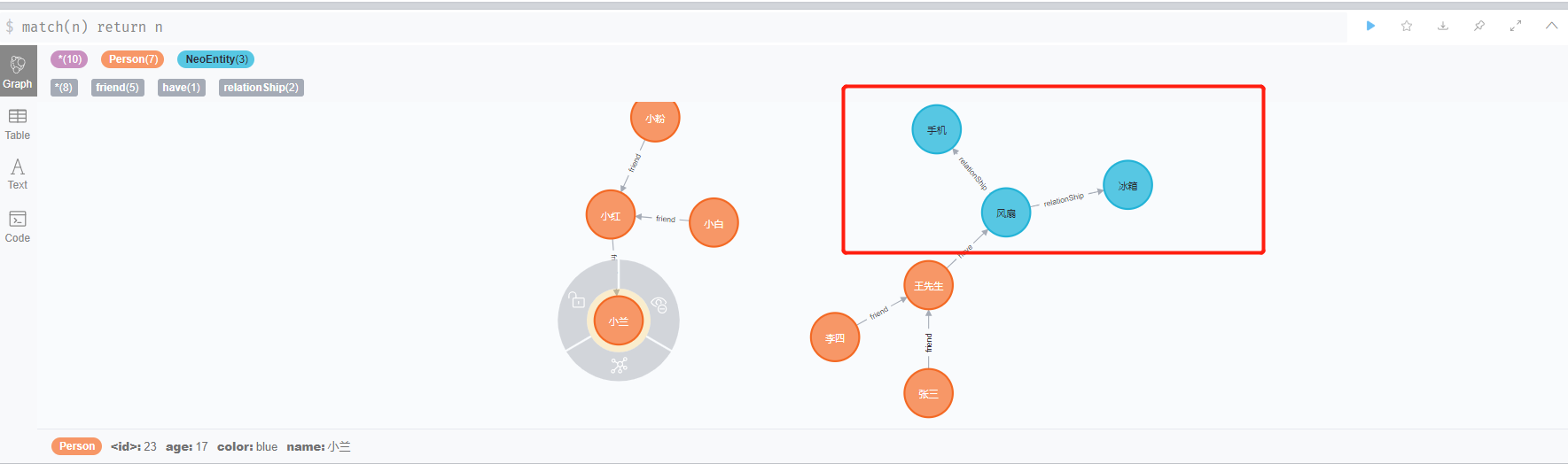
关于springboot整合neo4j图数据库实现增删改
第一步,引jar包<dependency><!-- 这个是手写表达式使用的包 --><groupId>org.neo4j.driver</groupId><artifactId>neo4j-java-driver</artifactId><version>1.5.0</version></depe
·
第一步,引jar包
<dependency>
<!-- 这个是手写表达式使用的包 -->
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>1.5.0</version>
</dependency>
<dependency>
<!-- 嵌入式开发需要的jar包 -->
<groupId>org.neo4j</groupId>
<artifactId>neo4j</artifactId>
<version>3.3.4</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-neo4j</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.8</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>4.1.19</version>
</dependency>
第二部,配置文件加属性
spring:
data:
neo4j:
uri: bolt://localhost:7687
username: neo4j
password: devote
第三步,创建实体类
/**
* 此实体类就是数据库里的标签
*/
package com.fy.entity;
/**
* @ClassName NeoEntity
* @author: maliang
* @Description TODO
* @date 2023/3/29 11:15
* @Version 1.0版本
*/
import lombok.Data;
import org.springframework.data.neo4j.core.schema.GeneratedValue;
import org.springframework.data.neo4j.core.schema.Id;
import org.springframework.data.neo4j.core.schema.Node;
/**
* 通用对象
*/
@NodeEntity(type = "neoEntity ")
@Setter
@Getter
@ToString
public class NeoEntity {
@Id
@GeneratedValue
private Long id;
private String name;
private String num;
private int age;
private String local;
}
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.neo4j.ogm.annotation.*;
/**
* 此数据库是属性之间的关系
*/
@RelationshipEntity(type = "relationShip")
@Setter
@Getter
@ToString
public class NeoShip {
@Id
@GeneratedValue
private Long id;
@StartNode
private NeoEntity parent;
@EndNode
private NeoEntity son;
}
package com.entity;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.neo4j.ogm.annotation.*;
/**
* @ClassName HaveShip
* @author: maliang
* @Description TODO
* @date 2023/3/29 11:18
* @Version 1.0版本
*/
@RelationshipEntity(type = "have")
@Setter
@Getter
@ToString
public class HaveShip {
@Id
@GeneratedValue
private Long id;
@StartNode
private NeoEntity parent;
@EndNode
private NeoEntity son;
}
创建两个mapper
package com.condition.dao;
import org.springframework.data.neo4j.repository.Neo4jRepository;
import org.springframework.stereotype.Component;
import com.condition.pojo.NeoEntity;
@Component
public interface NeoRespsitory extends Neo4jRepository<NeoEntity, Long> {
}
---------------------------------------------------------------------------
package com.condition.dao;
