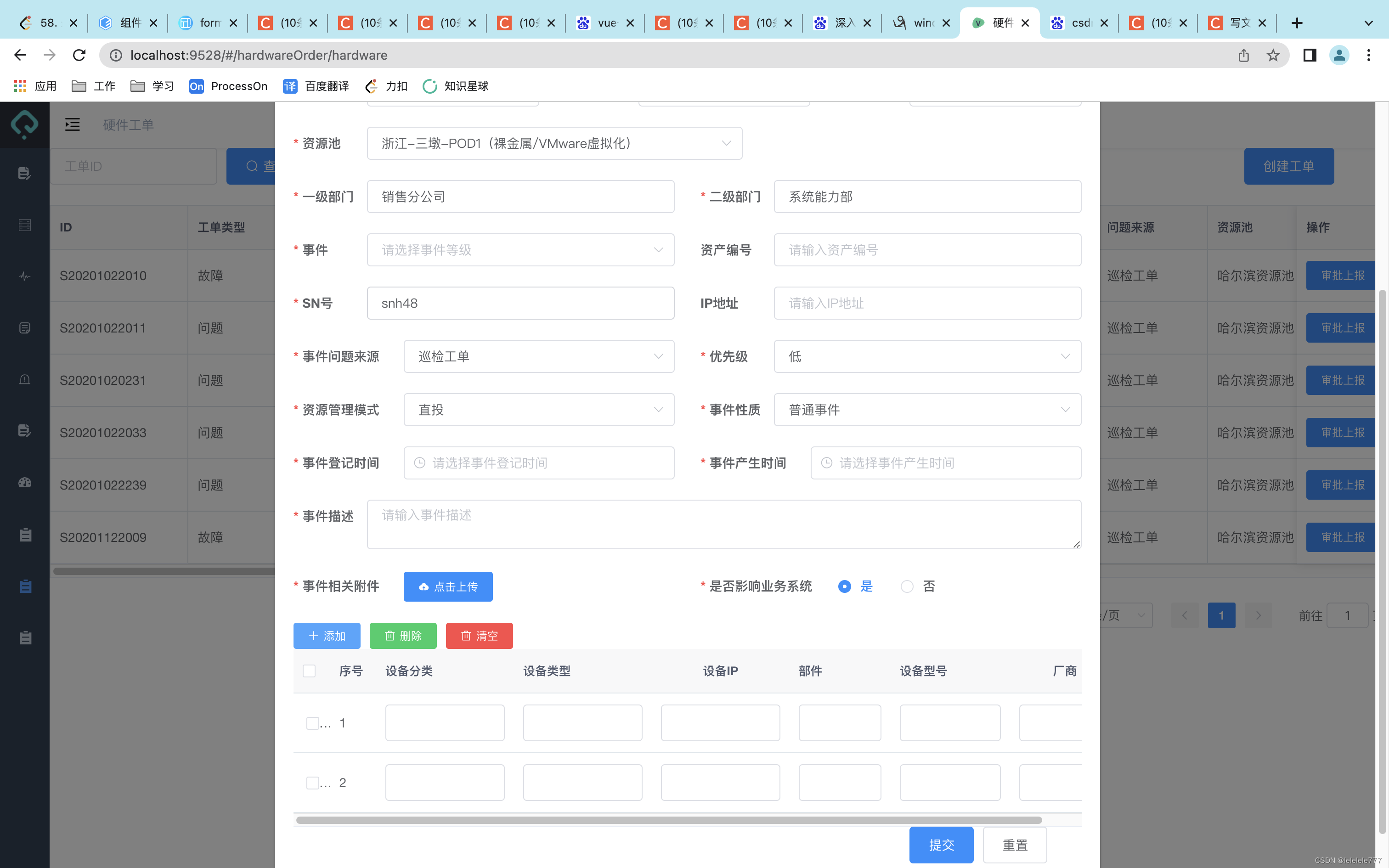
vue中添加附件以及表单内表格动态添加的功能实现
vue前端表单自动生成地址form-generatorvue中添加附件以及表单内表格动态添加的功能实现页面展示<el-col :span="12"><el-form-item label-width="120px" label="事件相关附件" prop="file" required><el-uploadclass="upload-demo"ref="upload"
·
vue前端表单自动生成地址
vue中添加附件以及表单内表格动态添加的功能实现
页面展示
<el-col :span="12">
<el-form-item label-width="120px" label="事件相关附件" prop="file" required>
<el-upload
class="upload-demo"
ref="upload"
:limit="1"
action ="dev-api/order/createHardWord"
:data="formData"
:file-list="fileList"
:http-request="httpRequest"
:on-change="handleChange"
:auto-upload="false">
<el-button size="small" type="primary" icon="el-icon-upload">
点击上传
</el-button>
</el-upload>
</el-form-item>
</el-col>
:limit="1" 限制附件数量
action ="dev-api/order/createHardWord":文件上传的地址
:data="formData" 绑定的表单数据
:file-list="fileList":文件列表
:http-request="httpRequest":请求方式
:on-change="handleChange":对附件进行处理
:auto-upload="false":是否自动提交 :否
参数
formData: {
orderID: '',
zt: '',
lcgdlx: '',
sjdjsj: '',
sjcssj: '',
lxr: '',
lxrdh: '',
lxryx: '',
zyc: '',
yjbm: '',
ejbm: '',
sjwtly: '',
yxj: '',
sjflone: '',
sjfltwo: '',
}
fileList: [],
// 覆盖默认的上传行为,可以自定义上传的实现,将上传的文件依次添加到fileList数组中,支持多个文件
方法
httpRequest(option) {
this.fileList.push(option)
},
handleChange (file, fileList) {
this.fileList = fileList
},
提交按钮
<el-col :span="8">
<el-form-item size="large">
<el-button type="primary" @click="submitForm('formData')">
提交</el-button>
<el-button @click="resetForm('formData')">重置</el-button>
</el-form-item>
</el-col>
//提交的方法
submitForm: function () {
// 使用form表单的数据格式
let file = ""
file = this.fileList.length ? this.fileList[0].raw : ''
let paramsData = new FormData()
// 将上传文件数组依次添加到参数paramsData中
paramsData.append('file', file)
paramsData.append('data', JSON.stringify(this.formData));
paramsData.append('dataArray', JSON.stringify(this.bcglXiangXiList));
axios.post('dev-api/order/createHardWord', paramsData, {
headers: {
'Content-Type': 'multipart/form-data; charset=utf-8'
},
})
.then(res => {
// console.log(paramsData.get('orderID'))
if (res.data = '1'){
console.log("成功")
this.$refs.elForm.resetFields()//清除表单信息
this.$refs.upload.clearFiles()//清空上传列表
this.fileList = []//集合清空
this.bcglXiangXiList = [];
this.dialogFormVisible = false//关闭对话框
this.$message.success("创建成功!")
}else {
this.$message.error("失败!,请检查");
}
}).catch(() => {
this.$message.error("失败!");
});
},
后端接收代码
使用MultipartFile 进行文件参数的接收,MultipartFile是一个接口,并继承于InputStreamSource,该方法的返回类型是InputStream输入流类型,然后根据需求对文件进行解析
InputStream fileInputStream = file.getInputStream(); Workbook workbook = new XSSFWorkbook(fileInputStream);
@PostMapping("createHardWord")
@LoginRequired
