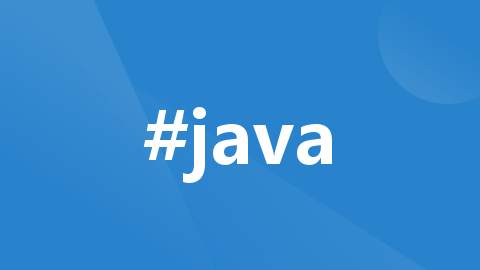
springboot配置前后端RSA非对称加密
3.前端页面引用jsencrypt.js(vue需要执行npm install jsencrypt安装,js文件获取则可以利用vue项目npm install安装后在本地modules里找到对应的js文件拷贝到自己的项目里引用)5.RSA解密,登录密码校验逻辑之前对密码进行解密(根据数据库存储密码的加密格式需要转换为对应的,方便后面校验)4.RSA加密,js登录请求前用公钥对密码进行加密。1.RS
·
1.RSA公钥私钥生成工具类
/**
* RSA密钥对生成工具类
*/
public class KeyPairGeneratorUtil {
public static KeyPair generateRSAKeyPair() {
try{
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
return keyPair;
}catch (Exception e) {
e.printStackTrace();
return null;
}
}
public static PublicKey getPublicKey(KeyPair keyPair) {
return keyPair.getPublic();
}
public static PrivateKey getPrivateKey(KeyPair keyPair) {
return keyPair.getPrivate();
}
}
2.RSA公钥后台请求接口
@RestController
public class RsaController {
private static KeyPair keyPair = KeyPairGeneratorUtil.generateRSAKeyPair();
//私钥
public static PrivateKey privateKey = KeyPairGeneratorUtil.getPrivateKey(keyPair);
//公钥
private static String publicKeyString = Base64.getEncoder().encodeToString(keyPair.getPublic().getEncoded());
@RequestMapping("/getPublicKey")
public Map<String,Object> getPublicKeyBase64(){
Map<String,Object> ret = new HashMap<>();
ret.put("publicKey",publicKeyString);
return ret;
}
}
3.前端页面引用jsencrypt.js(vue需要执行npm install jsencrypt安装,js文件获取则可以利用vue项目npm install安装后在本地modules里找到对应的js文件拷贝到自己的项目里引用)
4.RSA加密,js登录请求前用公钥对密码进行加密
//获取公钥
$.ajax({
url : '/getPublicKey',
type : 'POST',
dataType : 'json',
data : { },
success : function (data, status, xhr) {
let pass = $.trim($("#password").val());
pwdcache = pass;
let publicKey = data.publicKey;
console.log(data.publicKey)
// 获取密码对象
let encryptor = new JSEncrypt();
// 放入公钥
encryptor.setPublicKey(publicKey)
// 放入加密的内容并加密
let passByPublicKey = encryptor.encrypt(pass);
// 加密后的密文
$("#password").val(passByPublicKey);
$("#login").click();
},
error : function () {
}
});
5.RSA解密,登录密码校验逻辑之前对密码进行解密(根据数据库存储密码的加密格式需要转换为对应的,方便后面校验)
public String decrypt(String encryptedData) {
byte[] decodedData = java.util.Base64.getDecoder().decode(encryptedData);
try {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedData = cipher.doFinal(decodedData);
return new String(decryptedData);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
更多推荐
所有评论(0)