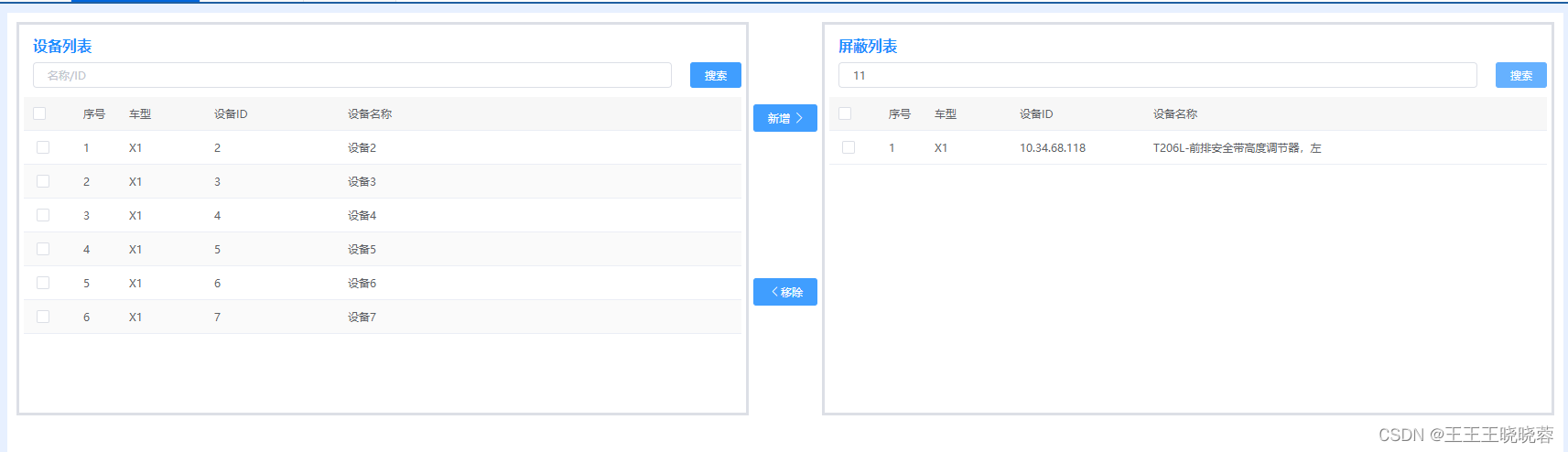
vue+element ui表格穿梭框
vue+element ui表格穿梭框
·
需求:
1.数据移动:左侧数据可移入右侧,右侧数据可移除回到左侧,且移回后顺序不改变
2.可进行模糊查询
(初始数据为请求后端接口获取,数据移动、模糊查询均为前端实现)
左侧框
<div class="transferbox">
<div class="topbox">
<span style="color:#1E90FF;font-size:16px;font-weight: 550;" v-if="centerDialog.form.shiledType == 2">设备列表</span>
<span style="color:#1E90FF;font-size:16px;font-weight: 550;" v-if="centerDialog.form.shiledType == 1">关重件列表</span>
</div>
<div class="level searchbox">
<el-input v-model="transferInfo.transferLeftSearch" :placeholder="centerDialog.form.shiledType == 2 ? '名称/ID' : '名称/代码'" clearable size="mini" />
<el-button type="primary" @click="eqpSearch('L')" size="mini">搜索</el-button>
</div>
<el-table ref="staffTable" :data="transferInfo.leftEqpData" stripe max-height="340" highlight-current-row tooltip-effect="dark" size="mini" @selection-change="handleSelectionChange_left">
<el-table-column type="selection" width="55">
</el-table-column>
<el-table-column label="序号" width="50">
<template slot-scope="scope">{{ (scope.$index + 1) }}</template>
</el-table-column>
<el-table-column v-for="item in transferInfo.columns" :key="item[transferInfo.key]" :prop="item.prop" :label="item.label" :min-width="item.width" :show-overflow-tooltip="true" :formatter="item.formatter">
</el-table-column>
</el-table>
</div>
中间按钮
<div class="centerSearchBox">
<el-button type="primary" size="mini" @click="addEqp()">新增 <span style="font-size: 14px;" class="icon iconfont icon-you"></span> </el-button>
<el-button type="primary" size="mini" @click="removeEqp()">
<span class="icon iconfont icon-xiangzuo_o"></span>移除
</el-button>
</div>
右侧框
<div class="transferbox">
<div class="topbox">
<span style="color:#1E90FF;font-size:16px;font-weight: 550;">屏蔽列表</span>
</div>
<div class="level searchbox">
<el-input v-model="transferInfo.transferRightSearch" :placeholder="centerDialog.form.shiledType == 2 ? '名称/ID' : '名称/代码'" clearable size="mini" />
<el-button type="primary" @click="eqpSearch('Y')" size="mini">搜索</el-button>
</div>
<el-table ref="staffTable" :data="transferInfo.rihghtEqpData" stripe highlight-current-row max-height="340" tooltip-effect="dark" size="mini" @selection-change="handleSelectionChange_right">
<el-table-column type="selection" width="55">
</el-table-column>
<el-table-column label="序号" width="50">
<template slot-scope="scope">{{ (scope.$index + 1) }}</template>
</el-table-column>
<el-table-column v-for="item in transferInfo.columns" :key="item[transferInfo.key]" :prop="item.prop" :label="item.label" :min-width="item.width" :show-overflow-tooltip="true" :formatter="item.formatter">
</el-table-column>
</el-table>
</div>
<script>
import api from "@/api"
export default {
name: "qoeShield",
// 初始数据定义处
data () {
return {
//穿梭框
transferInfo: {
key: "id",
