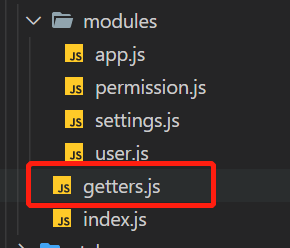
组件中调用Vuex的state,getters,mutations,actions,modules的数据传递、传参问题
1.State观点:state中的数据,Vuex规定只能通过mutations来进行提交操作,基本在组件中,调取相关mutations、actions等就能满足要求,所以,个人觉得state在组件中读取没有特殊情况下的话,也没多大意义。...mapMutations(['moduleAMutation']) 映射(moduleAMutation)为store里面mutatio...
vuex怎么传递数据到modules中,并获取数据 - 简书
vuex namespaced的作用以及使用方式_不屑哥的专栏-CSDN博客
提交 获取 getters、commit
const getters = {
sidebar: state => state.app.sidebar,
token: state => state.user.token,
avatar: state => state.user.avatar,
name: state => state.user.name,
status: state => state.user.status,
roles: state => state.user.roles,
menus: state => state.user.menus,
permission_routers: state => state.permission.routers,
addRouters: state => state.permission.addRouters,
};
export default getters
获取getters里面的数据
computed: {
...mapGetters([
'sidebar',
'avatar',
])
},
vuex中的store分模块管理,需要在store的index.js中引入各个模块,为了解决不同模块命名冲突的问题,将不同模块的namespaced:true,之后在不同页面中引入getter、actions、mutations时,需要加上所属的模块名
一、模块必写
示例:namespaced:true
必写原因 为,当多模块整合时,actions和mutations会整合为数组,但是getters不会,它是唯一的,不会整合,所以为了getters正常使用的同时每个模块具有独立性和可复用,namespaced:true必须写
1、声明分模块的store时加上namespaced:true
使用模块中的mutations、getters、actions时候,要加上模块名,例如使用commint执行mutations时
格式:模块名/模块中的mutations
xxx/setUserInfo
this.$store.commit("userInfo/setUserInfo",userInfo)
3、获取属性时同样加上模块名
格式:store.state.模块名.模块属性 一般外部推荐用this.的这种
this.$store.state.模块.num //数据 this.$store.state.userInfo.userName
this.$store.dispatch('模块/方法') //方法
this.$store.getters['模块/sum']
第一步:
import store from "@/store";
//state
store.state.count
store.state.moduleA.count
this.$store.state.count
this.$store.state.moduleA.count
//获取getters里面的token
store.getters.token
this.$store.getters.moduleA.num
//mutation 提交
store.commit('RESET_TOKEN','参数');
store.commit('user/RESET_TOKEN','参数');
this.$store.commit('moduleAMutation/RESET_TOKEN');
mutations: {
increment (state, payload) {
state.count += payload.amount
}
}
//action 提交
store.dispatch("user/ssologin");
store.dispatch("user/login",null);
this.$store.dispatch(`${moduleName}/getContractNumber`,'参数')
actions: {
increment (context) {
context.commit('increment')
}
increment ({ commit, state }, params) {
commit('increment','参数')
}
}
1.State
观点:state中的数据,Vuex规定只能通过mutations来进行提交操作,基本在组件中,调取相关mutations、actions等就能满足要求,所以,个人觉得state在组件中读取没有特殊情况下的话,也没多大意义。
...mapMutations(['moduleAMutation']) 映射(moduleAMutation)为store里面mutations定义的方法名
//moduleA--模块A
const moduleA = {
state:{
count:888
}
}
//组件中,获取State的数据
<template>
<div>
<span>基础获取方式:(通过该方式获取的,可以写在computed计算属性内,这样看着不复杂)</span>
<span>获取父store中的state下的count值:{{this.$store.state.count}}</span>
<span>获取moduleA模块中的state下的count值:{{this.$store.state.moduleA.count}}</span>
<hr>
<span>mapState辅助函数获取方式:</span>
<span>获取父store中的state下的count值:{{count}}</span>
<span>获取moduleA模块中的state下的count值:{{countA}}</span>
</div>
</template>
<script>
import {mapState} from 'vuex';
export default {
name: "Body",
computed: {
//获取父store中的state下的count值
...mapState(['count']),
//获取moduleA模块中的state下的count值
...mapState({
countA:state=>state.moduleA.countA
}),
}
}
</script>
2.Getters
rootState和 rootGetters参数顺序不要写反,一定是state在前,getter在后面,这是vuex的默认参数传递顺序。
rootGetters参数中,包括根store以及模块中的所有getters方法,直接rootGetters.方法名即可获取到。
