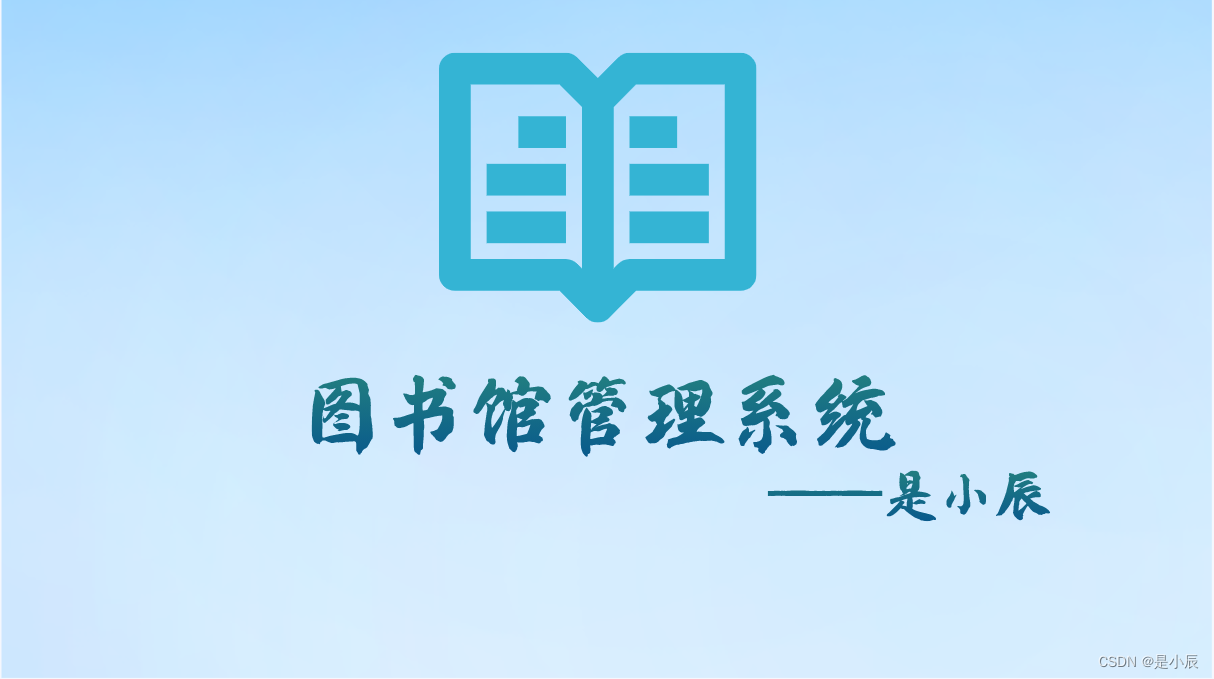
图书馆管理系统(Java编写,思路及源代码)
还在担心图书馆课设不会做吗?不用担心,一篇博客带你完成图书馆管理系统。

![]()
如果你已经学习了Java的三大特性(封装、继承、多态)及接口,那么你就可以尝试这个编写这个图书馆管理系统小项目,这个小项目主要的作用还是用来巩固Java的三大特性及接口的学习。(我前边的几个博客中也详细介绍了三大特性及接口:Java封装_是小辰的博客-CSDN博客 Java继承_是小辰的博客-CSDN博客 Java多态_是小辰的博客-CSDN博客 Java 接口_是小辰的博客-CSDN博客)
当这个图书馆管理系统运行的时候,首先会进入图书管理系统,然后要求使用者首先输入自己的名字,输入完成后选择进入系统的身份,有管理员和普通用户两种身份,如果身份是管理员要有增添图书,删除图书,查找图书,展示图书和退出系统五种功能;而是普通用户则要求有查找图书,借阅图书,归还图书和退出系统四种功能。
为了使代码清晰简洁,我们可以把不同功能的板块分成不同包,例如:把与书相关的都在book包中实现,把与身份有关的都在user包中实现,把与功能有关的都在function包中实现。而我们还需要一个程序入口,那么我们就可以再创建一个Main类。
如图:
一、book包
book包中我们可以实现创建图书对象及信息,管理图书,所以我们可以分为Book类和Book List类。
![]()
Book类
我们发现不论是管理员还是普通用户,他们除了退出系统之外的功能都是对书进行操作;所以我们首先创建一个Book类,在Book类使书具有String类型的书名(name),String类型的作者(author),int类型的价钱(price),String类型的书的类型(type)和Boolean类型的借阅状态(isBorrow)五种属性,在Book类中需要重写toString方法,需要注意的时要根据书的isBorrow的状态来打印已借出(true)或者未借出(false)。
package book;
public class Book {
private String name;
private String author;
private int price;
private String type;
private boolean isBorrow;
public Book(String name, String author, int price, String type) {
this.name = name;
this.author = author;
this.price = price;
this.type = type;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
", type='" + type + '\'' +
((isBorrowed == true) ? " 已借出":" 未借出") +
'}';
}
public Book() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public boolean isBorrow() {
return isBorrow;
}
public void setBorrow(boolean borrow) {
isBorrow = borrow;
}
}
BookList类
BookList类主要事为了方便操作Book类,我们可以把Book类的对象保存在Booklist类的Book类型的books数组中,我们使用实例代码块来创建几个Book类的对象并且存放在books数组中,为了方便管理,我们需要设置一个书的数量的成员变量并且仅对本类可见,所以我们是使用private关键字对其进行修饰,并且为其设置get和set方法。
