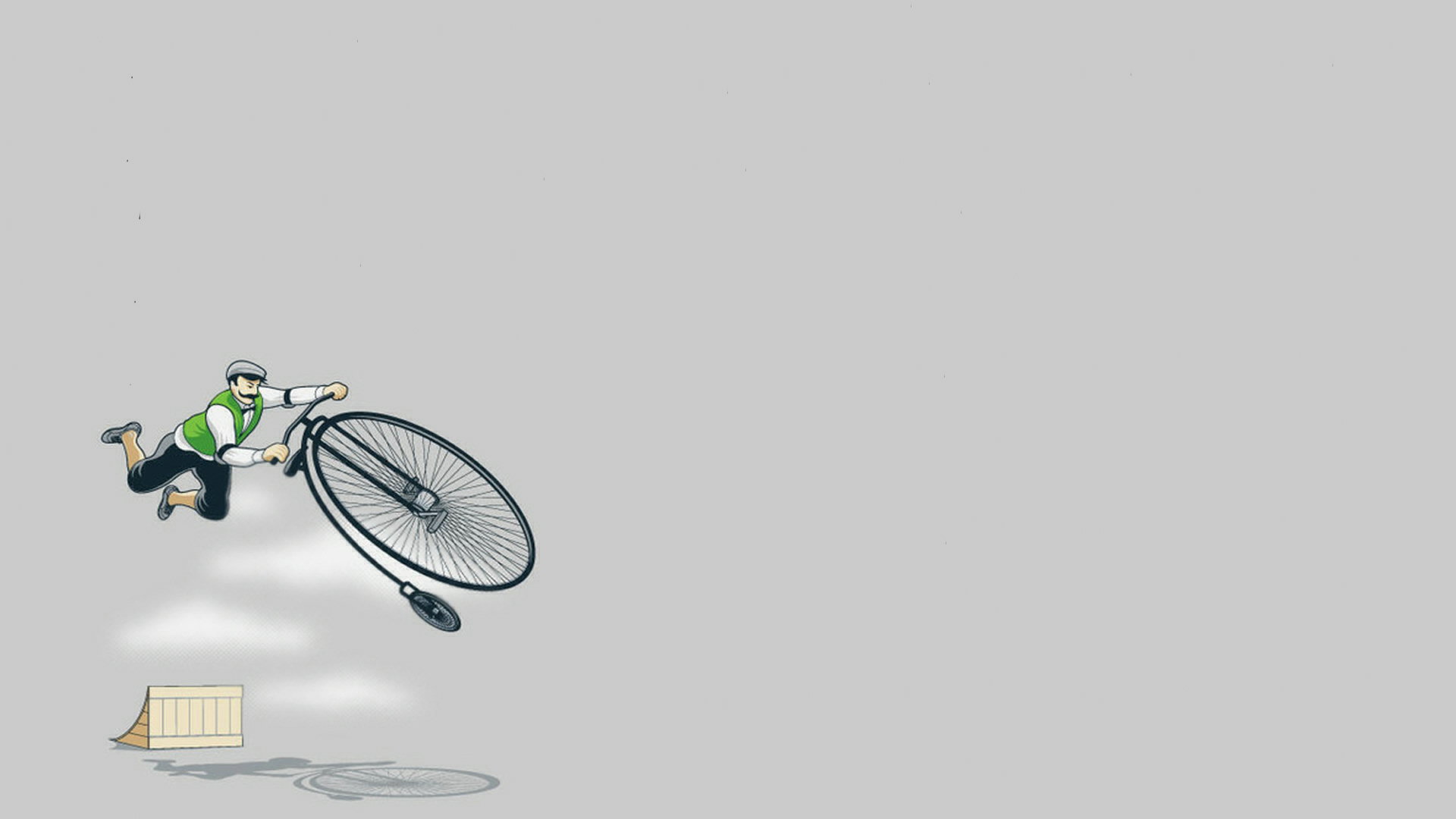
SpringBoot之@ConditionalOnProperty注解
在平时业务中,我们需要在配置文件中配置某个属性来决定是否需要将某些类进行注入,让Spring进行管理,而@ConditionalOnProperty能够实现该功能。@ConditionalOnProperty:根据属性值来控制类或某个方法是否需要加载。它既可以放在类上也可以放在方法上。

目录
在平时业务中,我们需要在配置文件中配置某个属性来决定是否需要将某些类进行注入,让Spring进行管理,而@ConditionalOnProperty能够实现该功能。
@ConditionalOnProperty:根据属性值来控制类或某个方法是否需要加载。它既可以放在类上也可以放在方法上。
1、SpringBoot实现
1.1 设置配置属性
在applicatio.properties或application.yml配置isload_bean = true;
#配置是否加载类
is_load_bean: true
1.2 编写加载类
编写加载类,使用@Component进行注解,为了便于区分,我们将@ConditionalOnProperty放在方法上。
/**
* @author: jiangjs
* @description: 使用@ConditionalOnProperty
* @date: 2023/4/24 10:20
**/
@Component
@Slf4j
public class UseConditionalOnProperty {
@Value("${is_load_bean}")
private String isLoadBean;
@Bean
@ConditionalOnProperty(value = "is_load_bean",havingValue = "true",matchIfMissing = true)
public void loadBean(){
log.info("是否加载当前类");
}
@Bean
public void compareLoadBean(){
log.info("加载bean属性:" + isLoadBean);
}
}
启动项目时输出打印的日志。如图:
将配置文件的数据信息改成false,则不会打印出结果。
2、ConditionalOnProperty属性与源码
2.1 属性
查看@ConditionalOnProperty源码可以看到该注解定义了几个属性。
@Retention(RetentionPolicy.RUNTIME)
@Target({ ElementType.TYPE, ElementType.METHOD })
@Documented
@Conditional(OnPropertyCondition.class)
public @interface ConditionalOnProperty {
/**
* name别名,数组类型,获取对应property名称的值,与name不能同时使用
*/
String[] value() default {};
/**
* 属性前缀,未指定时,自动以点"."结束,有效前缀由一个或多个词用点"."连接。
* 如:spring.datasource
*/
String prefix() default "";
/**
* 属性名称,配置属性完整名称或部分名称,可与prefix组合使用,不能与value同时使用
*/
String[] name() default {};
/**
* 可与name组合使用,比较获取到的属性值与havingValue的值是否相同,相同才加载配置
*/
String havingValue() default "";
/**
* 缺少该配置属性时是否加载,默认为false。如果为true,没有该配置属性时也会正常加载;反之则不会生效
*/
boolean matchIfMissing() default false;
}
1、属性name与value不能同时使用,我们在看源码时会发现做了判断
2、value相当于是name的别名一样,源码中会取value或name赋值给names的属性
3、havingValue:设置的属性值,当配置文件中配置的属性值与havingValue的值相同时才加载该类
4、matchIfMissing:当配置文件中缺少该配置时,是否需要加载类,true:表示加载,false:不加载
2.2 源码
查看OnPropertyCondition类中的getMatchOutcome()方法:
@Override
public ConditionOutcome getMatchOutcome(ConditionContext context, AnnotatedTypeMetadata metadata) {
//获取所有注解ConditionalOnProperty下的所有属性
