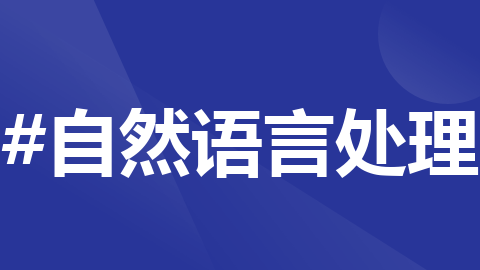
NLP(五十七)LangChain的结构化输出
本文主要介绍了LangChain的结构化输出,这在我们需要对模型输出结果进行结构化解析时较为有用,同时,我们也能接住结构化输出完成一些常见的NLP的任务,如NER等。
在使用大模型的时候,尽管我们的prompt已经要求大模型给出固定的输出格式,比如JSON,但很多时候,大模型还是会输出额外的信息,使得我们在对输出信息进行结构化的时候产生困难。LangChain工具可以很好地将输出信息进行结构化。
关于LangChain的结构化输出,可参考网址:https://python.langchain.com/docs/modules/model_io/output_parsers/ ,这其中,我们较为关注的是Structured output parser
,通过定义StructuredOutputParser
来使用,使用方式如下:
response_schemas = [
ResponseSchema(name="answer", description="answer to the user's question"),
ResponseSchema(name="source", description="source used to answer the user's question, should be a website.")
]
output_parser = StructuredOutputParser.from_response_schemas(response_schemas)
一个例子
首先,我们先来看一个简单的结构化输出的prompt的写法:
# -*- coding: utf-8 -*-
from langchain.output_parsers import StructuredOutputParser, ResponseSchema
from langchain.prompts import PromptTemplate
# 告诉他我们生成的内容需要哪些字段,每个字段类型式啥
response_schemas = [
ResponseSchema(type="string", name="bad_string", description="This a poorly formatted user input string"),
ResponseSchema(type="string", name="good_string", description="This is your response, a reformatted response")
]
# 初始化解析器
output_parser = StructuredOutputParser.from_response_schemas(response_schemas)
# 生成的格式提示符
format_instructions = output_parser.get_format_instructions()
print(format_instructions)
# 加入至template中
template = """
You will be given a poorly formatted string from a user.
Reformat it and make sure all the words are spelled correctly
{format_instructions}
% USER INPUT:
{user_input}
YOUR RESPONSE:
"""
# 将我们的格式描述嵌入到prompt中去,告诉llm我们需要他输出什么样格式的内容
prompt = PromptTemplate(
input_variables=["user_input"],
partial_variables={"format_instructions": format_instructions},
template=template
)
promptValue = prompt.format(user_input="welcom to califonya!")
print(promptValue)
此时,结构化输出的内容如下:
The output should be a markdown code snippet formatted in the following schema, including the leading and trailing "```json" and "```":
\```json
{
"bad_string": string // This a poorly formatted user input string
"good_string": string // This is your response, a reformatted response
}
\```
prompt的内容如下:
You will be given a poorly formatted string from a user.
Reformat it and make sure all the words are spelled correctly
The output should be a markdown code snippet formatted in the following schema, including the leading and trailing "```json" and "```":
\```json
{
"bad_string": string // This a poorly formatted user input string
"good_string": string // This is your response, a reformatted response
}
\```
% USER INPUT:
welcom to califonya!
YOUR RESPONSE:
将上述内容使用LLM进行回复,示例Pyhon代码如下(接上述代码):
from langchain.llms import OpenAI
# set api key
import os
os.environ["OPENAI_API_KEY"] = 'sk-xxx'
llm = OpenAI(model_name="text-davinci-003")
llm_output = llm(promptValue)
print(llm_output)
# 使用解析器进行解析生成的内容
print(output_parser.parse(llm_output))
输出结果如下:
```json
{
"bad_string": "welcom to califonya!",
"good_string": "Welcome to California!"
