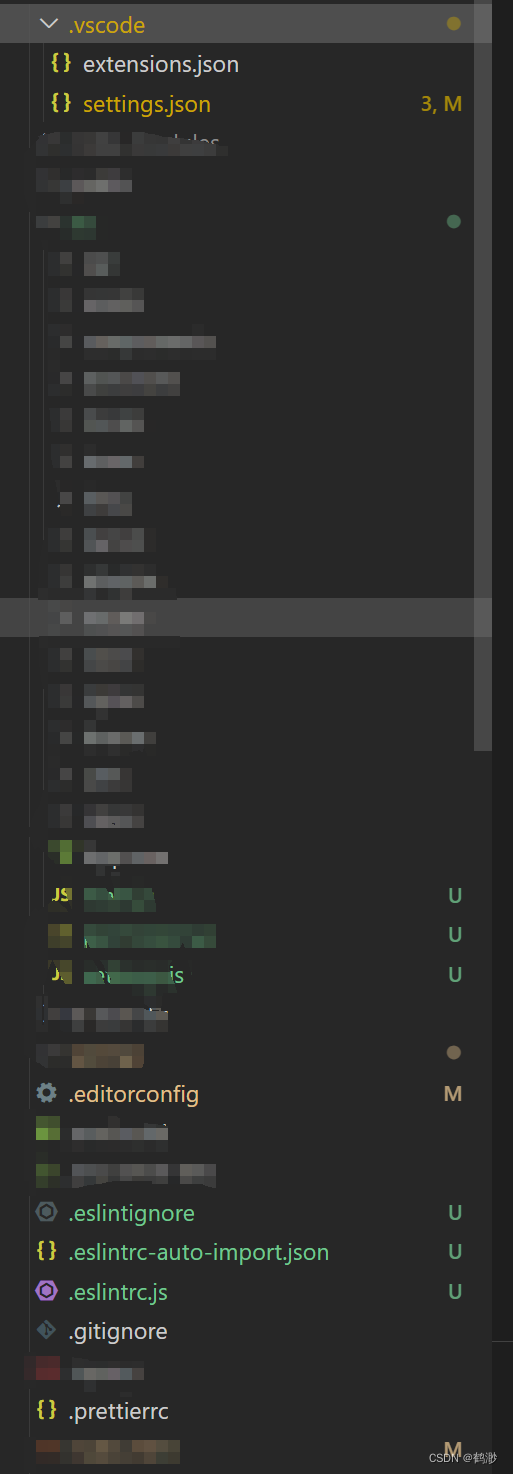
VScode 工作区配置 和 用户配置
这里主要介绍自己使用vscode常用的一些配置项和根据不同的项目来配置代码规范的一些记录

一、工作区配置
通常不同的项目都有不同的配置,我一般都是使用eslint和prettier一起用,所以经常会有这几个文件:
这里简单介绍一下这几个文件的作用吧。
1.vscode文件夹下
一般有两个文件,extensions.json和settings.json。
extensions.json
文件是用来配置推荐安装的 VS Code 插件的文件。在这个文件中,你可以列出你项目中推荐使用的一些插件,当你打开项目时,VS Code 会自动提示你是否安装这些插件。
比如:
{
"recommendations": ["johnsoncodehk.volar", "esbenp.prettier-vscode","dbaeumer.vscode-eslint"]
}
这三个插件分别代表:
johnsoncodehk.volar
: 与 Vue.js 相关的插件,提供了更好的 Vue.js 开发体验。
esbenp.prettier-vscode
: Prettier 的 VS Code 插件,用于格式化代码。
dbaeumer.vscode-eslint
: ESLint 的 VS Code 插件,用于提供 ESLint 集成支持。
settings.json
这是VS Code 中的设置文件一般用来设置项目的开发配置,比如常见的都是这些配置:
{
"window.title": "Admin", //设置 VS Code 窗口标题
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.detectIndentation": false,
"editor.formatOnPaste": false,
"editor.formatOnSave": true,
"editor.wordWrap": "on", //启用文本换行
"eslint.alwaysShowStatus": true,
"eslint.validate": [
"javascript",
"javascriptreact",
"vue",
"html",
{
"language": "vue",
"autoFix": true
}
],
"[javascript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[jsonc]": {
"editor.defaultFormatter": "HookyQR.beautify"
},
"[html]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[vue]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"editor.tabSize": 2,
"diffEditor.ignoreTrimWhitespace": false,
"files.associations": {
"*.vue": "vue"
},
"editor.suggestSelection": "first",
"vsintellicode.modify.editor.suggestSelection": "automaticallyOverrodeDefaultValue",
"prettier.tabWidth": 2,//指定 Prettier 的缩进宽度为 2 个空格
"prettier.semi": false,
"prettier.jsxSingleQuote": true,
"prettier.singleQuote": true,
"prettier.eslintIntegration": true, // 让prettier遵循eslint格式美化
// "[json]": {
// "editor.defaultFormatter": "esbenp.prettier-vscode"
// },
"files.exclude": {
// ".dockerignore": true,
// ".editorconfig": true,
// ".eslint*": true,
// ".travis*": true,
// "babel*": true,
// "package-lock*": true,
// "postcss*": true,
// "nginx*": true,
// ".git*": true,
// ".pretti*": true,
// ".vscode": true,
// ".stylelintrc.json": true,
// "*.md": true,
// "*.toml": true,
// "*firebase*": true,
// "appveyor.yml": true,
// "dist": true,
// "Dock*": true,
// "jest*": true,
// "node_modules": true,
// "README*": true
},
"javascript.format.enable": true, // 不启动JavaScript格式化
"files.autoSave": "onFocusChange",
"eslint.options": {
