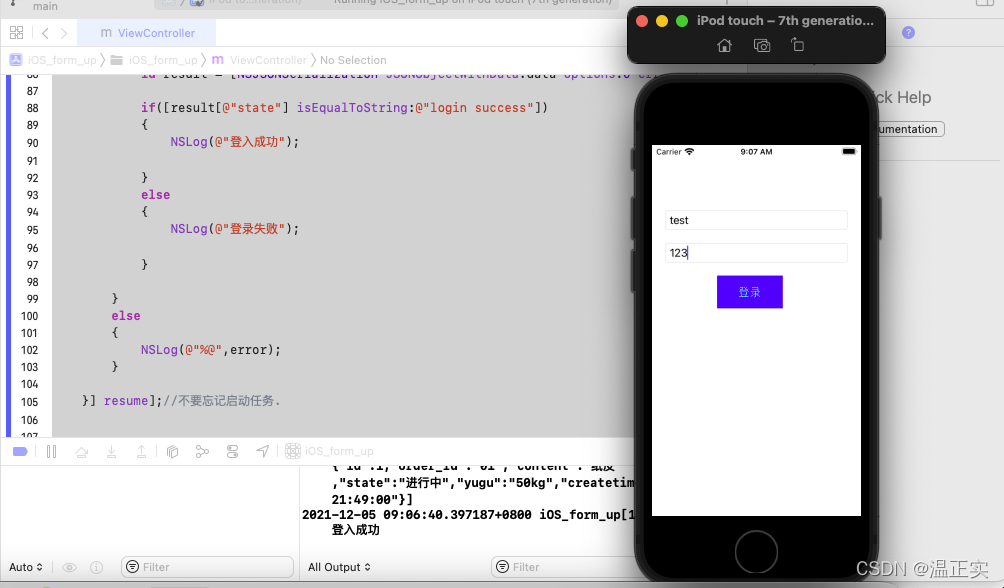
安卓表单提交vs iOS表单提交
先上服务器后台代码package test;import java.io.IOException;import javax.servlet.ServletException;import javax.servlet.annotation.WebServlet;import javax.servlet.http.HttpServlet;import javax.servlet.http.HttpSe
先上服务器后台代码
package test;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.JsonObject;
/**
* Servlet implementation class Android_Form_Up
*/
@WebServlet("/Android_Form_Up")
public class Android_Form_Up extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Android_Form_Up() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
response.setCharacterEncoding("utf-8");
request.setCharacterEncoding("utf-8");
// 获取Android和iOS发来的表单账号和密码
String account=request.getParameter("account");
System.out.println(account);
String password=request.getParameter("password");
System.out.println(password);
if(account.equals("test")&&password.equals("123")) {
//下面这行这是安卓的,安卓只返回一个普通文本字符串给前端
//response.getWriter().append("login success");
//下面这是iOS的,iOS返回一个json文本字符串给前端
JsonObject jsonContainer =new JsonObject();
//为当前的json对象添加键值对
jsonContainer.addProperty("state", "login success");
response.getWriter().print(jsonContainer);
System.out.println("登录成功");
}
else {
response.getWriter().append("login fail");
System.out.println("登录失败");
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
安卓前端代码
package com.example.android_form_up;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import android.util.Log;
import android.view.Gravity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLEncoder;
public class MainActivity extends AppCompatActivity {
private EditText form_account;
private EditText form_password;
private Button form_login;
private Handler handler;
private String result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
form_account=findViewById(R.id.form_account);
form_password=findViewById(R.id.form_password);
form_login=findViewById(R.id.form_login);
//设置点击登录按钮跳到账号和密码到服务器,服务器返回结果
form_login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//获取账号
String account=form_account.getText().toString();
String password=form_password.getText().toString();
//获取密码
//提交给服务器,注意这是耗时操作需要在子线程中进行
new Thread() {
@Override
public void run() {
String target;
target = "http://192.168.43.141:8080/myapp/Android_Form_Up";
URL url;
try {
url = new URL(target);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setRequestMethod("POST");
urlConnection.setConnectTimeout(30000);
urlConnection.setDoInput(true);
urlConnection.setDoOutput(true);
urlConnection.setUseCaches(false);
urlConnection.setInstanceFollowRedirects(true);
urlConnection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
DataOutputStream out=new DataOutputStream(urlConnection.getOutputStream());
String param="account="+ URLEncoder.encode(account,"utf-8")+
"&password="+ URLEncoder.encode(password,"utf-8");
out.writeBytes(param);
out.flush();
out.close();
if (urlConnection.getResponseCode() == HttpURLConnection.HTTP_OK) {
InputStreamReader in = new InputStreamReader(urlConnection.getInputStream());
BufferedReader buffer = new BufferedReader(in);
String inputLine = null;
while ((inputLine = buffer.readLine()) != null) {
//服务器返回结果
result = inputLine;
}
in.close();
}
urlConnection.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
Message m = handler.obtainMessage();
handler.sendMessage(m);
}
}.start();
handler = new Handler(Looper.getMainLooper()) {
@Override
public void handleMessage(Message msg) {