import org.springframework.data.neo4j.repository.Neo4jRepository;
import org.springframework.stereotype.Component;
import com.condition.pojo.NeoShip;
@Component
public interface NeoShipRespitory extends Neo4jRepository<NeoShip, Long>{
}
---------------------------------------------------------------------------
package com.condition.dao;
import org.springframework.data.neo4j.repository.Neo4jRepository;
import org.springframework.stereotype.Component;
import com.condition.pojo.HaveShip;
import com.condition.pojo.NeoEntity;
@Component
public interface HaveRespsitory extends Neo4jRepository<HaveShip, Long> {
}
在启动类上添加扫描配置
@EnableNeo4jRepositories(basePackages ="com.condition.dao")
@EntityScan(basePackages = "com.condition.pojo")
最后创建controller,测试一下
package com.condition.controller;
import java.util.ArrayList;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.condition.dao.HaveRespsitory;
import com.condition.dao.NeoRespsitory;
import com.condition.dao.NeoShipRespitory;
import com.condition.pojo.HaveShip;
import com.condition.pojo.NeoEntity;
import com.condition.pojo.NeoShip;
@RestController
@RequestMapping("neo4j")
public class NeoController {
@Autowired
private NeoRespsitory neo4jRepository;
@Autowired
private NeoShipRespitory neoshipRespitory;
@Autowired
private HaveRespsitory haveRespsitory;
/**
* 方法一
*/
@PostMapping("save")
private String save(@RequestBody List<NeoEntity> eNeoEntity) {
neo4jRepository.saveAll(eNeoEntity);
NeoEntity neoEntity = eNeoEntity.get(0);
List<NeoEntity> subList = eNeoEntity.subList(1, eNeoEntity.size());
List<NeoShip> list = new ArrayList<NeoShip>();
for (NeoEntity neoEntity2 : subList) {
NeoShip neoShip = new NeoShip();
neoShip.setParent(neoEntity);
neoShip.setSon(neoEntity2);
list.add(neoShip);
}
neoshipRespitory.saveAll(list);
return "success";
}
/**
* 方法二
*/
@GetMapping("findAll")
public Iterable<NeoEntity> findAll() {
return neo4jRepository.findAll();
}
/**
* 方法三
*/
@GetMapping("findAll1")
public Iterable<NeoShip> findAll1() {
return neoshipRespitory.findAll();
}
/**
* 方法四
*/
@GetMapping("findAll2")
public Iterable<HaveShip> findAll2() {
return haveRespsitory.findAll();
}
}
方法一执行完成后,
参数:
[
{
"name":"风扇",
"num":"001",
"age":"15",
"local":"北京"
},{
"name":"冰箱",
"num":"6545",
"age":"56",
"local":"杭州"
},{
"name":"手机",
"num":"08",
"age":"15",
"local":"深圳"
}
]
数据库现显示( match(n) return n )
方法二执行完成后:
此时查询的数据,主要是以同一标签为主
方法三执行完成后:
此时的数据主要是同一标签,但是存在上下级关系
方法四执行完成后:
此时的数据是不同标签,同时查出来的
更多推荐
所有评论(0)