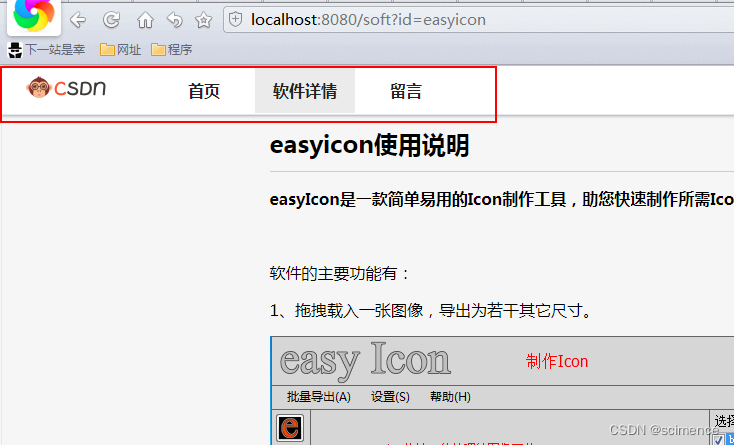
VUE 菜单栏、导航栏 实现
VUE 菜单栏、导航栏 的实现
·
1、index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>sci_soft</title>
<style>
body{background:url(""); background-color:#F6F6F6; display: block; margin: 0px;}
</style>
</head>
<body>
<div id="app0"></div>
<!-- built files will be auto injected -->
</body>
</html>
2、main.js
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
import axios from 'axios'
Vue.prototype.$http= axios
// axios.defaults.baseURL="http://localhost:44923";
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app0',
router,
components: { App },
template: '<App/>'
})
3、App.vue
<template>
<div id="app0">
<pannel-header></pannel-header>
<!-- <hello-world></hello-world> -->
<!-- <pannel-content v-bind:soft='"easyicon"'></pannel-content> -->
<router-view />
<!-- <router-link :to="{name:'soft', params: { id: 'easyicon' }}">跳转</router-link>
<router-link :to="{path:'/soft', query: { id: 'easyicon' }}">跳转</router-link> -->
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
import PannelHeader from './components/PannelHeader.vue'
import PannelContent from './components/PannelContent.vue'
export default {
components: { HelloWorld, PannelHeader, PannelContent },
name: 'App',
data()
{
return {
Height: window.innerHeight,
Width: window.innerWidth
}
}
}
</script>
<style>
.container {
/* width: 100%; */
/* max-width: 1366px!important; */
min-width: 900px;
padding: 0;
border: 1px solid #e3e9ed;
border-top: 0;
margin: 1px;
margin-top: 1px;
max-width: calc(100vw - 4px);
max-height: calc(100vh - 48px - 5px);
overflow:auto;
}
/* 为div添加边框属性 */
.border{
min-width: 10px;
min-height: 10px;
border:solid;
border-width: 1px;
border-color: gray;
background: white;
}
/* 为div添加边框阴影属性 */
.shadow{
/* width: 100%;
height: 100%; */
min-width: 10px;
min-height: 10px;
/* border:dashed; */
/* border:solid; */
border-radius: 10px;
border-width: 1px;
border-color: gray;
background: white;
/* background: yellow; */
/* background: rgb(238, 238, 238); */
/* box-shadow: 0 0 3px 0px #000 inset; */
box-shadow: 0 0 5px 0px rgb(126, 125, 125);
}
</style>
4、router/index.js
import Vue from 'vue'
import Router from 'vue-router'
import HelloWorld from '@/components/HelloWorld'
import PannelContent from '@/components/PannelContent.vue'
Vue.use(Router)
export default new Router({
mode: 'history',
routes: [
{
path: '/',
redirect:'/home'
},
{
path: '/home',
name: 'HelloWorld',
component: HelloWorld
},
{
path: '/soft',
name: '软件详情',
component: PannelContent
},
// {
// path: '/soft/:id',
// name: '软件详情',
// component: PannelContent
// },
]
})
5、PannelHeader.vue
<template>
<div class="container">
<div class="logo">
<a href="http://scimence.cn">
<img src="../assets/logo.png">
</a>
</div>
<div class = "menus">
<ul>
<li v-for="menu in menus">
<router-link v-bind:to="{ path: menu.path}" active-class="select">{{menu.tittle}}</router-link>
</li>
</ul>
</div>
</div>
</template>
<script>
export default {
name: 'PannelHeader',
data () {
return {
msg: 'Welcome to Your Vue.js App',
menus:
[
{tittle: "首页", path:"home"},
{tittle: "软件详情", path:"soft"},
{tittle: "留言", path:"message"}
]
}
},
methods: {
// menuClick(path){
// menus.forEach(element => {
