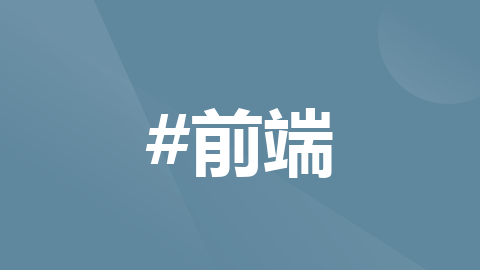
图片上传_RuoYi
当用户选择了要上传的图片文件时,隐藏的 input 元素的 change 事件将触发 handleFileInputChange() 函数。2.在 Vue 组件中定义 uploadUrl、uploadHeaders 和 fileList 等属性,并编写 beforeUpload() 和 handleFileChange() 等方法,用于实现图片上传功能。使用了若依提供的 uploadFile()
若依提供的组件和工具来实现图片上传功能例在前端页面中添加一个上传按钮和一个隐藏的 input 元素,并使用 element-ui 组件库提供的 el-upload 组件创建一个上传组件。
<template>
<el-upload
class="upload-demo"
:action="uploadUrl"
:headers="uploadHeaders"
:file-list="fileList"
:auto-upload="false"
:on-change="handleFileChange"
:before-upload="beforeUpload"
>
<el-button slot="trigger" size="small" type="primary">
上传图片
</el-button>
<div slot="tip" class="el-upload__tip">
只能上传 jpg/png 文件,且不超过 2MB
</div>
</el-upload>
<!-- 隐藏的 input 元素 -->
<input
type="file"
ref="fileInput"
style="width: 0; height: 0; border: none; margin: 0; padding: 0;"
@change="handleFileInputChange"
/>
</template>
在这个示例中,我们为 el-upload 组件绑定了一些属性,包括上传地址、请求头、上传文件列表、自动上传等。我们还为上传按钮绑定了一个点击事件,用于触发隐藏的 input 元素的点击事件。当用户选择了要上传的图片文件时,隐藏的 input 元素的 change 事件将触发 handleFileInputChange() 函数。
2.在 Vue 组件中定义 uploadUrl、uploadHeaders 和 fileList 等属性,并编写 beforeUpload() 和 handleFileChange() 等方法,用于实现图片上传功能。
<script>
import { uploadFile } from "@/api/common";
export default {
data() {
return {
// 图片上传地址
uploadUrl: "/common/upload",
// 图片上传请求头
uploadHeaders: { "Authorization": "Bearer " + getToken() },
// 图片文件列表
fileList: []
};
},
methods: {
// 文件上传前的校验
beforeUpload(file) {
const isJPG = file.type === "image/jpeg" || file.type === "image/png";
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isJPG) {
this.$message.error("上传的图片只能是 JPG/PNG 格式!");
}
if (!isLt2M) {
this.$message.error("上传的图片大小不能超过 2MB!");
}
return isJPG && isLt2M;
},
// 选择文件后触发
handleFileChange(file, fileList) {
const formData = new FormData();
formData.append("file", file.raw);
uploadFile(formData)
.then(response => {
this.$message.success("上传成功!");
const url = response.data.url;
const image = {
name: file.name,
url: url
};
this.fileList.push(image);
})
.catch(error => {
this.$message.error("上传失败!");
console.log(error);
});
},
// 触发隐藏的 input 元素的点击事件
showFileInput() {
this.$refs.fileInput.click();
}
}
};
</script>
使用了若依提供的 uploadFile() 方法,将文件上传到服务器,并将上传后的返回值中的图片 URL 添加到 fileList 中。
beforeUpload() 方法,用于校验上传的文件是否符合要求。
上传文件不符合要求,提示用户错误信息。
showFileInput() 方法,用于触发隐藏的 input 元素的点击事件。
更多推荐
所有评论(0)