// "plugins": ["html"]
},
// "workbench.statusBar.feedback.visible": false,
"vetur.format.options.tabSize": 2, //Vue 文件中的缩进大小
"vetur.format.defaultFormatter.css": "prettier",
"merge-conflict.autoNavigateNextConflict.enabled": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "explicit" //保存时执行 ESLint 修复
},
"[scss]": {
"editor.defaultFormatter": "HookyQR.beautify"
},
"prettier.requireConfig": true,
"editor.guides.indentation": false,
"editor.guides.highlightActiveIndentation": false,
"workbench.editor.empty.hint": "hidden" //隐藏空白编辑器的提示,可以根据个人偏好进行配置
}
一般都是需要什么配置去找什么配置,当前只有这两个还是不太行,一般还需要其他的文件来辅助。
2.根目录下
eslintrc.js 和 prettierrc ,看名字就知道都是干啥的,一般会有这样的配置:
.eslintrc.js
module.exports = {
root: true,
env: {
browser: true,
commonjs: true,
es6: true,
node: true
},
globals: {
defineEmits: true,
document: true,
localStorage: true,
GLOBAL_VAR: true,
window: true,
defineProps: true,
defineExpose: true,
$ref: true
},
plugins: ['@typescript-eslint', 'prettier', 'import'],
extends: [
'eslint:recommended',
'plugin:@typescript-eslint/recommended',
'plugin:vue/vue3-recommended',
'prettier',
'./.eslintrc-auto-import.json'
],
parserOptions: {
parser: '@typescript-eslint/parser',
sourceType: 'module',
ecmaFeatures: {
jsx: true,
tsx: true
}
},
rules: {
//close lf error
'import/no-unresolved': [0],
'vue/multi-word-component-names': 'off',
'vue/no-deprecated-router-link-tag-prop': 'off',
'import/extensions': 'off',
'import/no-absolute-path': 'off',
'no-async-promise-executor': 'off',
'import/no-extraneous-dependencies': 'off',
'vue/no-multiple-template-root': 'off',
'vue/html-self-closing': 'off',
'no-console': 'off',
'no-plusplus': 'off',
'no-useless-escape': 'off',
'no-bitwise': 'off',
'@typescript-eslint/no-explicit-any': ['off'],
'@typescript-eslint/explicit-module-boundary-types': ['off'],
'@typescript-eslint/ban-ts-comment': ['off'],
'vue/no-setup-props-destructure': ['off'],
'@typescript-eslint/no-empty-function': ['off'],
'vue/script-setup-uses-vars': ['off'],
//can config to 2 if need more then required
'@typescript-eslint/no-unused-vars': [0],
'no-param-reassign': ['off']
}
}
解释如下:
root: true
: 表示 ESLint 应该停止在父级目录中查找其他配置文件。
env
: 定义了代码运行的环境。这里包括浏览器、CommonJS、ES6 和 Node.js。
globals
: 定义了全局变量,这样 ESLint 不会发出未定义的错误。这里列出了一些常见的全局变量,比如document
、localStorage
、window
等。
plugins
: 插件列表,这里包括@typescript-eslint
、prettier
和import
。这些插件提供了额外的规则和功能。