}
\```
{'bad_string': 'welcom to califonya!', 'good_string': 'Welcome to California!'}
可以看到,大模型的输出结果正是我们所要求的JSON格式,且字段和数据类型、输出结果付出预期。
结构化抽取
有了上述的结构化输出,我们尝试对文本进行结构化抽取,并使得抽取结果按JSON格式输出。示例代码如下:
# -*- coding: utf-8 -*-
from langchain.output_parsers import StructuredOutputParser, ResponseSchema
from langchain.prompts import PromptTemplate
from langchain.llms import OpenAI
# set api key
import os
os.environ["OPENAI_API_KEY"] = 'sk-xxx'
llm = OpenAI(model_name="gpt-3.5-turbo")
# 告诉他我们生成的内容需要哪些字段,每个字段类型式啥
response_schemas = [
ResponseSchema(type="number", name="number", description="文本中的数字"),
ResponseSchema(type="string", name="people", description="文本中的人物"),
ResponseSchema(type="string", name="place", description="文本中的地点"),
]
# 初始化解析器
output_parser = StructuredOutputParser.from_response_schemas(response_schemas)
# 生成的格式提示符
format_instructions = output_parser.get_format_instructions()
print(format_instructions)
template = """
给定下面的文本,找出特定的结构化信息。
{format_instructions}
% USER INPUT:
{user_input}
YOUR RESPONSE:
"""
# prompt
prompt = PromptTemplate(
input_variables=["user_input"],
partial_variables={"format_instructions": format_instructions},
template=template
)
promptValue = prompt.format(user_input="张晓明今天在香港坐了2趟地铁。")
print(promptValue)
llm_output = llm(promptValue)
print(llm_output)
# 使用解析器进行解析生成的内容
print(output_parser.parse(llm_output))
在这个例子中,我们要求从输入文本中抽取出number、people、place字段,数据类型分别为number、string、string,抽取要求为文本中的数字、人物、地点。注意,ResponseSchema中的数据类型(type)与JSON数据类型一致。
对两个样例文本进行抽取,抽取结果如下:
输入:张晓明今天在香港坐了2趟地铁。
抽取结果:{‘number’: 2, ‘people’: ‘张晓明’, ‘place’: ‘香港’}
输入:昨天B站14周年的分享会上,B站CEO陈睿对这个指标做了官方的定义,用户观看视频所花费的时间,也就是播放分钟数。
抽取结果:{‘number’: 14, ‘people’: ‘陈睿’, ‘place’: ‘B站’}
抽取的结果大多符合预期,除了第二句中将B站识别为地点,不太合理。当然,我们写的prompt过于简单,读者可以尝试更多更好的prompt的描述方式,这样也许能提升抽取的效果。
结构化抽取进阶
在这个例子中,我们使用结构化输出,来实现NLP中的常见任务:命名实体识别(NER)
,我们需要从文本中抽取出其中的时间、人物、地点、组织机构等,并以JSON格式输出,每个字段都以列表形式呈现。
实现的Python代码如下:
# -*- coding: utf-8 -*-
from langchain.output_parsers import StructuredOutputParser, ResponseSchema
from langchain.prompts import PromptTemplate
from langchain.llms import OpenAI
# set api key
import os
