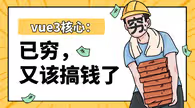
vben admin配置详解(Table, Form)
vben这个后台管理系统的框架,基于ant-design-vue组件库封装了很多好用的组件,我们在日常开发中用的最多的就是Table, Form组件了。下面就简单介绍一下。
vben这个后台管理系统的框架,基于ant-design-vue组件库封装了很多好用的组件,我们在日常开发中用的最多的就是Table, Form组件了。下面就简单介绍一下。
监听菜单折叠
const { getCollapsed } = useMenuSetting()
const isCollapsed = ref(false)
watch(
getCollapsed,
(val) => {
isCollapsed.value = val
},
{ immediate: true },
)
获取当前语言类型
获取当前语言类型,然后做一些事情。比如设置不同的样式,调整UI。
import { useLocaleStoreWithOut } from '/@/store/modules/locale'
// 获取当前语言
const localeStore = useLocaleStoreWithOut()
const locale = localeStore.getLocale
const localeClass = computed(() => {
return locale === 'en' ? 'en-item mrb-15' : 'item mrb-15'
})
或者这种
import { useLocale } from '/@/locales/useLocale'
const { getAntdLocale } = useLocale()
const locale = getAntdLocale.value.locale
监听语言切换做UI处理
import { useLocaleStoreWithOut } from '/@/store/modules/locale';
const localeStore = useLocaleStoreWithOut();
const locale = localeStore.getLocale;
export default function isEnLocale() {
return locale === 'en';
}
权限设置
多个权限是或者的关系。
-
v-auth指令,指定唯一值。内部也是调用
hasPermission
去做判断的。不可以动态添加判断。 -
hasPermission
动态添加去做判断。结合v-if, v-show
做显示隐藏。
import { usePermission } from '/@/hooks/web/usePermission';
const { hasPermission } = usePermission();
if (!hasPermission(['xxx1', 'xxx2'])) {
createMessage.info('暂无查看权限! ');
return;
}
开启页面缓存。
vben全局注册组件
-
input
-
layout
-
button-group
- button (vben自己封装的) 组件名称
a-button
-
preIcon,前置图标
-
postIcon后置图标 内部也提供默认插槽。
-
<a-button> <plus-outlined /> 新增 </a-button>
useTable返回的配置对象
setLoading,
setColumns,
getColumns,
getDataSource,
getRawDataSource,
reload,
getPaginationRef,
setPagination,
getSelectRows,
getSelectRowKeys,
setSelectedRowKeys,
clearSelectedRowKeys,
setProps,
redoHeight,
setTableData,
deleteSelectRowByKey,
updateTableData,
deleteTableDataRecord,
insertTableDataRecord,
updateTableDataRecord,
getRowSelection,
getForm,
setShowPagination,
getShowPagination,
expandAll,
collapseAll,
reload
参数类型
export interface FetchParams {
searchInfo?: Recordable;
page?: number;
sortInfo?: Recordable;
filterInfo?: Recordable;
}
searchInfo、filterInfo、sortInfo内容会被结构出来,放在同一层传递出去。目的是为了更好地区分吧。
updateTableData
(index: number, key: string, value: any)
更新表格数据(单条数据单个值) 异步方法指定哪个记录更新哪个字段值。
getForm
获取搜索区域对象,做一些操作比如表单校验。
BasicTableProps useTable 参数
export interface BasicTableProps<T = any> {
clickToRowSelect?: boolean;
isTreeTable?: boolean;
sortFn?: (sortInfo: SorterResult) => any;
filterFn?: (data: Partial<Recordable<string[]>>) => any;
inset?: boolean;
showTableSetting?: boolean;
tableSetting?: TableSetting;
striped?: boolean;
autoCreateKey?: boolean;
summaryFunc?: (...arg: any) => Recordable[];
summaryData?: Recordable[];
showSummary?: boolean;
canColDrag?: boolean;
api?: (...arg: any) => Promise<any>;
beforeFetch?: Fn;
afterFetch?: Fn;
handleSearchInfoFn?: Fn;
fetchSetting?: Partial<FetchSetting>;
immediate?: boolean;
emptyDataIsShowTable?: boolean;
searchInfo?: Recordable;
* {
field: 'name',
order: 'ascend',
}
*/
defSort?: Recordable;
useSearchForm?: boolean;
formConfig?: Partial<FormProps>;
columns: BasicColumn[];
showIndexColumn?: boolean;
indexColumnProps?: BasicColumn;
* actionColumn: {
width: 100,
title: '操作',