//根据服务器返回值做出相应操作
if(result.equals("login success")) {
Toast.makeText(getBaseContext(), "登录成功", Toast.LENGTH_LONG).show();
Log.d("结果:","登录成功");
}
else {
Toast.makeText(getBaseContext(), "登录失败,密码错误", Toast.LENGTH_LONG).show();
Log.d("结果:","密码错误");
}
super.handleMessage(msg);
}
};
}
});
}
}
xml布局文件代码
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/form_account"
android:layout_width="131dp"
android:layout_height="90dp"
android:hint="请填写账号"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.575"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.176" />
<EditText
android:id="@+id/form_password"
android:layout_width="140dp"
android:layout_height="75dp"
android:hint="请填写密码"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.516"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.391" />
<Button
android:id="@+id/form_login"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="登录"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.534" />
</androidx.constraintlayout.widget.ConstraintLayout>
注意在AndroidMainfest.xml中配置网络权限
<!-- 打开网络权限 --> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.INTERNET" />
运行效果图:
前端
后台
iOS 代码
//
// ViewController.m
// iOS_form_up
//
// Created by 温国强 on 2021/12/2.
//
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
UITextField *textField;
UITextField *textField2;
- (void)viewDidLoad {
[super viewDidLoad];
//创建输入框
textField = [[UITextField alloc]initWithFrame:CGRectMake(20, 100, 280, 30)];
// UITextBorderStyleRoundedRect // 边框风格
textField.borderStyle = UITextBorderStyleRoundedRect;
// 设置提示文字
textField.placeholder = @"请输入账号";
// 将控件添加到当前视图上
[self.view addSubview:textField];
textField2 = [[UITextField alloc]initWithFrame:CGRectMake(20, 150, 280, 30)];
// UITextBorderStyleRoundedRect // 边框风格
textField2.borderStyle = UITextBorderStyleRoundedRect;
// 设置提示文字
textField2.placeholder = @"请输入密码";
// 将控件添加到当前视图上
[self.view addSubview:textField2];
UIButton *btn = [[UIButton alloc]init];
//2.添加按钮
[self.view addSubview:btn];
//3.设置iframe
btn.frame =CGRectMake(100,200,100, 50);
//4.设置背景色
btn.backgroundColor = [UIColor blueColor];
//6.设置文字颜色
//设置文字
[btn setTitle:@"登录"forState:UIControlStateNormal];
//设置颜色
[btn setTitleColor:[UIColor cyanColor]forState:UIControlStateNormal];
[btn setTitleColor:[UIColor blueColor]forState:UIControlStateHighlighted];
//7.监听按钮点击
[btn addTarget:self action:@selector(btClick:) forControlEvents:UIControlEventTouchUpInside];
[btn sendActionsForControlEvents:UIControlEventTouchUpInside];
}
-(IBAction)btClick:(id)sender{
//获取输入框的字符串
NSLog(@"%@",textField.text);
NSLog(@"%@",textField2.text);
NSLog(@"hai");
NSString *urlString = [NSString stringWithFormat:@"http://192.168.43.141:8080/myapp/Android_Form_Up?account=%@&password=%@",textField.text,textField2.text];
//0.1因为有中文要进行百分号转义.(*** 注意点: 当有汉字时候***)
NSString *convertString = [urlString stringByAddingPercentEncodingWithAllowedCharacters:[NSCharacterSet URLQueryAllowedCharacterSet]];
//1.创建URL
NSURL *URL = [NSURL URLWithString:convertString];
//2.创建会话开启任务并启动(默认是数据任务是GET)
[[[NSURLSession sharedSession] dataTaskWithURL:URL completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) {
//3.错误处理
if(error == nil)
{
id result = [NSJSONSerialization JSONObjectWithData:data options:0 error:NULL];
if([result[@"state"] isEqualToString:@"login success"])
{
NSLog(@"登入成功");
}
else
{
NSLog(@"登录失败");
}
}
else
{
NSLog(@"%@",error);
}
}] resume];//不要忘记启动任务.
}
@end
运行效果图:
更多推荐
所有评论(0)