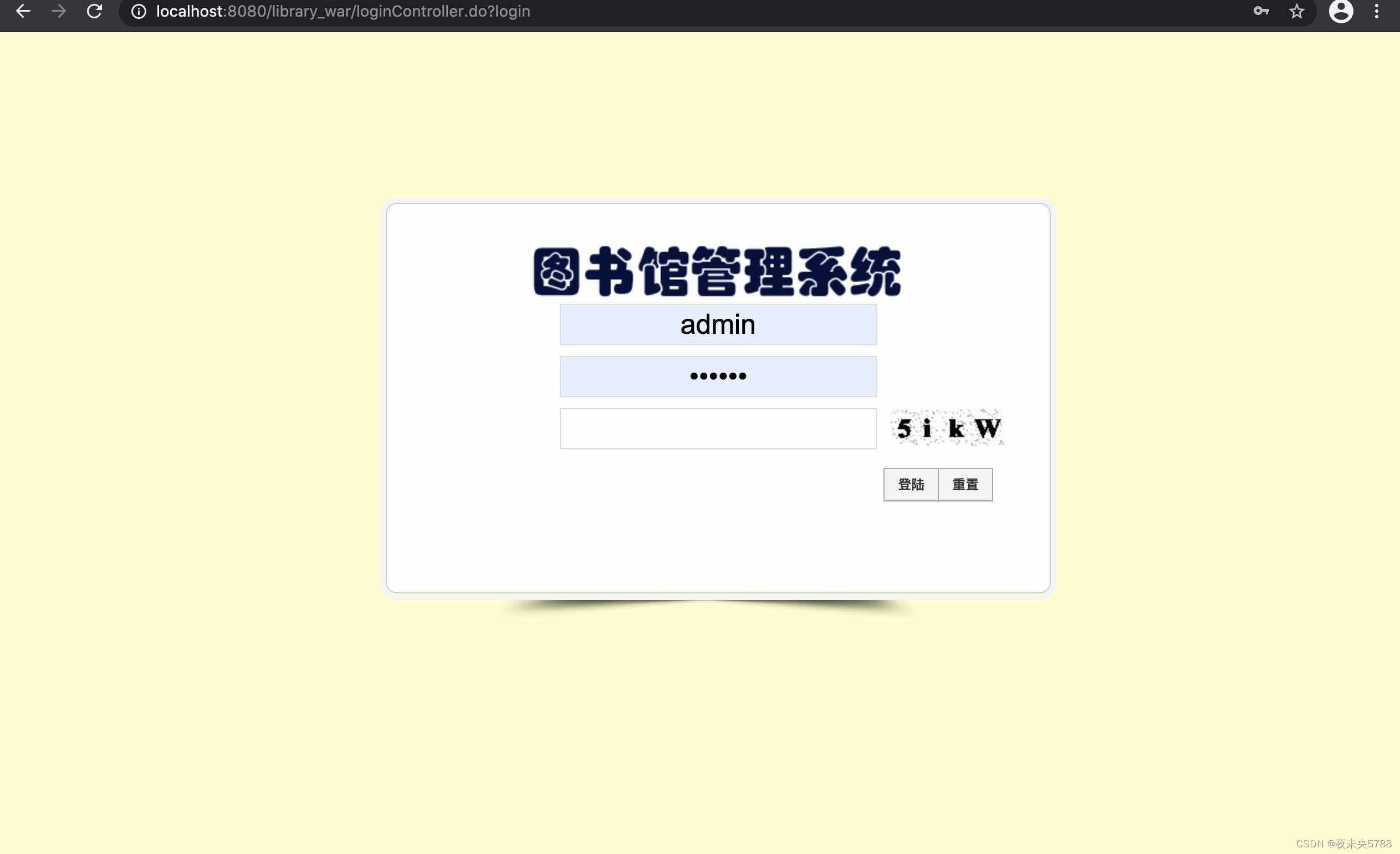
java项目-图书馆管理系统源码
本毕业设计运用了使用技术:spring mvc+spring+hibernate,数据库使用了当前较为流行的Mysql5.7。根据本校图书馆的工作流程与实际的需求和特色,本系统需满足以下几个方面的要求:1.对新书进行登记入库、下架管理;2.对借书读者信息提供维护功能;3.提供图书借书、续借、还书功能;4.提供图书超期未还自动扣款功能;5.提供数据导入功能。共22张表1.运行环境:最好是java j
作者主页:夜未央5788
简介:Java领域优质创作者、Java项目、学习资料、技术互助
文末获取源码
项目介绍
本毕业设计运用了使用技术:spring mvc+spring+hibernate,数据库使用了当前较为流行的Mysql5.7。根据本校图书馆的工作流程与实际的需求和特色,本系统需满足以下几个方面的要求:
1.对新书进行登记入库、下架管理;
2.对借书读者信息提供维护功能;
3.提供图书借书、续借、还书功能;
4.提供图书超期未还自动扣款功能;
5.提供数据导入功能。
共22张表
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可
4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
5.是否Maven项目: 是;查看源码目录中是否包含pom.xml;若包含,则为maven项目,否则为非maven项目
6.数据库:MySql 5.7版本;
技术栈
1. spring mvc+spring+hibernate
2. JSP+easyUI+BootStrap+JQuery
使用说明
1. 使用Navicat或者其它工具,在mysql中创建对应名称的数据库,并导入项目的sql文件;
2. 将项目中dbconfig.properties配置文件中的数据库配置改为自己的配置
3. 使用IDEA/Eclipse/MyEclipse导入项目,Eclipse/MyEclipse导入时,若为maven项目请选择maven;若为maven项目,导入成功后请执行maven clean;maven install命令,配置tomcat,然后运行;
4. 运行项目,输入localhost:8080/xxx 登录
运行截图
代码相关
图书管理控制器
@Controller
@RequestMapping("/tBBookController")
public class TBBookController extends BaseController {
/**
* Logger for this class
*/
private static final Logger logger = Logger.getLogger(TBBookController.class);
@Autowired
private TBBookServiceI tBBookService;
@Autowired
private SystemService systemService;
/**
* 图书表列表 页面跳转
*
* @return
*/
@RequestMapping(params = "tBBook")
public ModelAndView tBBook(HttpServletRequest request) {
return new ModelAndView("buss/book/tBBookList");
}
/**
* 出版社表列表 页面跳转
*
* @return
*/
@RequestMapping(params = "press")
public ModelAndView press(HttpServletRequest request) {
return new ModelAndView("buss/book/press");
}
/**
* 出版社显示列表
*
* @param request
* @param response
* @param dataGrid
*/
@RequestMapping(params = "datagridPress")
public void datagridPress(TBPressEntity tbPress, HttpServletRequest request, HttpServletResponse response, DataGrid dataGrid) {
CriteriaQuery cq = new CriteriaQuery(TBPressEntity.class, dataGrid);
com.bjpowernode.core.extend.hqlsearch.HqlGenerateUtil.installHql(cq, tbPress, request.getParameterMap());
this.systemService.getDataGridReturn(cq, true);
TagUtil.datagrid(response, dataGrid);
}
/**
* easyui AJAX请求数据
*
* @param request
* @param response
* @param dataGrid
* @param user
*/
@RequestMapping(params = "datagrid")
public void datagrid(TBBookEntity tBBook,HttpServletRequest request, HttpServletResponse response, DataGrid dataGrid) {
CriteriaQuery cq = new CriteriaQuery(TBBookEntity.class, dataGrid);
//查询条件组装器
com.bjpowernode.core.extend.hqlsearch.HqlGenerateUtil.installHql(cq, tBBook, request.getParameterMap());
