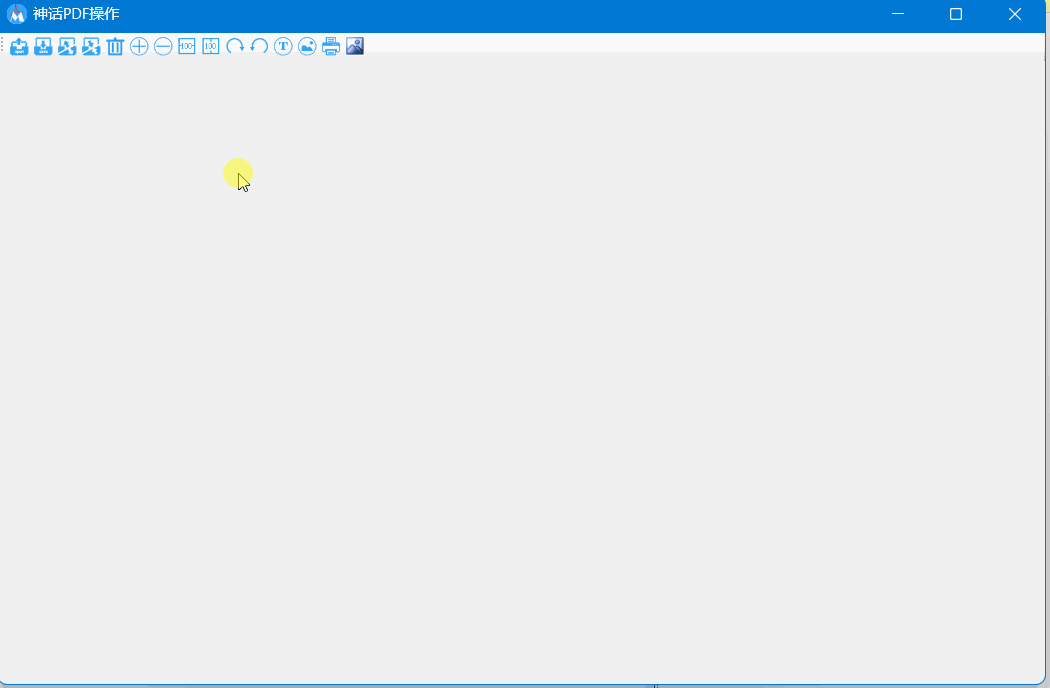
【c#系列】PDF进行操作-浏览、分割、合并、插入、删除(1)
工作所需,需要对PDF进行浏览,分割、插入、删除和合并等功能,并不需要其它多余的功能,一番搜索后,利用开源代码,终于实现了简单的操作,把开发流程进行的简单记录以备不时之需。

工作所需,需要对PDF进行浏览,分割、插入、删除和合并等功能,并不需要其它多余的功能,一番搜索后,利用开源代码,终于实现了简单的操作,把开发流程进行的简单记录以备不时之需。
先预览一下:
一、开发环境及用到的开源类库
1. 开发工具: Visual Studio 2022;
2. 开发环境: Windows 11 家庭中文版;
3. 用到的开源类库:
PdfiumViewer开源类库,该类库 是一个 WinForms 控件,它承载一个 PdfRenderer 控件并添加一个工具栏来保存或打印 PDF 文件。
itextSharp开源类库的是PDF库,它允许你创建,调整,检查和维护的可移植文档格式文件(PDF),我主要用它来实现PDF的分割、插入、删除、合并等该操作。
二、开发步骤记录
(一)界面设置
1. 创建项目。
运行Visual Studio 2022,创建新项目,windows窗体应用,如下图所示。
下一步,命名为MyPdf,选择合适位置,创建项目,如下图所示。
2. 设置主窗体属性
Form1的name设置为“mythMain”,Text设置为“神话PDF操作”,StartPosition设置为“System.Windows.Forms.FormStartPosition.CenterScreen”,如图所示。
3. 页面布局
添加toolStrip菜单,name设置为“ToolsBox”,在toolStrip菜单中添加2个Button,名称分别设置为“OpenBT_Tool”和“SaveBT_Tool”,并对应设置小图标。最终如图所示。
4. 安装PdfiumViewer开源类库
在解决方案管理中,在“引用”上单击右键选择“管理NuGet程序包”,如下图。
下图为安装PdfiumViewer开源类库,很简单,单击安装即可。
安装完毕后工具箱中会出现PdfViewer工具栏。
在窗口中拖入2个PdfViewer,分别设置:name属性为“GuidMenuePdf”和“ViewerPdf”;Dock属性为“ System.Windows.Forms.DockStyle.Left”和“System.Windows.Forms.DockStyle.Fill”;ShowBookmarks属性均为false;ZoomMode属性均设为“PdfiumViewer.PdfViewerZoomMode.FitBest;”;ShowToolbar左侧对齐的设为false,另一个为true。最终页面排版如下图所示。
5. 安装 itextSharp开源类库
itextSharp开源类库的安装与PdfiumViewer开源类库安装类似,如下图。
ok我们的界面设置基本就完成了,下面就是代码编写部分。
(二)代码编写
(一). 实现预览PDF功能
1. 添加载入PDF文件功能
双击toolStrip中的OpenBT_Tool,添加代码:
private int currentPage = 0;
private void OpenBT_Tool_Click(object sender, EventArgs e)
{
using (var form = new OpenFileDialog())
{
form.Filter = "PDF Files (*.pdf)|*.pdf|All Files (*.*)|*.*";
form.RestoreDirectory = true;
form.Title = "Open PDF File";
if (form.ShowDialog(this) != DialogResult.OK)
{
Dispose();
return;
}
StartPdf(form.FileName);
}
}
private void StartPdf(string pdfPath)
{
GuidMenuePdf.Document?.Dispose();
GuidMenuePdf.Document = OpenDocument(pdfPath);
ViewerPdf.Document?.Dispose();
ViewerPdf.Document = GuidMenuePdf.Document;
}
private PdfiumViewer.IPdfDocument OpenDocument(string pdfPath)
{
try
{
return PdfiumViewer.PdfDocument.Load(this, pdfPath);
}
catch (Exception ex)
{
MessageBox.Show(this, ex.Message, Text, MessageBoxButtons.OK, MessageBoxIcon.Error);
return null;
}
}
运行程序,出现了System.DllNotFoundException:“无法加载 DLL“pdfium.dll”: 找不到指定的模块。 (异常来自 HRESULT:0x8007007E)”错误,如下图
我们需要在项目属性中将目标平台选择X64,这样问题就解决,入下图
我们的运行效果如下图。
2. 添加点击左侧切换右侧预览功能
在构造函数添加单击事件,并添加GuidMenuePdf_cilck函数,具体代码如下:
public mythMain()
{
InitializeComponent();
GuidMenuePdf.Renderer.MouseClick += GuidMenuePdf_cilck;//这是我们添加的代码
}
private void GuidMenuePdf_cilck(object sender, MouseEventArgs e)
{
PdfiumViewer.PdfRenderer currentRenderer = (PdfiumViewer.PdfRenderer)sender;
currentPage = currentRenderer.PointToPdf(e.Location).Page;//获取左侧单击的页码是多少
if (e.Button == MouseButtons.Left)//单击左键,我们实现的在右侧切换到相应界面
{
ViewerPdf.Renderer.Page = currentPage;
}
else
{
//单击右键我们准备实现的一些功能
