion
try
{
WebClient webClient = new WebClient();
System.Collections.Specialized.NameValueCollection nameValueCollection = new System.Collections.Specialized.NameValueCollection();
nameValueCollection.Add("q", content);
nameValueCollection.Add("from", languageFrom);
nameValueCollection.Add("to", languageTo);
nameValueCollection.Add("appid", appId);
nameValueCollection.Add("salt", randomNum);
nameValueCollection.Add("sign", md5Sign);
string apiUrl = "http://api.fanyi.baidu.com/api/trans/vip/translate";
byte[] bytes = webClient.UploadValues(apiUrl, "POST", nameValueCollection);
string resultContent = Encoding.UTF8.GetString(bytes);
FeedbackResult feedbackResult = new JavaScriptSerializer().Deserialize<FeedbackResult>(resultContent);
feedbackResult.ResponseJson = resultContent;
return feedbackResult;
}
catch (Exception ex)
{
System.Windows.Forms.MessageBox.Show(ex.Message);
return new FeedbackResult()
{
Error_code = "-1",
Error_msg = ex.Message
};
}
}
}
/// <summary>
/// 反馈结果类
/// </summary>
public class FeedbackResult
{
/// <summary>
/// 错误号,翻译出错时有此项,翻译成功时该项为null
/// </summary>
public string Error_code { set; get; }
/// <summary>
/// 错误消息,翻译出错时代表出错信息,翻译成功时该项为null
/// </summary>
public string Error_msg { set; get; }
/// <summary>
/// 起始语言
/// </summary>
public string From { set; get; }
/// <summary>
/// 目标语言
/// </summary>
public string To { set; get; }
/// <summary>
/// 响应的源Json字符串,自定义项
/// </summary>
public string ResponseJson { set; get; }
/// <summary>
/// 翻译结果,翻译失败时该项为null,翻译成功时按照换行符"\n"来分割的数组,元组数据
/// </summary>
public TranslateResult[] Trans_result { set; get; }
}
/// <summary>
/// 翻译结果类
/// </summary>
public class TranslateResult
{
/// <summary>
/// 源文本 Source
/// </summary>
public string Src { set; get; }
/// <summary>
/// 翻译后的文本 Destination
/// </summary>
public string Dst { set; get; }
}
/// <summary>
/// 语言枚举常数
/// </summary>
public class Language
{
public const string 自动检测 = "auto";
public const string 中文 = "zh";
public const string 英语 = "en";
public const string 粤语 = "yue";
public const string 文言文 = "wyw";
public const string 日语 = "jp";
public const string 韩语 = "kor";
public const string 法语 = "fra";
public const string 西班牙语 = "spa";
public const string 泰语 = "th";
public const string 阿拉伯语 = "ara";
public const string 俄语 = "ru";
public const string 葡萄牙语 = "pt";
public const string 德语 = "de";
public const string 意大利语 = "it";
public const string 希腊语 = "el";
public const string 荷兰语 = "nl";
public const string 波兰语 = "pl";
public const string 保加利亚语 = "bul";
public const string 爱沙尼亚语 = "est";
public const string 丹麦语 = "dan";
public const string 芬兰语 = "fin";
public const string 捷克语 = "cs";
public const string 罗马尼亚语 = "rom";
public const string 斯洛文尼亚语 = "slo";
public const string 瑞典语 = "swe";
public const string 匈牙利语 = "hu";
public const string 繁体中文 = "cht";
public const string 越南语 = "vie";
}
}
三、窗体FormTranslator测试程序如下:
文件FormTranslator.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace TranslatorDemo
{
public partial class FormTranslator : Form
{
public FormTranslator()
{
InitializeComponent();
/*string test = @"上料X轴 错误
上料X轴 伺服错误
上料X轴 手自动冲突
上料Y轴 错误
上料Y轴 伺服错误
上料Y轴 手自动冲突
上料Z轴 错误
上料Z轴 伺服错误
上料Z轴 手自动冲突
上料模组取料夹爪气缸 原位报警
上料模组取料夹爪气缸 动位报警
上料模组取料夹爪气缸 手自动冲突
上料模组取料夹爪气缸 错误
上料模组升降气缸 原位报警
上料模组升降气缸 动位报警
上料模组升降气缸 手自动冲突
上料模组升降气缸 错误
上料模组滑台气缸 原位报警
上料模组滑台气缸 动位报警
上料模组滑台气缸 手自动冲突
上料模组滑台气缸 错误
上料模组 取料失败
上料模组 中途掉料
上料模组 夹爪放料报警
上料模组 夹爪取料报警";
string english = @"message queue
death knight
sword seven
the move speed is 200 mm/s
thunder bird";*/
}
private void bntToEnglish_Click(object sender, EventArgs e)
{
rtxtEnglish.Clear();
FeedbackResult feedbackResult = TranslateUtil.TranslatePost(rtxtChinese.Text.Trim(), Language.中文, Language.英语);
TranslateResult[] contents = feedbackResult.Trans_result;
for (int i = 0; contents != null && i < contents.Length; i++)
{
rtxtEnglish.AppendText($"【{contents[i].Dst}】<-【{contents[i].Src}】\n");
}
rtxtEnglish.AppendText($"响应的源Json数据为:\n{feedbackResult.ResponseJson}");
}
private void btnToChinese_Click(object sender, EventArgs e)
{
rtxtChinese.Clear();
FeedbackResult feedbackResult = TranslateUtil.TranslateGet(rtxtEnglish.Text.Trim(), Language.英语, Language.中文);
TranslateResult[] contents = feedbackResult.Trans_result;
for (int i = 0; contents != null && i < contents.Length; i++)
{
rtxtChinese.AppendText($"【{contents[i].Dst}】<-【{contents[i].Src}】\n");
}
rtxtChinese.AppendText($"响应的源Json数据为:\n{feedbackResult.ResponseJson}");
}
}
}
四、测试运行如图:
【需要到百度上申请开发者账号appId和密码password】
①中文--->英文
②英文--->中文
更多推荐
所有评论(0)