List<AnnotationAttributes> allAnnotationAttributes = annotationAttributesFromMultiValueMap(
metadata.getAllAnnotationAttributes(ConditionalOnProperty.class.getName()));
List<ConditionMessage> noMatch = new ArrayList<>();
List<ConditionMessage> match = new ArrayList<>();
//遍历注解中的属性
for (AnnotationAttributes annotationAttributes : allAnnotationAttributes) {
//创建判定的结果,ConditionOutcome只有两个属性:match,message
ConditionOutcome outcome = determineOutcome(annotationAttributes, context.getEnvironment());
(outcome.isMatch() ? match : noMatch).add(outcome.getConditionMessage());
}
if (!noMatch.isEmpty()) {
//如果有属性没有匹配,则返回
return ConditionOutcome.noMatch(ConditionMessage.of(noMatch));
}
return ConditionOutcome.match(ConditionMessage.of(match));
}
在上述源码中determineOutcome()是关键方法,我们来看看:
private ConditionOutcome determineOutcome(AnnotationAttributes annotationAttributes, PropertyResolver resolver) {
//初始化
Spec spec = new Spec(annotationAttributes);
List<String> missingProperties = new ArrayList<>();
List<String> nonMatchingProperties = new ArrayList<>();
//收集属性,将结果赋值给missingProperties,nonMatchingProperties
spec.collectProperties(resolver, missingProperties, nonMatchingProperties);
if (!missingProperties.isEmpty()) {
//missingProperties属性不为空,说明设置matchIfMissing的是false,则不加载类
return ConditionOutcome.noMatch(ConditionMessage.forCondition(ConditionalOnProperty.class, spec)
.didNotFind("property", "properties").items(Style.QUOTE, missingProperties));
}
if (!nonMatchingProperties.isEmpty()) {
//nonMatchingProperties属性不为空,则设置的属性值与havingValue值不匹配,则不加载类
return ConditionOutcome.noMatch(ConditionMessage.forCondition(ConditionalOnProperty.class, spec)
.found("different value in property", "different value in properties")
.items(Style.QUOTE, nonMatchingProperties));
}
return ConditionOutcome
.match(ConditionMessage.forCondition(ConditionalOnProperty.class, spec).because("matched"));
}
Spec是OnPropertyCondition的一个静态内部类,初始化@ConditionalOnProperty中的属性。
private static class Spec {
private final String prefix;
private final String havingValue;
private final String[] names;
private final boolean matchIfMissing;
//初始化,给各属性赋值
Spec(AnnotationAttributes annotationAttributes) {
String prefix = annotationAttributes.getString("prefix").trim();