}
}
3. 在导航图片中添加右键菜单
在主窗口拖入工具箱中的ContextMenuStrip,name属性设置ToolMenue,并添加三项分别为:删除、插入、分割;名称分别为: Del_ToolStripMenuItem,Insert_ToolStripMenuItem,Splite_ToolStripMenuItem,如下图:
GuidMenuePdf_cilck中添加右键显示代码:
ToolMenue.Show(MousePosition.X, MousePosition.Y);//显示右键菜单,位置为鼠标所在位置
这样我们在左侧导航菜单图片上单击右键就会出现菜单了。
4. 实现删除功能
新建Form窗口,并进行设置,效果如下图:
页面设计代码具体如下:
this.PdfInfoLB = new System.Windows.Forms.Label();
this.DelTxt = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.CancleBT = new System.Windows.Forms.Button();
this.DelteBT = new System.Windows.Forms.Button();
this.Tslb = new System.Windows.Forms.Label();
this.WxtsLB = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// PdfInfoLB
//
this.PdfInfoLB.AutoSize = true;
this.PdfInfoLB.Location = new System.Drawing.Point(33, 37);
this.PdfInfoLB.Name = "PdfInfoLB";
this.PdfInfoLB.Size = new System.Drawing.Size(165, 15);
this.PdfInfoLB.TabIndex = 1;
this.PdfInfoLB.Text = "本文档共5页,准备删除";
//
// DelTxt
//
this.DelTxt.Location = new System.Drawing.Point(204, 32);
this.DelTxt.Name = "DelTxt";
this.DelTxt.Size = new System.Drawing.Size(289, 25);
this.DelTxt.TabIndex = 2;
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(499, 37);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(22, 15);
this.label1.TabIndex = 3;
this.label1.Text = "页";
//
// CancleBT
//
this.CancleBT.Location = new System.Drawing.Point(123, 160);
this.CancleBT.Name = "CancleBT";
this.CancleBT.Size = new System.Drawing.Size(75, 23);
this.CancleBT.TabIndex = 4;
this.CancleBT.Text = "取消删除";
this.CancleBT.UseVisualStyleBackColor = true;
this.CancleBT.Click += new System.EventHandler(this.CancleBT_Click);
//
// DelteBT
//
this.DelteBT.Location = new System.Drawing.Point(318, 160);
this.DelteBT.Name = "DelteBT";
this.DelteBT.Size = new System.Drawing.Size(75, 23);
this.DelteBT.TabIndex = 5;
this.DelteBT.Text = "确认删除";
this.DelteBT.UseVisualStyleBackColor = true;
this.DelteBT.Click += new System.EventHandler(this.DelteBT_Click);
//
// Tslb
//
this.Tslb.AutoSize = true;
this.Tslb.ForeColor = System.Drawing.Color.Red;
this.Tslb.Location = new System.Drawing.Point(33, 72);
this.Tslb.Name = "Tslb";
this.Tslb.Size = new System.Drawing.Size(82, 15);
this.Tslb.TabIndex = 6;
this.Tslb.Text = "温馨提示:";
//
// WxtsLB
//
this.WxtsLB.AutoSize = true;
this.WxtsLB.Location = new System.Drawing.Point(110, 72);
this.WxtsLB.Name = "WxtsLB";
this.WxtsLB.Size = new System.Drawing.Size(256, 15);
this.WxtsLB.TabIndex = 7;
this.WxtsLB.Text = "1.删除单页,请直接输入页码,如:2";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(110, 98);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(402, 15);
this.label2.TabIndex = 8;
this.label2.Text = "2.删除多页且不连续,请输入页码并用\",\"分割,如:2,4,6";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(110, 124);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(402, 15);
this.label3.TabIndex = 9;