// element.tittle = "选中";
// if(element.path == path) element.selected = true;
// else element.selected = false;
// });
// }
}
}
</script>
<style scoped>
.container {
width: 100%;
height: 48px;
line-height: 48px;
background-color: white;
box-shadow: 0 0 5px 0px rgb(126, 125, 125);
}
.logo {
float:left;
max-width: 80px;
height: 100%;
padding-left: 24px;
}
.logo img {
display: block;
width: 80px;
min-width: 80px;
height: 44px;
/* margin-top: calc((48px - 44px)/ 2); */
}
.menus {
float: left;
padding-left: 48px;
/* float:right; */
/* padding-right: 48px; */
}
.menus ul
{
list-style-type:none;
margin: 0px;
padding: 0px;
}
.menus li
{
float:left;
border-right: 1px solid white;
height: 46px;
margin-top: 1px;
}
.menus a:link,a:visited
{
display:block;
font-weight:bold;
color:#222222;
width:100px;
height: 100%;
text-align:center;
margin: 0px;
text-decoration:none;
/* text-transform:uppercase; */
}
.menus a:active
{
color:red;
}
.menus a:hover
{
background-color: #F6F6F6;
}
.select{
background-color: #ebebeb;
}
/* .menus router-link{
display:block;
font-weight:bold;
color:#222222;
width:100px;
height: 100%;
text-align:center;
margin: 0px;
text-decoration:none;
}
.menus router-link:hover
{
background-color: #F6F6F6;
} */
</style>
6、PanneContent.vue
<template>
<div class="container">
<div id="page-detail" v-if="visible">
<div class='title'>{{tittle}}</div>
<div class='content' v-html="note">
</div>
</div>
<a class="download" target="_blank" v-bind:href='url'>立即下载</a>
</div>
</template>
<script>
export default {
name: 'PanneContent',
props: {
soft: String,
},
data () {
return {
visible: true,
msg: 'Welcome to Your Vue.js App',
menus:
[
{tittle: "首页", path:""},
{tittle: "软件详情", path:""},
{tittle: "留言", path:""}
],
tittle: "",
note: "note信息",
url: ""
}
},
mounted()
{
// this.soft = this.$route.params.soft;
},
created()
{
this.soft = this.$route.query.id;
// this.$router.push({ path: '/soft', query: { id: 'easyicon' }});
// this.soft = this.$route.params.id;
// this.$router.push({ name: '/soft', params: { id: 'easyicon' }});
var that = this;
// this.$http.get('http://localhost:44923/pages/NoteInfo.aspx?soft=easyicon')
this.$http.get('/pages/NoteInfo.aspx', {params:{soft: this.soft}})
.then((response)=>{
// console.log("log信息");
// console.log(response);
that.tittle = response.data.tittle;
that.url = response.data.url;
that.note = that.base64ToString(response.data.note);
that.visible = true;
})
.catch((response)=>{
console.log(response);
this.note = "异常信息";
that.visible = false;
})
},
methods: {
// string转为base64
stringToBase64(str) {
return new Buffer.from(str).toString("base64");
},
// base64转字符串
base64ToString(b64) {
return new Buffer.from(b64, "base64").toString();
},
}
}
</script>
<style scoped>
#page-detail {
width: 60%;
min-width: 810px;
margin: 0 auto;
}
#page-detail .title {
font-weight: 700;
font-size: 24px;
padding: 12px 0;
border-bottom: 1px solid #ccc;
}
#page-detail .content {
margin-top: 15px;
}
.content p {
word-wrap: break-word;
}
a:hover {
background-color: rgb(233,104,107);
}
.download {
position: fixed;
left: 80%;
top: 75%;
float: right;
background: rgb(255,105,143);
color: white;
border: 2px solid gray;
padding: 15px;
margin: 20px;
font-family: SimHei;
