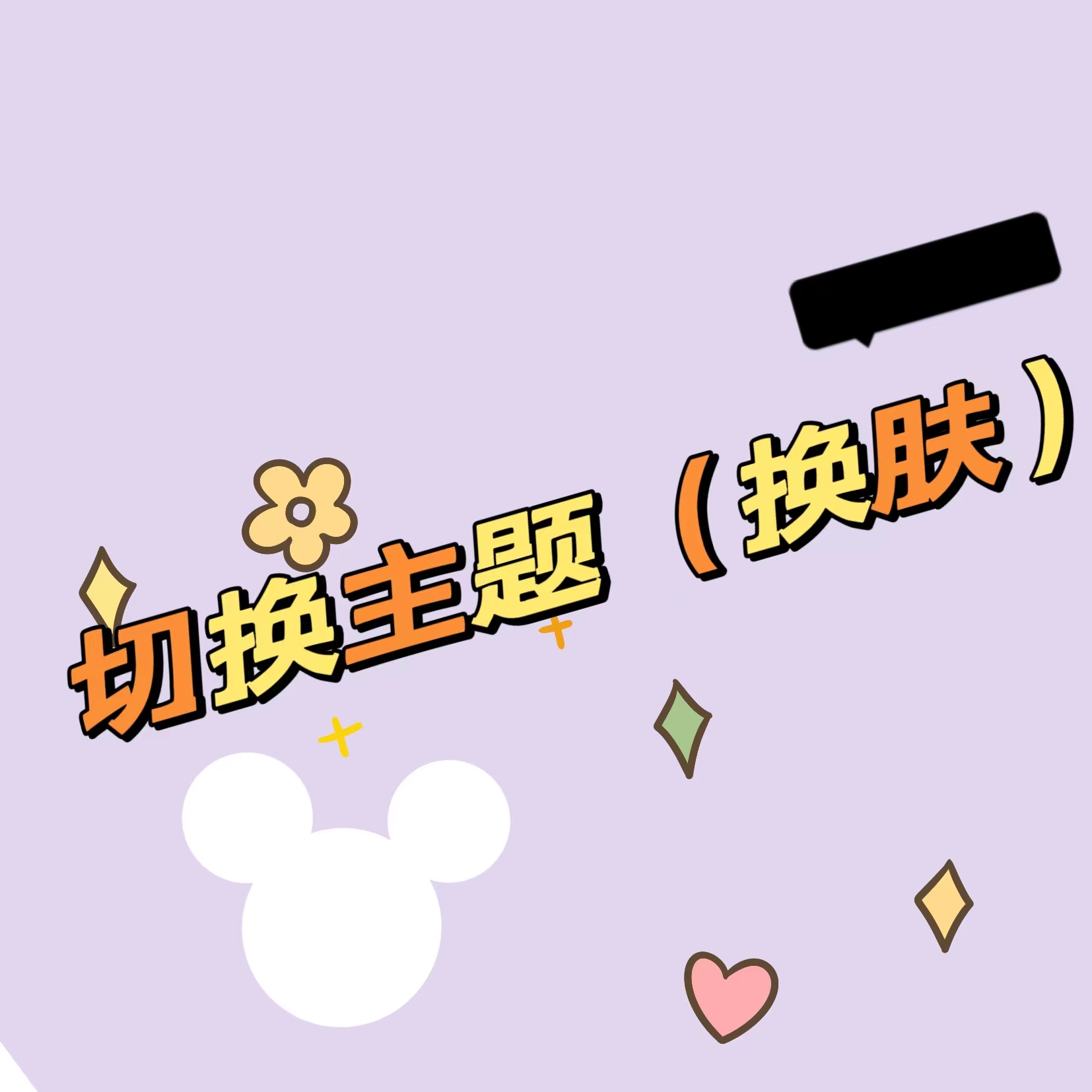
vue切换不同主题(换肤)
vue切换不同主题风格
文章目录
主题换肤
方法一:css3 var()方法
一,var定义与基础用法
1、定义
var() 函数用于插入自定义的属性值,如果一个属性值在多处被使用,该方法就很有用 。
var(custom-property-name, value)
值 | 描述 |
---|---|
custom-property-name | 必需。自定义属性的名称,必需以 – 开头。 |
value | 可选。备用值,在属性不存在的时候使用。 |
2、基础用法
先定义不同属性值,之后再使用。在具体项目中可以区分属性定义文件和使用文件,便于管理。
注意:var中的自定义属性名称必须以–开头
:root {
--main-bg-color: coral;
--main-txt-color: blue;
--main-padding: 15px;
}
#div1 {
background-color: var(--main-bg-color);
color: var(--main-txt-color);
padding: var(--main-padding);
}
二、项目中使用
项目中需要切换三个主题(浅蓝色、白色、深蓝色),通过按钮切换不同主题。使用进行vuex管理。
下面的使用方法是我在项目中使用的,使用的是var(),具体切换切换过程可以自行调整。
1、定义属性
定义三种不同主题,相同区域不同的样式
individual.js文件
module.exports = {
themeLight: {
// 工作区表格头字体颜色
tableHeadFontColor: '#696969',
// 工作区表格头背景颜色
tableHeadBgColor: '#87CEFA',
},
themeDark: {
// 工作区表格头字体颜色
tableHeadFontColor: '#fff',
// 工作区表格头背景颜色
tableHeadBgColor: '#000080',
},
themeWhite: {
// 工作区表格头字体颜色
tableHeadFontColor: '#000000',
// 工作区表格头背景颜色
tableHeadBgColor: '#fff',
}
}
2、设置样式
修改el-table样式,想要修改哪个样式就给谁设置
index.scss文件
不要忘记需要把index.scss样式文件在main.js中引入
.table {
.el-table th.el-table__cell > .cell {
color: var(--table-head-font-color);
}
.el-table .el-table__header-wrapper th,
.el-table .el-table__fixed-header-wrapper th {
background: var(--table-head-bg-color);
}
}
3、具体给谁的样式
<el-table
class="table"
:data="tableData"
stripe
:style="{'--table-head-font-color': individual.tableHeadFontColor,'--table-head-bg-color': individual.tableHeadBgColor}"
>
<el-table-column
prop="date"
label="日期"
width="180">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
width="180">
</el-table-column>
<el-table-column
prop="address"
label="地址">
</el-table-column>
</el-table>
4、切换函数
1、若依框架store文件夹中seeting.js文件,如果不是使用若依框架可以自己定义js文件
import individual from '@/individual' //引入定义的属性
const { themeDark, themeLight, themeWhite } = themes //三种不同主题