package book;
public class BookList {
private Book[] books = new Book[10];
private int usedSize;//记录当前书架上有几本书
public BookList() {
books[0] = new Book("三国演义","罗贯中",20,"小说");
books[1] = new Book("西游记","吴承恩",30,"小说");
books[2] = new Book("水浒传","施耐庵",25,"小说");
this.usedSize = 3;
}
public Book getBook(int pos) {
return books[pos];
}
public int getUsedSize() {
return usedSize;
}
public void setUsedSize(int usedSize) {
this.usedSize = usedSize;
}
public void setBooks(int pos,Book book) {
books[pos] = book;
}
}
二、function
funtion包中实现书籍的增加、删除、查找、展示、借阅、归还以及系统的退出,这些实现统一用一个接口完成,所以:
IFunction接口
Ifunction接口是所有功能类的公共规范,所以它应该具有抽象 方法work,而且这个方法的操作对象应该是Booklist类的对象;
package function;
public interface IFunction {
void work(BookList bookList);
}
AddFunction类
package function;
public class AddFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("新增图书!");//业务逻辑!!!
Scanner scanner = new Scanner(System.in);
System.out.println("请输入图书的名字:");
String name = scanner.nextLine();
System.out.println("请输入图书的作者:");
String author = scanner.nextLine();
System.out.println("请输入图书的类型:");
String type = scanner.nextLine();
System.out.println("请输入图书的价格:");
int price = scanner.nextInt();
Book book = new Book(name,author,price,type);
int currentSize = bookList.getUsedSize();
bookList.setBooks(currentSize,book);
bookList.setUsedSize(currentSize+1);
System.out.println("新增图书成功!!");
//scanner.close();
}
}
DeleteFunction类
package function;
public class DeleteFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("删除图书!");
//1、找到你要删除的图书是否存在?