font-size: 2em;
text-size: 40px;
text-align: center;
text-decoration: none;
}
</style>
7、HelloWorld.vue
<template>
<div class="hello content">
<h1>{{ msg }}</h1>
<h2>Essential Links</h2>
<ul>
<li>
<a
href="https://vuejs.org"
target="_blank"
>
Core Docs
</a>
</li>
<li>
<a
href="https://forum.vuejs.org"
target="_blank"
>
Forum
</a>
</li>
<li>
<a
href="https://chat.vuejs.org"
target="_blank"
>
Community Chat
</a>
</li>
<li>
<a
href="https://twitter.com/vuejs"
target="_blank"
>
Twitter
</a>
</li>
<br>
<li>
<a
href="http://vuejs-templates.github.io/webpack/"
target="_blank"
>
Docs for This Template
</a>
</li>
</ul>
<h2>Ecosystem</h2>
<ul>
<li>
<a
href="http://router.vuejs.org/"
target="_blank"
>
vue-router
</a>
</li>
<li>
<a
href="http://vuex.vuejs.org/"
target="_blank"
>
vuex
</a>
</li>
<li>
<a
href="http://vue-loader.vuejs.org/"
target="_blank"
>
vue-loader
</a>
</li>
<li>
<a
href="https://github.com/vuejs/awesome-vue"
target="_blank"
>
awesome-vue
</a>
</li>
</ul>
</div>
</template>
<script>
export default {
name: 'HelloWorld',
data () {
return {
msg: 'Welcome to Your Vue.js App'
}
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
.content {
width: 60%;
margin: 0 auto;
}
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
附录:proxy配置
config/index.js -> proxyTable
'use strict'
// Template version: 1.3.1
// see http://vuejs-templates.github.io/webpack for documentation.
const path = require('path')
module.exports = {
dev: {
// Paths
assetsSubDirectory: 'static',
assetsPublicPath: '/',
proxyTable: {
// http://localhost:44923/pages/NoteInfo.aspx
'/pages': {
target: 'http://localhost:44923',
ws: true,
changeOrgin: true,
}
},
// Various Dev Server settings
host: 'localhost', // can be overwritten by process.env.HOST
port: 8080, // can be overwritten by process.env.PORT, if port is in use, a free one will be determined
autoOpenBrowser: false,
errorOverlay: true,
notifyOnErrors: true,
poll: false, // https://webpack.js.org/configuration/dev-server/#devserver-watchoptions-
/**
* Source Maps
*/
// https://webpack.js.org/configuration/devtool/#development
devtool: 'cheap-module-eval-source-map',
// If you have problems debugging vue-files in devtools,
// set this to false - it *may* help
// https://vue-loader.vuejs.org/en/options.html#cachebusting
cacheBusting: true,
cssSourceMap: true
},
build: {
// Template for index.html
index: path.resolve(__dirname, '../dist/index.html'),
// Paths
assetsRoot: path.resolve(__dirname, '../dist'),
assetsSubDirectory: 'static',
assetsPublicPath: '/',
/**
* Source Maps
*/
productionSourceMap: true,
// https://webpack.js.org/configuration/devtool/#production
devtool: '#source-map',
// Gzip off by default as many popular static hosts such as
// Surge or Netlify already gzip all static assets for you.
// Before setting to `true`, make sure to:
// npm install --save-dev compression-webpack-plugin
productionGzip: false,
productionGzipExtensions: ['js', 'css'],
// Run the build command with an extra argument to
// View the bundle analyzer report after build finishes:
// `npm run build --report`
// Set to `true` or `false` to always turn it on or off
bundleAnalyzerReport: process.env.npm_config_report
}
}
更多推荐
所有评论(0)