try{
//自定义追加查询条件
}catch (Exception e) {
throw new BusinessException(e.getMessage());
}
cq.add();
this.tBBookService.getDataGridReturn(cq, true);
TagUtil.datagrid(response, dataGrid);
}
/**
* 删除图书表
*
* @return
*/
@RequestMapping(params = "doDel")
@ResponseBody
public AjaxJson doDel(TBBookEntity tBBook, HttpServletRequest request) {
AjaxJson j = new AjaxJson();
tBBook = systemService.getEntity(TBBookEntity.class, tBBook.getId());
String message = "图书表删除成功";
try{
tBBookService.delete(tBBook);
systemService.addLog(message, Globals.Log_Type_DEL, Globals.Log_Leavel_INFO);
}catch(Exception e){
e.printStackTrace();
message = "图书表删除失败";
throw new BusinessException(e.getMessage());
}
j.setMsg(message);
return j;
}
/**
* 批量删除图书表
*
* @return
*/
@RequestMapping(params = "doBatchDel")
@ResponseBody
public AjaxJson doBatchDel(String ids,HttpServletRequest request){
AjaxJson j = new AjaxJson();
String message = "图书表删除成功";
try{
for(String id:ids.split(",")){
TBBookEntity tBBook = systemService.getEntity(TBBookEntity.class,
id
);
tBBookService.delete(tBBook);
systemService.addLog(message, Globals.Log_Type_DEL, Globals.Log_Leavel_INFO);
}
}catch(Exception e){
e.printStackTrace();
message = "图书表删除失败";
throw new BusinessException(e.getMessage());
}
j.setMsg(message);
return j;
}
/**
* 添加图书表
*
* @param ids
* @return
*/
@RequestMapping(params = "doAdd")
@ResponseBody
public AjaxJson doAdd(TBBookEntity tBBook, HttpServletRequest request) {
AjaxJson j = new AjaxJson();
String message = "图书表添加成功";
try{
tBBook.setStatus(Globals.BOOK_RETURN);
tBBookService.save(tBBook);
systemService.addLog(message, Globals.Log_Type_INSERT, Globals.Log_Leavel_INFO);
}catch(Exception e){
e.printStackTrace();
message = "图书表添加失败";
throw new BusinessException(e.getMessage());
}
j.setMsg(message);
return j;
}
/**
* 更新图书表
*
* @param ids
* @return
*/
@RequestMapping(params = "doUpdate")
@ResponseBody
public AjaxJson doUpdate(TBBookEntity tBBook, HttpServletRequest request) {
AjaxJson j = new AjaxJson();
String message = "图书表更新成功";
TBBookEntity t = tBBookService.get(TBBookEntity.class, tBBook.getId());
try {
MyBeanUtils.copyBeanNotNull2Bean(tBBook, t);
tBBookService.saveOrUpdate(t);
systemService.addLog(message, Globals.Log_Type_UPDATE, Globals.Log_Leavel_INFO);
} catch (Exception e) {
e.printStackTrace();
message = "图书表更新失败";
throw new BusinessException(e.getMessage());
}
j.setMsg(message);
return j;
}
/**
* 图书表新增页面跳转
*
* @return
*/
@RequestMapping(params = "goAdd")
public ModelAndView goAdd(TBBookEntity tBBook, HttpServletRequest req) {
if (StringUtil.isNotEmpty(tBBook.getId())) {
tBBook = tBBookService.getEntity(TBBookEntity.class, tBBook.getId());