dataIndex: 'action',
},
*/
actionColumn?: BasicColumn;
ellipsis?: boolean;
canResize?: boolean;
resizeHeightOffset?: number;
clearSelectOnPageChange?: boolean;
rowKey?: string | ((record: Recordable) => string);
dataSource?: Recordable[];
titleHelpMessage?: string | string[];
maxHeight?: number;
bordered?: boolean;
pagination?: PaginationProps | boolean;
loading?: boolean;
* The column contains children to display
* @default 'children'
* @type string | string[]
*/
childrenColumnName?: string;
* Override default table elements
* @type object
*/
components?: object;
* Expand all rows initially
* @default false
* @type boolean
*/
defaultExpandAllRows?: boolean;
* Initial expanded row keys
* @type string[]
*/
defaultExpandedRowKeys?: string[];
* Current expanded row keys
* @type string[]
*/
expandedRowKeys?: string[];
* Expanded container render for each row
* @type Function
*/
expandedRowRender?: (record?: ExpandedRowRenderRecord<T>) => VNodeChild | JSX.Element;
* Customize row expand Icon.
* @type Function | VNodeChild
*/
expandIcon?: Function | VNodeChild | JSX.Element;
* Whether to expand row by clicking anywhere in the whole row
* @default false
* @type boolean
*/
expandRowByClick?: boolean;
* The index of `expandIcon` which column will be inserted when `expandIconAsCell` is false. default 0
*/
expandIconColumnIndex?: number;
* Table footer renderer
* @type Function | VNodeChild
*/
footer?: Function | VNodeChild | JSX.Element;
* Indent size in pixels of tree data
* @default 15
* @type number
*/
indentSize?: number;
* i18n text including filter, sort, empty text, etc
* @default { filterConfirm: 'Ok', filterReset: 'Reset', emptyText: 'No Data' }
* @type object
*/
locale?: object;
* Row's className
* @type Function
*/
rowClassName?: (record: TableCustomRecord<T>, index: number) => string;
* Row selection config
* @type object
*/
rowSelection?: TableRowSelection;
* Set horizontal or vertical scrolling, can also be used to specify the width and height of the scroll area.
* It is recommended to set a number for x, if you want to set it to true,
* you need to add style .ant-table td { white-space: nowrap; }.
* @type object
*/
scroll?: { x?: number | true; y?: number };
* Whether to show table header
* @default true
* @type boolean
*/
showHeader?: boolean;
* Size of table
* @default 'default'
* @type string
*/
size?: SizeType;
* Table title renderer
* @type Function | ScopedSlot
*/
title?: VNodeChild | JSX.Element | string | ((data: Recordable) => string);
* Set props on per header row
* @type Function
*/
customHeaderRow?: (column: ColumnProps, index: number) => object;
* Set props on per row
* @type Function
*/
customRow?: (record: T, index: number) => object;
* `table-layout` attribute of table element
* `fixed` when header/columns are fixed, or using `column.ellipsis`
*
* @see https://developer.mozilla.org/en-US/docs/Web/CSS/table-layout
* @version 1.5.0
*/
tableLayout?: 'auto' | 'fixed' | string;
* the render container of dropdowns in table
* @param triggerNode
* @version 1.5.0
*/
getPopupContainer?: (triggerNode?: HTMLElement) => HTMLElement;
* Data can be changed again before rendering.
* The default configuration of general user empty data.