this.label3.Text = "3.删除多页连续页码,请用\"-\",如:2-6,表示删除2到6页";
//
// DelForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(578, 208);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.WxtsLB);
this.Controls.Add(this.Tslb);
this.Controls.Add(this.DelteBT);
this.Controls.Add(this.CancleBT);
this.Controls.Add(this.label1);
this.Controls.Add(this.DelTxt);
this.Controls.Add(this.PdfInfoLB);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedToolWindow;
this.Name = "DelForm";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterParent;
this.Text = "删除页面";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label PdfInfoLB;
private System.Windows.Forms.TextBox DelTxt;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Button CancleBT;
private System.Windows.Forms.Button DelteBT;
private System.Windows.Forms.Label Tslb;
private System.Windows.Forms.Label WxtsLB;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
后台代码:
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MyPdf
{
public partial class DelForm : Form
{
private int deletePage, totalPage;
public ArrayList alist;
public DelForm (int delete,int total)
{
deletePage = delete;
totalPage = total;
InitializeComponent();
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Font = new System.Drawing.Font("Microsoft Sans Serif", 12F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Pixel, ((byte)(134)));
this.DialogResult = DialogResult.Cancel;
}
private void CancleBT_Click(object sender, EventArgs e)
{
this.Close();
}
private void DelteBT_Click(object sender, EventArgs e)
{
if (DelTxt.Text.Trim()=="")
{
MessageBox.Show("删除页面不能为空!");
DelTxt.Focus();
return;
}
alist = new ArrayList();
string[] selectOp = DelTxt.Text.Split(',');
for (int i = 0; i < selectOp.Length; i++)
{
if (string.IsNullOrEmpty(selectOp[i])|| selectOp[i].IndexOf('-')==0)
{
MessageBox.Show("删除页码输入有误!");
DelTxt.Focus();
return;
}
int result = IsNumeric(selectOp[i]);
if (result != -1)
{
alist.Add(result);
}
else if (selectOp[i].IndexOf('-') > 0)
{
string[] tempArea = selectOp[i].Split('-');
if (tempArea.Length == 2)
{
int temp0 = IsNumeric(tempArea[0]);//获取起点数值
int temp1 = IsNumeric(tempArea[1]);//获取终点数值
if (temp0<=temp1)
{
for (int k = temp0; k <= temp1; k++)
{
alist.Add(k);
}
}
else
{
for (int k = temp1; k <= temp0; k++)
{
alist.Add(k);
}
}
}
}
}
this.DialogResult = DialogResult.OK;
this.Close();
}
public int IsNumeric(string str)
{
int i;
if (str != null && Regex.IsMatch(str, @"^\d+$"))
i = int.Parse(str);
else
i = -1;
return i;
}
private void DelForm_Load(object sender, EventArgs e)
{
PdfInfoLB.Text = "本文档共" + totalPage.ToString() + "页,准备删除";
DelTxt.Text = deletePage.ToString();
}
private void DelTxt_KeyPress(object sender, KeyPressEventArgs e)
{
if (!Char.IsNumber(e.KeyChar)&&e.KeyChar!='\b'&&e.KeyChar!=','&&e.KeyChar!='-')
{
e.Handled = true;
}
}
}
}
敬请期待下一篇:
源代码下载
相关连接
更多推荐
所有评论(0)