有这样的一个场景: Vuex 定义了某个数据 list, 它是一个数组,如果只想得到小于 10 的数据,最容易想到的方法可能是在组件的计算属性里进行过滤。这样写完全没有问题。但如果还有其他的组件也需要过滤后的数据时,就得把 computed 的代 码完全复制一份,而且需要修改过滤方法时,每个用到的组件都得修改,这明显不是我们期望的结 果。如果能将 computed的方法也提取出来就方便多了, getters 就是来做这件事的。
个人观点:针对以上这个场景,getters其实就相当于是一个普通方法。getters和mutations/actions之间其实没有任何关系,所以说,在此处getters只要能获取到根store或者兄弟模块的state参数值,getters方法,它的目标其实就已经达到了
//模块中
const moduleA = {
getters:{
showStateData(state,getters,rootState,rootGetters){
console.log('当前模块的count值:'+state.countA);
console.log('根store中的count值:'+rootState.count);
console.log('兄弟模块moduleB中的countB值:'+rootState.moduleB.countB);
console.log('根store中的名为increNum的getters方法:'+rootGetters.increNum);
console.log('兄弟模块moduleB中的名为moduleBGetter的getters方法:'+rootGetters.moduleBGetter);
},
======================================================================================================
//就是此处。该getters针对下面组件中有参数的情况
moduleAGetter:(state,rootState,rootGetters)=>(param,name)=>{
console.log(param)
console.log(name)
}
}
}
//组件中使用时,如何传参问题
<template>
<div>
我是Body<hr>
<button @click="moduleAGetter">getters获取数据</button>
<button @click="changeNameGetter">更名后的getters获取数据</button>
</div>
</template>
<script>
import {mapGetters} from 'vuex';
export default {
name: "Body",
methods: {
//1.普通情况下,分为如下两种情况:
commonGetter(){
//⑴无参数引用getter
return this.$store.getters.moduleAGetter;
//⑵有参数引用getter,该这么传(亦可传递对象)。或者这样子传也好:commonGetter(id,name){...}
return this.$store.getters.moduleAGetter(996,'Mr.Liu');//请查看上面模块中定义的moduleAGetter方法。
},
},
computed: {
//2.mapGetters辅助函数,分为如下三种情况:
//⑴无参数引用getter
...mapGetters(['moduleAGetter']),
//⑵无参数引用getter,更名情况下
...mapGetters({
changeNameGetter:'moduleAGetter'
}),
//⑶有参数引用getter
//暂未找到mapGetter如何使用辅助函数传参情况,如有知道,欢迎大家补充
},
}
</script>
3.Mutations
mutations,唯一的用途就是:用来修改state中的数据。他不可能去拿根store或者兄弟模块的getters,mutations,actions等。
个人观点:mutaions只能修改state中的数据,况且应该是只能修改本模块的state中的数据,所以它是无法拿到根store和兄弟模块的state的值,只能拿到自己模块的state值
//模块中
const moduleA = {
mutations:{
moduleAMutation(state){
console.log('当前模块的count值:'+state.count);
},
=======================================================================================================
//paramMutation针对下面组件中传参情况下使用
paramMutation(state,obj){
console.log(obj.id);
console.log(obj.name);
console.log('A模块中的count属性值:'+state.count);
},
}
}
//组件中使用时,如何传参问题
//目前个人研究完,模块中的mutaion中,默认第一个传递参数是state,这个是Vuex定死的的,剩下的自定义参数部分
//个人研究发现我们只能传递一个。如果要传递多个参数,只能以对象的形式传递。如有错误或者指正,欢迎评论修改
<template>
<div>
我是Body<hr>
<button @click="commonMutation">⑴基础mutations获取数据</button>
<button @click="moduleAMutation">⑵辅助函数mutations获取数据</button>
<button @click="changeNameMutation">⑶改名mutations获取数据</button>
<button @click="paramMutation({id:996,name:'Mr.Liu'})">⑷有参数普通mutations获取数据</button>
<button @click="paramMapMutation({id:996,name:'Mr.Liu'})">⑸有参数辅助函数mutations获取数据</button>
</div>
</template>
<script>
import {mapGetters,mapActions,mapMutations} from 'vuex';
export default {
name: "Body",
methods: {
⑴commonMutation(){
this.$store.commit('moduleAMutation');
},
commonMutation(data) {
this.$store.commit("add",data) //Mutation方法,参数 普通
}
⑵...mapMutations(['moduleAMutation']),
2、调用:moduleAMutation(){this.moduleAMutation}
⑶...mapMutations({
changeNameMutation:'moduleAMutation'
}),