* You can configured globally through [ConfigProvider](https://antdv.com/components/config-provider-cn/)
*
* @version 1.5.4
*/
transformCellText?: Function;
* Callback executed before editable cell submit value, not for row-editor
*
* The cell will not submit data while callback return false
*/
beforeEditSubmit?: (data: {
record: Recordable;
index: number;
key: string | number;
value: any;
}) => Promise<any>;
* Callback executed when pagination, filters or sorter is changed
* @param pagination
* @param filters
* @param sorter
* @param currentDataSource
*/
onChange?: (pagination: any, filters: any, sorter: any, extra: any) => void;
* Callback executed when the row expand icon is clicked
*
* @param expanded
* @param record
*/
onExpand?: (expande: boolean, record: T) => void;
* Callback executed when the expanded rows change
* @param expandedRows
*/
onExpandedRowsChange?: (expandedRows: string[] | number[]) => void;
onColumnsChange?: (data: ColumnChangeParam[]) => void;
}
sortFn 排序
export interface SorterResult {
column: ColumnProps;
order: SortOrder;
field: string;
columnKey: string;
}
sortFn(sortInfo: SorterResult) {
const sortMap = {
inviteBidTime: 'invite_bid_time',
openBidTime: 'open_bid_time',
};
const { order, columnKey } = sortInfo;
return {
field: sortMap[columnKey],
order,
};
},
单元格列属性还需要配置
sorter: true,
sortDirections: ['descend', 'ascend'],
tableSetting
单独设置表格操作项。在showTableSetting
设置为true时生效。
export interface TableSetting {
redo?: boolean;
size?: boolean;
setting?: boolean;
fullScreen?: boolean;
}
showTableSetting: true,
tableSetting: {
redo: true,
setting: false,
},
api
defSort, sortFn, pageSize, page等等参会被作为请求参数。
*
* 会将defSort, pageSize, page传入,作为默认值
*/
api: (params) => {
console.log('============', params);
return demoListApi(params);
},
beforeFetch 处理请求数据
beforeFetch(params) {
console.log('params', params);
},
afterFetch 处理响应数据
afterFetch(params) {
console.log('params====响应', params);
},
pagination 分页
可以用于设置不使用分页的数据,我只想要设置总数据。用于dataSource,注意pageSize默认值是20。数据量超过20,就会自动分页,所以我们需要将pageSize设置大一点。
export interface PaginationProps {
* total number of data items
* @default 0
* @type number
*/
total?: number;
* default initial page number
* @default 1
* @type number
*/
defaultCurrent?: number;
* current page number
* @type number
*/
current?: number;
* default number of data items per page
* @default 10
* @type number
*/
defaultPageSize?: number;
* number of data items per page
* @type number
*/
pageSize?: number;
* Whether to hide pager on single page
* @default false
* @type boolean
*/
hideOnSinglePage?: boolean;
* determine whether pageSize can be changed
* @default false
* @type boolean
*/
showSizeChanger?: boolean;
* specify the sizeChanger options
* @default ['10', '20', '30', '40']
* @type string[]
*/
pageSizeOptions?: string[];
* determine whether you can jump to pages directly
* @default false
* @type boolean
*/
showQuickJumper?: boolean | object;
* to display the total number and range
* @type Function
*/
showTotal?: (total: number, range: [number, number]) => any;
* specify the size of Pagination, can be set to small
* @default ''
* @type string
*/
size?: string;
* whether to setting simple mode
* @type boolean
*/
simple?: boolean;
* to customize item innerHTML
* @type Function
*/
itemRender?: (props: PaginationRenderProps) => VNodeChild | JSX.Element;
}
BasicColumn 列配置
export interface BasicColumn extends ColumnProps {
children?: BasicColumn[];
filters?: {
text: string;
value: string;
children?:
| unknown[]
| (((props: Record<string, unknown>) => unknown[]) & (() => unknown[]) & (() => unknown[]));
}[];
flag?: 'INDEX' | 'DEFAULT' | 'CHECKBOX' | 'RADIO' | 'ACTION';
customTitle?: VueNode;
slots?: Recordable;
defaultHidden?: boolean;
helpMessage?: string | string[];
format?: CellFormat;
edit?: boolean;
editRow?: boolean;
editable?: boolean;
editComponent?: ComponentType;
editComponentProps?: Recordable;
* {
required: true,
validator: async (rule, value) => {
if (!value) {
return Promise.reject('请输入xxx');
}
if (value && value.length > 100) {
return Promise.reject('最大不能超过100个字');
}
return Promise.resolve();
},
trigger: 'change',
},
*/
editRule?: boolean | ((text: string, record: Recordable) => Promise<string>);
editValueMap?: (value: any) => string;
onEditRow?: () => void;
auth?: RoleEnum | RoleEnum[] | string | string[];
ifShow?: boolean | ((column: BasicColumn) => boolean);
}
export interface ColumnProps<T> {
* specify how content is aligned
* @default 'left'
* @type string
*/
align?: 'left' | 'right' | 'center';
* ellipsize cell content, not working with sorter and filters for now.
