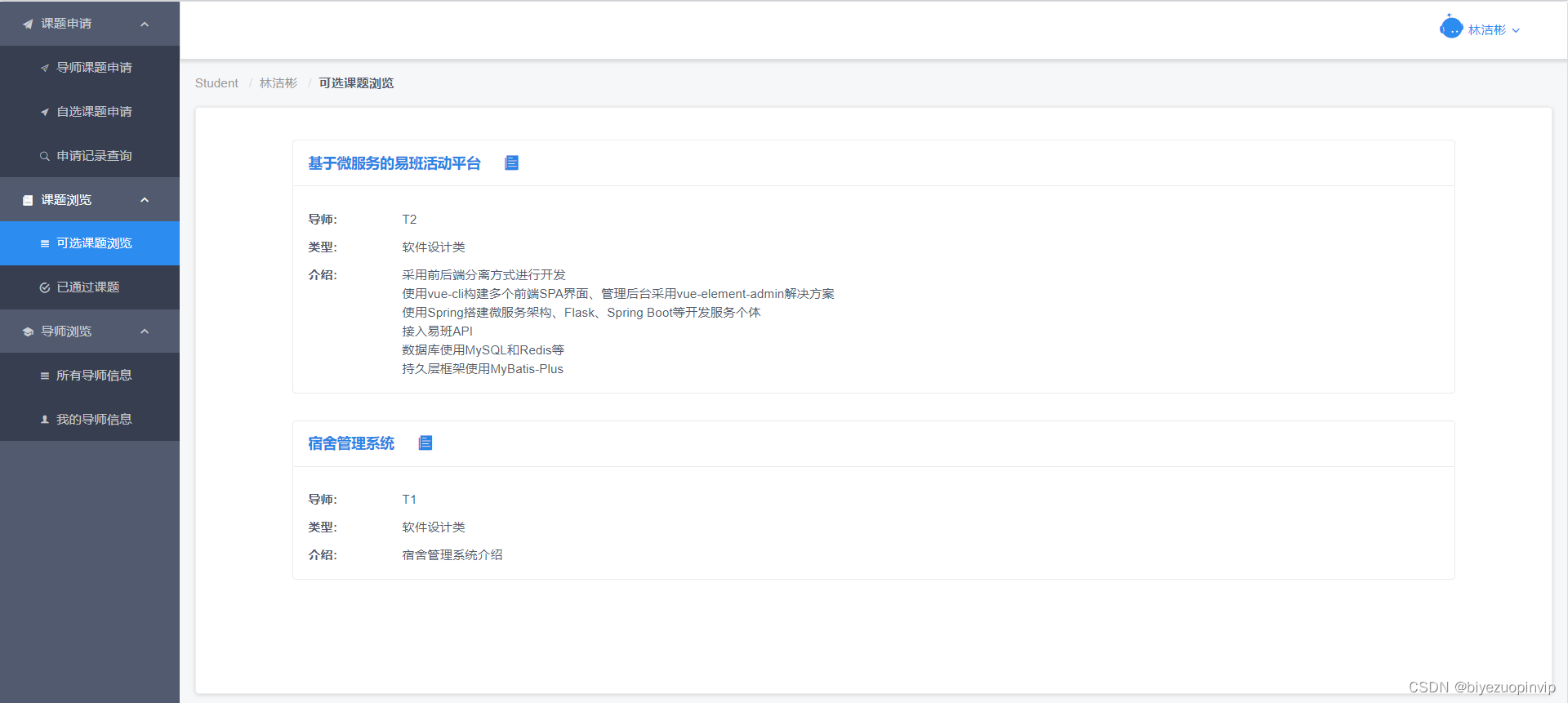
基于Spring Boot+Vue的毕业设计选题管理系统设计与实现
(3)学生:需要查询可以选择的课题和可以选择的导师,可以申请课题,考虑到有学生提出自己课题的情况,学生也可以根据导师对自选课题的要求向指定导师申请自己的课题,同时也可以查询自己的申请记录以及申请情况,学生也可以查询自己通过的课题的信息和导师的信息。(2)导师:需要能够发布课题,并且可以对课题进行管理,导师需要处理学生对课题的申请,在处理后能够查看申请的处理记录,导师对于已通过的学生,可以查看学生的
目录
1 开发环境与开发工具 4
1.1 开发环境 4
1.2 开发工具 4
2 开发日志 5
3 系统分析 6
3.1 需求分析 6
3.2 功能分析 6
4 系统设计 7
4.1 数据库概念结构设计 7
4.2 数据库逻辑结构设计 8
4.3 数据库的实施 11
5 系统实现 13
5.1 系统实现的技术方案 13
5.2 登陆功能的实现 13
5.3 管理员功能实现 14
5.3.1 添加学生用户 14
5.3.2 添加导师用户 16
5.3.3 从文件导入用户 17
5.3.4 用户查询页面 18
5.3.5 用户信息修改 19
5.3.5 删除用户 19
5.4 导师功能模块 20
5.4.1 修改信息 20
5.4.2 添加课题 22
5.4.3 课题管理 22
5.4.4 申请处理 24
5.4.5 申请处理记录 26
5.4.6 指导学生查询 26
5.5 学生功能 27
5.5.1 导师课题申请 27
5.5.2 学生课题申请 28
5.5.3 申请记录查询 28
5.5.4 可选课题浏览 29
5.5.4 已通过课题浏览 29
5.5.5 可选导师浏览 30
5.5.6 学生的导师信息 30
5.6 总结 32
6 参考文献 33
1 开发环境与开发工具
1.1 开发环境
(1)开发语言:Java 13、Python 3.7、Node.js 12.13.1
(2)开发设备系统:Windows 10
(3)数据库系统软件:MySQL 8.0、Redis 3.2
(4)Java Web 框架:Spring Boot 2.3.0
(5)Java 数据库框架:ORM持久层框架 MyBatis-Plus 3.3.2
(6)Python Web 框架:Flask 1.1.1
(7)Python 数据库框架:基于PyMySQL的Web-MySQL
(8)前端框架:基于webpack的Vue.js脚手架 Vue-CLI
(9)UI库:基于Vue.js的View UI
1.2 开发工具
(1)前端SPA页面开发工具:Visual Studio Code
(2)Java后端开发工具:IntelliJ IDEA Ultimate
(3)Java依赖管理器:Maven 3.6
(4)Python后端开发工具:PyCharm Professional
(5)数据库工具:MySQL Workbench 8.0 CE
(6)项目版本管理工具:Git、GitHub、GitHub Desktop
(7)URL接口测试工具:Postman
3 系统分析
3.1 需求分析
毕业设计选题系统是一个用于毕业学生进行毕业设计选题和导师管理课题的系统,作为一个高校使用的系统,有着许多用户的使用,对于不同用户,使用的操作系统可能不同,在平台拥有的权限也不同,本文转载自http://www.biyezuopin.vip/onews.asp?id=15222因此本系统设计了3种用户,分别是系统管理员、导师、毕业学生。
不同用户的功能如下:
(1)系统管理员:能够添加用户和修改用户信息。
(2)导师:需要能够发布课题,并且可以对课题进行管理,导师需要处理学生对课题的申请,在处理后能够查看申请的处理记录,导师对于已通过的学生,可以查看学生的信息。
(3)学生:需要查询可以选择的课题和可以选择的导师,可以申请课题,考虑到有学生提出自己课题的情况,学生也可以根据导师对自选课题的要求向指定导师申请自己的课题,同时也可以查询自己的申请记录以及申请情况,学生也可以查询自己通过的课题的信息和导师的信息。
package com.arukione.curriculum_design.service;
import com.arukione.curriculum_design.exception.PermissionException;
import com.arukione.curriculum_design.mapper.ApplicationMapper;
import com.arukione.curriculum_design.mapper.TeacherMapper;
import com.arukione.curriculum_design.mapper.TopicInfoMapper;
import com.arukione.curriculum_design.mapper.TopicTypeMapper;
import com.arukione.curriculum_design.model.DTO.Request.TopicInfo;
import com.arukione.curriculum_design.model.DTO.Response.Response;
import com.arukione.curriculum_design.model.DTO.Response.SelectableTeacherResponse;
import com.arukione.curriculum_design.model.DTO.Response.SelectableTopicResponse;
import com.arukione.curriculum_design.model.DTO.Response.UserInfoResponse;
import com.arukione.curriculum_design.model.VO.SelectableTopicInfo;
import com.arukione.curriculum_design.model.entity.*;