⑷paramMutation(obj){
this.$store.commit('moduleAMutation',obj);
this.$store.commit('moduleAMutation',{a:1,b:2});
},
⑸...mapMutations(obj){//传参,使用辅助函数,参数目前我研究完,只能传递到上面<button @click="paramMapMutation({id:996,name:'Mr.Liu'})"><button>调用的地方
paramMapMutation:'moduleAMutation',
})
}
}
</script>
<style scoped>
</style>
4.Actions
Actions主要用来①提交mutations ②执行异步操作
Vuex为Actions提供rootState参数,来获取根store,兄弟模块中的数据。
个人观点:既然已经分模块,各个模块数据之间要相互独立,所以模块A中的actions也不可能去提交模块B或者跟store中的mutations吧,所以actions部分,只能获取到根store或者兄弟模块中的state数据即可
actions 回调:
https://segmentfault.com/q/1010000012992271
actions: {
actionA ({ commit }) {
return new Promise((resolve, reject) => {
setTimeout(() => {
commit('someMutation')
resolve()
}, 1000)
})
}
}
this.$store.dispatch('getUserAuth').then(()=>{
// 接口请求完成后
})
getCertificationStatus(context, vm){
return new Promise((resolve, reject) => {
axios.post('/realNameUtils/gotoStatusPage')
.then((res)=>{
context.commit('certificationStatus',res.data.content)
})
})
}
}
// 获取编号
async getNumber() {
// 在 actions 函数里 await 也行 看具体需求 此例只作为演示 await 调用
const obj = await this.$store.dispatch(`${moduleName}/getContractNumber`)
this.contractInfo.fileNo = obj.fileNo
},
//模块中
const moduleA = {
actions:{
getStateData({state,commit,getters,rootState,rootGetters}){
console.log('当前模块的count值:'+state.count);
console.log('根store中的count值:'+rootState.count);
console.log('兄弟模块moduleB中的count值:'+rootState.moduleB.count);
rootGetters.increment();//获取根store中的名为increment的getters方法
getters.moduleAIncrement();//获取当前模块中的getters方法,传参的话直接在括号中传即可,可以使多个参数,也可以是一个obj对象
},
=======================================================================================================
//moduleAAction针对下面组件中传参情况下使用
moduleAAction({state,commit,rootState},obj){//此处传递了一个obj对象参数
console.log(state)
console.log(obj.id);
console.log(obj.name);
console.log('A模块中的count属性值:'+state.count);
console.log('父模块中的count属性值:'+rootState.count);
console.log('兄弟模块moduleB中的count属性:'+rootState.moduleB.count);
commit('moduleAIncr',obj.id);//此处为将obj对象的id参数,继续提交mutaions使用
}
},
mutations:{
//此处额外附名为moduleAIncr的mutations方法,仅用作下面部分参考使用
moduleAIncr:(state,num)=>{
console.log(num);
state.count +=num;
console.log(state.count)
}
}
}
//组件中使用时,如何传参问题(其实和上面的Mutations传参一样,没有区别)
<template>
<div>
我是Body<hr>
<hr>
<button @click="commonAction">⑴基础actions获取数据</button>
<button @click="moduleAAction">⑵辅助函数actions获取数据</button>
<button @click="changeNameAction">⑶改名actions获取数据</button>
<button @click="paramAction({id:996,name:'Mr.Liu'})">⑷有参数普通actions获取数据</button>
<button @click="paramMapAction({id:996,name:'Mr.Liu'})">⑸有参数辅助函数actions获取数据</button>
</div>
</template>
<script>
import {mapGetters,mapActions,mapMutations} from 'vuex';
export default {
name: "Body",
methods: {
⑴commonAction(){
this.$store.dispatch('moduleAAction')
},
⑵...mapActions(['moduleAAction']),
⑶...mapActions({
changeNameAction:'moduleAAction'
}),
⑷paramAction(id,name){
this.$store.dispatch('moduleAAction',id,name);
this.$store.dispatch('moduleAAction',{a:1,b:2});
},
⑸...mapActions({//传参,使用辅助函数,参数目前我研究完,只能传递到上面<button @click="paramMapAction({id:996,name:'Mr.Liu'})"><button>调用的地方
paramMapAction:'moduleAAction'
})
}
}
</script>
<style scoped>
</style>
更多推荐
所有评论(0)