if (StringUtils.hasText(prefix) && !prefix.endsWith(".")) {
prefix = prefix + ".";
}
this.prefix = prefix;
this.havingValue = annotationAttributes.getString("havingValue");
this.names = getNames(annotationAttributes);
this.matchIfMissing = annotationAttributes.getBoolean("matchIfMissing");
}
//处理name与value
private String[] getNames(Map<String, Object> annotationAttributes) {
String[] value = (String[]) annotationAttributes.get("value");
String[] name = (String[]) annotationAttributes.get("name");
//限制了value或name必须指定
Assert.state(value.length > 0 || name.length > 0,
"The name or value attribute of @ConditionalOnProperty must be specified");
//value和name只能有一个存在,不能同时使用
Assert.state(value.length == 0 || name.length == 0,
"The name and value attributes of @ConditionalOnProperty are exclusive");
return (value.length > 0) ? value : name;
}
private void collectProperties(PropertyResolver resolver, List<String> missing, List<String> nonMatching) {
//遍历names,即value或name的值
for (String name : this.names) {
//前缀 + name,获取配置文件中key
String key = this.prefix + name;
//验证配置属性中包含key
if (resolver.containsProperty(key)) {
//如包含,则获取key对应的值,与havingValue的值进行匹配
if (!isMatch(resolver.getProperty(key), this.havingValue)) {
//不匹配则添加到nonMatching
nonMatching.add(name);
}
}
else {
//验证配置属性中没有包含key,判断是否配置了matchIfMissing属性
if (!this.matchIfMissing) {
//该属性不为true则添加到missing中
missing.add(name);
}
}
}
}
private boolean isMatch(String value, String requiredValue) {
//验证requiredValue是否有值
if (StringUtils.hasLength(requiredValue)) {
//有值,则进行比较,不区分大小写
return requiredValue.equalsIgnoreCase(value);
}
//没有值,则验证value是否等于false
//这也是为什么name, value不配置值的情况下, 类依然会被加载的原因
return !"false".equalsIgnoreCase(value);
}
@Override
public String toString() {
StringBuilder result = new StringBuilder();
result.append("(");
result.append(this.prefix);
if (this.names.length == 1) {
result.append(this.names[0]);
}
else {
result.append("[");
result.append(StringUtils.arrayToCommaDelimitedString(this.names));
result.append("]");
}
if (StringUtils.hasLength(this.havingValue)) {
result.append("=").append(this.havingValue);
}
result.append(")");
return result.toString();
}
}
更多推荐
所有评论(0)