* tableLayout would be fixed when ellipsis is true.
* @default false
* @type boolean
*/
ellipsis?: boolean;
* Span of this column's title
* 表头合并
* @type number
*/
colSpan?: number;
* Display field of the data record, could be set like a.b.c
*
* 列数据在数据项中对应的 key,支持 a.b.c 的嵌套写法
*
* @type string
*/
dataIndex?: string;
* Default filtered values
* @type string[]
*/
defaultFilteredValue?: string[];
* Default order of sorted values: 'ascend' 'descend' null
*
* 默认排序规则
*
* @type string
*/
defaultSortOrder?: SortOrder;
* Customized filter overlay
* @type any (slot)
*/
filterDropdown?:
| VNodeChild
| JSX.Element
| ((props: FilterDropdownProps) => VNodeChild | JSX.Element);
* Whether filterDropdown is visible
* @type boolean
*/
filterDropdownVisible?: boolean;
* Whether the dataSource is filtered
* @default false
* @type boolean
*/
filtered?: boolean;
* Controlled filtered value, filter icon will highlight
* @type string[]
*/
filteredValue?: string[];
* Customized filter icon
* @default false
* @type any
*/
filterIcon?: boolean | VNodeChild | JSX.Element;
* Whether multiple filters can be selected
* @default true
* @type boolean
*/
filterMultiple?: boolean;
* Filter menu config
* @type object[]
*/
filters?: ColumnFilterItem[];
* Set column to be fixed: true(same as left) 'left' 'right'
*
* 列是否固定,可选 true(等效于 left) 'left' 'right'
* @default false
* @type boolean | string
*/
fixed?: boolean | 'left' | 'right';
* Unique key of this column, you can ignore this prop if you've set a unique dataIndex
* vue绑定的key 如果已经设置了唯一的 dataIndex,可以忽略这个属性
* @type string
*/
key?: string;
* Renderer of the table cell. The return value should be a VNode, or an object for colSpan/rowSpan config
*
* 生成复杂数据的渲染函数,参数分别为当前行的值,当前行数据,行索引,@return 里面可以设置表格行/列合并,可参考 demo 表格行/列合并
*
* @type Function | ScopedSlot
*/
customRender?: CustomRenderFunction<T> | VNodeChild | JSX.Element;
* Sort function for local sort, see Array.sort's compareFunction. If you need sort buttons only, set to true
*
* 开启排序
* @type boolean | Function
*/
sorter?: boolean | Function;
* Order of sorted values: 'ascend' 'descend' false
* @type boolean | string
*/
sortOrder?: boolean | SortOrder;
* supported sort way, could be 'ascend', 'descend'
* @default ['ascend', 'descend']
* @type string[]
*/
sortDirections?: SortOrder[];
* Title of this column
* @type any (string | slot)
*/
title?: VNodeChild | JSX.Element;
* Width of this column
* @type string | number
*/
width?: string | number;
* Set props on per cell
* 设置单元格属性 格式化单元格数据(并不是,没啥效果)
* @type Function
*/
customCell?: (record: T, rowIndex: number) => object;
* Set props on per header cell
* 设置表头属性 外层设计的会覆盖customHeaderCell返回的
* @type object
*/
customHeaderCell?: (column: ColumnProps<T>) => object;
* Callback executed when the confirm filter button is clicked, Use as a filter event when using template or jsx
* @type Function
*/
onFilter?: (value: any, record: T) => boolean;
* Callback executed when filterDropdownVisible is changed, Use as a filterDropdownVisible event when using template or jsx
* @type Function
*/
onFilterDropdownVisibleChange?: (visible: boolean) => void;
* When using columns, you can setting this property to configure the properties that support the slot,
* such as slots: { filterIcon: 'XXX'}
* {
* title?: string;
filterIcon?: string;
filterDropdown?: string;
customRender?: string;
[key: string]: string | undefined;
* }
一般使用customRender设置插槽名称即可。
* @type object
*/
slots?: Recordable<string>;
}
format
可以拿到当前单元格和行的记录,做一些处理。
export type CellFormat =
| string