os.environ["OPENAI_API_KEY"] = 'sk-xxx'
llm = OpenAI(model_name="gpt-3.5-turbo")
# 告诉他我们生成的内容需要哪些字段,每个字段类型式啥
response_schemas = [
ResponseSchema(type="array", name="time", description="文本中的日期时间列表"),
ResponseSchema(type="array", name="people", description="文本中的人物列表"),
ResponseSchema(type="array", name="place", description="文本中的地点列表"),
ResponseSchema(type="array", name="org", description="文本中的组织机构列表"),
]
# 初始化解析器
output_parser = StructuredOutputParser.from_response_schemas(response_schemas)
# 生成的格式提示符
format_instructions = output_parser.get_format_instructions()
print(format_instructions)
template = """
给定下面的文本,找出特定的实体信息,并以结构化数据格式返回。
{format_instructions}
% USER INPUT:
{user_input}
YOUR RESPONSE:
"""
# prompt
prompt = PromptTemplate(
input_variables=["user_input"],
partial_variables={"format_instructions": format_instructions},
template=template
)
promptValue = prompt.format(user_input="6月26日,广汽集团在科技日上首次公开展示飞行汽车项目,飞行汽车GOVE完成全球首飞。广汽研究院院长吴坚表示,GOVE可以垂直起降,并搭载双备份多旋翼飞行系统,保障飞行安全。")
print(promptValue)
llm_output = llm(promptValue)
print(llm_output)
# 使用解析器进行解析生成的内容
print(output_parser.parse(llm_output))
我们在三个示例文本中进行实验,看看大模型在NER方面的表现:
输入:6月26日周一,乌克兰总统泽连斯基视察了乌克兰军队东线司令部总部,而就在几小时前,俄罗斯宣布控制该国东部顿涅茨克以南的利夫诺波尔。
抽取结果:{‘time’: [‘6月26日周一’], ‘people’: [‘泽连斯基’], ‘place’: [‘乌克兰军队东线司令部总部’, ‘顿涅茨克’, ‘利夫诺波尔’], ‘org’: [‘乌克兰总统’, ‘俄罗斯’]}
输入:日前,马自达找来梁家辉代言汽车,引发了业内的热议。相信很多人对于马自达的品牌认知来自梁家辉的那部电影。
抽取结果:{‘time’: [], ‘people’: [‘梁家辉’], ‘place’: [], ‘org’: [‘马自达’]}
输入:6月26日,广汽集团在科技日上首次公开展示飞行汽车项目,飞行汽车GOVE完成全球首飞。广汽研究院院长吴坚表示,GOVE可以垂直起降,并搭载双备份多旋翼飞行系统,保障飞行安全。
抽取结果:{‘time’: [‘6月26日’], ‘people’: [‘吴坚’], ‘place’: [], ‘org’: [‘广汽集团’, ‘广汽研究院’]}
可以看到,在经过简单的prompt和结构化输出后,大模型的抽取结果大致上令人满意。
总结
本文主要介绍了LangChain的结构化输出,这在我们需要对模型输出结果进行结构化解析时较为有用,同时,我们也能接住结构化输出完成一些常见的NLP的任务,如NER等。
参考文献
- LangChain 中文入门教程: https://liaokong.gitbook.io/llm-kai-fa-jiao-cheng/
- Structured output parser: https://python.langchain.com/docs/modules/model_io/output_parsers/structured
欢迎关注我的公众号NLP奇幻之旅,原创技术文章第一时间推送。
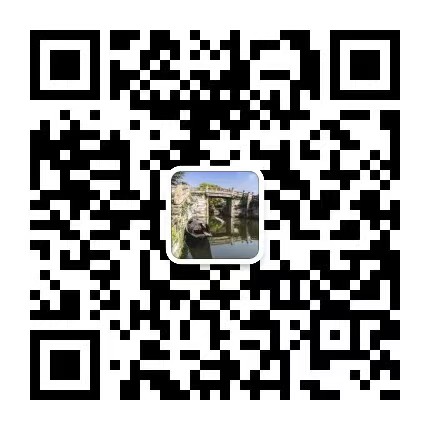
欢迎关注我的知识星球“自然语言处理奇幻之旅”,笔者正在努力构建自己的技术社区。
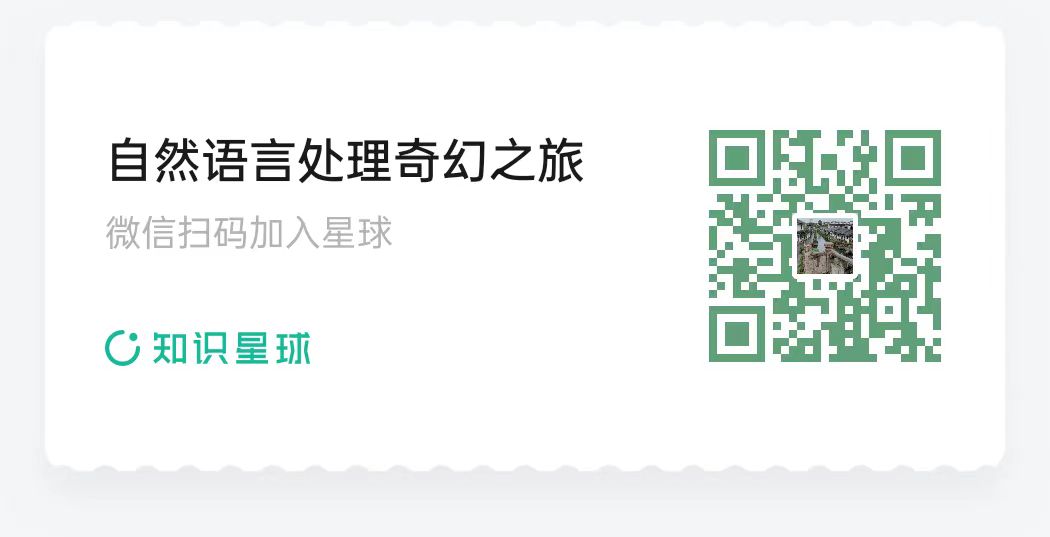
更多推荐
所有评论(0)