const storageSetting = JSON.parse(localStorage.getItem('layout-setting')) || ''
const state = {
sideTheme: storageSetting.sideTheme || sideTheme,
individual: {
// 工作区表格头字体颜色
tableHeadFontColor: storageSetting.individual === undefined ? themeDark.tableHeadFontColor : storageSetting.individual.tableHeadFontColor,
// 工作区表格头背景颜色
tableHeadBgColor: storageSetting.individual === undefined ? themeDark.tableHeadBgColor : storageSetting.individual.tableHeadBgColor,
}
}
const mutations = {
CHANGE_SETTING: (state, { key, value }) => {
if (state.hasOwnProperty(key)) {
state[key] = value
if (key === 'sideTheme') {
if (value === 'theme-dark') {
state.individual= themeDark
}
else if (value === 'theme-light') {
state.individual= themeLight
}
else if (value === 'theme-white') {
state.individual= themeWhite
}
}
}
},
CHANGE_INDIVIDUAL: (state, data) => {
state.individual = data
}
}
const actions = {
// 个性化修改
changeThemes({ commit }, data) {
commit('CHANGE_INDIVIDUAL', data)
},
}
export default {
namespaced: true,
state,
mutations,
actions
}
2、切换按钮
<div @click="handleTheme('theme-dark')">深蓝色</div>
<div @click="handleTheme('theme-light')">浅蓝色</div>
<div @click="handleTheme('theme-white')">白色</div>
3、切换函数
点击按钮传递不同主题参数,设置主题
computed: {
individual: {
get() {
return this.$store.state.settings.individual;
},
},
}
methods: {
handleTheme(val) {
this.$store.dispatch("settings/changeSetting", {
key: "sideTheme",
value: val,
});
this.sideTheme = val;
},
}
方法二 sass
设置两个主题的样式,通过按钮传递主题参数,进行切换主题
一、文件分类
在assets中创建themes文件夹
dark为深颜色主题,tint为浅颜色主题。(element-variables.scss样式属性定义,styls.scss样式定义)
二、使用
1、定义样式属性
(1)、分别在dark与tint中定义样式
dark文件夹中element-variables.scss
$--background-color-base: rgb(9, 9, 53); // 整体背景颜色
dark文件夹中styls.scss
@import "./element-variables.scss"; //引入样式属性定义
$theme-dark:(
background-base: $--background-color-base,
);
tint文件夹中element-variables.scss