transferLeftSearch: "",
transferRightSearch: "",
multipleSelection_left: [],
multipleSelection_right: [],
columns: [
{ prop: "model", label: "车型", width: 50 },
{ prop: "equipmentId", label: "设备ID" },
{ prop: "equipmentName", label: "设备名称", width: 240 },
],
leftEqpData: [],
rihghtEqpData: [],
},
transferInfo_equipment: [],//查询的所有设备数据
transferInfo_equipmentL: [],//左侧数据
transferInfo_equipmentR: [],//右侧数据
allDataR: [],
};
},
created () {
this.initialloading(); //初始化加载
},
activated () {
if (!this.$route.meta.isUseCache) {
this.initialloading(); //初始化加载
}
},
methods: {
// 初始加载
initialloading () {
this.getAllEqpList()
},
//获取所有设备
getAllEqpList () {
//获取新的数据源——清空所有数据
this.transferInfo_equipment = []
this.transferInfo_equipmentL = []
this.transferInfo_equipmentR = []
this.transferInfo.leftEqpData = []
this.transferInfo.rihghtEqpData = []
//请求参数根据自己情况
let param = {
equipmentType: "",
modelOrOrderNum: "X1",//车型/订单号
num: 1,
}
//接口请求(此处可用自己的请求方法)
api.qoeShield.getEquipment(param).then((res) => {
if (res.code == 200) {
this.transferInfo.leftEqpData = res.data;
this.transferInfo_equipment = JSON.parse(JSON.stringify(this.transferInfo.leftEqpData))
this.transferInfo_equipmentL = JSON.parse(JSON.stringify(this.transferInfo.leftEqpData))
} else {
this.transferInfo.leftEqpData = []
this.transferInfo_equipment = []
this.transferInfo_equipmentL = []
}
});
},
//左侧复选框勾选
handleSelectionChange_left (val) {
this.transferInfo.multipleSelection_left = val
},
//右侧复选框勾选
handleSelectionChange_right (val) {
this.transferInfo.multipleSelection_right = val
},
//设备模糊查询 L:左侧查询;R:右侧查询
eqpSearch (type) {
let allData = []
if (type == 'L') {
allData = JSON.parse(JSON.stringify(this.transferInfo_equipmentL))
this.transferInfo.leftEqpData = this.searchResult(this.transferInfo.transferLeftSearch, allData)
} else {
allData = JSON.parse(JSON.stringify(this.allDataR))
if (this.transferInfo.transferRightSearch && this.transferInfo.transferRightSearch !== '' && this.transferInfo.transferRightSearch != null) {
this.transferInfo.rihghtEqpData = this.searchResult(this.transferInfo.transferRightSearch, allData)
} else {
let map = new Map();
for (let item of this.allDataR) {
if (!map.has(item.id)) {
map.set(item.id, item);
};
};
this.transferInfo.rihghtEqpData = [...map.values()];
}
}
},
//搜索
searchResult (text, data) {
if (text) {
return data.filter((item) => { return item.equipmentName.indexOf(text) > -1 || item.equipmentId.indexOf(text) > -1 })
} else {
return data;
}
},
//新增
addEqp () {
if (this.transferInfo.multipleSelection_left == 0) return
//合并两个数组
this.transferInfo.rihghtEqpData.push(...this.transferInfo.multipleSelection_left)
//去重
// this.transferInfo.rihghtEqpData = Array.from(new Set(this.transferInfo.rihghtEqpData))
//左侧数据移除右侧中的数据
let equpmentAll = JSON.parse(JSON.stringify(this.transferInfo_equipment))