extends
: 继承了一系列预设的规则集,包括了一些推荐的规则,TypeScript 相关的规则,Vue3 推荐的规则,以及 Prettier 的规则。
parserOptions
: 定义了解析器选项,这里使用了 TypeScript 解析器@typescript-eslint/parser
,并设置了一些选项,如支持 JSX 和 TSX。
rules
: 定义了具体的规则配置。这里关闭了一些规则,例如关闭了一些 import 相关的规则、关闭了一些 TypeScript 相关的规则、关闭了一些 Vue 相关的规则等。每个规则的具体含义可以根据规则的名称查找 ESLint 文档进行了解
.prettierrc
{
"useTabs": false,
"tabWidth": 2,
"printWidth": 120,
"singleQuote": true,
"trailingComma": "none",
"bracketSpacing": true,
"semi": false,
"htmlWhitespaceSensitivity": "ignore"
}
useTabs
: 表示是否使用制表符(Tab)进行缩进。如果设置为false
,则使用空格进行缩进。
tabWidth
: 表示缩进的空格数目。如果使用空格进行缩进,定义每级缩进的空格数。
printWidth
: 表示代码行的最大宽度,超过这个宽度则进行换行。
singleQuote
: 表示是否使用单引号。如果设置为true
,则使用单引号;如果设置为false
,则使用双引号。
trailingComma
: 表示对象、数组等最后一个元素后是否加逗号。可选值为"none"
、"es5"
、"all"
。
bracketSpacing
: 表示花括号是否有空格。如果设置为true
,则花括号内部有空格。
semi
: 表示是否使用分号作为语句的结束符。如果设置为false
,则不使用分号。
htmlWhitespaceSensitivity
: 表示 HTML 文件中空格的敏感性。可选值为"css"
(保留 CSS 规则的空格)和"ignore"
(忽略空格)。这些配置项用于规范代码风格,确保团队成员之间的代码风格一致。 Prettier 会根据这些配置对代码进行格式化。
除此之外,还可以引入这些配置:
.eslintrc-auto-import.json
定义全局变量,以避免 ESLint 报告这些变量未定义的错误
比如:
{
"globals": {
"axiosReq": true,
"computed": true,
"createApp": true,
"createLogger": true,
"createNamespacedHelpers": true,
"createStore": true,
"customRef": true,
"defineAsyncComponent": true,
"defineComponent": true,
"effectScope": true,
"EffectScope": true,
"getCurrentInstance": true,
"getCurrentScope": true,
"h": true,
"inject": true,
"isReadonly": true,
"isRef": true,
"mapActions": true,
"mapGetters": true,
"mapMutations": true,
"mapState": true,
"markRaw": true,
"nextTick": true,
"onActivated": true,
"onBeforeMount": true,
"onBeforeUnmount": true,
"onBeforeUpdate": true,
"onDeactivated": true,
"onErrorCaptured": true,
"onMounted": true,
"onRenderTracked": true,
"onRenderTriggered": true,
"onScopeDispose": true,
"onServerPrefetch": true,
"onUnmounted": true,
"onUpdated": true,
"provide": true,
"reactive": true,
"readonly": true,
"ref": true,
"resolveComponent": true,
"shallowReactive": true,
"shallowReadonly": true,
"shallowRef": true,
"toRaw": true,
"toRef": true,
"toRefs": true,
"triggerRef": true,
"unref": true,
"useAttrs": true,
"useCommon": true,
"useCssModule": true,
"useCssVars": true,
"useElement": true,
"useRoute": true,
"useRouter": true,
"useSlots": true,
"useStore": true,
"useVueRouter": true,
"watch": true,
"watchEffect": true
}
}
这些变量看起来是与 Vue 3 和 Vue 相关的一些功能和 API 相关的,包括
