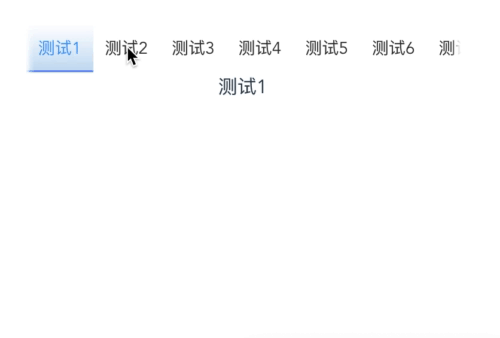
Vue封装Tabs标签页
示例一组件Tabs:<template><div class="my-tabs"><div class="tabs-bar"><div class="tabs-bar-nav"><divclass="tabs-tab"v-for="(tab, index) in tabList":class="[tabIndex == tab.
·
示例一
组件Tabs:
<template>
<div class="my-tabs">
<div class="tabs-bar">
<div class="tabs-bar-nav">
<div
class="tabs-tab"
v-for="(tab, index) in tabList"
:class="[tabIndex == tab.index ? 'tabs-active' : '']"
:style="tab.style"
@click="changeTab(tab)"
:key="index"
>
{{ tab.name }}
</div>
</div>
</div>
<div class="tabs-content">
<slot></slot>
</div>
</div>
</template>
<script>
export default {
name: "MyTabs",
props: {
tabList: Array,
tabIndex: Number,
},
data() {
return {};
},
methods: {
changeTab: function (tab) {
this.$emit("changeTab", tab);
},
},
};
</script>
<style lang="less" scoped>
.my-tabs {
font-size: 14px;
color: #444;
}
.tabs-bar {
border-bottom: 1px solid #eee;
position: relative;
padding: 5px 0;
}
.tabs-bar-nav {
display: table;
position: absolute;
bottom: -1px;
width: 100%;
}
.tabs-tab {
min-width: 110px;
padding: 5px 0;
position: relative;
display: inline-block;
text-align: center;
cursor: pointer;
}
.tabs-active {
border-bottom: 1px solid #409eff;
color: #409eff;
}
.tabs-content {
padding-left: 20px;
padding-right: 20px;
padding-bottom: 30px;
}
</style>
引用组建Tabs:
<template>
<div>
<MyTabs :tabList="tabList" :tabIndex="tabIndex" @changeTab="changeTab">
<div
v-for="(item, index) in tabList"
:key="index"
:class="currentTab === item.index ? 'show' : 'hidden'"
>
{{ item.component }}
</div>
</MyTabs>
</div>
</template>
<script>
import MyTabs from "../components/Tabs";
export default {
name: "Tabs",
data() {
return {
currentTab: 0,
tabIndex: 0,
currentContent: "one",
tabList: [
{
index: 0,
name: "选项一",
component: "one",
style: "width: 48%;",
},
{
index: 1,
name: "选项二",
component: "two",
style: "width: 48%;",
},
],
};
},
components: {
MyTabs,
},
created() {},
mounted() {},
methods: {
// 切换选项卡
changeTab: function (tab) {
this.tabIndex = tab.index;
this.currentTab = tab.index;
},
},
};
</script>
<style lang="less" scoped>
.show {
display: block;
}
.hidden {
display: none;
}
</style>
示例二
组件tabs:
<template>
<div class="header" ref="hhh">
<div class="header_search-left">
<!-- <slot name="header_tab_img"></slot> -->
</div>
<div class="header_tab" ref="headertab">
<ul ref="tabitem">
<li
v-for="(item, index) in listArray"
:key="index"
:class="index == current ? 'activeheader' : ''"
@click="changeTab(index, $event, item)"
>
{{ item.name }}
</li>
</ul>
</div>
<div class="header_search-right"></div>
</div>
</template>
<script>
export default {
name: "HeaderTab",
props: {
current: Number,
listArray: {
type: Array,
},
},
data() {
return {};
},
methods: {
changeTab(index, e, item) {
this.$emit("changeTab", item);
this.current = index; // 高亮当前
let tab = this.$refs.headertab; // 包裹 ul的 div
let tabitem = this.$refs.tabitem; // 包裹 li的 ul
let winWidth = this.$refs.hhh.clientWidth; // 当前屏幕的宽度
let liList = e.target; // 当前点击的li
if (liList) {
// 当前li左偏移, li的宽度, 中间值(当前屏幕的宽度 - li的宽度) /2, 目标值 (中间值 - 当前li左偏移), 整个ul的宽度
let liLeft = liList.offsetLeft,
liWidth = liList.offsetWidth,
liCenter = (winWidth - liWidth) / 2,
liTarget = liLeft - liCenter;
let ulWidth = tabitem.offsetWidth;
if (liTarget < 0) {
tab.scrollLeft = 0;
return;
}
// winWidth(375) - ulWidth(436) = -61
if (liTarget < winWidth - ulWidth) {
tab.scrollLeft = -(winWidth - ulWidth) + liWidth;
return;
}
tab.scrollLeft = liTarget;
}
},
},
};
</script>
<style lang="less" scoped>
.header {
width: 100%;
height: 40px;
background-color: #fff;
display: flex;
border-radius: 8px;
