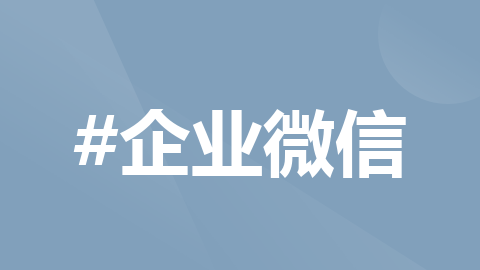
企业微信开发:一步一步搞定消息接收与推送(山东通消息推送)
一步步带大家配置企业的消息推送和接收,简单开发

背景
最近接手一个政府项目,要接入“山东通”app应用,实现消息的接收和推送。
对接方给了一堆文档,看的稀里糊涂的。
最终发现文档和企业微信的文档完全一致。
那不如先搞定企业微信的消息推送。
企业微信
注册
首先注册一个企业微信,没有企业一样可以注册。链接:注册地址
创建应用
登录企业微信之后,找到应用管理,创建自己的应用。
点击刚刚创建的应用,可以看到如下界面:
这里有几个重要的参数。
- AgentId
- Secret
这2个参数在后面开发中会用到。
可见范围可以选择本企业的成员或者部门。
添加的用户可以看到账号信息,发送消息的时候会用到。
获取token
查看企业微信提供的消息发送的接口文档,地址如下:接口地址
可以看到,企业微信提供了消息发送的接口,注意里面有一个参数是access_token。
OK,发送消息之前我们要先获取access_token。
先查看获取access_token的文档。接口地址。
看上面文档,得出结论:
- 接口地址:https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=ID&corpsecret=SECRET
- 参数corpid: 企业ID
- 参数corpsecret: 应用凭证密钥
先找到企业ID,点击“我的企业”,查看企业信息。
点击“应用管理”,点击自己新建的应用,找到上面提到的重要信息Secret,就是corpsecret。
用postman等API工具调用接口获取token试试。
可以看到获取token成功。
也可以编写代码获取token,java代码如下:
private static String getAccessToken() throws IOException {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=" + CORP_ID + "&corpsecret=" + AGENT_SECRET)
.get()
.build();
Response response = client.newCall(request).execute();
String responseBody = response.body().string();
JsonObject jsonObject = new Gson().fromJson(responseBody, JsonObject.class);
String accessToken = jsonObject.get("access_token").getAsString();
return accessToken;
}
使用到了OKhttp,需要在pom引入:
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.14.7</version>
</dependency>
接收消息设置
获取access_token之后,调用发送消息接口,失败了。
返回错误值如下:
{
"errcode": 60020,
"errmsg": "not allow to access from your ip, hint: [1695214342431490144412769], from ip: 113.*.*.251, more info at https://open.work.weixin.qq.com/devtool/query?e=60020"
}
错误大意:不安全的访问IP。
并给了一个地址,https://open.work.weixin.qq.com/devtool/query?e=60020。
意思就是需要我们配置“企业可信IP”。
在应用管理界面,拖到最底下可以看到“企业可信IP”,点击进去,竟然无法配置,因为必须先配置可信域名或者设置消息服务器URL。
服了,那就先配置 “设置消息服务器URL”。
在应用管理界面,找到“接收消息”,点击“设置API接收”。
进入API接收消息设置页面。
可以看到需要配置一个URL。
点击获取帮助,可以看到提供的帮助信息。
看了半天,反正没怎么懂,幸亏找到了企业微信提供的代码库。地址如下:开发资源。
在代码库有已有库,提供了c++ 、python、php、java、golang、c#等语言版本,下载地址。
根据提供的代码库,写了一个可以接收消息验证的URL。代码如下:
- controller
@RestController
@RequestMapping("msg")
public class MsgController {
@Autowired
private AppInfo appInfo;
@GetMapping("/receiveMsg")
public String receiveMsg(String msg_signature, String timestamp, String nonce, String echostr) throws UnsupportedEncodingException {
System.out.println(appInfo.getsCorpID());
echostr = MsgUtil.handleEchoStr(echostr);
String res = null;
try {
res = MsgUtil.getEchoStr(appInfo, msg_signature, timestamp, nonce, echostr);
} catch (AesException e) {
throw new RuntimeException(e);
}
return res;
}
}
- AppInfo:配置类,配置了前面“api接收消息”页面的需要的Token和EncodingAESKey参数,这2个参数可以随机获取之后,放到自己程序里面配置好。
@Component
//prefix前缀需要和yml配置文件里的匹配。
@ConfigurationProperties(prefix = "app")
public class AppInfo {
private String sToken;
private String sCorpID;
private String sEncodingAESKey;
}
- msgUtil :工具类,调用了企业微信提供的工具。
@Component
public class MsgUtil {
/**
* 处理echostr
*
* @param verifyEchoStr
* @return
* @throws UnsupportedEncodingException
*/
public static String handleEchoStr(String verifyEchoStr) throws UnsupportedEncodingException {
String enStr = URLEncoder.encode(verifyEchoStr, "UTF-8");
String reStr = enStr.replace("+", "%2B");
String echostr = URLDecoder.decode(reStr, "UTF-8");
return echostr;
}
/**
*
* @param appInfo
* @param msg_signature
* @param timestamp
* @param nonce
* @param echostr
* @return
* @throws AesException
*/
public static String getEchoStr(AppInfo appInfo, String msg_signature, String timestamp, String nonce, String echostr) throws AesException {
String sToken = appInfo.getsToken();
String sCorpID = appInfo.getsCorpID();
String sEncodingAESKey = appInfo.getsEncodingAESKey();
WXBizMsgCrypt wxcpt = new WXBizMsgCrypt(sToken, sEncodingAESKey, sCorpID);
String sEchoStr = null; //需要返回的明文
try {
sEchoStr = wxcpt.VerifyURL(msg_signature, timestamp,
nonce, echostr);
System.out.println("verifyurl echostr: " + sEchoStr);
// 验证URL成功,将sEchoStr返回
// HttpUtils.SetResponse(sEchoStr);
} catch (Exception e) {
//验证URL失败,错误原因请查看异常
e.printStackTrace();
}
return sEchoStr;
}
}
程序部署之后,配置API接收消息:
如果调用失败或者参数(token或EncodingAESKey)配置的有问题,会提示如下:
成功如下:
企业可信IP
再次点击“企业可信IP”,配置IP,可以看到可以配置了。
配置的IP就是要调用发送消息的电脑公网IP,最多可以配置120个。
一般配置部署的服务器IP就可以了。
消息发送
再次调用接口发送消息。
服了,终于成功了。
打开手机,看到企业微信有刚才发送的消息了。
发送消息代码如下:
public static void sendMsg() throws IOException {
OkHttpClient client = new OkHttpClient();
String accessToken = getAccessToken();
System.out.println(accessToken);
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"touser\" : \"2a4ace25deb05ddd9871dcbaaf4e712c|iskylei\",\"msgtype\" : \"text\",\"agentid\" : " + AGENT_ID + ",\"text\" : {\"content\" : \"Hello, World!\"},\"safe\":0}");
Request request = new Request.Builder()
.url(API_URL + "?access_token=" + accessToken)
.post(body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
}
更多推荐
所有评论(0)