$--background-color-base: #e0f2fde2; // 整体背景颜色
dark文件夹中styls.scss
@import "./element-variables.scss"; //引入样式属性定义
$theme-dark:(
background-base: $--background-color-base,
);
(2)
themes.scss
themes文件夹下创建thems.css文件 把两个主题都引入过来,并定义好主题对象themes
@import "dark/styls.scss";
@import "tint/styls.scss";
$themes: (
theme-dark: $theme-dark,
theme-tint: $theme-tint
)
(3)
handle.scss
之后把themes.scss引入到handle.scss,使用我们定义好的themes对象
handle.scss文件主要 编写对 themes对象的处理和一些方法,
//导入两大主题
@import "themes.scss";
//遍历主题map
@mixin themeify {
@each $theme-name, $theme-map in $themes {
//!global 把局部变量强升为全局变量
$theme-map: $theme-map !global;
//判断html的data-theme的属性值 #{}是sass的插值表达式
//& sass嵌套里的父容器标识 @content是混合器插槽,像vue的slot
// @if $theme-name == default {
// & {
// @content;
// }
// } @else {
[data-theme="#{$theme-name}"] & {
@content;
}
// }
}
}
@function themed($key) {
@return map-get($theme-map, $key);
}
@import "common.scss";
@mixin 定义了一个混合器 themeify 并定义了一个函数themed且返回值是对应主题下的对应变量的值。
由于对具体元素的样式设置都放在了common.scss里面,因此把他放在最后引入,否则报错(主题还未设置好,相当于themes和themed都undefine)
(4)
common.scss
所有需要根据主题调整样式的元素都写在这里面,我们只根据主题改变了class="app-main"的元素的background,调用混合器之后(即在@include themeyfy{}里面)即可编写样式
新的元素按照同样的方式 调用混合器themeify, 使用themed函数设置同名变量
.app-main {
@include themeify{
background:themed("background-base");
}
}
给class=“app-main"的元素设置background,采用的是在dark.scss和tint.scss下我们配置好的同名变量"background-base” 此名称可改变,但要在dark.scss和tint.scss以及此处保持一致,否则报错。
(5)引入
App.vue中
特别注意 这里要写上lang=“scss”
<style lang="scss">
@import 'assets/theme/handle.scss'
</style>
2、切换函数
<div class="box light" @click="setTheme('tint')">浅色</div>
<div class="box light" @click="setTheme('dark')">深色</div>
handleTheme(val) {
window.document.documentElement.setAttribute("data-theme", val)
}
一开始我们没有设置主题,而是点击按钮之后才开始设置和切换主题,你也可以直接在created生命周期内调用handleTheme()方法设置主题,即为你的项目默认主题。
两种切换主题放大大致思路:定义属性——给谁用样式属性——点击切换
更多推荐
所有评论(0)