position: relative;
overflow: hidden;
}
.header_tab {
width: 90%;
height: 40px;
display: flex;
flex-wrap: nowrap;
overflow: scroll;
padding-left: 19px;
padding-right: 19px;
// 平滑滚动
transition: all 0.05s linear;
scroll-behavior: smooth;
scrollbar-width: none;
}
.header_tab::-webkit-scrollbar {
display: none;
}
ul {
display: inline-block;
white-space: nowrap;
margin: 0;
padding-left: 0;
padding-right: 19px;
height: 40px;
}
li {
display: inline-block;
height: 40px;
line-height: 40px;
padding: 0px 10px;
font-size: 14px;
color: #333;
cursor: pointer;
// 点击高亮某一项时,将原来的字体变大,会导致没有高亮的元素距离底部有空隙,会出现纵向滚动条
// margin-top: -1px;
}
.activeheader {
font-size: 14px;
color: #2b96ff;
// font-weight: 700;
position: relative;
background: linear-gradient(
180deg,
rgba(71, 151, 220, 0) 0%,
rgba(71, 151, 220, 0.35) 100%
);
&:after {
position: absolute;
content: "";
width: 100%;
height: 2px;
/* bottom: 0; */
left: calc(50% - 52px);
background-color: #2b96ff;
border-radius: 1px;
height: 0.1rem;
box-sizing: border-box;
background-color: #3e75fc;
position: absolute;
left: 0;
bottom: 0.05rem;
z-index: 1;
transition: transform 0.3s ease-in-out;
transform-origin: 0 0;
}
}
.header_search-left {
width: 25px;
height: 40px;
position: absolute;
top: 0;
left: 0;
z-index: 1000;
background-image: -webkit-linear-gradient(
to right,
rgba(255, 255, 255, 1) 0%,
rgba(255, 255, 255, 1) 55%,
rgba(255, 255, 255, 1) 70%,
rgba(255, 255, 255, 0.8) 80%,
rgba(255, 255, 255, 0.3) 90%,
rgba(255, 255, 255, 0.1) 100%
);
background-image: linear-gradient(
to right,
rgba(255, 255, 255, 1) 0%,
rgba(255, 255, 255, 1) 55%,
rgba(255, 255, 255, 1) 70%,
rgba(255, 255, 255, 0.8) 80%,
rgba(255, 255, 255, 0.3) 90%,
rgba(255, 255, 255, 0.1) 100%
);
img {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
margin: auto;
}
}
.header_search-right {
width: 25px;
height: 40px;
position: absolute;
top: 0;
right: 0;
z-index: 1000;
background-image: -webkit-linear-gradient(
to left,
rgba(255, 255, 255, 1) 0%,
rgba(255, 255, 255, 1) 55%,
rgba(255, 255, 255, 1) 70%,
rgba(255, 255, 255, 0.8) 80%,
rgba(255, 255, 255, 0.3) 90%,
rgba(255, 255, 255, 0.1) 100%
);
background-image: linear-gradient(
to left,
rgba(255, 255, 255, 1) 0%,
rgba(255, 255, 255, 1) 55%,
rgba(255, 255, 255, 1) 70%,
rgba(255, 255, 255, 0.8) 80%,
rgba(255, 255, 255, 0.3) 90%,
rgba(255, 255, 255, 0.1) 100%
);
img {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
margin: auto;
}
}
</style>
引用组件tabs:
<template>
<div style="width: 400px">
<header-tab :listArray="list" :current="current" @changeTab="changeTab">
</header-tab>
<div
v-for="(item, index) in list"
:key="index"
:class="currentTab === item.id ? 'show' : 'hidden'"
>
{{ item.name }}
</div>
</div>
</template>
<script>
import HeaderTab from "../components/tabs";
export default {
components: {
HeaderTab,
},
data() {
return {
current: 0,
currentTab: 0,
list: [
{ id: 0, name: "测试1" },
{ id: 1, name: "测试2" },
{ id: 2, name: "测试3" },
{ id: 3, name: "测试4" },
{ id: 4, name: "测试5" },
{ id: 5, name: "测试6" },
{ id: 6, name: "测试7" },
{ id: 7, name: "测试8" },
{ id: 8, name: "测试9" },
{ id: 9, name: "测试10" },
{ id: 10, name: "测试11" },
{ id: 11, name: "测试12" },
{ id: 12, name: "测试13" },
{ id: 13, name: "测试14" },
{ id: 14, name: "测试15" },
{ id: 15, name: "测试16" },
{ id: 16, name: "测试17" },
{ id: 17, name: "测试18" },
{ id: 18, name: "测试19" },
],
};
},
created() {},
mounted() {},
methods: {
// 切换选项卡
changeTab(tab) {
this.current = tab.id;
this.currentTab = tab.id;
},
},
};
</script>
<style lang="less" scoped>
.show {
display: block;
}
.hidden {
display: none;
}
</style>
更多推荐
所有评论(0)