ref
、reactive
、computed
、provide
、watch
、watchEffect
等。通过将这些变量添加到
globals
字段中,告诉 ESLint 这些变量是全局可用的,不需要在代码中显式声明或导入。这种做法有助于提高开发效率,同时确保代码中使用的全局变量能够被正确地识别和检查,而不会导致未定义的错误。
.eslintignore
用于配置 ESLint 忽略检查的文件和目录。
public
node_modules
.history
.husky
src/static
src/components/*
src/uni_modules/*
src/utils/*
dist
axios
*.d.ts
public: 忽略 public 目录下的文件和子目录。
node_modules: 忽略 node_modules 目录下的文件和子目录。
.history: 忽略 .history 目录下的文件和子目录。
.husky: 忽略 .husky 目录下的文件和子目录。
src/static: 忽略 src/static 目录下的文件和子目录。
src/components/*: 忽略 src/components 目录下的所有文件。
src/uni_modules/*: 忽略 src/uni_modules 目录下的所有文件。
src/utils/*: 忽略 src/utils 目录下的所有文件。
dist: 忽略 dist 目录下的文件和子目录。
axios: 忽略 axios 文件。
*.d.ts: 忽略以 .d.ts 结尾的文件。
这些规则用于告诉 ESLint 在检查代码时忽略这些文件和目录,通常用于排除一些不需要进行代码检查的文件或者由工具生成的文件。
.editorconfig
用于配置代码编辑器的行为,以确保在不同编辑器中保持一致的代码风格
# https://editorconfig.org
root = true
[*]
charset = utf-8
indent_style = space
indent_size = 2
end_of_line = lf
insert_final_newline = true
trim_trailing_whitespace = true
[*.md]
insert_final_newline = false
trim_trailing_whitespace = false
root = true
: 表示这是最顶层的.editorconfig
文件,编辑器在查找.editorconfig
文件时将停止搜索。
[*]
: 适用于所有文件的默认配置。
charset = utf-8
: 文件编码使用 UTF-8。indent_style = space
: 缩进使用空格。indent_size = 2
: 缩进大小为 2 个空格。end_of_line = lf
: 换行符使用 LF (Unix 风格)。insert_final_newline = true
: 在文件的末尾插入一个空白行。trim_trailing_whitespace = true
: 去除行尾的空格。
[*.md]
: 适用于 Markdown 文件的配置。
insert_final_newline = false
: 不在 Markdown 文件的末尾插入空白行。trim_trailing_whitespace = false
: 不去除 Markdown 文件行尾的空格。这些设置旨在提供一致的编辑器配置,以避免因编辑器不同而引起的格式化问题。例如,它确保所有文件都使用相同的字符集、缩进风格等。
上面这些是不用的工作区,或者说是不同的项目之间的配置,下面是自己的vscode的一些配置,当然如果有上面的配置,用户配置只是补充
二、用户配置
点击设置,随便搜点啥,点击这个链接就到了用户设置里,当然也可以根据此来进行工作区的设置,这里有中文,比较方便。
{
// 添加 vue 支持
"eslint.validate": [
"javascript",
"javascriptreact",
{
"language": "vue",
"autoFix": false
}
],
// //火花组件的配置
// "powermode.enabled": true, //启动
// "powermode.presets": "flames", // 火花效果
// "powermode.enableShake": true, // 去除代码抖动
// "powermode.shake.enabled": false,
// "powermode.combo.counterEnabled": "hide",
// "powermode.combo.timerEnabled": "hide",
// #这个按用户自身习惯选择
"vetur.format.defaultFormatter.html": "js-beautify-html",
// #让vue中的js按编辑器自带的ts格式进行格式化
"vetur.format.defaultFormatter.js": "vscode-typescript",
"vetur.format.defaultFormatterOptions": {
"js-beautify-html": {
"wrap_attributes": "force-aligned"
// #vue组件中html代码格式化样式
}
},
// // 格式化stylus, 需安装Manta's Stylus Supremacy插件