// let equpmentR = JSON.parse(JSON.stringify(this.transferInfo.rihghtEqpData))
this.allDataR.push(...this.transferInfo.multipleSelection_left)
let eqpArrR = this.allDataR
this.$nextTick(() => {
equpmentAll = equpmentAll.filter((x) =>
!eqpArrR.some((i) => i.id == x.id)
);
this.transferInfo.leftEqpData = equpmentAll
this.transferInfo_equipmentL = JSON.parse(JSON.stringify(this.transferInfo.leftEqpData))
this.transferInfo_equipmentR = JSON.parse(JSON.stringify(this.transferInfo.rihghtEqpData))
this.eqpSearch("L")
})
},
//移除
removeEqp () {
if (this.transferInfo.multipleSelection_right == 0) return
//右侧数据移除
let selectData = JSON.parse(JSON.stringify(this.transferInfo.multipleSelection_right))//右侧选择的数据
let equpmentR = JSON.parse(JSON.stringify(this.transferInfo.rihghtEqpData))
equpmentR = equpmentR.filter((x) =>
!selectData.some((i) => i.id == x.id)
);
this.allDataR = this.allDataR.filter((x) =>
!selectData.some((i) => i.id == x.id)
);
//左侧数据=全部数据-右侧数据
this.transferInfo.rihghtEqpData = equpmentR
//被移除数据还原到左侧中
let equpmentAll = JSON.parse(JSON.stringify(this.transferInfo_equipment))
this.$nextTick(() => {
let arrEqp = equpmentAll.filter((x) =>
!this.allDataR.some((i) => i.id == x.id)
);
this.transferInfo.leftEqpData = arrEqp
this.transferInfo_equipmentL = JSON.parse(JSON.stringify(this.transferInfo.leftEqpData))
this.transferInfo_equipmentR = JSON.parse(JSON.stringify(this.transferInfo.rihghtEqpData))
})
},
//数据回显
getRowInfo () {
this.getAllEqpList()
//回显数据可根据自己情况请求接口
let selectedDataId = ["46", "1544924280746176514", "1544924344650592257"]//被选择数据,右侧数据
//回显
let equpmentAll = JSON.parse(JSON.stringify(this.transferInfo_equipment))//获取所有数据源
let equpmentR = []
for (let i = 0; i < selectedDataId.length; i++) {
for (let j = 0; j < equpmentAll.length; j++) {
if (equpmentAll[j].id == selectedDataId[i]) {
equpmentR.push(equpmentAll[j])
equpmentAll.splice(j, 1)
}
}
}
this.transferInfo.leftEqpData = equpmentAll
this.transferInfo.rihghtEqpData = equpmentR
this.transferInfo_equipmentL = JSON.parse(JSON.stringify(this.transferInfo.leftEqpData))
this.transferInfo_equipmentR = JSON.parse(JSON.stringify(this.transferInfo.rihghtEqpData))
this.allDataR = JSON.parse(JSON.stringify(this.transferInfo_equipmentR))
},
}
};
</script>
样式
<style lang="less" >
.qoeShield {
display: flex;
background: #fff;
height: 100%;
padding: 10px;
.transferbox {
height: 430px;
border: 3px solid #dcdfe6;
padding: 5px;
.topbox {
margin: 10px 10px;
}
.searchbox {
display: flex;
margin: 0 0 10px 10px;
.el-button {
margin-left: 20px;
}
}
}
.centerSearchBox {
display: flex;
flex-direction: column;
justify-content: space-around;
width: 80px;
height: 400px;
padding: 10px 5px;
color: #ffff;
.el-button + .el-button {
margin-left: 0 !important;
.icon {
font-size: 14px !important;
}
}
}
}
</style>
更多推荐
所有评论(0)