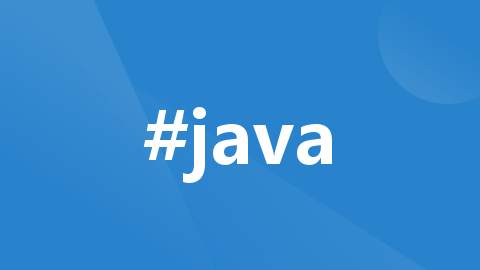
Java文件复制多种方法
java文件复制的方法有几种方式

1、InputStream与OutputStream
创建两个文件 - 源和目标。然后我们从源创建InputStream并使用OutputStream将其写入目标文件进行 java 复制文件操作。
private static void copyFileUsingStream(File source, File dest) throws IOException {
InputStream is = null;
OutputStream os = null;
try {
is = new FileInputStream(source);
os = new FileOutputStream(dest);
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
is.close();
os.close();
}
}
2、Apache Commons IO FileUtils
copyFile(File srcFile, File destFile)可用于在 java 中复制文件。如果您已经在项目中使用 Apache Commons IO,那么使用它来简化代码是有意义的。它在内部使用 Java NIO FileChannel,因此如果您尚未将其用于其他功能,则可以避免使用此包装器方法。下面是使用apache commons io进行java复制文件操作的方法
private static void copyFileUsingApacheCommonsIO(File source, File dest) throws IOException {
FileUtils.copyFile(source, dest);
}
3、Files类的copy()方法在 java 中复制文件
private static void copyFileUsingJava7Files(File source, File dest) throws IOException {
Files.copy(source.toPath(), dest.toPath());
}
4、使用BufferedInputStream/BufferedOutputStream高效字节流进行复制文件
private static void bufferedStreamCopyFile(File srcFile, File desFile) throwsIOException {
//使用缓冲字节流进行文件复制
BufferedInputStream bis = new BufferedInputStream(newFileInputStream(srcFile));
BufferedOutputStream bos = new BufferedOutputStream(newFileOutputStream(desFile));
byte[] b = new byte[1024];
Integer len = 0;
//一次读取1024字节的数据
while((len = bis.read(b)) != -1) {
bos.write(b, 0, len);
}
bis.close();
bos.close();
}
5、使用FileReader/FileWriter字符流进行文件复制
注意这种方式只能复制只包含字符的文件,也就意味着你用记事本打开该文件你能够读懂
private static void readerWriterCopyFile(File srcFile, File desFile) throwsIOException {
//使用字符流进行文件复制,注意:字符流只能复制只含有汉字的文件
FileReader fr = newFileReader(srcFile);
FileWriter fw = newFileWriter(desFile);
Integer by = 0;
while((by = fr.read()) != -1) {
fw.write(by);
}
fr.close();
fw.close();
}
6、使用BufferedReader/BufferedWriter高效字符流进行文件复制
意这种方式只能复制只包含字符的文件,也就意味着你用记事本打开该文件你能够读懂
private static void bufferedReaderWriterCopyFile(File srcFile, File desFile) throwsIOException {
//使用带缓冲区的高效字符流进行文件复制
BufferedReader br = new BufferedReader(newFileReader(srcFile));
BufferedWriter bw = new BufferedWriter(newFileWriter(desFile));
char[] c = new char[1024];
Integer len = 0;
while((len = br.read(c)) != -1) {
bw.write(c, 0, len);
}
//方式二
/*String s = null;
while((s = br.readLine()) != null) {
bw.write(s);
bw.newLine();
}*/
br.close();
bw.close();
}
7、使用BufferedReader/BufferedWriter高效字符流进行文件复制
注意这种方式只能复制只包含字符的文件,也就意味着你用记事本打开该文件你能够读懂
private static void bufferedReaderWriterCopyFile(File srcFile, File desFile) throwsIOException {
//使用带缓冲区的高效字符流进行文件复制
BufferedReader br = new BufferedReader(newFileReader(srcFile));
BufferedWriter bw = new BufferedWriter(newFileWriter(desFile));
char[] c = new char[1024];
Integer len = 0;
while((len = br.read(c)) != -1) {
bw.write(c, 0, len);
}
//方式二
/*String s = null;
while((s = br.readLine()) != null) {
bw.write(s);
bw.newLine();
}*/
br.close();
bw.close();
}
8、使用FileChannel复制
Java NIO包括transferFrom方法,根据文档应该比文件流复制的速度更快
private static void copyFileUsingFileChannels(File source, File dest) throws IOException {
FileChannel inputChannel = null;
FileChannel outputChannel = null;
try {
inputChannel = new FileInputStream(source).getChannel();
outputChannel = new FileOutputStream(dest).getChannel();
outputChannel.transferFrom(inputChannel, 0, inputChannel.size());
} finally {
inputChannel.close();
outputChannel.close();
}
}
更多推荐
所有评论(0)