import com.arukione.curriculum_design.utils.Generator;
import com.arukione.curriculum_design.utils.HTTPStatus;
import com.arukione.curriculum_design.utils.Message;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Service
public class StudentService {
final UserService userService;
final TopicInfoMapper topicInfoMapper;
final TeacherMapper teacherMapper;
final ApplicationMapper applicationMapper;
final TopicTypeMapper topicTypeMapper;
@Autowired
public StudentService(UserService userService, TopicInfoMapper topicInfoMapper, TeacherMapper teacherMapper, ApplicationMapper applicationMapper, TopicTypeMapper topicTypeMapper) {
this.userService = userService;
this.topicInfoMapper = topicInfoMapper;
this.teacherMapper = teacherMapper;
this.applicationMapper = applicationMapper;
this.topicTypeMapper = topicTypeMapper;
}
public SelectableTopicResponse getAllTopic(String accessToken) {
try {
Student student = (Student) userService.permission(accessToken, "Student");
String profId = student.getProfessionId();
ArrayList<SelectableTopicInfo> selectableTopicInfos = topicInfoMapper.getSelectableTopicInfo(profId);
return new SelectableTopicResponse(HTTPStatus.OK, selectableTopicInfos);
} catch (PermissionException permissionException) {
return new SelectableTopicResponse(HTTPStatus.NotAllowed, Message.USER_PERMISSION_ERROR);
} catch (NullPointerException npe) {
return new SelectableTopicResponse(HTTPStatus.Unauthorized, Message.NO_LOGIN_STATUS);
}
}
public SelectableTeacherResponse getAllTeacher(String accessToken) {
try {
Student student = (Student) userService.permission(accessToken, "Student");
String profId = student.getProfessionId();
QueryWrapper<Teacher> teacherQueryWrapper = new QueryWrapper<>();
teacherQueryWrapper.eq("GuideProfID", profId);
List<Teacher> teachers = teacherMapper.selectList(teacherQueryWrapper);
return new SelectableTeacherResponse(HTTPStatus.OK, teachers);
} catch (PermissionException permissionException) {
return new SelectableTeacherResponse(HTTPStatus.NotAllowed, Message.USER_PERMISSION_ERROR);
} catch (NullPointerException npe) {
return new SelectableTeacherResponse(HTTPStatus.Unauthorized, Message.NO_LOGIN_STATUS);
}
}
public Response addApply(String accessToken, String topicId) {
Response response = canApply(accessToken);
if(response.getStatus()!=200) return response;
String sid = response.getMessage();
try {
return getApplyResult(sid, topicId);
}catch (Exception e){
if(e.getMessage().contains("for key 'PRIMARY'"))
return new Response(HTTPStatus.Failed, "不能再次申请该课题");
else{
e.printStackTrace();
return new Response(HTTPStatus.Failed, e.getMessage());
}
}
}