req.setAttribute("tBBookPage", tBBook);
}
return new ModelAndView("buss/book/tBBook-add");
}
/**
* 图书表编辑页面跳转
*
* @return
*/
@RequestMapping(params = "goUpdate")
public ModelAndView goUpdate(TBBookEntity tBBook, HttpServletRequest req) {
if (StringUtil.isNotEmpty(tBBook.getId())) {
tBBook = tBBookService.getEntity(TBBookEntity.class, tBBook.getId());
req.setAttribute("tBBookPage", tBBook);
}
return new ModelAndView("buss/book/tBBook-update");
}
/**
* 统计集合页面
*
* @return
*/
@RequestMapping(params = "bookStatisticTabs")
public ModelAndView bookStatisticTabs(HttpServletRequest request) {
return new ModelAndView("buss/book/tBBookReport");
}
/**
* 报表数据生成
*
* @return
*/
@RequestMapping(params = "bookCount")
@ResponseBody
public List<Highchart> bookCount(HttpServletRequest request,String reportType, HttpServletResponse response) {
List<Highchart> list = new ArrayList<Highchart>();
Highchart hc = new Highchart();
StringBuffer sb = new StringBuffer();
sb.append("SELECT booktype ,count(booktype) FROM TBBookEntity group by booktype");
List bookTypeList = systemService.findByQueryString(sb.toString());
Long count = systemService.getCountForJdbc("SELECT COUNT(1) FROM T_B_book WHERE 1=1");
List lt = new ArrayList();
hc = new Highchart();
hc.setName("图书种类统计");
hc.setType(reportType);
Map<String, Object> map;
String hql = "from TSType where typegroupid='" + Globals.TYPEGROUP_ID+ "'";
List<TSType> typeList = systemService.findByQueryString(hql);
Map<String, String> typeMap = new HashMap<String, String>();
if(typeList.size() > 0){
for(TSType type : typeList){
typeMap.put(type.getTypecode(), type.getTypename());
}
}
if (bookTypeList.size() > 0) {
for (Object object : bookTypeList) {
map = new HashMap<String, Object>();
Object[] obj = (Object[]) object;
map.put("name", typeMap.get(obj[0]));
map.put("y", obj[1]);
Long groupCount = (Long) obj[1];
Double percentage = 0.0;
if (count != null && count.intValue() != 0) {
percentage = new Double(groupCount)/count;
}
map.put("percentage", percentage*100);
lt.add(map);
}
}
hc.setData(lt);
list.add(hc);
return list;
}
/**
* 报表打印
* @param request
* @param response
* @throws IOException
*/
@RequestMapping(params = "export")
public void export(HttpServletRequest request, HttpServletResponse response)
throws IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
String type = request.getParameter("type");
String svg = request.getParameter("svg");
String filename = request.getParameter("filename");
filename = filename == null ? "chart" : filename;
ServletOutputStream out = response.getOutputStream();
try {
if (null != type && null != svg) {
svg = svg.replaceAll(":rect", "rect");
