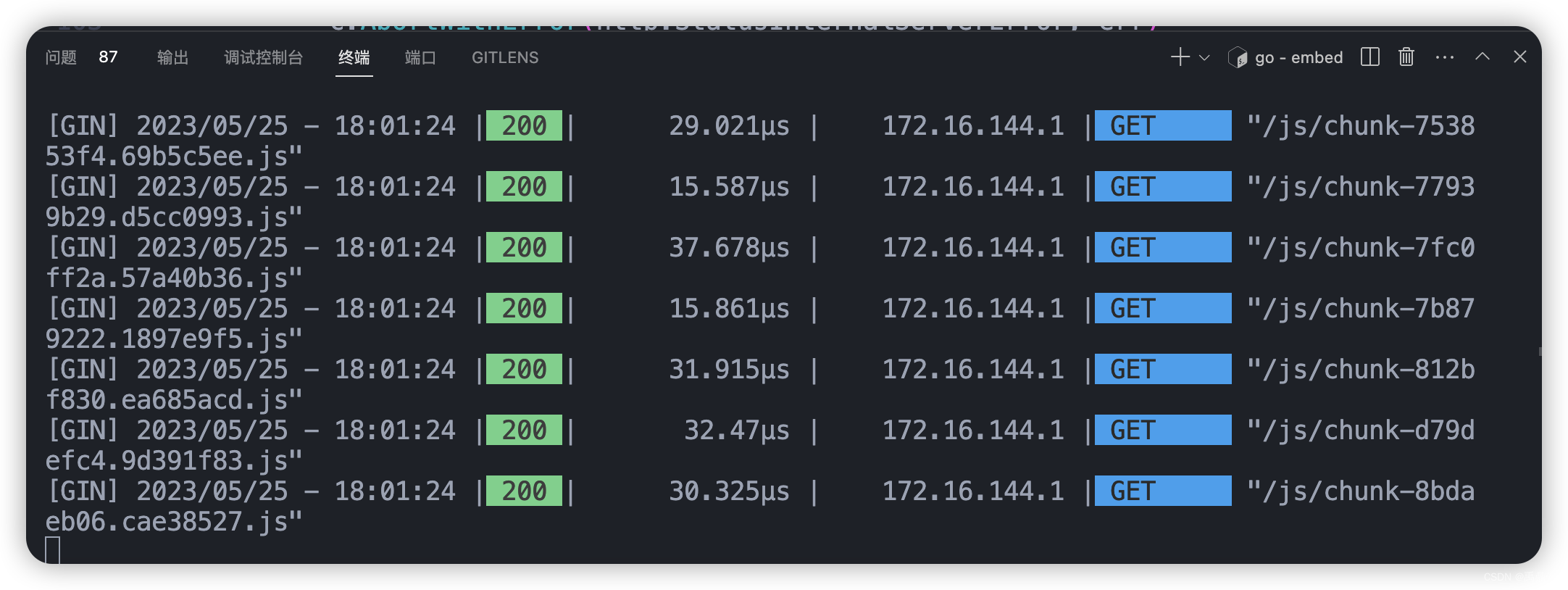
go embed 实现gin + vue静态资源嵌入
golang1.16出来以后,早就有打算把项目的前端代码打包更换成embed。在此之前,使用的是进行的打包。但是今天打包时却报了个错:而且通过各种手段尝试均无果之后,果断把决定立即将其更换为embed进行资源嵌入管理。但是在折腾的时候,其实也是遇到了不少的坑,在这里总结一下。关于embed,具体的功能这里就不多做介绍了,网上有一堆资料,但是这些资料并不能解决我的问题,我翻阅了无数的网页,也没有找到
前言
golang
1.16出来以后,早就有打算把ckman项目的前端代码打包更换成embed。在此之前,使用的是pkger进行的打包。
但是今天打包时却报了个错:
而且通过各种手段尝试均无果之后,果断把决定立即将其更换为embed
进行资源嵌入管理。
但是在折腾的时候,其实也是遇到了不少的坑,在这里总结一下。
关于embed
,具体的功能这里就不多做介绍了,网上有一堆资料,但是这些资料并不能解决我的问题,我翻阅了无数的网页,也没有找到一个能确切解决我的问题的方案。因此决定自己记录一下。
embed资源嵌入
ckman
项目的后端框架是gin
,前端代码是vue
,我们可以从这里下载到其前端代码的静态资源。目录大概长这个样子:
第一版代码(完全不能用)
第一版代码如下:
package main
import (
"embed"
"fmt"
"net/http"
"github.com/gin-gonic/gin"
)
//go:embed static/dist
var f embed.FS
func main() {
r := gin.Default()
r.NoRoute(func(c *gin.Context) {
data, err := f.ReadFile("static/dist/index.html")
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
c.Data(http.StatusOK, "text/html; charset=utf-8", data)
})
err := r.Run("0.0.0.0:5999")
if err != nil {
fmt.Println(err)
}
}
通过后台日志,可以看到资源是可以加载出来的:
但是前端完全没有任何显示:
因此,这一版代码是完全不能工作的。
第二版代码(静态资源可以加载)
上一版代码不能工作,肯定是资源加载不全导致的,因此,在第二版代码里,我做了下改动:
package main
import (
"embed"
"fmt"
"net/http"
"github.com/gin-gonic/gin"
)
//go:embed static/dist
var f embed.FS
func main() {
r := gin.Default()
st, _ := fs.Sub(f, "static/dist")
r.StaticFS("/", http.FS(st))
r.NoRoute(func(c *gin.Context) {
data, err := f.ReadFile("static/dist/index.html")
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
c.Data(http.StatusOK, "text/html; charset=utf-8", data)
})
err := r.Run("0.0.0.0:5999")
if err != nil {
fmt.Println(err)
}
}
与上一版代码相比,只增加了两行内容:
st, _ := fs.Sub(f, "static/dist")
r.StaticFS("/", http.FS(st))
此时首页正常加载出来了。
但是,当我讲这段代码放到项目里使用时,却根本无法工作!