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要删除的图书名字:");
String name = scanner.nextLine();//水浒传
int currentSize = bookList.getUsedSize();
int delIndex = -1;
int i = 0;
for (; i < currentSize; i++) {
Book book = bookList.getBook(i);
if (book.getName().equals(name)) {
delIndex = i;
break;
}
}
if (i == currentSize) {
System.out.println("没有你删除的这本书!");
return;
}
for (int j = delIndex; j < currentSize - 1; j++) {
//[j] = [j+1]
Book book = bookList.getBook(j + 1);
bookList.setBooks(j, book);
}
bookList.setBooks(currentSize - 1, null);
bookList.setUsedSize(currentSize - 1);
System.out.println("删除图书成功!");
}
}
BorrowFunction类
package function;
public class BorrowFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("借阅图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要借阅的图书的名字:");
String name = scanner.nextLine();//水浒传
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
if(book.getName().equals(name)) {
if(book.isBorrowed()) {
System.out.println("该书已经被借出!");
}else{
book.setBorrowed(true);
}
return;
}
}
System.out.println("没有你要借阅的图书!");
}
}
FindFunction类
package function;
public class FindFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("查找图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要查找的图书姓名:");
String name = scanner.nextLine();//水浒传
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
if(book.getName().equals(name)) {
System.out.println("找到这本书了!");
System.out.println(book);
return;
}
}
//代码走到这里!!
System.out.println("没有你要查找的这本书!");
}
}
ReturnFunction类
package function;
public class ReturnFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("归还图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要归还的图书的名字:");
String name = scanner.nextLine();//水浒传
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
if(book.getName().equals(name)) {
book.setBorrowed(false);
return;
}
}
System.out.println("没有你要归还的图书!");
}
}
ShowFunction类
package function;
public class ShowFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("打印所有图书!");
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
System.out.println(book);
}
}
}
ExitFunction类
package function;
public class ExitFunction implements IFunction{
@Override
public void work(BookList bookList) {
System.out.println("退出系统!");
System.exit(0);
}
}
三、user包
用户分为两种,所以:
User类
User类应该有保存功能类的Ifunction类型的数组、抽象方法main方法、根据用户选择对booklist对象进行操作的dofunction方法的功能。
package user;
import function.IFunction;
public abstract class User {
protected String name;
public IFunction[] iFunctions;//这里我没有分配空间
public User(String name) {
this.name = name;
}
public abstract int menu();
public void doOperation(int choice, BookList bookList){
this.iFunctions[choice].work(bookList);
}
}
AdminUser类
此类继承User类,需要根据对应的功能重写main方法,并在构造方法中对其Ifunction数组做出赋值选择其需要实现的功能。
package user;
import java.util.Scanner;
import function.*;
public class AdminUser extends User{
public AdminUser(String name) {
super(name);
this.iFunctions = new IFunction[]{
new ExitFunction(),
new FindFunction(),
new AddFunction(),
new DeleteFunction(),
new ShowFunction()
};
}
public int menu() {
System.out.println("管理员菜单!");
System.out.println("****************************");
System.out.println("hello " + this.name +" 欢迎来到图书小练习");
System.out.println("1. 查找图书");
System.out.println("2. 新增图书");
System.out.println("3. 删除图书");
System.out.println("4. 显示图书");
System.out.println("0. 退出系统!");
System.out.println("****************************");
System.out.println("请输入你的操作:");
Scanner scanner = new Scanner(System.in);
int choice = scanner.nextInt();
return choice;
}
}
NormalUser类
package user;
import java.util.Scanner;
import function.*;
public class NormalUser extends User{
public NormalUser(String name) {
super(name);
this.iFunctions = new IFunction[] {
new ExitFunction(),
new FindFunction(),
new BorrowFunction(),
new ReturnFunction()
};
}
public int menu() {
System.out.println("普通用户的菜单!");
System.out.println("****************************");
System.out.println("hello " + this.name +" 欢迎来到图书小练习");
System.out.println("1. 查找图书");
System.out.println("2. 借阅图书");
System.out.println("3. 归还图书");
System.out.println("0. 退出系统!");
System.out.println("****************************");
System.out.println("请输入你的操作:");
Scanner scanner = new Scanner(System.in);
int choice = scanner.nextInt();
return choice;
}
}
更多推荐
所有评论(0)