String ext = "";
Transcoder t = null;
if (type.equals("image/png")) {
ext = "png";
t = new PNGTranscoder();
} else if (type.equals("image/jpeg")) {
ext = "jpg";
t = new JPEGTranscoder();
} else if (type.equals("application/pdf")) {
ext = "pdf";
t = (Transcoder) new PDFTranscoder();
} else if (type.equals("image/svg+xml"))
ext = "svg";
response.addHeader("Content-Disposition",
"attachment; filename=" + new String(filename.getBytes("GBK"),"ISO-8859-1") + "." + ext);
response.addHeader("Content-Type", type);
if (null != t) {
TranscoderInput input = new TranscoderInput(
new StringReader(svg));
TranscoderOutput output = new TranscoderOutput(out);
try {
t.transcode(input, output);
} catch (TranscoderException e) {
out
.print("Problem transcoding stream. See the web logs for more details.");
e.printStackTrace();
}
} else if (ext.equals("svg")) {
// out.print(svg);
OutputStreamWriter writer = new OutputStreamWriter(out,
"UTF-8");
writer.append(svg);
writer.close();
} else
out.print("Invalid type: " + type);
} else {
response.addHeader("Content-Type", "text/html");
out
.println("Usage:\n\tParameter [svg]: The DOM Element to be converted."
+ "\n\tParameter [type]: The destination MIME type for the elment to be transcoded.");
}
} finally {
if (out != null) {
out.flush();
out.close();
}
}
}
/**
* 图书导入
*
* @return
*/
@RequestMapping(params = "upload")
public ModelAndView upload(HttpServletRequest req) {
return new ModelAndView("buss/book/bookUpload");
}
/**
* 图书导入
* @param request
* @param response
* @return
*/
@SuppressWarnings("unchecked")
@RequestMapping(params = "importExcel", method = RequestMethod.POST)
@ResponseBody
public AjaxJson importExcel(HttpServletRequest request, HttpServletResponse response) {
AjaxJson j = new AjaxJson();
MultipartHttpServletRequest multipartRequest = (MultipartHttpServletRequest) request;
Map<String, MultipartFile> fileMap = multipartRequest.getFileMap();
for (Map.Entry<String, MultipartFile> entity : fileMap.entrySet()) {
MultipartFile file = entity.getValue();// 获取上传文件对象
ImportParams params = new ImportParams();
try {
List<TBBookEntity> listBooks =
(List<TBBookEntity>)ExcelImportUtil.importExcelByIs(file.getInputStream(),TBBookEntity.class,params);
for (TBBookEntity book : listBooks) {
if(book != null && book.getBookname() != null){
book.setStatus(Globals.BOOK_RETURN);
tBBookService.save(book);
}
}
j.setMsg("文件导入成功!");
} catch (Exception e) {
j.setMsg("文件导入失败!");
logger.error(ExceptionUtil.getExceptionMessage(e));
}finally{
try {
file.getInputStream().close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return j;
}
}
如果也想学习本系统,下面领取。回复:003ssh
更多推荐
所有评论(0)