原因就是路由冲突了。
因为gin
框架采用了wildchard
的方式对路由进行处理,我们在上面的代码中:
r.StaticFS("/", http.FS(st))
其实在gin
处理时,已经匹配了/*filepath
,因此,当我们还有其他路由需要处理时,就无法工作了,也就出现了路由冲突。
我们将上面代码改一下,模拟路由冲突的场景:
package main
import (
"embed"
"fmt"
"net/http"
"github.com/gin-gonic/gin"
)
//go:embed static/dist
var f embed.FS
func main() {
r := gin.Default()
st, _ := fs.Sub(f, "static/dist")
r.StaticFS("/", http.FS(st))
r.NoRoute(func(c *gin.Context) {
data, err := f.ReadFile("static/dist/index.html")
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
c.Data(http.StatusOK, "text/html; charset=utf-8", data)
})
r.GET("/api/hello", func(c *gin.Context) {
c.JSON(200, gin.H{
"msg": "hello world",
})
})
err := r.Run("0.0.0.0:5999")
if err != nil {
fmt.Println(err)
}
}
我们在这里增加了一个/api/hello
的路由,当访问这个路由时,返回{"msg":"hello world"}
。
当再次运行时,发现直接panic
了:
报错内容为: conflicts with existing wildcard '/*filepath' in existing prefix '/*filepath'
接下来,我们就来解决这个问题。
第三版代码(解决路由冲突问题)
解决路由冲突的方法有很多,但目前为止,都没有一个比较优雅的解决方案。比如重定向,代价就是要修改前端代码,这么做会显得比较非主流,而且修改前端代码,对我挑战有点大,因此没有用这种方案。
第二种方案是使用中间件。但目前为止没有一款比较好的中间件可以完美支持embed的资源嵌入。因此需要自己实现。
实现代码如下:
const INDEX = "index.html"
type ServeFileSystem interface {
http.FileSystem
Exists(prefix string, path string) bool
}
type localFileSystem struct {
http.FileSystem
root string
indexes bool
}
func LocalFile(root string, indexes bool) *localFileSystem {
return &localFileSystem{
FileSystem: gin.Dir(root, indexes),
root: root,
indexes: indexes,
}
}
func (l *localFileSystem) Exists(prefix string, filepath string) bool {
if p := strings.TrimPrefix(filepath, prefix); len(p) < len(filepath) {
name := path.Join(l.root, p)
stats, err := os.Stat(name)
if err != nil {
return false
}
if stats.IsDir() {
if !l.indexes {
index := path.Join(name, INDEX)
_, err := os.Stat(index)
if err != nil {
return false
}
}
}
return true
}
return false
}
func ServeRoot(urlPrefix, root string) gin.HandlerFunc {
return Serve(urlPrefix, LocalFile(root, false))
}
// Static returns a middleware handler that serves static files in the given directory.
func Serve(urlPrefix string, fs ServeFileSystem) gin.HandlerFunc {
fileserver := http.FileServer(fs)
if urlPrefix != "" {
fileserver = http.StripPrefix(urlPrefix, fileserver)
}
return func(c *gin.Context) {
if fs.Exists(urlPrefix, c.Request.URL.Path) {
fileserver.ServeHTTP(c.Writer, c.Request)
c.Abort()
}
}
}
type embedFileSystem struct {
http.FileSystem
}
func (e embedFileSystem) Exists(prefix string, path string) bool {
_, err := e.Open(path)
if err != nil {
return false
}
return true
}
func EmbedFolder(fsEmbed embed.FS, targetPath string) ServeFileSystem {
fsys, err := fs.Sub(fsEmbed, targetPath)
if err != nil {
panic(err)
}
return embedFileSystem{
FileSystem: http.FS(fsys),
}
}
这样我们在路由中加入该中间件:
func main() {
r := gin.Default()
r.Use(Serve("/", EmbedFolder(f, "static/dist")))
r.NoRoute(func(c *gin.Context) {
data, err := f.ReadFile("static/dist/index.html")
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
c.Data(http.StatusOK, "text/html; charset=utf-8", data)
})
r.GET("/api/hello", func(c *gin.Context) {
c.JSON(200, gin.H{
"msg": "hello world",
})
})
err := r.Run("0.0.0.0:5999")
if err != nil {
fmt.Println(err)
}
}
此时,既能访问静态资源:
也能访问其他的路由接口:
注意事项
我们在调试时需要注意每次运行记得清理浏览器缓存,否则有可能会影响到当前的运行结果。
参考文档
- Golang通过Embed嵌入静态资源
推荐一个零声学院免费教程,个人觉得老师讲得不错,分享给大家:[Linux,Nginx,ZeroMQ,MySQL,Redis,
fastdfs,MongoDB,ZK,流媒体,CDN,P2P,K8S,Docker,
TCP/IP,协程,DPDK等技术内容,点击立即学习: C/C++Linux服务器开发/高级架构师
更多推荐
所有评论(0)