public AjaxResult createHardWord(@RequestParam("file") MultipartFile file, @RequestParam("data") String data, @RequestParam("dataArray") String dataArray) {
int i = orderService.createHardWord(file, data, dataArray);
return AjaxResult.success(i);
}
表格内的添加和删除,选中功能的实现,有些是参考其他的文章,添加了多选的功能
<el-col :span="24">
<el-table-column type="selection" width="30" align="center" />
<el-button type="primary" icon="el-icon-plus" size="mini" @click="handleAddDetails">
添加
</el-button>
<el-button
type="success"
icon="el-icon-delete"
size="mini"
@click="handleDeleteDetails">删除</el-button>
<el-button
type="danger"
icon="el-icon-delete"
size="mini"
@click="handleDeleteAllDetails">清空</el-button>
<el-table
:data="bcglXiangXiList"
:row-class-name="rowClassName"
@selection-change="handleDetailSelectionChange"
ref="tb">
<el-table-column type="selection" width="40"/>
<el-table-column label="序号" prop="xh" width="50">
</el-table-column>
<el-table-column label="设备分类" width="150" prop="sbfl">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].sbfl">
</el-input>
</template>
</el-table-column>
<el-table-column label="设备类型" prop="sblx" width="150">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].sblx">
</el-input>
</template>
</el-table-column>
<el-table-column label="设备IP" align="center" prop="ip" width="150">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].ip">
</el-input>
</template>
</el-table-column>
<el-table-column label="部件" width="110" prop="bj">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].bj">
</el-input>
</template>
</el-table-column>
<el-table-column label="设备型号" prop="sbxh" width="130">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].sbxh">
</el-input>
</template>
</el-table-column>
<el-table-column label="厂商" prop="cs" width="120">
<template slot-scope="scope">
<el-input
clearable
v-model="bcglXiangXiList[scope.row.xh-1].cs">
</el-input>
</template>
</el-table-column>
</el-table>
</el-col>
对表格内容进行处理
handleAddDetails() {
if (this.bcglXiangXiList == undefined) {
this.bcglXiangXiList = new Array();
}
let obj = {};
obj.sbfl = "";
obj.sblx = "";
obj.ip = "";
obj.bj = "";
obj.sbxh = "";
obj.cs = "";
this.bcglXiangXiList.push(obj);
},
handleDeleteDetails() {
if (this.checkedDetail.length == 0) {
this.$alert("请先选择要删除的数据", "提示", {
confirmButtonText: "确定",
});
} else {
this.bcglXiangXiList.splice(this.checkedDetail[0].xh - 1, 1);
}
},
handleDeleteAllDetails() {
this.bcglXiangXiList = undefined;
},
//弹窗
rowClassName({ row, rowIndex }) {
row.xh = rowIndex + 1;
},
//框选中数据
handleDetailSelectionChange(selection) {
this.ghs = selection.map(item => item.xh)
this.selectedNum = selection.length
},
参数:
bcglXiangXiList: [],
更多推荐
所有评论(0)