// "stylusSupremacy.insertColons": false, // 是否插入冒号
// "stylusSupremacy.insertSemicolons": false, // 是否插入分好
// "stylusSupremacy.insertBraces": false, // 是否插入大括号
// "stylusSupremacy.insertNewLineAroundImports": false, // import之后是否换行
// "stylusSupremacy.insertNewLineAroundBlocks": false,
//代码格式化
// vscode默认启用了根据文件类型自动设置tabsize的选项
"editor.detectIndentation": false,
// 重新设定tabsize
"editor.tabSize": 2,
// #每次保存的时候自动格式化
"editor.formatOnSave": true,
"files.autoSave": "onFocusChange",
"files.autoSaveDelay": 3000,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "never"
},
"editor.semanticTokenColorCustomizations": {
"[Default Dark+]": {}
},
// #使用带引号替代双引号
// "prettier.singleQuote": false,
// #让函数(名)和后面的括号之间加个空格
"javascript.format.insertSpaceBeforeFunctionParenthesis": true,
// "tabnine.experimentalAutoImports": true,
// "emmet.preferences": {},
// "prettier.jsxSingleQuote": false,
// "html.completion.attributeDefaultValue": "singlequotes",
// "[vue]": {
// "editor.defaultFormatter": "esbenp.prettier-vscode"
// },
//关闭vetur标签闭合检查(用于解决iview标签报错)
"explorer.confirmDelete": true,
//auto Rename tag
"editor.linkedEditing": true,
//auto close tags
"html.autoClosingTags": true,
"javascript.autoClosingTags": true,
"typescript.autoClosingTags": true,
//auto import 为JavaScript//TypeScript使用auto-import suggestions,.在文件移动时更新导入,并在top使用绝对路径组织导入。
"javascript.suggest.autoImports": true,
"typescript.suggest.autoImports": true,
"javascript.updateImportsOnFileMove.enabled": "always",
"typescript.updateImportsOnFileMove.enabled": "always",
//括号颜色和水平
"editor.bracketPairColorization.independentColorPoolPerBracketType": true,
"editor.bracketPairColorization.enabled": true,
"editor.guides.bracketPairs": true,
"cSpell.userWords": ["pview"],
"editor.accessibilitySupport": "off",
"merge-conflict.autoNavigateNextConflict.enabled": true,
"volar.autoCompleteRefs": true,
"cSpell.languageSettings": [],
"prettier.semi": false,
"tabnine.experimentalAutoImports": true,
"workbench.editorAssociations": {
"*.ttf": "default"
},
"prettier.singleQuote": true,
"prettier.jsxSingleQuote": true,
"workbench.colorTheme": "Monokai Dimmed",
"C_Cpp.commentContinuationPatterns": ["/**"],
"git.suggestSmartCommit": false,
"git.confirmSync": false,
"window.zoomLevel": 1,
"editor.fontVariations": false
}
我上面的配置是自己常用的,可以参考,基本每一个配置都有对应的中文解释,可以很方便的看懂。
over~
更多推荐
所有评论(0)