public Response addApply(String accessToken, String tid, TopicInfo topicInfo) {
Response response = canApply(accessToken);
if(response.getStatus()!=200) return response;
String sid = response.getMessage();
String topicId = Generator.generateTopicID();
Topic topic = Topic.builder()
.topicId(topicId)
.topicName(topicInfo.getTopicName())
.introduction(topicInfo.getIntroduction())
.tid(tid)
.sid(sid)
.typeId(topicInfo.getTypeId())
.source("1")
.build();
try {
if (topicInfoMapper.insert(topic) != 1) return new Response(HTTPStatus.Failed, Message.DB_NO_DATA);
return getApplyResult(sid, topicId);
} catch (Exception e){
if(e.getMessage().contains("for key 'PRIMARY'"))
return new Response(HTTPStatus.Failed, "不能再次申请该课题");
else{
e.printStackTrace();
return new Response(HTTPStatus.Failed, e.getMessage());
}
}
}
private Response canApply(String accessToken) {
try {
Student student = (Student) userService.permission(accessToken, "Student");
QueryWrapper<Application> applicationQueryWrapper = new QueryWrapper<>();
applicationQueryWrapper.eq("SID", student.getSid()).eq("Status", "1");
if (applicationMapper.selectOne(applicationQueryWrapper) != null)
return new Response(HTTPStatus.Failed, "你已有课题,无法再申请");
return new Response(HTTPStatus.OK, student.getSid());
} catch (PermissionException permissionException) {
return new Response(HTTPStatus.NotAllowed, Message.USER_PERMISSION_ERROR);
} catch (NullPointerException npe) {
return new Response(HTTPStatus.Unauthorized, Message.NO_LOGIN_STATUS);
}
}
private Response getApplyResult(String sid, String topicId) {
Application application = new Application();
application.setSid(sid);
application.setTopicId(topicId);
application.setApplyTime(Generator.getNowTime());
application.setStatus("0");
return userService.opsResult(applicationMapper.insert(application));
}
//获取我的导师信息
public UserInfoResponse getMyTeacher(String accessToken) {
try {
Student stu = (Student) userService.permission(accessToken, "Student");
//获取已通过的申请记录
QueryWrapper<Application> applicationQueryWrapper = new QueryWrapper<>();
applicationQueryWrapper.eq("SID", stu.getSid()).eq("Status", "1");
Application application = applicationMapper.selectOne(applicationQueryWrapper);
if(application == null) return new UserInfoResponse(HTTPStatus.Failed, Message.DB_NO_DATA);
Topic topic = topicInfoMapper.selectById(application.getTopicId());
return new UserInfoResponse(200, teacherMapper.selectById(topic.getTid()));
} catch (PermissionException permissionException) {
return new UserInfoResponse(HTTPStatus.NotAllowed, Message.USER_PERMISSION_ERROR);
} catch (NullPointerException npe) {
return new UserInfoResponse(HTTPStatus.Unauthorized, Message.NO_LOGIN_STATUS);