| ((text: string, record: Recordable, index: number) => string | number)
| Map<string | number, any>;
format?: CellFormat;
format(text) {
return text ? dayjs(text).format('YYYY-MM-DD') : '';
},
basicTable插槽
useForm返回的配置对象
setProps,
setFieldsValue,
updateSchema,
scrollToField,
resetSchema,
clearValidate,
resetFields,
removeSchemaByFiled,
getFieldsValue,
appendSchemaByField,
validateFields,
validate,
submit,
useForm 参数
export interface FormProps {
layout?: 'vertical' | 'inline' | 'horizontal';
model?: Recordable;
labelWidth?: number | string;
labelAlign?: 'left' | 'right';
rowProps?: RowProps;
submitOnReset?: boolean;
labelCol?: Partial<ColEx>;
wrapperCol?: Partial<ColEx>;
baseRowStyle?: CSSProperties;
baseColProps?: Partial<ColEx>;
schemas?: FormSchema[];
mergeDynamicData?: Recordable;
compact?: boolean;
emptySpan?: number | Partial<ColEx>;
size?: 'default' | 'small' | 'large';
disabled?: boolean;
* fieldMapToTime: [
// datetime为时间组件在表单内的字段,startTime,endTime为转化后的开始时间与结束时间
// 'YYYY-MM-DD'为时间格式,参考moment
['datetime', ['startTime', 'endTime'], 'YYYY-MM-DD'],
// 支持多个字段
['datetime1', ['startTime1', 'endTime1'], 'YYYY-MM-DD HH:mm:ss'],
提交后获取表单数据就会有startTime,endTime字段
],
*/
fieldMapToTime?: FieldMapToTime;
autoSetPlaceHolder?: boolean;
autoSubmitOnEnter?: boolean;
rulesMessageJoinLabel?: boolean;
showAdvancedButton?: boolean;
autoFocusFirstItem?: boolean;
autoAdvancedLine?: number;
alwaysShowLines?: number;
showActionButtonGroup?: boolean;
resetButtonOptions?: Partial<ButtonProps>;
submitButtonOptions?: Partial<ButtonProps>;
actionColOptions?: Partial<ColEx>;
showResetButton?: boolean;
showSubmitButton?: boolean;
resetFunc?: () => Promise<void>;
submitFunc?: () => Promise<void>;
transformDateFunc?: (date: any) => string;
colon?: boolean;
}
labelCol
export interface ColEx {
style?: any;
* raster number of cells to occupy, 0 corresponds to display: none
* @default none (0)
* @type ColSpanType
*/
span?: ColSpanType;
* raster order, used in flex layout mode
* @default 0
* @type ColSpanType
*/
order?: ColSpanType;
* the layout fill of flex
* @default none
* @type ColSpanType
*/
flex?: ColSpanType;
* the number of cells to offset Col from the left
* @default 0
* @type ColSpanType
*/
offset?: ColSpanType;
* the number of cells that raster is moved to the right
* @default 0
* @type ColSpanType
*/
push?: ColSpanType;
* the number of cells that raster is moved to the left
* @default 0
* @type ColSpanType
*/
pull?: ColSpanType;
* <576px and also default setting, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
xs?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
* ≥576px, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
sm?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
* ≥768px, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
md?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
* ≥992px, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
lg?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
* ≥1200px, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
xl?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
* ≥1600px, could be a span value or an object containing above props
* @type { span: ColSpanType, offset: ColSpanType } | ColSpanType
*/
xxl?: { span: ColSpanType; offset: ColSpanType } | ColSpanType;
}
按钮相关操作
submitButtonOptions, submitFunc
,如果有不需要提交和重置的功能,而是需要其他按钮的功能,我们就可以定义按钮文本并实现对应的逻辑。一个障眼法 也可以使用插槽。
resetButtonOptions?: Partial<ButtonProps>;
submitButtonOptions?: Partial<ButtonProps>;
actionColOptions?: Partial<ColEx>;
showResetButton?: boolean;
showSubmitButton?: boolean;
resetFunc?: () => Promise<void>;
submitFunc?: () => Promise<void>;
插槽
名称 | 说明 |
---|---|
formFooter | 表单底部区域 |
formHeader | 表单顶部区域 |
resetBefore | 重置按钮前 |
submitBefore | 提交按钮前 |
advanceBefore | 展开按钮前 |
advanceAfter | 展开按钮后 |
表单项配置列表 SchemaForm
export interface FormSchema {
field: string;
changeEvent?: string;
* componentProps({ formModel }) {
return {
showSearch: true,
api: queryCountryCode,
labelField: 'cn',
valueField: 'id',
onChange: (_) => {
formModel.province = null;
// formModel.city = null;
},
};
},
*/
valueField?: string;
label: string | VNode;
subLabel?: string;
helpMessage?:
| string
| string[]
| ((renderCallbackParams: RenderCallbackParams) => string | string[]);
helpComponentProps?: Partial<HelpComponentProps>;
labelWidth?: string | number;
disabledLabelWidth?: boolean;
component: ComponentType;
* component: 'ApiSelect',
componentProps(props) {
return {
showSearchcomponentProps: true,
labelField: 'productName',
valueField: 'productCode',
resultField: 'results',
api: (params) =>
fetchProductPage(
Object.assign(params, {
pageReq: {
pageSize: 500,
pageNo: 1,
},
}),
),
onChange(val, options) {
// options是请求接口的原始数据
props.formModel.productModel = options.productModel;
props.formModel.productType = options.productType;
},
};
},
*/
componentProps?:
| ((opt: {
schema: FormSchema;
tableAction: TableActionType;
formActionType: FormActionType;
formModel: Recordable;
}) => Recordable)
| object;
required?: boolean | ((renderCallbackParams: RenderCallbackParams) => boolean);
suffix?: string | number | ((values: RenderCallbackParams) => string | number);
* rules: [
{
required: true,
validator: async (rule, value) => {
if (!value) {
return Promise.reject('请输入xxx');
}
if (value && value.length > 100) {
return Promise.reject('最大不能超过100个字');
}
return Promise.resolve();
},
trigger: 'change',
},
],
*/
rules?: Rule[];
rulesMessageJoinLabel?: boolean;
itemProps?: Partial<FormItem>;
colProps?: Partial<ColEx>;
defaultValue?: any;
isAdvanced?: boolean;
span?: number;
ifShow?: boolean | ((renderCallbackParams: RenderCallbackParams) => boolean);
show?: boolean | ((renderCallbackParams: RenderCallbackParams) => boolean);
render?: (renderCallbackParams: RenderCallbackParams) => VNode | VNode[] | string;
renderColContent?: (renderCallbackParams: RenderCallbackParams) => VNode | VNode[] | string;
renderComponentContent?:
| ((renderCallbackParams: RenderCallbackParams) => any)
| VNode
| VNode[]
| string;
slot?: string;
colSlot?: string;
dynamicDisabled?: boolean | ((renderCallbackParams: RenderCallbackParams) => boolean);
dynamicRules?: (renderCallbackParams: RenderCallbackParams) => Rule[];
}
ifShow
做一些表单值联动隐藏表单项处理。
ifShow(payload) {
}
ifShow(payload) {
if (payload.model.type === '1') {
return true;
} else {
return false;
}
},
componentProps 所渲染的组件的 props
-
当值为对象类型时,该对象将作为
component
所对应组件的的 props 传入组件。就是ant-design-vue中组件对应的props。 -
当值为一个函数时候参数是一个对象有 4 个属性。但是一般使用
formModel, schema
就可以了。做变动联动处理就可以使用到。-
schema
: 表单的整个 schemas -
formActionType
: 操作表单的函数。与 useForm 返回的操作函数一致 -
formModel
: 表单的双向绑定对象,这个值是响应式的。所以可以方便处理很多操作 -
tableAction
: 操作表格的函数,与 useTable 返回的操作函数一致。注意该参数只在表格内开启搜索表单的时候有值,其余情况为null
。
-
component: 'ApiSelect',
componentProps(props) {
return {
showSearchcomponentProps: true,
labelField: 'productName',
valueField: 'productCode',
resultField: 'results',
api: (params) =>
fetchProductPage(
Object.assign(params, {
pageReq: {
pageSize: 500,
pageNo: 1,
},
}),
),
onChange(val, options) {
props.formModel.productModel = options.productModel;
props.formModel.productType = options.productType;
},
};
}
更多推荐
所有评论(0)