}
}
//获得已通过的课题信息
public Map<String, Object> getAllowTopic(String accessToken) {
Map<String, Object> response = new HashMap<>();
try {
Student stu = (Student) userService.permission(accessToken, "Student");
QueryWrapper<Topic> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("SID", stu.getSid()).eq("Source", 0);
Topic topic = topicInfoMapper.selectOne(queryWrapper);
if(topic==null){
QueryWrapper<Application> applicationQueryWrapper = new QueryWrapper<>();
applicationQueryWrapper.eq("SID", stu.getSid()).eq("Status", "1");
Application application = applicationMapper.selectOne(applicationQueryWrapper);
System.out.println(application);
if (application == null) {
response.put("status", HTTPStatus.Failed);
response.put("message","没有获取到数据");
return response;
}
topic = topicInfoMapper.selectById(application.getTopicId());
}
TopicType type = topicTypeMapper.getTopicType(topic.getTypeId());
response.put("status", HTTPStatus.OK);
response.put("topic",topic);
response.put("type",type);
Teacher teacher = teacherMapper.selectById(topic.getTid());
response.put("name", teacher.getName());
return response;
} catch (PermissionException permissionException) {
response.put("status", HTTPStatus.NotAllowed);
response.put("message",Message.USER_PERMISSION_ERROR);
return response;
} catch (NullPointerException npe) {
response.put("status", HTTPStatus.Unauthorized);
response.put("message",Message.NO_LOGIN_STATUS);
return response;
}
}
public Map<String, Object> getApplicationInfo(String accessToken) {
Map<String, Object> response = new HashMap<>();
try {
Student stu = (Student) userService.permission(accessToken, "Student");
ArrayList<Application> applies = applicationMapper.getApplicationsOfSID(stu.getSid());
if (applies == null || applies.size()==0) {
response.put("status", HTTPStatus.Failed);
response.put("message","没有获取到数据");
return response;
}
ArrayList<Object> mapData =new ArrayList<>();
for (Application apply : applies) {
Map<String, Object> data = new HashMap<>();
Topic topic = topicInfoMapper.selectById(apply.getTopicId());
data.put("topicInfo", topic);
data.put("teacherName", teacherMapper.selectById(topic.getTid()).getName());
data.put("applyInfo", apply);
mapData.add(data);
}
response.put("status", HTTPStatus.OK);
response.put("applyRecord", mapData);
return response;
} catch (PermissionException permissionException) {
response.put("status", HTTPStatus.NotAllowed);
response.put("message",Message.USER_PERMISSION_ERROR);
return response;
} catch (NullPointerException npe) {
response.put("status", HTTPStatus.Unauthorized);
response.put("message",Message.NO_LOGIN_STATUS);
return response;
}
}
}
更多推荐
所有评论(0)